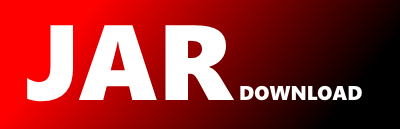
com.genesys.internal.workspace.api.UcsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of workspace Show documentation
Show all versions of workspace Show documentation
A Java library to interface to Genesys Workspace public API
/*
* Workspace API
* Agent API
*
* OpenAPI spec version: 1.0.0
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.genesys.internal.workspace.api;
import com.genesys.internal.common.ApiCallback;
import com.genesys.internal.common.ApiClient;
import com.genesys.internal.common.ApiException;
import com.genesys.internal.common.ApiResponse;
import com.genesys.internal.common.Configuration;
import com.genesys.internal.common.Pair;
import com.genesys.internal.common.ProgressRequestBody;
import com.genesys.internal.common.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import com.genesys.internal.workspace.model.AgentHistoryData;
import com.genesys.internal.workspace.model.ApiErrorResponse;
import com.genesys.internal.workspace.model.ApiSuccessResponse;
import com.genesys.internal.workspace.model.AssignInteractionToContactData;
import com.genesys.internal.workspace.model.CallCompletedData;
import com.genesys.internal.workspace.model.CallNoteData;
import com.genesys.internal.workspace.model.ConfigResponse;
import com.genesys.internal.workspace.model.ContactDetailsData;
import com.genesys.internal.workspace.model.ContactHistoryData;
import com.genesys.internal.workspace.model.CreateContactData;
import com.genesys.internal.workspace.model.IdentifyContactData;
import com.genesys.internal.workspace.model.InteractionDetailsData;
import com.genesys.internal.workspace.model.LuceneSearchData;
import com.genesys.internal.workspace.model.LuceneSearchInteractionData;
import com.genesys.internal.workspace.model.UpdateContactData;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class UcsApi {
private ApiClient apiClient;
public UcsApi() {
this(Configuration.getDefaultApiClient());
}
public UcsApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for assignInteractionToContact
* @param id id of the Interaction (required)
* @param assignInteractionToContactData (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call assignInteractionToContactCall(String id, AssignInteractionToContactData assignInteractionToContactData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = assignInteractionToContactData;
// create path and map variables
String localVarPath = "/ucs/interactions/{id}/assign-contact"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call assignInteractionToContactValidateBeforeCall(String id, AssignInteractionToContactData assignInteractionToContactData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling assignInteractionToContact(Async)");
}
// verify the required parameter 'assignInteractionToContactData' is set
if (assignInteractionToContactData == null) {
throw new ApiException("Missing the required parameter 'assignInteractionToContactData' when calling assignInteractionToContact(Async)");
}
com.squareup.okhttp.Call call = assignInteractionToContactCall(id, assignInteractionToContactData, progressListener, progressRequestListener);
return call;
}
/**
* Assign the interaction to a contact
*
* @param id id of the Interaction (required)
* @param assignInteractionToContactData (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse assignInteractionToContact(String id, AssignInteractionToContactData assignInteractionToContactData) throws ApiException {
ApiResponse resp = assignInteractionToContactWithHttpInfo(id, assignInteractionToContactData);
return resp.getData();
}
/**
* Assign the interaction to a contact
*
* @param id id of the Interaction (required)
* @param assignInteractionToContactData (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse assignInteractionToContactWithHttpInfo(String id, AssignInteractionToContactData assignInteractionToContactData) throws ApiException {
com.squareup.okhttp.Call call = assignInteractionToContactValidateBeforeCall(id, assignInteractionToContactData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Assign the interaction to a contact (asynchronously)
*
* @param id id of the Interaction (required)
* @param assignInteractionToContactData (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call assignInteractionToContactAsync(String id, AssignInteractionToContactData assignInteractionToContactData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = assignInteractionToContactValidateBeforeCall(id, assignInteractionToContactData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for createContact
* @param createContactData (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call createContactCall(CreateContactData createContactData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = createContactData;
// create path and map variables
String localVarPath = "/ucs/contacts/create";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call createContactValidateBeforeCall(CreateContactData createContactData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'createContactData' is set
if (createContactData == null) {
throw new ApiException("Missing the required parameter 'createContactData' when calling createContact(Async)");
}
com.squareup.okhttp.Call call = createContactCall(createContactData, progressListener, progressRequestListener);
return call;
}
/**
* Create a new contact
*
* @param createContactData (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse createContact(CreateContactData createContactData) throws ApiException {
ApiResponse resp = createContactWithHttpInfo(createContactData);
return resp.getData();
}
/**
* Create a new contact
*
* @param createContactData (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse createContactWithHttpInfo(CreateContactData createContactData) throws ApiException {
com.squareup.okhttp.Call call = createContactValidateBeforeCall(createContactData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Create a new contact (asynchronously)
*
* @param createContactData (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call createContactAsync(CreateContactData createContactData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = createContactValidateBeforeCall(createContactData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for deleteContact
* @param id id of the Contact (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call deleteContactCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/ucs/contacts/{id}/delete"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call deleteContactValidateBeforeCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling deleteContact(Async)");
}
com.squareup.okhttp.Call call = deleteContactCall(id, progressListener, progressRequestListener);
return call;
}
/**
* Delete an existing contact
*
* @param id id of the Contact (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse deleteContact(String id) throws ApiException {
ApiResponse resp = deleteContactWithHttpInfo(id);
return resp.getData();
}
/**
* Delete an existing contact
*
* @param id id of the Contact (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteContactWithHttpInfo(String id) throws ApiException {
com.squareup.okhttp.Call call = deleteContactValidateBeforeCall(id, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Delete an existing contact (asynchronously)
*
* @param id id of the Contact (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call deleteContactAsync(String id, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = deleteContactValidateBeforeCall(id, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for findOrCreatePhoneCall
* @param id id of the Voice Interaction (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call findOrCreatePhoneCallCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/ucs/voice/{id}/find-or-create"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call findOrCreatePhoneCallValidateBeforeCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling findOrCreatePhoneCall(Async)");
}
com.squareup.okhttp.Call call = findOrCreatePhoneCallCall(id, progressListener, progressRequestListener);
return call;
}
/**
* Find or create phone call in UCS
*
* @param id id of the Voice Interaction (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse findOrCreatePhoneCall(String id) throws ApiException {
ApiResponse resp = findOrCreatePhoneCallWithHttpInfo(id);
return resp.getData();
}
/**
* Find or create phone call in UCS
*
* @param id id of the Voice Interaction (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse findOrCreatePhoneCallWithHttpInfo(String id) throws ApiException {
com.squareup.okhttp.Call call = findOrCreatePhoneCallValidateBeforeCall(id, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Find or create phone call in UCS (asynchronously)
*
* @param id id of the Voice Interaction (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call findOrCreatePhoneCallAsync(String id, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = findOrCreatePhoneCallValidateBeforeCall(id, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getAgentHistory
* @param agentHistoryData (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getAgentHistoryCall(AgentHistoryData agentHistoryData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = agentHistoryData;
// create path and map variables
String localVarPath = "/ucs/get-agent-history";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getAgentHistoryValidateBeforeCall(AgentHistoryData agentHistoryData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = getAgentHistoryCall(agentHistoryData, progressListener, progressRequestListener);
return call;
}
/**
* Get the history of interactions for the agent
*
* @param agentHistoryData (optional)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse getAgentHistory(AgentHistoryData agentHistoryData) throws ApiException {
ApiResponse resp = getAgentHistoryWithHttpInfo(agentHistoryData);
return resp.getData();
}
/**
* Get the history of interactions for the agent
*
* @param agentHistoryData (optional)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getAgentHistoryWithHttpInfo(AgentHistoryData agentHistoryData) throws ApiException {
com.squareup.okhttp.Call call = getAgentHistoryValidateBeforeCall(agentHistoryData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get the history of interactions for the agent (asynchronously)
*
* @param agentHistoryData (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getAgentHistoryAsync(AgentHistoryData agentHistoryData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getAgentHistoryValidateBeforeCall(agentHistoryData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getContactDetails
* @param id id of the Contact (required)
* @param contactDetailsData (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getContactDetailsCall(String id, ContactDetailsData contactDetailsData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = contactDetailsData;
// create path and map variables
String localVarPath = "/ucs/contacts/{id}/get-details"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getContactDetailsValidateBeforeCall(String id, ContactDetailsData contactDetailsData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling getContactDetails(Async)");
}
// verify the required parameter 'contactDetailsData' is set
if (contactDetailsData == null) {
throw new ApiException("Missing the required parameter 'contactDetailsData' when calling getContactDetails(Async)");
}
com.squareup.okhttp.Call call = getContactDetailsCall(id, contactDetailsData, progressListener, progressRequestListener);
return call;
}
/**
* Get the details of a contact
*
* @param id id of the Contact (required)
* @param contactDetailsData (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse getContactDetails(String id, ContactDetailsData contactDetailsData) throws ApiException {
ApiResponse resp = getContactDetailsWithHttpInfo(id, contactDetailsData);
return resp.getData();
}
/**
* Get the details of a contact
*
* @param id id of the Contact (required)
* @param contactDetailsData (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getContactDetailsWithHttpInfo(String id, ContactDetailsData contactDetailsData) throws ApiException {
com.squareup.okhttp.Call call = getContactDetailsValidateBeforeCall(id, contactDetailsData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get the details of a contact (asynchronously)
*
* @param id id of the Contact (required)
* @param contactDetailsData (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getContactDetailsAsync(String id, ContactDetailsData contactDetailsData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getContactDetailsValidateBeforeCall(id, contactDetailsData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getContactHistory
* @param id id of the Contact (required)
* @param contactHistoryData (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getContactHistoryCall(String id, ContactHistoryData contactHistoryData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = contactHistoryData;
// create path and map variables
String localVarPath = "/ucs/contacts/{id}/get-history"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getContactHistoryValidateBeforeCall(String id, ContactHistoryData contactHistoryData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling getContactHistory(Async)");
}
// verify the required parameter 'contactHistoryData' is set
if (contactHistoryData == null) {
throw new ApiException("Missing the required parameter 'contactHistoryData' when calling getContactHistory(Async)");
}
com.squareup.okhttp.Call call = getContactHistoryCall(id, contactHistoryData, progressListener, progressRequestListener);
return call;
}
/**
* Get the history of interactions for a contact
*
* @param id id of the Contact (required)
* @param contactHistoryData (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse getContactHistory(String id, ContactHistoryData contactHistoryData) throws ApiException {
ApiResponse resp = getContactHistoryWithHttpInfo(id, contactHistoryData);
return resp.getData();
}
/**
* Get the history of interactions for a contact
*
* @param id id of the Contact (required)
* @param contactHistoryData (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getContactHistoryWithHttpInfo(String id, ContactHistoryData contactHistoryData) throws ApiException {
com.squareup.okhttp.Call call = getContactHistoryValidateBeforeCall(id, contactHistoryData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get the history of interactions for a contact (asynchronously)
*
* @param id id of the Contact (required)
* @param contactHistoryData (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getContactHistoryAsync(String id, ContactHistoryData contactHistoryData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getContactHistoryValidateBeforeCall(id, contactHistoryData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getInteractionDetails
* @param id id of the Interaction (required)
* @param interactionDetailsData (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getInteractionDetailsCall(String id, InteractionDetailsData interactionDetailsData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = interactionDetailsData;
// create path and map variables
String localVarPath = "/ucs/interactions/{id}/get-details"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getInteractionDetailsValidateBeforeCall(String id, InteractionDetailsData interactionDetailsData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling getInteractionDetails(Async)");
}
com.squareup.okhttp.Call call = getInteractionDetailsCall(id, interactionDetailsData, progressListener, progressRequestListener);
return call;
}
/**
* Get the content of the interaction
*
* @param id id of the Interaction (required)
* @param interactionDetailsData (optional)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse getInteractionDetails(String id, InteractionDetailsData interactionDetailsData) throws ApiException {
ApiResponse resp = getInteractionDetailsWithHttpInfo(id, interactionDetailsData);
return resp.getData();
}
/**
* Get the content of the interaction
*
* @param id id of the Interaction (required)
* @param interactionDetailsData (optional)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getInteractionDetailsWithHttpInfo(String id, InteractionDetailsData interactionDetailsData) throws ApiException {
com.squareup.okhttp.Call call = getInteractionDetailsValidateBeforeCall(id, interactionDetailsData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get the content of the interaction (asynchronously)
*
* @param id id of the Interaction (required)
* @param interactionDetailsData (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getInteractionDetailsAsync(String id, InteractionDetailsData interactionDetailsData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getInteractionDetailsValidateBeforeCall(id, interactionDetailsData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getLuceneIndexes
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getLuceneIndexesCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/ucs/get-lucene-indexes";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getLuceneIndexesValidateBeforeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = getLuceneIndexesCall(progressListener, progressRequestListener);
return call;
}
/**
* Get the lucene indexes for ucs
* This request returns all the lucene indexes for contact.
* @return ConfigResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ConfigResponse getLuceneIndexes() throws ApiException {
ApiResponse resp = getLuceneIndexesWithHttpInfo();
return resp.getData();
}
/**
* Get the lucene indexes for ucs
* This request returns all the lucene indexes for contact.
* @return ApiResponse<ConfigResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getLuceneIndexesWithHttpInfo() throws ApiException {
com.squareup.okhttp.Call call = getLuceneIndexesValidateBeforeCall(null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get the lucene indexes for ucs (asynchronously)
* This request returns all the lucene indexes for contact.
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getLuceneIndexesAsync(final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getLuceneIndexesValidateBeforeCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for identifyContact
* @param id id of the Interaction (required)
* @param identifyContactData (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call identifyContactCall(String id, IdentifyContactData identifyContactData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = identifyContactData;
// create path and map variables
String localVarPath = "/ucs/interactions/{id}/identify-contact"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call identifyContactValidateBeforeCall(String id, IdentifyContactData identifyContactData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling identifyContact(Async)");
}
// verify the required parameter 'identifyContactData' is set
if (identifyContactData == null) {
throw new ApiException("Missing the required parameter 'identifyContactData' when calling identifyContact(Async)");
}
com.squareup.okhttp.Call call = identifyContactCall(id, identifyContactData, progressListener, progressRequestListener);
return call;
}
/**
* Identify the contact for the interaction
*
* @param id id of the Interaction (required)
* @param identifyContactData (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse identifyContact(String id, IdentifyContactData identifyContactData) throws ApiException {
ApiResponse resp = identifyContactWithHttpInfo(id, identifyContactData);
return resp.getData();
}
/**
* Identify the contact for the interaction
*
* @param id id of the Interaction (required)
* @param identifyContactData (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse identifyContactWithHttpInfo(String id, IdentifyContactData identifyContactData) throws ApiException {
com.squareup.okhttp.Call call = identifyContactValidateBeforeCall(id, identifyContactData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Identify the contact for the interaction (asynchronously)
*
* @param id id of the Interaction (required)
* @param identifyContactData (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call identifyContactAsync(String id, IdentifyContactData identifyContactData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = identifyContactValidateBeforeCall(id, identifyContactData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for searchContacts
* @param luceneSearchData (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call searchContactsCall(LuceneSearchData luceneSearchData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = luceneSearchData;
// create path and map variables
String localVarPath = "/ucs/contacts/search";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call searchContactsValidateBeforeCall(LuceneSearchData luceneSearchData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'luceneSearchData' is set
if (luceneSearchData == null) {
throw new ApiException("Missing the required parameter 'luceneSearchData' when calling searchContacts(Async)");
}
com.squareup.okhttp.Call call = searchContactsCall(luceneSearchData, progressListener, progressRequestListener);
return call;
}
/**
* Search for contacts. If 'sortCriteria' or 'startIndex' is specified, the query is based on SQL, otherwise on Lucene
*
* @param luceneSearchData (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse searchContacts(LuceneSearchData luceneSearchData) throws ApiException {
ApiResponse resp = searchContactsWithHttpInfo(luceneSearchData);
return resp.getData();
}
/**
* Search for contacts. If 'sortCriteria' or 'startIndex' is specified, the query is based on SQL, otherwise on Lucene
*
* @param luceneSearchData (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse searchContactsWithHttpInfo(LuceneSearchData luceneSearchData) throws ApiException {
com.squareup.okhttp.Call call = searchContactsValidateBeforeCall(luceneSearchData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Search for contacts. If 'sortCriteria' or 'startIndex' is specified, the query is based on SQL, otherwise on Lucene (asynchronously)
*
* @param luceneSearchData (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call searchContactsAsync(LuceneSearchData luceneSearchData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = searchContactsValidateBeforeCall(luceneSearchData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for searchInteractions
* @param luceneSearchInteractionData (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call searchInteractionsCall(LuceneSearchInteractionData luceneSearchInteractionData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = luceneSearchInteractionData;
// create path and map variables
String localVarPath = "/ucs/ixn/search";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call searchInteractionsValidateBeforeCall(LuceneSearchInteractionData luceneSearchInteractionData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'luceneSearchInteractionData' is set
if (luceneSearchInteractionData == null) {
throw new ApiException("Missing the required parameter 'luceneSearchInteractionData' when calling searchInteractions(Async)");
}
com.squareup.okhttp.Call call = searchInteractionsCall(luceneSearchInteractionData, progressListener, progressRequestListener);
return call;
}
/**
* Search for interactions based on search query, using lucene search
*
* @param luceneSearchInteractionData (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse searchInteractions(LuceneSearchInteractionData luceneSearchInteractionData) throws ApiException {
ApiResponse resp = searchInteractionsWithHttpInfo(luceneSearchInteractionData);
return resp.getData();
}
/**
* Search for interactions based on search query, using lucene search
*
* @param luceneSearchInteractionData (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse searchInteractionsWithHttpInfo(LuceneSearchInteractionData luceneSearchInteractionData) throws ApiException {
com.squareup.okhttp.Call call = searchInteractionsValidateBeforeCall(luceneSearchInteractionData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Search for interactions based on search query, using lucene search (asynchronously)
*
* @param luceneSearchInteractionData (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call searchInteractionsAsync(LuceneSearchInteractionData luceneSearchInteractionData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = searchInteractionsValidateBeforeCall(luceneSearchInteractionData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for setCallCompleted
* @param id id of the Interaction (required)
* @param callCompletedData (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call setCallCompletedCall(String id, CallCompletedData callCompletedData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = callCompletedData;
// create path and map variables
String localVarPath = "/ucs/interactions/{id}/set-completed"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call setCallCompletedValidateBeforeCall(String id, CallCompletedData callCompletedData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling setCallCompleted(Async)");
}
// verify the required parameter 'callCompletedData' is set
if (callCompletedData == null) {
throw new ApiException("Missing the required parameter 'callCompletedData' when calling setCallCompleted(Async)");
}
com.squareup.okhttp.Call call = setCallCompletedCall(id, callCompletedData, progressListener, progressRequestListener);
return call;
}
/**
* Set the call as being completed
*
* @param id id of the Interaction (required)
* @param callCompletedData (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse setCallCompleted(String id, CallCompletedData callCompletedData) throws ApiException {
ApiResponse resp = setCallCompletedWithHttpInfo(id, callCompletedData);
return resp.getData();
}
/**
* Set the call as being completed
*
* @param id id of the Interaction (required)
* @param callCompletedData (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse setCallCompletedWithHttpInfo(String id, CallCompletedData callCompletedData) throws ApiException {
com.squareup.okhttp.Call call = setCallCompletedValidateBeforeCall(id, callCompletedData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Set the call as being completed (asynchronously)
*
* @param id id of the Interaction (required)
* @param callCompletedData (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call setCallCompletedAsync(String id, CallCompletedData callCompletedData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = setCallCompletedValidateBeforeCall(id, callCompletedData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for setCallNote
* @param id id of the Interaction (required)
* @param callNoteData (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call setCallNoteCall(String id, CallNoteData callNoteData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = callNoteData;
// create path and map variables
String localVarPath = "/ucs/interactions/{id}/set-note"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call setCallNoteValidateBeforeCall(String id, CallNoteData callNoteData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling setCallNote(Async)");
}
// verify the required parameter 'callNoteData' is set
if (callNoteData == null) {
throw new ApiException("Missing the required parameter 'callNoteData' when calling setCallNote(Async)");
}
com.squareup.okhttp.Call call = setCallNoteCall(id, callNoteData, progressListener, progressRequestListener);
return call;
}
/**
* Set the note for the call
*
* @param id id of the Interaction (required)
* @param callNoteData (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse setCallNote(String id, CallNoteData callNoteData) throws ApiException {
ApiResponse resp = setCallNoteWithHttpInfo(id, callNoteData);
return resp.getData();
}
/**
* Set the note for the call
*
* @param id id of the Interaction (required)
* @param callNoteData (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse setCallNoteWithHttpInfo(String id, CallNoteData callNoteData) throws ApiException {
com.squareup.okhttp.Call call = setCallNoteValidateBeforeCall(id, callNoteData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Set the note for the call (asynchronously)
*
* @param id id of the Interaction (required)
* @param callNoteData (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call setCallNoteAsync(String id, CallNoteData callNoteData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = setCallNoteValidateBeforeCall(id, callNoteData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for updateContact
* @param id id of the Contact (required)
* @param updateContactData (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call updateContactCall(String id, UpdateContactData updateContactData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = updateContactData;
// create path and map variables
String localVarPath = "/ucs/contacts/{id}/update"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call updateContactValidateBeforeCall(String id, UpdateContactData updateContactData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling updateContact(Async)");
}
// verify the required parameter 'updateContactData' is set
if (updateContactData == null) {
throw new ApiException("Missing the required parameter 'updateContactData' when calling updateContact(Async)");
}
com.squareup.okhttp.Call call = updateContactCall(id, updateContactData, progressListener, progressRequestListener);
return call;
}
/**
* Update attributes of an existing contact
*
* @param id id of the Contact (required)
* @param updateContactData (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse updateContact(String id, UpdateContactData updateContactData) throws ApiException {
ApiResponse resp = updateContactWithHttpInfo(id, updateContactData);
return resp.getData();
}
/**
* Update attributes of an existing contact
*
* @param id id of the Contact (required)
* @param updateContactData (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse updateContactWithHttpInfo(String id, UpdateContactData updateContactData) throws ApiException {
com.squareup.okhttp.Call call = updateContactValidateBeforeCall(id, updateContactData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Update attributes of an existing contact (asynchronously)
*
* @param id id of the Contact (required)
* @param updateContactData (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call updateContactAsync(String id, UpdateContactData updateContactData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = updateContactValidateBeforeCall(id, updateContactData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy