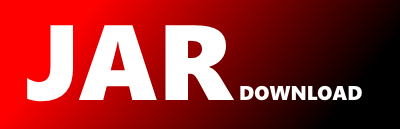
com.genesys.internal.workspace.model.VoicemakecallData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of workspace Show documentation
Show all versions of workspace Show documentation
A Java library to interface to Genesys Workspace public API
/*
* Workspace API
* Agent API
*
* OpenAPI spec version: 1.0.0
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.genesys.internal.workspace.model;
import java.util.Objects;
import com.genesys.internal.workspace.model.Kvpair;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* VoicemakecallData
*/
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2017-12-15T23:20:11.555Z")
public class VoicemakecallData {
@SerializedName("destination")
private String destination = null;
@SerializedName("location")
private String location = null;
@SerializedName("userData")
private List userData = null;
@SerializedName("reasons")
private List reasons = null;
@SerializedName("extensions")
private List extensions = null;
@SerializedName("outboundCallerId")
private String outboundCallerId = null;
public VoicemakecallData destination(String destination) {
this.destination = destination;
return this;
}
/**
* Directory number of the party the call will be transferred to.
* @return destination
**/
@ApiModelProperty(required = true, value = "Directory number of the party the call will be transferred to.")
public String getDestination() {
return destination;
}
public void setDestination(String destination) {
this.destination = destination;
}
public VoicemakecallData location(String location) {
this.location = location;
return this;
}
/**
* Name of the remote location in the form of <SwitchName> or <T-ServerApplicationName>@<SwitchName>. This value is used by Workspace to set the location attribute for the corresponding T-Server requests. When there is no need to specify a T-Server for location, this parameter must have the value NULL, not an empty string.
* @return location
**/
@ApiModelProperty(value = "Name of the remote location in the form of or @. This value is used by Workspace to set the location attribute for the corresponding T-Server requests. When there is no need to specify a T-Server for location, this parameter must have the value NULL, not an empty string.")
public String getLocation() {
return location;
}
public void setLocation(String location) {
this.location = location;
}
public VoicemakecallData userData(List userData) {
this.userData = userData;
return this;
}
public VoicemakecallData addUserDataItem(Kvpair userDataItem) {
if (this.userData == null) {
this.userData = new ArrayList();
}
this.userData.add(userDataItem);
return this;
}
/**
* A key/value pairs list of the user data that should be attached to the call.
* @return userData
**/
@ApiModelProperty(value = "A key/value pairs list of the user data that should be attached to the call.")
public List getUserData() {
return userData;
}
public void setUserData(List userData) {
this.userData = userData;
}
public VoicemakecallData reasons(List reasons) {
this.reasons = reasons;
return this;
}
public VoicemakecallData addReasonsItem(Kvpair reasonsItem) {
if (this.reasons == null) {
this.reasons = new ArrayList();
}
this.reasons.add(reasonsItem);
return this;
}
/**
* A collection of key/value pairs. For details about reasons, refer to the [Genesys Events and Models Reference Manual](https://docs.genesys.com/Documentation/System).
* @return reasons
**/
@ApiModelProperty(value = "A collection of key/value pairs. For details about reasons, refer to the [Genesys Events and Models Reference Manual](https://docs.genesys.com/Documentation/System).")
public List getReasons() {
return reasons;
}
public void setReasons(List reasons) {
this.reasons = reasons;
}
public VoicemakecallData extensions(List extensions) {
this.extensions = extensions;
return this;
}
public VoicemakecallData addExtensionsItem(Kvpair extensionsItem) {
if (this.extensions == null) {
this.extensions = new ArrayList();
}
this.extensions.add(extensionsItem);
return this;
}
/**
* A collection of key/value pairs. For details about extensions, refer to the [Genesys Events and Models Reference Manual](https://docs.genesys.com/Documentation/System).
* @return extensions
**/
@ApiModelProperty(value = "A collection of key/value pairs. For details about extensions, refer to the [Genesys Events and Models Reference Manual](https://docs.genesys.com/Documentation/System).")
public List getExtensions() {
return extensions;
}
public void setExtensions(List extensions) {
this.extensions = extensions;
}
public VoicemakecallData outboundCallerId(String outboundCallerId) {
this.outboundCallerId = outboundCallerId;
return this;
}
/**
* The caller ID information to display on the destination party's phone. The value should be set as CPNDigits. For more information about caller ID, see the [SIP Server Deployment Guide] (https://docs.genesys.com/Documentation/SIPS).
* @return outboundCallerId
**/
@ApiModelProperty(value = "The caller ID information to display on the destination party's phone. The value should be set as CPNDigits. For more information about caller ID, see the [SIP Server Deployment Guide] (https://docs.genesys.com/Documentation/SIPS).")
public String getOutboundCallerId() {
return outboundCallerId;
}
public void setOutboundCallerId(String outboundCallerId) {
this.outboundCallerId = outboundCallerId;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
VoicemakecallData voicemakecallData = (VoicemakecallData) o;
return Objects.equals(this.destination, voicemakecallData.destination) &&
Objects.equals(this.location, voicemakecallData.location) &&
Objects.equals(this.userData, voicemakecallData.userData) &&
Objects.equals(this.reasons, voicemakecallData.reasons) &&
Objects.equals(this.extensions, voicemakecallData.extensions) &&
Objects.equals(this.outboundCallerId, voicemakecallData.outboundCallerId);
}
@Override
public int hashCode() {
return Objects.hash(destination, location, userData, reasons, extensions, outboundCallerId);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class VoicemakecallData {\n");
sb.append(" destination: ").append(toIndentedString(destination)).append("\n");
sb.append(" location: ").append(toIndentedString(location)).append("\n");
sb.append(" userData: ").append(toIndentedString(userData)).append("\n");
sb.append(" reasons: ").append(toIndentedString(reasons)).append("\n");
sb.append(" extensions: ").append(toIndentedString(extensions)).append("\n");
sb.append(" outboundCallerId: ").append(toIndentedString(outboundCallerId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy