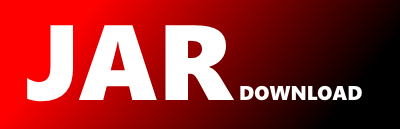
com.genesys.internal.authentication.api.AuthenticationApi Maven / Gradle / Ivy
The newest version!
/*
* Authentication API
* Authentication API
*
* OpenAPI spec version: 9.0.000.00.872
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.genesys.internal.authentication.api;
import com.genesys.internal.common.ApiCallback;
import com.genesys.internal.common.ApiClient;
import com.genesys.internal.common.ApiException;
import com.genesys.internal.common.ApiResponse;
import com.genesys.internal.common.Configuration;
import com.genesys.internal.common.Pair;
import com.genesys.internal.common.ProgressRequestBody;
import com.genesys.internal.common.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import com.genesys.internal.authentication.model.AuthSchemeLookupData;
import com.genesys.internal.authentication.model.ChangePasswordOperation;
import com.genesys.internal.authentication.model.CloudUserDetails;
import com.genesys.internal.authentication.model.DefaultOAuth2AccessToken;
import com.genesys.internal.authentication.model.ErrorResponse;
import com.genesys.internal.authentication.model.ModelApiResponse;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class AuthenticationApi {
private ApiClient apiClient;
public AuthenticationApi() {
this(Configuration.getDefaultApiClient());
}
public AuthenticationApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for authorize
* @param responseType The response type to let the Authentication API know which grant flow you're using. Possible values are 'code' for Authorization Code Grant or 'token' for Implicit Grant. For more information about this parameter, see [Response Type](https://tools.ietf.org/html/rfc6749#section-3.1.1). (required)
* @param redirectUri The URI that you want users to be redirected to after entering valid credentials during an Implicit or Authorization Code grant. The Authentication includes this URI as part of the URI it returns in the 'Location' header. (required)
* @param clientId The ID of the application or service that is registered as the client. You'll need to get this value from your PureEngage Cloud representative. (required)
* @param authorization Basic authorization. For example: 'Authorization: Basic Y3...MQ==' (optional)
* @param scope Scope (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call authorizeCall(String responseType, String redirectUri, String clientId, String authorization, String scope, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/oauth/authorize";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (responseType != null)
localVarQueryParams.addAll(apiClient.parameterToPair("response_type", responseType));
if (scope != null)
localVarQueryParams.addAll(apiClient.parameterToPair("scope", scope));
if (redirectUri != null)
localVarQueryParams.addAll(apiClient.parameterToPair("redirect_uri", redirectUri));
if (clientId != null)
localVarQueryParams.addAll(apiClient.parameterToPair("client_id", clientId));
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call authorizeValidateBeforeCall(String responseType, String redirectUri, String clientId, String authorization, String scope, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'responseType' is set
if (responseType == null) {
throw new ApiException("Missing the required parameter 'responseType' when calling authorize(Async)");
}
// verify the required parameter 'redirectUri' is set
if (redirectUri == null) {
throw new ApiException("Missing the required parameter 'redirectUri' when calling authorize(Async)");
}
// verify the required parameter 'clientId' is set
if (clientId == null) {
throw new ApiException("Missing the required parameter 'clientId' when calling authorize(Async)");
}
com.squareup.okhttp.Call call = authorizeCall(responseType, redirectUri, clientId, authorization, scope, progressListener, progressRequestListener);
return call;
}
/**
* Perform authorization.
* Perform authorization based on the code grant type — either Authorization Code Grant or Implicit Grant. For more information, see [Authorization Endpoint](https://tools.ietf.org/html/rfc6749#section-3.1). **Note:** For the optional **scope** parameter, the Authorization API supports only the '*' value.
* @param responseType The response type to let the Authentication API know which grant flow you're using. Possible values are 'code' for Authorization Code Grant or 'token' for Implicit Grant. For more information about this parameter, see [Response Type](https://tools.ietf.org/html/rfc6749#section-3.1.1). (required)
* @param redirectUri The URI that you want users to be redirected to after entering valid credentials during an Implicit or Authorization Code grant. The Authentication includes this URI as part of the URI it returns in the 'Location' header. (required)
* @param clientId The ID of the application or service that is registered as the client. You'll need to get this value from your PureEngage Cloud representative. (required)
* @param authorization Basic authorization. For example: 'Authorization: Basic Y3...MQ==' (optional)
* @param scope Scope (optional)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void authorize(String responseType, String redirectUri, String clientId, String authorization, String scope) throws ApiException {
authorizeWithHttpInfo(responseType, redirectUri, clientId, authorization, scope);
}
/**
* Perform authorization.
* Perform authorization based on the code grant type — either Authorization Code Grant or Implicit Grant. For more information, see [Authorization Endpoint](https://tools.ietf.org/html/rfc6749#section-3.1). **Note:** For the optional **scope** parameter, the Authorization API supports only the '*' value.
* @param responseType The response type to let the Authentication API know which grant flow you're using. Possible values are 'code' for Authorization Code Grant or 'token' for Implicit Grant. For more information about this parameter, see [Response Type](https://tools.ietf.org/html/rfc6749#section-3.1.1). (required)
* @param redirectUri The URI that you want users to be redirected to after entering valid credentials during an Implicit or Authorization Code grant. The Authentication includes this URI as part of the URI it returns in the 'Location' header. (required)
* @param clientId The ID of the application or service that is registered as the client. You'll need to get this value from your PureEngage Cloud representative. (required)
* @param authorization Basic authorization. For example: 'Authorization: Basic Y3...MQ==' (optional)
* @param scope Scope (optional)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse authorizeWithHttpInfo(String responseType, String redirectUri, String clientId, String authorization, String scope) throws ApiException {
com.squareup.okhttp.Call call = authorizeValidateBeforeCall(responseType, redirectUri, clientId, authorization, scope, null, null);
return apiClient.execute(call);
}
/**
* Perform authorization. (asynchronously)
* Perform authorization based on the code grant type — either Authorization Code Grant or Implicit Grant. For more information, see [Authorization Endpoint](https://tools.ietf.org/html/rfc6749#section-3.1). **Note:** For the optional **scope** parameter, the Authorization API supports only the '*' value.
* @param responseType The response type to let the Authentication API know which grant flow you're using. Possible values are 'code' for Authorization Code Grant or 'token' for Implicit Grant. For more information about this parameter, see [Response Type](https://tools.ietf.org/html/rfc6749#section-3.1.1). (required)
* @param redirectUri The URI that you want users to be redirected to after entering valid credentials during an Implicit or Authorization Code grant. The Authentication includes this URI as part of the URI it returns in the 'Location' header. (required)
* @param clientId The ID of the application or service that is registered as the client. You'll need to get this value from your PureEngage Cloud representative. (required)
* @param authorization Basic authorization. For example: 'Authorization: Basic Y3...MQ==' (optional)
* @param scope Scope (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call authorizeAsync(String responseType, String redirectUri, String clientId, String authorization, String scope, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = authorizeValidateBeforeCall(responseType, redirectUri, clientId, authorization, scope, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for changePassword
* @param request request (required)
* @param authorization OAuth 2.0 Bearer Token. Example: \"Authorization: bearer a4b5da75-a584-4053-9227-0f0ab23ff06e\" (optional, default to bearer)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call changePasswordCall(ChangePasswordOperation request, String authorization, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = request;
// create path and map variables
String localVarPath = "/change-password";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call changePasswordValidateBeforeCall(ChangePasswordOperation request, String authorization, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'request' is set
if (request == null) {
throw new ApiException("Missing the required parameter 'request' when calling changePassword(Async)");
}
com.squareup.okhttp.Call call = changePasswordCall(request, authorization, progressListener, progressRequestListener);
return call;
}
/**
* Change password.
* Change the user's password.
* @param request request (required)
* @param authorization OAuth 2.0 Bearer Token. Example: \"Authorization: bearer a4b5da75-a584-4053-9227-0f0ab23ff06e\" (optional, default to bearer)
* @return ModelApiResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ModelApiResponse changePassword(ChangePasswordOperation request, String authorization) throws ApiException {
ApiResponse resp = changePasswordWithHttpInfo(request, authorization);
return resp.getData();
}
/**
* Change password.
* Change the user's password.
* @param request request (required)
* @param authorization OAuth 2.0 Bearer Token. Example: \"Authorization: bearer a4b5da75-a584-4053-9227-0f0ab23ff06e\" (optional, default to bearer)
* @return ApiResponse<ModelApiResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse changePasswordWithHttpInfo(ChangePasswordOperation request, String authorization) throws ApiException {
com.squareup.okhttp.Call call = changePasswordValidateBeforeCall(request, authorization, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Change password. (asynchronously)
* Change the user's password.
* @param request request (required)
* @param authorization OAuth 2.0 Bearer Token. Example: \"Authorization: bearer a4b5da75-a584-4053-9227-0f0ab23ff06e\" (optional, default to bearer)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call changePasswordAsync(ChangePasswordOperation request, String authorization, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = changePasswordValidateBeforeCall(request, authorization, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getInfo
* @param authorization The OAuth 2 bearer access token you received from `/auth/v3/oauth/token`. For example: \"Authorization: bearer a4b5da75-a584-4053-9227-0f0ab23ff06e\" (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getInfoCall(String authorization, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/userinfo";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getInfoValidateBeforeCall(String authorization, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling getInfo(Async)");
}
com.squareup.okhttp.Call call = getInfoCall(authorization, progressListener, progressRequestListener);
return call;
}
/**
* Get user information by access token.
* Get information about a user by their OAuth 2 access token.
* @param authorization The OAuth 2 bearer access token you received from `/auth/v3/oauth/token`. For example: \"Authorization: bearer a4b5da75-a584-4053-9227-0f0ab23ff06e\" (required)
* @return CloudUserDetails
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CloudUserDetails getInfo(String authorization) throws ApiException {
ApiResponse resp = getInfoWithHttpInfo(authorization);
return resp.getData();
}
/**
* Get user information by access token.
* Get information about a user by their OAuth 2 access token.
* @param authorization The OAuth 2 bearer access token you received from `/auth/v3/oauth/token`. For example: \"Authorization: bearer a4b5da75-a584-4053-9227-0f0ab23ff06e\" (required)
* @return ApiResponse<CloudUserDetails>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getInfoWithHttpInfo(String authorization) throws ApiException {
com.squareup.okhttp.Call call = getInfoValidateBeforeCall(authorization, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get user information by access token. (asynchronously)
* Get information about a user by their OAuth 2 access token.
* @param authorization The OAuth 2 bearer access token you received from `/auth/v3/oauth/token`. For example: \"Authorization: bearer a4b5da75-a584-4053-9227-0f0ab23ff06e\" (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getInfoAsync(String authorization, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getInfoValidateBeforeCall(authorization, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for retrieveToken
* @param grantType The grant type you use to implement authentication. (required)
* @param authorization Basic authorization. For example: 'Authorization: Basic Y3...MQ==' (optional)
* @param accept The media type the Authentication API should should use for the response. For example: 'Accept: application/x-www-form-urlencoded' (optional)
* @param scope Scope (optional)
* @param clientId The ID of the application or service that is registered as the client. You'll need to get this value from your PureEngage Cloud representative. (optional)
* @param refreshToken See [Refresh Token](https://tools.ietf.org/html/rfc6749#section-1.5) for details. (optional)
* @param username The agent's username. (optional)
* @param password The agent's password (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call retrieveTokenCall(String grantType, String authorization, String accept, String scope, String clientId, String refreshToken, String username, String password, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/oauth/token";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
if (accept != null)
localVarHeaderParams.put("Accept", apiClient.parameterToString(accept));
Map localVarFormParams = new HashMap();
if (grantType != null)
localVarFormParams.put("grant_type", grantType);
if (scope != null)
localVarFormParams.put("scope", scope);
if (clientId != null)
localVarFormParams.put("client_id", clientId);
if (refreshToken != null)
localVarFormParams.put("refresh_token", refreshToken);
if (username != null)
localVarFormParams.put("username", username);
if (password != null)
localVarFormParams.put("password", password);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/x-www-form-urlencoded"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call retrieveTokenValidateBeforeCall(String grantType, String authorization, String accept, String scope, String clientId, String refreshToken, String username, String password, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'grantType' is set
if (grantType == null) {
throw new ApiException("Missing the required parameter 'grantType' when calling retrieveToken(Async)");
}
com.squareup.okhttp.Call call = retrieveTokenCall(grantType, authorization, accept, scope, clientId, refreshToken, username, password, progressListener, progressRequestListener);
return call;
}
/**
* Retrieve access token.
* Retrieve an access token based on the grant type — Authorization Code Grant, Resource Owner Password Credentials Grant or Client Credentials Grant. For more information, see [Token Endpoint](https://tools.ietf.org/html/rfc6749). **Note:** For the optional **scope** parameter, the Authorization API supports only the '*' value.
* @param grantType The grant type you use to implement authentication. (required)
* @param authorization Basic authorization. For example: 'Authorization: Basic Y3...MQ==' (optional)
* @param accept The media type the Authentication API should should use for the response. For example: 'Accept: application/x-www-form-urlencoded' (optional)
* @param scope Scope (optional)
* @param clientId The ID of the application or service that is registered as the client. You'll need to get this value from your PureEngage Cloud representative. (optional)
* @param refreshToken See [Refresh Token](https://tools.ietf.org/html/rfc6749#section-1.5) for details. (optional)
* @param username The agent's username. (optional)
* @param password The agent's password (optional)
* @return DefaultOAuth2AccessToken
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public DefaultOAuth2AccessToken retrieveToken(String grantType, String authorization, String accept, String scope, String clientId, String refreshToken, String username, String password) throws ApiException {
ApiResponse resp = retrieveTokenWithHttpInfo(grantType, authorization, accept, scope, clientId, refreshToken, username, password);
return resp.getData();
}
/**
* Retrieve access token.
* Retrieve an access token based on the grant type — Authorization Code Grant, Resource Owner Password Credentials Grant or Client Credentials Grant. For more information, see [Token Endpoint](https://tools.ietf.org/html/rfc6749). **Note:** For the optional **scope** parameter, the Authorization API supports only the '*' value.
* @param grantType The grant type you use to implement authentication. (required)
* @param authorization Basic authorization. For example: 'Authorization: Basic Y3...MQ==' (optional)
* @param accept The media type the Authentication API should should use for the response. For example: 'Accept: application/x-www-form-urlencoded' (optional)
* @param scope Scope (optional)
* @param clientId The ID of the application or service that is registered as the client. You'll need to get this value from your PureEngage Cloud representative. (optional)
* @param refreshToken See [Refresh Token](https://tools.ietf.org/html/rfc6749#section-1.5) for details. (optional)
* @param username The agent's username. (optional)
* @param password The agent's password (optional)
* @return ApiResponse<DefaultOAuth2AccessToken>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse retrieveTokenWithHttpInfo(String grantType, String authorization, String accept, String scope, String clientId, String refreshToken, String username, String password) throws ApiException {
com.squareup.okhttp.Call call = retrieveTokenValidateBeforeCall(grantType, authorization, accept, scope, clientId, refreshToken, username, password, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Retrieve access token. (asynchronously)
* Retrieve an access token based on the grant type — Authorization Code Grant, Resource Owner Password Credentials Grant or Client Credentials Grant. For more information, see [Token Endpoint](https://tools.ietf.org/html/rfc6749). **Note:** For the optional **scope** parameter, the Authorization API supports only the '*' value.
* @param grantType The grant type you use to implement authentication. (required)
* @param authorization Basic authorization. For example: 'Authorization: Basic Y3...MQ==' (optional)
* @param accept The media type the Authentication API should should use for the response. For example: 'Accept: application/x-www-form-urlencoded' (optional)
* @param scope Scope (optional)
* @param clientId The ID of the application or service that is registered as the client. You'll need to get this value from your PureEngage Cloud representative. (optional)
* @param refreshToken See [Refresh Token](https://tools.ietf.org/html/rfc6749#section-1.5) for details. (optional)
* @param username The agent's username. (optional)
* @param password The agent's password (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call retrieveTokenAsync(String grantType, String authorization, String accept, String scope, String clientId, String refreshToken, String username, String password, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = retrieveTokenValidateBeforeCall(grantType, authorization, accept, scope, clientId, refreshToken, username, password, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for signIn
* @param username The agent's username, formatted as 'tenant\\username'. (required)
* @param password The agent's password. (required)
* @param saml Specifies whether to login using [Security Assertion Markup Language](https://en.wikipedia.org/wiki/Security_Assertion_Markup_Language) (SAML). (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call signInCall(String username, String password, Boolean saml, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/sign-in";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
if (username != null)
localVarFormParams.put("username", username);
if (password != null)
localVarFormParams.put("password", password);
if (saml != null)
localVarFormParams.put("saml", saml);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/x-www-form-urlencoded"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call signInValidateBeforeCall(String username, String password, Boolean saml, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'username' is set
if (username == null) {
throw new ApiException("Missing the required parameter 'username' when calling signIn(Async)");
}
// verify the required parameter 'password' is set
if (password == null) {
throw new ApiException("Missing the required parameter 'password' when calling signIn(Async)");
}
com.squareup.okhttp.Call call = signInCall(username, password, saml, progressListener, progressRequestListener);
return call;
}
/**
* Perform form-based authentication.
* Perform form-based authentication by submitting an agent's username and password.
* @param username The agent's username, formatted as 'tenant\\username'. (required)
* @param password The agent's password. (required)
* @param saml Specifies whether to login using [Security Assertion Markup Language](https://en.wikipedia.org/wiki/Security_Assertion_Markup_Language) (SAML). (optional)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void signIn(String username, String password, Boolean saml) throws ApiException {
signInWithHttpInfo(username, password, saml);
}
/**
* Perform form-based authentication.
* Perform form-based authentication by submitting an agent's username and password.
* @param username The agent's username, formatted as 'tenant\\username'. (required)
* @param password The agent's password. (required)
* @param saml Specifies whether to login using [Security Assertion Markup Language](https://en.wikipedia.org/wiki/Security_Assertion_Markup_Language) (SAML). (optional)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse signInWithHttpInfo(String username, String password, Boolean saml) throws ApiException {
com.squareup.okhttp.Call call = signInValidateBeforeCall(username, password, saml, null, null);
return apiClient.execute(call);
}
/**
* Perform form-based authentication. (asynchronously)
* Perform form-based authentication by submitting an agent's username and password.
* @param username The agent's username, formatted as 'tenant\\username'. (required)
* @param password The agent's password. (required)
* @param saml Specifies whether to login using [Security Assertion Markup Language](https://en.wikipedia.org/wiki/Security_Assertion_Markup_Language) (SAML). (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call signInAsync(String username, String password, Boolean saml, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = signInValidateBeforeCall(username, password, saml, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for signOut
* @param authorization The OAuth 2 bearer access token you received from `/auth/v3/oauth/token`. For example: \"Authorization: bearer a4b5da75-a584-4053-9227-0f0ab23ff06e\" (required)
* @param global Specifies whether to invalidate all tokens for the current user (`true`) or only the current token (`false`). (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call signOutCall(String authorization, Boolean global, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/sign-out";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (global != null)
localVarQueryParams.addAll(apiClient.parameterToPair("global", global));
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call signOutValidateBeforeCall(String authorization, Boolean global, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling signOut(Async)");
}
com.squareup.okhttp.Call call = signOutCall(authorization, global, progressListener, progressRequestListener);
return call;
}
/**
* Sign-out a logged in user.
* Sign-out the current user and invalidate either the current token or all tokens associated with the user.
* @param authorization The OAuth 2 bearer access token you received from `/auth/v3/oauth/token`. For example: \"Authorization: bearer a4b5da75-a584-4053-9227-0f0ab23ff06e\" (required)
* @param global Specifies whether to invalidate all tokens for the current user (`true`) or only the current token (`false`). (optional)
* @return ModelApiResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ModelApiResponse signOut(String authorization, Boolean global) throws ApiException {
ApiResponse resp = signOutWithHttpInfo(authorization, global);
return resp.getData();
}
/**
* Sign-out a logged in user.
* Sign-out the current user and invalidate either the current token or all tokens associated with the user.
* @param authorization The OAuth 2 bearer access token you received from `/auth/v3/oauth/token`. For example: \"Authorization: bearer a4b5da75-a584-4053-9227-0f0ab23ff06e\" (required)
* @param global Specifies whether to invalidate all tokens for the current user (`true`) or only the current token (`false`). (optional)
* @return ApiResponse<ModelApiResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse signOutWithHttpInfo(String authorization, Boolean global) throws ApiException {
com.squareup.okhttp.Call call = signOutValidateBeforeCall(authorization, global, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Sign-out a logged in user. (asynchronously)
* Sign-out the current user and invalidate either the current token or all tokens associated with the user.
* @param authorization The OAuth 2 bearer access token you received from `/auth/v3/oauth/token`. For example: \"Authorization: bearer a4b5da75-a584-4053-9227-0f0ab23ff06e\" (required)
* @param global Specifies whether to invalidate all tokens for the current user (`true`) or only the current token (`false`). (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call signOutAsync(String authorization, Boolean global, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = signOutValidateBeforeCall(authorization, global, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for tenantInfo
* @param lookupData Data for scheme lookup. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call tenantInfoCall(AuthSchemeLookupData lookupData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = lookupData;
// create path and map variables
String localVarPath = "/auth-scheme";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call tenantInfoValidateBeforeCall(AuthSchemeLookupData lookupData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = tenantInfoCall(lookupData, progressListener, progressRequestListener);
return call;
}
/**
* Get authentication scheme.
* Get the authentication scheme by user name or tenant name. The return value is 'saml' if the contact center has [Security Assertion Markup Language](https://en.wikipedia.org/wiki/Security_Assertion_Markup_Language) (SAML) enabled; otherwise, the return value is 'basic'.
* @param lookupData Data for scheme lookup. (optional)
* @return ModelApiResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ModelApiResponse tenantInfo(AuthSchemeLookupData lookupData) throws ApiException {
ApiResponse resp = tenantInfoWithHttpInfo(lookupData);
return resp.getData();
}
/**
* Get authentication scheme.
* Get the authentication scheme by user name or tenant name. The return value is 'saml' if the contact center has [Security Assertion Markup Language](https://en.wikipedia.org/wiki/Security_Assertion_Markup_Language) (SAML) enabled; otherwise, the return value is 'basic'.
* @param lookupData Data for scheme lookup. (optional)
* @return ApiResponse<ModelApiResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse tenantInfoWithHttpInfo(AuthSchemeLookupData lookupData) throws ApiException {
com.squareup.okhttp.Call call = tenantInfoValidateBeforeCall(lookupData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get authentication scheme. (asynchronously)
* Get the authentication scheme by user name or tenant name. The return value is 'saml' if the contact center has [Security Assertion Markup Language](https://en.wikipedia.org/wiki/Security_Assertion_Markup_Language) (SAML) enabled; otherwise, the return value is 'basic'.
* @param lookupData Data for scheme lookup. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call tenantInfoAsync(AuthSchemeLookupData lookupData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = tenantInfoValidateBeforeCall(lookupData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy