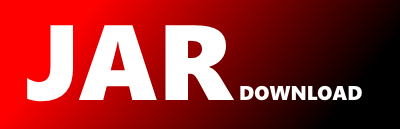
com.genesys.workspace.models.KeyValueCollection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of workspace Show documentation
Show all versions of workspace Show documentation
A Java library to interface to Genesys Workspace public API
package com.genesys.workspace.models;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
public class KeyValueCollection implements Iterable {
private Map data;
public KeyValueCollection() {
this.data = new HashMap<>();
}
public void addString(String key, String value) {
this.data.put(key, new KeyValuePair(key, value));
}
public void addInt(String key, int value) {
this.data.put(key, new KeyValuePair(key, value));
}
public void addList(String key, KeyValueCollection value) {
this.data.put(key, new KeyValuePair(key, value));
}
public String getString(String key) {
KeyValuePair pair = data.get(key);
return pair != null? pair.getStringValue(): null;
}
@Override
public Iterator iterator() {
return this.data.values().iterator();
}
public Integer getInt(String key) {
KeyValuePair pair = data.get(key);
return pair != null? pair.getIntValue(): null;
}
public KeyValueCollection getList(String key) {
KeyValuePair pair = data.get(key);
return pair != null? pair.getListValue(): null;
}
@Override
public String toString() {
String str = "[";
for(KeyValuePair pair : this.data.values()) {
switch (pair.getValueType()) {
case STRING:
str += " STRING: " + pair.getKey() + "=" + pair.getStringValue();
break;
case INT:
str += " INT: " + pair.getKey() + "=" + pair.getIntValue();
break;
case LIST:
str += " LIST: " + pair.getKey() + "=" + pair.getListValue();
break;
}
}
str += "]";
return str;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy