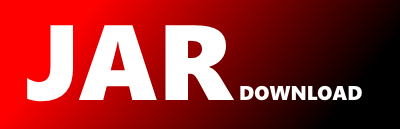
com.genesys.internal.workspace.api.TargetsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of workspace Show documentation
Show all versions of workspace Show documentation
A Java library to interface to Genesys Workspace public API
/*
* Workspace API
* Agent API
*
* OpenAPI spec version: 1.0.0
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.genesys.internal.workspace.api;
import com.genesys.internal.common.ApiCallback;
import com.genesys.internal.common.ApiClient;
import com.genesys.internal.common.ApiException;
import com.genesys.internal.common.ApiResponse;
import com.genesys.internal.common.Configuration;
import com.genesys.internal.common.Pair;
import com.genesys.internal.common.ProgressRequestBody;
import com.genesys.internal.common.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import com.genesys.internal.workspace.model.ApiErrorResponse;
import com.genesys.internal.workspace.model.ApiSuccessResponse;
import java.math.BigDecimal;
import com.genesys.internal.workspace.model.PersonalFavoriteData;
import com.genesys.internal.workspace.model.RecentTargetData;
import com.genesys.internal.workspace.model.TargetsResponse;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class TargetsApi {
private ApiClient apiClient;
public TargetsApi() {
this(Configuration.getDefaultApiClient());
}
public TargetsApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for ackRecentMissedCalls
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call ackRecentMissedCallsCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/targets/recents/ack-missed-calls";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call ackRecentMissedCallsValidateBeforeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = ackRecentMissedCallsCall(progressListener, progressRequestListener);
return call;
}
/**
* Acknowledge missed calls.
* Acknowledge missed calls in the list of recent targets.
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse ackRecentMissedCalls() throws ApiException {
ApiResponse resp = ackRecentMissedCallsWithHttpInfo();
return resp.getData();
}
/**
* Acknowledge missed calls.
* Acknowledge missed calls in the list of recent targets.
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse ackRecentMissedCallsWithHttpInfo() throws ApiException {
com.squareup.okhttp.Call call = ackRecentMissedCallsValidateBeforeCall(null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Acknowledge missed calls. (asynchronously)
* Acknowledge missed calls in the list of recent targets.
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call ackRecentMissedCallsAsync(final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = ackRecentMissedCallsValidateBeforeCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for addRecentTarget
* @param recentTargetData (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call addRecentTargetCall(RecentTargetData recentTargetData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = recentTargetData;
// create path and map variables
String localVarPath = "/targets/recents/add";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call addRecentTargetValidateBeforeCall(RecentTargetData recentTargetData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'recentTargetData' is set
if (recentTargetData == null) {
throw new ApiException("Missing the required parameter 'recentTargetData' when calling addRecentTarget(Async)");
}
com.squareup.okhttp.Call call = addRecentTargetCall(recentTargetData, progressListener, progressRequestListener);
return call;
}
/**
* Add a target.
* Add a target that the agent recently used.
* @param recentTargetData (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse addRecentTarget(RecentTargetData recentTargetData) throws ApiException {
ApiResponse resp = addRecentTargetWithHttpInfo(recentTargetData);
return resp.getData();
}
/**
* Add a target.
* Add a target that the agent recently used.
* @param recentTargetData (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse addRecentTargetWithHttpInfo(RecentTargetData recentTargetData) throws ApiException {
com.squareup.okhttp.Call call = addRecentTargetValidateBeforeCall(recentTargetData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Add a target. (asynchronously)
* Add a target that the agent recently used.
* @param recentTargetData (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call addRecentTargetAsync(RecentTargetData recentTargetData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = addRecentTargetValidateBeforeCall(recentTargetData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for deletePersonalFavorite
* @param id The ID of the target. (required)
* @param type The type of target. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call deletePersonalFavoriteCall(String id, String type, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/targets/personal-favorites/delete/{type}/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()))
.replaceAll("\\{" + "type" + "\\}", apiClient.escapeString(type.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call deletePersonalFavoriteValidateBeforeCall(String id, String type, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling deletePersonalFavorite(Async)");
}
// verify the required parameter 'type' is set
if (type == null) {
throw new ApiException("Missing the required parameter 'type' when calling deletePersonalFavorite(Async)");
}
com.squareup.okhttp.Call call = deletePersonalFavoriteCall(id, type, progressListener, progressRequestListener);
return call;
}
/**
* Delete a target.
* Delete the target from the agent's personal favorites
* @param id The ID of the target. (required)
* @param type The type of target. (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse deletePersonalFavorite(String id, String type) throws ApiException {
ApiResponse resp = deletePersonalFavoriteWithHttpInfo(id, type);
return resp.getData();
}
/**
* Delete a target.
* Delete the target from the agent's personal favorites
* @param id The ID of the target. (required)
* @param type The type of target. (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deletePersonalFavoriteWithHttpInfo(String id, String type) throws ApiException {
com.squareup.okhttp.Call call = deletePersonalFavoriteValidateBeforeCall(id, type, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Delete a target. (asynchronously)
* Delete the target from the agent's personal favorites
* @param id The ID of the target. (required)
* @param type The type of target. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call deletePersonalFavoriteAsync(String id, String type, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = deletePersonalFavoriteValidateBeforeCall(id, type, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for get
* @param searchTerm The text to search for in targets. (required)
* @param filterName Filter the search based on this field. (optional)
* @param types A comma-separated list of types to include in the search. Valid values are acd-queue, agent-group, agent, route-point, skill and custom-contact. (optional)
* @param excludeGroup A comma-separated list of agent group names. Workspace excludes those groups from the search. (optional)
* @param excludeFromGroup A comma-separated list of agent group names. Workspace excludes agents from these groups in the search. (optional)
* @param restrictToGroup A comma-separated list of agent group names. Workspace only searches for targets who belong to the groups in this list. (optional)
* @param sort The sort order, either `asc` (ascending) or `desc` (descending). The default is `asc`. (optional)
* @param limit The number of results to return. The default value is 50. (optional)
* @param matchType Specify whether the search should only return exact matches. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getCall(String searchTerm, String filterName, String types, String excludeGroup, String excludeFromGroup, String restrictToGroup, String sort, BigDecimal limit, String matchType, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/targets";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (searchTerm != null)
localVarQueryParams.addAll(apiClient.parameterToPair("searchTerm", searchTerm));
if (filterName != null)
localVarQueryParams.addAll(apiClient.parameterToPair("filterName", filterName));
if (types != null)
localVarQueryParams.addAll(apiClient.parameterToPair("types", types));
if (excludeGroup != null)
localVarQueryParams.addAll(apiClient.parameterToPair("excludeGroup", excludeGroup));
if (excludeFromGroup != null)
localVarQueryParams.addAll(apiClient.parameterToPair("excludeFromGroup", excludeFromGroup));
if (restrictToGroup != null)
localVarQueryParams.addAll(apiClient.parameterToPair("restrictToGroup", restrictToGroup));
if (sort != null)
localVarQueryParams.addAll(apiClient.parameterToPair("sort", sort));
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
if (matchType != null)
localVarQueryParams.addAll(apiClient.parameterToPair("matchType", matchType));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getValidateBeforeCall(String searchTerm, String filterName, String types, String excludeGroup, String excludeFromGroup, String restrictToGroup, String sort, BigDecimal limit, String matchType, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'searchTerm' is set
if (searchTerm == null) {
throw new ApiException("Missing the required parameter 'searchTerm' when calling get(Async)");
}
com.squareup.okhttp.Call call = getCall(searchTerm, filterName, types, excludeGroup, excludeFromGroup, restrictToGroup, sort, limit, matchType, progressListener, progressRequestListener);
return call;
}
/**
* Search for targets.
* Search for targets by the specified search term.
* @param searchTerm The text to search for in targets. (required)
* @param filterName Filter the search based on this field. (optional)
* @param types A comma-separated list of types to include in the search. Valid values are acd-queue, agent-group, agent, route-point, skill and custom-contact. (optional)
* @param excludeGroup A comma-separated list of agent group names. Workspace excludes those groups from the search. (optional)
* @param excludeFromGroup A comma-separated list of agent group names. Workspace excludes agents from these groups in the search. (optional)
* @param restrictToGroup A comma-separated list of agent group names. Workspace only searches for targets who belong to the groups in this list. (optional)
* @param sort The sort order, either `asc` (ascending) or `desc` (descending). The default is `asc`. (optional)
* @param limit The number of results to return. The default value is 50. (optional)
* @param matchType Specify whether the search should only return exact matches. (optional)
* @return TargetsResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TargetsResponse get(String searchTerm, String filterName, String types, String excludeGroup, String excludeFromGroup, String restrictToGroup, String sort, BigDecimal limit, String matchType) throws ApiException {
ApiResponse resp = getWithHttpInfo(searchTerm, filterName, types, excludeGroup, excludeFromGroup, restrictToGroup, sort, limit, matchType);
return resp.getData();
}
/**
* Search for targets.
* Search for targets by the specified search term.
* @param searchTerm The text to search for in targets. (required)
* @param filterName Filter the search based on this field. (optional)
* @param types A comma-separated list of types to include in the search. Valid values are acd-queue, agent-group, agent, route-point, skill and custom-contact. (optional)
* @param excludeGroup A comma-separated list of agent group names. Workspace excludes those groups from the search. (optional)
* @param excludeFromGroup A comma-separated list of agent group names. Workspace excludes agents from these groups in the search. (optional)
* @param restrictToGroup A comma-separated list of agent group names. Workspace only searches for targets who belong to the groups in this list. (optional)
* @param sort The sort order, either `asc` (ascending) or `desc` (descending). The default is `asc`. (optional)
* @param limit The number of results to return. The default value is 50. (optional)
* @param matchType Specify whether the search should only return exact matches. (optional)
* @return ApiResponse<TargetsResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getWithHttpInfo(String searchTerm, String filterName, String types, String excludeGroup, String excludeFromGroup, String restrictToGroup, String sort, BigDecimal limit, String matchType) throws ApiException {
com.squareup.okhttp.Call call = getValidateBeforeCall(searchTerm, filterName, types, excludeGroup, excludeFromGroup, restrictToGroup, sort, limit, matchType, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Search for targets. (asynchronously)
* Search for targets by the specified search term.
* @param searchTerm The text to search for in targets. (required)
* @param filterName Filter the search based on this field. (optional)
* @param types A comma-separated list of types to include in the search. Valid values are acd-queue, agent-group, agent, route-point, skill and custom-contact. (optional)
* @param excludeGroup A comma-separated list of agent group names. Workspace excludes those groups from the search. (optional)
* @param excludeFromGroup A comma-separated list of agent group names. Workspace excludes agents from these groups in the search. (optional)
* @param restrictToGroup A comma-separated list of agent group names. Workspace only searches for targets who belong to the groups in this list. (optional)
* @param sort The sort order, either `asc` (ascending) or `desc` (descending). The default is `asc`. (optional)
* @param limit The number of results to return. The default value is 50. (optional)
* @param matchType Specify whether the search should only return exact matches. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getAsync(String searchTerm, String filterName, String types, String excludeGroup, String excludeFromGroup, String restrictToGroup, String sort, BigDecimal limit, String matchType, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getValidateBeforeCall(searchTerm, filterName, types, excludeGroup, excludeFromGroup, restrictToGroup, sort, limit, matchType, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getPersonalFavorites
* @param limit Number of results to return. The default value is 50. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getPersonalFavoritesCall(BigDecimal limit, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/targets/personal-favorites";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getPersonalFavoritesValidateBeforeCall(BigDecimal limit, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = getPersonalFavoritesCall(limit, progressListener, progressRequestListener);
return call;
}
/**
* Return personal favorites.
* Return the agent's personal favorites.
* @param limit Number of results to return. The default value is 50. (optional)
* @return TargetsResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TargetsResponse getPersonalFavorites(BigDecimal limit) throws ApiException {
ApiResponse resp = getPersonalFavoritesWithHttpInfo(limit);
return resp.getData();
}
/**
* Return personal favorites.
* Return the agent's personal favorites.
* @param limit Number of results to return. The default value is 50. (optional)
* @return ApiResponse<TargetsResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getPersonalFavoritesWithHttpInfo(BigDecimal limit) throws ApiException {
com.squareup.okhttp.Call call = getPersonalFavoritesValidateBeforeCall(limit, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Return personal favorites. (asynchronously)
* Return the agent's personal favorites.
* @param limit Number of results to return. The default value is 50. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getPersonalFavoritesAsync(BigDecimal limit, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getPersonalFavoritesValidateBeforeCall(limit, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getRecentTargets
* @param limit The number of results to return. The default value is 50. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getRecentTargetsCall(BigDecimal limit, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/targets/recents";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("limit", limit));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getRecentTargetsValidateBeforeCall(BigDecimal limit, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = getRecentTargetsCall(limit, progressListener, progressRequestListener);
return call;
}
/**
* Get recently used targets.
* Get recently used targets for the current agent.
* @param limit The number of results to return. The default value is 50. (optional)
* @return TargetsResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TargetsResponse getRecentTargets(BigDecimal limit) throws ApiException {
ApiResponse resp = getRecentTargetsWithHttpInfo(limit);
return resp.getData();
}
/**
* Get recently used targets.
* Get recently used targets for the current agent.
* @param limit The number of results to return. The default value is 50. (optional)
* @return ApiResponse<TargetsResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getRecentTargetsWithHttpInfo(BigDecimal limit) throws ApiException {
com.squareup.okhttp.Call call = getRecentTargetsValidateBeforeCall(limit, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get recently used targets. (asynchronously)
* Get recently used targets for the current agent.
* @param limit The number of results to return. The default value is 50. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getRecentTargetsAsync(BigDecimal limit, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getRecentTargetsValidateBeforeCall(limit, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getTarget
* @param id The ID of the target. (required)
* @param type The type of target to retrieve. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getTargetCall(BigDecimal id, String type, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/targets/{type}/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()))
.replaceAll("\\{" + "type" + "\\}", apiClient.escapeString(type.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getTargetValidateBeforeCall(BigDecimal id, String type, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling getTarget(Async)");
}
// verify the required parameter 'type' is set
if (type == null) {
throw new ApiException("Missing the required parameter 'type' when calling getTarget(Async)");
}
com.squareup.okhttp.Call call = getTargetCall(id, type, progressListener, progressRequestListener);
return call;
}
/**
* Get a target.
* Get a specific target by type and ID. Targets can be agents, agent groups, queues, route points, skills, and custom contacts.
* @param id The ID of the target. (required)
* @param type The type of target to retrieve. (required)
* @return TargetsResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TargetsResponse getTarget(BigDecimal id, String type) throws ApiException {
ApiResponse resp = getTargetWithHttpInfo(id, type);
return resp.getData();
}
/**
* Get a target.
* Get a specific target by type and ID. Targets can be agents, agent groups, queues, route points, skills, and custom contacts.
* @param id The ID of the target. (required)
* @param type The type of target to retrieve. (required)
* @return ApiResponse<TargetsResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getTargetWithHttpInfo(BigDecimal id, String type) throws ApiException {
com.squareup.okhttp.Call call = getTargetValidateBeforeCall(id, type, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a target. (asynchronously)
* Get a specific target by type and ID. Targets can be agents, agent groups, queues, route points, skills, and custom contacts.
* @param id The ID of the target. (required)
* @param type The type of target to retrieve. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getTargetAsync(BigDecimal id, String type, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getTargetValidateBeforeCall(id, type, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for savePersonalFavorite
* @param personalFavoriteData (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call savePersonalFavoriteCall(PersonalFavoriteData personalFavoriteData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = personalFavoriteData;
// create path and map variables
String localVarPath = "/targets/personal-favorites/save";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call savePersonalFavoriteValidateBeforeCall(PersonalFavoriteData personalFavoriteData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'personalFavoriteData' is set
if (personalFavoriteData == null) {
throw new ApiException("Missing the required parameter 'personalFavoriteData' when calling savePersonalFavorite(Async)");
}
com.squareup.okhttp.Call call = savePersonalFavoriteCall(personalFavoriteData, progressListener, progressRequestListener);
return call;
}
/**
* Save a personal favorite.
* Save a target to the agent's personal favorites in the specified category.
* @param personalFavoriteData (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse savePersonalFavorite(PersonalFavoriteData personalFavoriteData) throws ApiException {
ApiResponse resp = savePersonalFavoriteWithHttpInfo(personalFavoriteData);
return resp.getData();
}
/**
* Save a personal favorite.
* Save a target to the agent's personal favorites in the specified category.
* @param personalFavoriteData (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse savePersonalFavoriteWithHttpInfo(PersonalFavoriteData personalFavoriteData) throws ApiException {
com.squareup.okhttp.Call call = savePersonalFavoriteValidateBeforeCall(personalFavoriteData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Save a personal favorite. (asynchronously)
* Save a target to the agent's personal favorites in the specified category.
* @param personalFavoriteData (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call savePersonalFavoriteAsync(PersonalFavoriteData personalFavoriteData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = savePersonalFavoriteValidateBeforeCall(personalFavoriteData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy