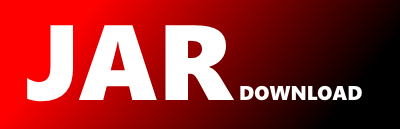
com.generallycloud.baseio.component.ByteArrayInputStream Maven / Gradle / Ivy
/*
* Copyright 2015-2017 GenerallyCloud.com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.generallycloud.baseio.component;
import java.io.IOException;
import java.io.InputStream;
public class ByteArrayInputStream extends InputStream {
/**
* An array of bytes that was provided by the creator of the stream.
* Elements buf[0]
through buf[count-1]
are the
* only bytes that can ever be read from the stream; element
* buf[pos]
is the next byte to be read.
*/
protected byte buf[];
/**
* The index of the next character to read from the input stream buffer.
* This value should always be nonnegative and not larger than the value of
* count
. The next byte to be read from the input stream
* buffer will be buf[pos]
.
*/
protected int pos;
/**
* The currently marked position in the stream. ByteArrayInputStream
* objects are marked at position zero by default when constructed. They
* may be marked at another position within the buffer by the
* mark()
method. The current buffer position is set to this
* point by the reset()
method.
*
* If no mark has been set, then the value of mark is the offset passed to
* the constructor (or 0 if the offset was not supplied).
*
* @since JDK1.1
*/
protected int mark = 0;
/**
* The index one greater than the last valid character in the input stream
* buffer. This value should always be nonnegative and not larger than the
* length of buf
. It is one greater than the position of the
* last byte within buf
that can ever be read from the input
* stream buffer.
*/
protected int count;
/**
* Creates a ByteArrayInputStream
so that it uses
* buf
as its buffer array. The buffer array is not copied.
* The initial value of pos
is 0
and the initial
* value of count
is the length of buf
.
*
* @param buf
* the input buffer.
*/
public ByteArrayInputStream(byte buf[]) {
this.buf = buf;
this.pos = 0;
this.count = buf.length;
}
/**
* Creates ByteArrayInputStream
that uses buf
as
* its buffer array. The initial value of pos
is
* offset
and the initial value of count
is the
* minimum of offset+length
and buf.length
. The
* buffer array is not copied. The buffer's mark is set to the specified
* offset.
*
* @param buf
* the input buffer.
* @param offset
* the offset in the buffer of the first byte to read.
* @param length
* the maximum number of bytes to read from the buffer.
*/
public ByteArrayInputStream(byte buf[], int offset, int length) {
this.buf = buf;
this.pos = offset;
this.count = Math.min(offset + length, buf.length);
this.mark = offset;
}
/**
* Reads the next byte of data from this input stream. The value byte is
* returned as an int
in the range 0
to
* 255
. If no byte is available because the end of the stream
* has been reached, the value -1
is returned.
*
* This read
method cannot block.
*
* @return the next byte of data, or -1
if the end of the
* stream has been reached.
*/
@Override
public int read() {
return (pos < count) ? (buf[pos++] & 0xff) : -1;
}
/**
* Reads up to len
bytes of data into an array of bytes from
* this input stream. If pos
equals count
, then
* -1
is returned to indicate end of file. Otherwise, the
* number k
of bytes read is equal to the smaller of
* len
and count-pos
. If k
is
* positive, then bytes buf[pos]
through
* buf[pos+k-1]
are copied into b[off]
through
* b[off+k-1]
in the manner performed by
* System.arraycopy
. The value k
is added into
* pos
and k
is returned.
*
* This read
method cannot block.
*
* @param b
* the buffer into which the data is read.
* @param off
* the start offset in the destination array b
* @param len
* the maximum number of bytes read.
* @return the total number of bytes read into the buffer, or
* -1
if there is no more data because the end of the
* stream has been reached.
* @exception NullPointerException
* If b
is null
.
* @exception IndexOutOfBoundsException
* If off
is negative, len
is
* negative, or len
is greater than
* b.length - off
*/
@Override
public int read(byte b[], int off, int len) {
if (b == null) {
throw new NullPointerException();
} else if (off < 0 || len < 0 || len > b.length - off) {
throw new IndexOutOfBoundsException();
}
if (pos >= count) {
return -1;
}
int avail = count - pos;
if (len > avail) {
len = avail;
}
if (len <= 0) {
return 0;
}
System.arraycopy(buf, pos, b, off, len);
pos += len;
return len;
}
/**
* Skips n
bytes of input from this input stream. Fewer bytes
* might be skipped if the end of the input stream is reached. The actual
* number k
of bytes to be skipped is equal to the smaller of
* n
and count-pos
. The value k
is
* added into pos
and k
is returned.
*
* @param n
* the number of bytes to be skipped.
* @return the actual number of bytes skipped.
*/
@Override
public long skip(long n) {
long k = count - pos;
if (n < k) {
k = n < 0 ? 0 : n;
}
pos += k;
return k;
}
/**
* Returns the number of remaining bytes that can be read (or skipped over)
* from this input stream.
*
* The value returned is count - pos
, which is the number
* of bytes remaining to be read from the input buffer.
*
* @return the number of remaining bytes that can be read (or skipped over)
* from this input stream without blocking.
*/
@Override
public int available() {
return count - pos;
}
/**
* Tests if this InputStream
supports mark/reset. The
* markSupported
method of ByteArrayInputStream
* always returns true
.
*
* @since JDK1.1
*/
@Override
public boolean markSupported() {
return true;
}
/**
* Set the current marked position in the stream. ByteArrayInputStream
* objects are marked at position zero by default when constructed. They
* may be marked at another position within the buffer by this method.
*
* If no mark has been set, then the value of the mark is the offset passed
* to the constructor (or 0 if the offset was not supplied).
*
*
* Note: The readAheadLimit
for this class has no meaning.
*
* @since JDK1.1
*/
@Override
public void mark(int readAheadLimit) {
mark = pos;
}
/**
* Resets the buffer to the marked position. The marked position is 0
* unless another position was marked or an offset was specified in the
* constructor.
*/
@Override
public void reset() {
pos = mark;
}
/**
* Closing a ByteArrayInputStream has no effect. The methods in
* this class can be called after the stream has been closed without
* generating an IOException.
*/
@Override
public void close() throws IOException {}
/**
* @return the buf
*/
public byte[] getBuf() {
return buf;
}
/**
* @return the pos
*/
public int getPos() {
return pos;
}
/**
* @return the count
*/
public int getCount() {
return count;
}
}