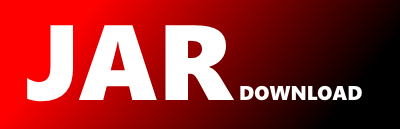
com.gempukku.libgdx.graph.pipeline.producer.postprocessor.GaussianBlurPipelineNodeProducer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of libgdx-graph Show documentation
Show all versions of libgdx-graph Show documentation
libGDX-graph runtime library for pipeline rendering
The newest version!
package com.gempukku.libgdx.graph.pipeline.producer.postprocessor;
import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.graphics.GL20;
import com.badlogic.gdx.graphics.g3d.utils.RenderContext;
import com.badlogic.gdx.graphics.glutils.ShaderProgram;
import com.badlogic.gdx.math.MathUtils;
import com.badlogic.gdx.utils.JsonValue;
import com.badlogic.gdx.utils.ObjectMap;
import com.gempukku.libgdx.graph.pipeline.RenderPipeline;
import com.gempukku.libgdx.graph.pipeline.RenderPipelineBuffer;
import com.gempukku.libgdx.graph.pipeline.config.postprocessor.GaussianBlurPipelineNodeConfiguration;
import com.gempukku.libgdx.graph.pipeline.producer.FloatFieldOutput;
import com.gempukku.libgdx.graph.pipeline.producer.FullScreenRender;
import com.gempukku.libgdx.graph.pipeline.producer.PipelineRenderingContext;
import com.gempukku.libgdx.graph.pipeline.producer.node.OncePerFrameJobPipelineNode;
import com.gempukku.libgdx.graph.pipeline.producer.node.PipelineNode;
import com.gempukku.libgdx.graph.pipeline.producer.node.PipelineNodeProducerImpl;
import com.gempukku.libgdx.graph.pipeline.producer.node.PipelineRequirements;
public class GaussianBlurPipelineNodeProducer extends PipelineNodeProducerImpl {
public GaussianBlurPipelineNodeProducer() {
super(new GaussianBlurPipelineNodeConfiguration());
}
@Override
public PipelineNode createNodeForSingleInputs(JsonValue data, ObjectMap> inputFields) {
final ShaderProgram shaderProgram = new ShaderProgram(
Gdx.files.classpath("shader/viewToScreenCoords.vert"),
Gdx.files.classpath("shader/gaussianBlur.frag"));
if (!shaderProgram.isCompiled())
throw new IllegalArgumentException("Error compiling shader: " + shaderProgram.getLog());
final PipelineNode.FieldOutput processorEnabled = (PipelineNode.FieldOutput) inputFields.get("enabled");
PipelineNode.FieldOutput blurRadius = (PipelineNode.FieldOutput) inputFields.get("blurRadius");
if (blurRadius == null)
blurRadius = new FloatFieldOutput(0f);
final PipelineNode.FieldOutput renderPipelineInput = (PipelineNode.FieldOutput) inputFields.get("input");
final PipelineNode.FieldOutput finalBlurRadius = blurRadius;
return new OncePerFrameJobPipelineNode(configuration, inputFields) {
@Override
protected void executeJob(PipelineRenderingContext pipelineRenderingContext, PipelineRequirements pipelineRequirements, ObjectMap outputValues) {
RenderPipeline renderPipeline = renderPipelineInput.getValue(pipelineRenderingContext, pipelineRequirements);
boolean enabled = processorEnabled == null || processorEnabled.getValue(pipelineRenderingContext, null);
int blurRadius = MathUtils.round(finalBlurRadius.getValue(pipelineRenderingContext, null));
if (enabled && blurRadius > 0) {
float[] kernel = GaussianBlurKernel.getKernel(blurRadius);
RenderPipelineBuffer currentBuffer = renderPipeline.getDefaultBuffer();
RenderContext renderContext = pipelineRenderingContext.getRenderContext();
renderContext.setDepthTest(0);
renderContext.setDepthMask(false);
renderContext.setBlending(false, 0, 0);
renderContext.setCullFace(GL20.GL_BACK);
shaderProgram.bind();
shaderProgram.setUniformi("u_blurRadius", blurRadius);
shaderProgram.setUniformf("u_pixelSize", 1f / currentBuffer.getWidth(), 1f / currentBuffer.getHeight());
shaderProgram.setUniform1fv("u_kernel", kernel, 0, kernel.length);
shaderProgram.setUniformi("u_vertical", 1);
RenderPipelineBuffer tempBuffer = executeBlur(shaderProgram, renderPipeline, currentBuffer, pipelineRenderingContext.getRenderContext(), pipelineRenderingContext.getFullScreenRender());
shaderProgram.setUniformi("u_vertical", 0);
RenderPipelineBuffer finalBuffer = executeBlur(shaderProgram, renderPipeline, tempBuffer, pipelineRenderingContext.getRenderContext(), pipelineRenderingContext.getFullScreenRender());
renderPipeline.returnFrameBuffer(tempBuffer);
renderPipeline.swapColorTextures(currentBuffer, finalBuffer);
renderPipeline.returnFrameBuffer(finalBuffer);
}
OutputValue output = outputValues.get("output");
if (output != null)
output.setValue(renderPipeline);
}
@Override
public void dispose() {
shaderProgram.dispose();
}
};
}
private RenderPipelineBuffer executeBlur(ShaderProgram shaderProgram, RenderPipeline renderPipeline, RenderPipelineBuffer sourceBuffer,
RenderContext renderContext, FullScreenRender fullScreenRender) {
RenderPipelineBuffer resultBuffer = renderPipeline.getNewFrameBuffer(sourceBuffer);
resultBuffer.beginColor();
shaderProgram.setUniformi("u_sourceTexture", renderContext.textureBinder.bind(sourceBuffer.getColorBufferTexture()));
fullScreenRender.renderFullScreen(shaderProgram);
resultBuffer.endColor();
return resultBuffer;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy