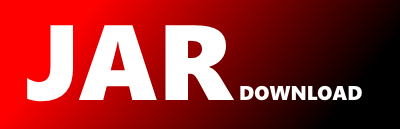
com.genesys.internal.workspace.api.MediaApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of workspace Show documentation
Show all versions of workspace Show documentation
A Java library to interface to Genesys Workspace public API
/*
* Workspace API
* Agent API
*
* OpenAPI spec version: v9.0.000.20.2204
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.genesys.internal.workspace.api;
import com.genesys.internal.common.ApiCallback;
import com.genesys.internal.common.ApiClient;
import com.genesys.internal.common.ApiException;
import com.genesys.internal.common.ApiResponse;
import com.genesys.internal.common.Configuration;
import com.genesys.internal.common.Pair;
import com.genesys.internal.common.ProgressRequestBody;
import com.genesys.internal.common.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import com.genesys.internal.workspace.model.AcceptData6;
import com.genesys.internal.workspace.model.AddCommentData;
import com.genesys.internal.workspace.model.AddContentData;
import com.genesys.internal.workspace.model.ApiErrorResponse;
import com.genesys.internal.workspace.model.ApiSuccessResponse;
import java.io.File;
import com.genesys.internal.workspace.model.FormData;
import com.genesys.internal.workspace.model.LogoutMediaData;
import com.genesys.internal.workspace.model.MediaStartMonitoringData;
import com.genesys.internal.workspace.model.MediaStopMonitoringData;
import com.genesys.internal.workspace.model.NotReadyForAgentData;
import com.genesys.internal.workspace.model.NotReadyForMediaData;
import com.genesys.internal.workspace.model.PlaceInQueueData;
import com.genesys.internal.workspace.model.PutOnHoldData;
import com.genesys.internal.workspace.model.ReadyForMediaData;
import com.genesys.internal.workspace.model.RejectData;
import com.genesys.internal.workspace.model.TransferData;
import com.genesys.internal.workspace.model.UserData;
import com.genesys.internal.workspace.model.UserData2;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class MediaApi {
private ApiClient apiClient;
public MediaApi() {
this(Configuration.getDefaultApiClient());
}
public MediaApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for accept
* @param mediatype The media channel. (required)
* @param id The ID of the interaction to accept. (required)
* @param acceptData Request parameters. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call acceptCall(String mediatype, String id, AcceptData6 acceptData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = acceptData;
// create path and map variables
String localVarPath = "/media/{mediatype}/interactions/{id}/accept"
.replaceAll("\\{" + "mediatype" + "\\}", apiClient.escapeString(mediatype.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call acceptValidateBeforeCall(String mediatype, String id, AcceptData6 acceptData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'mediatype' is set
if (mediatype == null) {
throw new ApiException("Missing the required parameter 'mediatype' when calling accept(Async)");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling accept(Async)");
}
com.squareup.okhttp.Call call = acceptCall(mediatype, id, acceptData, progressListener, progressRequestListener);
return call;
}
/**
* Accept an incoming interaction.
* Accept the specified interaction.
* @param mediatype The media channel. (required)
* @param id The ID of the interaction to accept. (required)
* @param acceptData Request parameters. (optional)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse accept(String mediatype, String id, AcceptData6 acceptData) throws ApiException {
ApiResponse resp = acceptWithHttpInfo(mediatype, id, acceptData);
return resp.getData();
}
/**
* Accept an incoming interaction.
* Accept the specified interaction.
* @param mediatype The media channel. (required)
* @param id The ID of the interaction to accept. (required)
* @param acceptData Request parameters. (optional)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse acceptWithHttpInfo(String mediatype, String id, AcceptData6 acceptData) throws ApiException {
com.squareup.okhttp.Call call = acceptValidateBeforeCall(mediatype, id, acceptData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Accept an incoming interaction. (asynchronously)
* Accept the specified interaction.
* @param mediatype The media channel. (required)
* @param id The ID of the interaction to accept. (required)
* @param acceptData Request parameters. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call acceptAsync(String mediatype, String id, AcceptData6 acceptData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = acceptValidateBeforeCall(mediatype, id, acceptData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for addAttachment
* @param mediatype media-type of interaction to add attachment (required)
* @param id id of interaction (required)
* @param attachment The file to upload. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call addAttachmentCall(String mediatype, String id, File attachment, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/media/{mediatype}/interactions/{id}/add-attachment"
.replaceAll("\\{" + "mediatype" + "\\}", apiClient.escapeString(mediatype.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
if (attachment != null)
localVarFormParams.put("attachment", attachment);
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"multipart/form-data"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call addAttachmentValidateBeforeCall(String mediatype, String id, File attachment, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'mediatype' is set
if (mediatype == null) {
throw new ApiException("Missing the required parameter 'mediatype' when calling addAttachment(Async)");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling addAttachment(Async)");
}
com.squareup.okhttp.Call call = addAttachmentCall(mediatype, id, attachment, progressListener, progressRequestListener);
return call;
}
/**
* Add an attachment to the open-media interaction
* Add an attachment to the interaction specified in the id path parameter
* @param mediatype media-type of interaction to add attachment (required)
* @param id id of interaction (required)
* @param attachment The file to upload. (optional)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse addAttachment(String mediatype, String id, File attachment) throws ApiException {
ApiResponse resp = addAttachmentWithHttpInfo(mediatype, id, attachment);
return resp.getData();
}
/**
* Add an attachment to the open-media interaction
* Add an attachment to the interaction specified in the id path parameter
* @param mediatype media-type of interaction to add attachment (required)
* @param id id of interaction (required)
* @param attachment The file to upload. (optional)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse addAttachmentWithHttpInfo(String mediatype, String id, File attachment) throws ApiException {
com.squareup.okhttp.Call call = addAttachmentValidateBeforeCall(mediatype, id, attachment, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Add an attachment to the open-media interaction (asynchronously)
* Add an attachment to the interaction specified in the id path parameter
* @param mediatype media-type of interaction to add attachment (required)
* @param id id of interaction (required)
* @param attachment The file to upload. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call addAttachmentAsync(String mediatype, String id, File attachment, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = addAttachmentValidateBeforeCall(mediatype, id, attachment, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for addContent
* @param mediatype media-type of interaction (required)
* @param id id of the interaction (required)
* @param addContentData (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call addContentCall(String mediatype, String id, AddContentData addContentData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = addContentData;
// create path and map variables
String localVarPath = "/media/{mediatype}/interactions/{id}/add-content"
.replaceAll("\\{" + "mediatype" + "\\}", apiClient.escapeString(mediatype.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call addContentValidateBeforeCall(String mediatype, String id, AddContentData addContentData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'mediatype' is set
if (mediatype == null) {
throw new ApiException("Missing the required parameter 'mediatype' when calling addContent(Async)");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling addContent(Async)");
}
com.squareup.okhttp.Call call = addContentCall(mediatype, id, addContentData, progressListener, progressRequestListener);
return call;
}
/**
* Create the interaction in UCS database
* Create the interaction in UCS database
* @param mediatype media-type of interaction (required)
* @param id id of the interaction (required)
* @param addContentData (optional)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse addContent(String mediatype, String id, AddContentData addContentData) throws ApiException {
ApiResponse resp = addContentWithHttpInfo(mediatype, id, addContentData);
return resp.getData();
}
/**
* Create the interaction in UCS database
* Create the interaction in UCS database
* @param mediatype media-type of interaction (required)
* @param id id of the interaction (required)
* @param addContentData (optional)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse addContentWithHttpInfo(String mediatype, String id, AddContentData addContentData) throws ApiException {
com.squareup.okhttp.Call call = addContentValidateBeforeCall(mediatype, id, addContentData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Create the interaction in UCS database (asynchronously)
* Create the interaction in UCS database
* @param mediatype media-type of interaction (required)
* @param id id of the interaction (required)
* @param addContentData (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call addContentAsync(String mediatype, String id, AddContentData addContentData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = addContentValidateBeforeCall(mediatype, id, addContentData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for addDocument
* @param mediatype media-type of interaction to add attachment (required)
* @param id id of interaction (required)
* @param formData (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call addDocumentCall(String mediatype, String id, FormData formData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = formData;
// create path and map variables
String localVarPath = "/media/{mediatype}/interactions/{id}/add-document"
.replaceAll("\\{" + "mediatype" + "\\}", apiClient.escapeString(mediatype.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call addDocumentValidateBeforeCall(String mediatype, String id, FormData formData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'mediatype' is set
if (mediatype == null) {
throw new ApiException("Missing the required parameter 'mediatype' when calling addDocument(Async)");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling addDocument(Async)");
}
// verify the required parameter 'formData' is set
if (formData == null) {
throw new ApiException("Missing the required parameter 'formData' when calling addDocument(Async)");
}
com.squareup.okhttp.Call call = addDocumentCall(mediatype, id, formData, progressListener, progressRequestListener);
return call;
}
/**
* Add an attachment to the open-media interaction
* Add an attachment to the interaction specified in the id path parameter
* @param mediatype media-type of interaction to add attachment (required)
* @param id id of interaction (required)
* @param formData (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse addDocument(String mediatype, String id, FormData formData) throws ApiException {
ApiResponse resp = addDocumentWithHttpInfo(mediatype, id, formData);
return resp.getData();
}
/**
* Add an attachment to the open-media interaction
* Add an attachment to the interaction specified in the id path parameter
* @param mediatype media-type of interaction to add attachment (required)
* @param id id of interaction (required)
* @param formData (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse addDocumentWithHttpInfo(String mediatype, String id, FormData formData) throws ApiException {
com.squareup.okhttp.Call call = addDocumentValidateBeforeCall(mediatype, id, formData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Add an attachment to the open-media interaction (asynchronously)
* Add an attachment to the interaction specified in the id path parameter
* @param mediatype media-type of interaction to add attachment (required)
* @param id id of interaction (required)
* @param formData (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call addDocumentAsync(String mediatype, String id, FormData formData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = addDocumentValidateBeforeCall(mediatype, id, formData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for assignContact
* @param mediatype media-type of interaction (required)
* @param id id of interaction (required)
* @param contactId id of contact (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call assignContactCall(String mediatype, String id, String contactId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/media/{mediatype}/interactions/{id}/assign-contact/{contactId}"
.replaceAll("\\{" + "mediatype" + "\\}", apiClient.escapeString(mediatype.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()))
.replaceAll("\\{" + "contactId" + "\\}", apiClient.escapeString(contactId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call assignContactValidateBeforeCall(String mediatype, String id, String contactId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'mediatype' is set
if (mediatype == null) {
throw new ApiException("Missing the required parameter 'mediatype' when calling assignContact(Async)");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling assignContact(Async)");
}
// verify the required parameter 'contactId' is set
if (contactId == null) {
throw new ApiException("Missing the required parameter 'contactId' when calling assignContact(Async)");
}
com.squareup.okhttp.Call call = assignContactCall(mediatype, id, contactId, progressListener, progressRequestListener);
return call;
}
/**
* Assign the contact to the open interaction
* Assign the contact to the open interaction specified in the contactId path parameter
* @param mediatype media-type of interaction (required)
* @param id id of interaction (required)
* @param contactId id of contact (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse assignContact(String mediatype, String id, String contactId) throws ApiException {
ApiResponse resp = assignContactWithHttpInfo(mediatype, id, contactId);
return resp.getData();
}
/**
* Assign the contact to the open interaction
* Assign the contact to the open interaction specified in the contactId path parameter
* @param mediatype media-type of interaction (required)
* @param id id of interaction (required)
* @param contactId id of contact (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse assignContactWithHttpInfo(String mediatype, String id, String contactId) throws ApiException {
com.squareup.okhttp.Call call = assignContactValidateBeforeCall(mediatype, id, contactId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Assign the contact to the open interaction (asynchronously)
* Assign the contact to the open interaction specified in the contactId path parameter
* @param mediatype media-type of interaction (required)
* @param id id of interaction (required)
* @param contactId id of contact (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call assignContactAsync(String mediatype, String id, String contactId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = assignContactValidateBeforeCall(mediatype, id, contactId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for asyncPutOnHold
* @param id The ID of the interaction. (required)
* @param putOnHoldData (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call asyncPutOnHoldCall(String id, PutOnHoldData putOnHoldData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = putOnHoldData;
// create path and map variables
String localVarPath = "/media/chat/interactions/{id}/put-on-hold"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call asyncPutOnHoldValidateBeforeCall(String id, PutOnHoldData putOnHoldData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling asyncPutOnHold(Async)");
}
// verify the required parameter 'putOnHoldData' is set
if (putOnHoldData == null) {
throw new ApiException("Missing the required parameter 'putOnHoldData' when calling asyncPutOnHold(Async)");
}
com.squareup.okhttp.Call call = asyncPutOnHoldCall(id, putOnHoldData, progressListener, progressRequestListener);
return call;
}
/**
* Put the interaction chat on hold
* Put the interaction chat on hold.
* @param id The ID of the interaction. (required)
* @param putOnHoldData (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse asyncPutOnHold(String id, PutOnHoldData putOnHoldData) throws ApiException {
ApiResponse resp = asyncPutOnHoldWithHttpInfo(id, putOnHoldData);
return resp.getData();
}
/**
* Put the interaction chat on hold
* Put the interaction chat on hold.
* @param id The ID of the interaction. (required)
* @param putOnHoldData (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse asyncPutOnHoldWithHttpInfo(String id, PutOnHoldData putOnHoldData) throws ApiException {
com.squareup.okhttp.Call call = asyncPutOnHoldValidateBeforeCall(id, putOnHoldData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Put the interaction chat on hold (asynchronously)
* Put the interaction chat on hold.
* @param id The ID of the interaction. (required)
* @param putOnHoldData (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call asyncPutOnHoldAsync(String id, PutOnHoldData putOnHoldData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = asyncPutOnHoldValidateBeforeCall(id, putOnHoldData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for attachMediaUserData
* @param mediatype The media channel. (required)
* @param id The ID of the interaction. (required)
* @param userData The data to attach to the interaction. This is an array of objects with the properties key, type, and value. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call attachMediaUserDataCall(String mediatype, String id, UserData userData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = userData;
// create path and map variables
String localVarPath = "/media/{mediatype}/interactions/{id}/attach-user-data"
.replaceAll("\\{" + "mediatype" + "\\}", apiClient.escapeString(mediatype.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call attachMediaUserDataValidateBeforeCall(String mediatype, String id, UserData userData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'mediatype' is set
if (mediatype == null) {
throw new ApiException("Missing the required parameter 'mediatype' when calling attachMediaUserData(Async)");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling attachMediaUserData(Async)");
}
// verify the required parameter 'userData' is set
if (userData == null) {
throw new ApiException("Missing the required parameter 'userData' when calling attachMediaUserData(Async)");
}
com.squareup.okhttp.Call call = attachMediaUserDataCall(mediatype, id, userData, progressListener, progressRequestListener);
return call;
}
/**
* Attach user data to the interaction.
* Attach the provided data to the specified interaction.
* @param mediatype The media channel. (required)
* @param id The ID of the interaction. (required)
* @param userData The data to attach to the interaction. This is an array of objects with the properties key, type, and value. (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse attachMediaUserData(String mediatype, String id, UserData userData) throws ApiException {
ApiResponse resp = attachMediaUserDataWithHttpInfo(mediatype, id, userData);
return resp.getData();
}
/**
* Attach user data to the interaction.
* Attach the provided data to the specified interaction.
* @param mediatype The media channel. (required)
* @param id The ID of the interaction. (required)
* @param userData The data to attach to the interaction. This is an array of objects with the properties key, type, and value. (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse attachMediaUserDataWithHttpInfo(String mediatype, String id, UserData userData) throws ApiException {
com.squareup.okhttp.Call call = attachMediaUserDataValidateBeforeCall(mediatype, id, userData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Attach user data to the interaction. (asynchronously)
* Attach the provided data to the specified interaction.
* @param mediatype The media channel. (required)
* @param id The ID of the interaction. (required)
* @param userData The data to attach to the interaction. This is an array of objects with the properties key, type, and value. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call attachMediaUserDataAsync(String mediatype, String id, UserData userData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = attachMediaUserDataValidateBeforeCall(mediatype, id, userData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for attachments
* @param mediatype media-type of interaction (required)
* @param id id of interaction (required)
* @param documentId id of document to get (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call attachmentsCall(String mediatype, String id, String documentId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/media/{mediatype}/interactions/{id}/attachments/{documentId}"
.replaceAll("\\{" + "mediatype" + "\\}", apiClient.escapeString(mediatype.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()))
.replaceAll("\\{" + "documentId" + "\\}", apiClient.escapeString(documentId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/octet-stream"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call attachmentsValidateBeforeCall(String mediatype, String id, String documentId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'mediatype' is set
if (mediatype == null) {
throw new ApiException("Missing the required parameter 'mediatype' when calling attachments(Async)");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling attachments(Async)");
}
// verify the required parameter 'documentId' is set
if (documentId == null) {
throw new ApiException("Missing the required parameter 'documentId' when calling attachments(Async)");
}
com.squareup.okhttp.Call call = attachmentsCall(mediatype, id, documentId, progressListener, progressRequestListener);
return call;
}
/**
* Get the attachment of the interaction
* Get the attachment of the interaction specified in the documentId path parameter
* @param mediatype media-type of interaction (required)
* @param id id of interaction (required)
* @param documentId id of document to get (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String attachments(String mediatype, String id, String documentId) throws ApiException {
ApiResponse resp = attachmentsWithHttpInfo(mediatype, id, documentId);
return resp.getData();
}
/**
* Get the attachment of the interaction
* Get the attachment of the interaction specified in the documentId path parameter
* @param mediatype media-type of interaction (required)
* @param id id of interaction (required)
* @param documentId id of document to get (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse attachmentsWithHttpInfo(String mediatype, String id, String documentId) throws ApiException {
com.squareup.okhttp.Call call = attachmentsValidateBeforeCall(mediatype, id, documentId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get the attachment of the interaction (asynchronously)
* Get the attachment of the interaction specified in the documentId path parameter
* @param mediatype media-type of interaction (required)
* @param id id of interaction (required)
* @param documentId id of document to get (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call attachmentsAsync(String mediatype, String id, String documentId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = attachmentsValidateBeforeCall(mediatype, id, documentId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for complete
* @param mediatype The media channel. (required)
* @param id The ID of the interaction to complete. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call completeCall(String mediatype, String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/media/{mediatype}/interactions/{id}/complete"
.replaceAll("\\{" + "mediatype" + "\\}", apiClient.escapeString(mediatype.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call completeValidateBeforeCall(String mediatype, String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'mediatype' is set
if (mediatype == null) {
throw new ApiException("Missing the required parameter 'mediatype' when calling complete(Async)");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling complete(Async)");
}
com.squareup.okhttp.Call call = completeCall(mediatype, id, progressListener, progressRequestListener);
return call;
}
/**
* Complete the interaction.
* Marks the specified interaction as complete.
* @param mediatype The media channel. (required)
* @param id The ID of the interaction to complete. (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse complete(String mediatype, String id) throws ApiException {
ApiResponse resp = completeWithHttpInfo(mediatype, id);
return resp.getData();
}
/**
* Complete the interaction.
* Marks the specified interaction as complete.
* @param mediatype The media channel. (required)
* @param id The ID of the interaction to complete. (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse completeWithHttpInfo(String mediatype, String id) throws ApiException {
com.squareup.okhttp.Call call = completeValidateBeforeCall(mediatype, id, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Complete the interaction. (asynchronously)
* Marks the specified interaction as complete.
* @param mediatype The media channel. (required)
* @param id The ID of the interaction to complete. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call completeAsync(String mediatype, String id, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = completeValidateBeforeCall(mediatype, id, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for deleteMediaUserData
* @param mediatype The media channel. (required)
* @param id The ID of the interaction. (required)
* @param userData The keys of the data to remove. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call deleteMediaUserDataCall(String mediatype, String id, UserData2 userData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = userData;
// create path and map variables
String localVarPath = "/media/{mediatype}/interactions/{id}/delete-user-data"
.replaceAll("\\{" + "mediatype" + "\\}", apiClient.escapeString(mediatype.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call deleteMediaUserDataValidateBeforeCall(String mediatype, String id, UserData2 userData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'mediatype' is set
if (mediatype == null) {
throw new ApiException("Missing the required parameter 'mediatype' when calling deleteMediaUserData(Async)");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling deleteMediaUserData(Async)");
}
// verify the required parameter 'userData' is set
if (userData == null) {
throw new ApiException("Missing the required parameter 'userData' when calling deleteMediaUserData(Async)");
}
com.squareup.okhttp.Call call = deleteMediaUserDataCall(mediatype, id, userData, progressListener, progressRequestListener);
return call;
}
/**
* Remove key/value pairs from user data.
* Delete data with the specified keys from the interaction's user data.
* @param mediatype The media channel. (required)
* @param id The ID of the interaction. (required)
* @param userData The keys of the data to remove. (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse deleteMediaUserData(String mediatype, String id, UserData2 userData) throws ApiException {
ApiResponse resp = deleteMediaUserDataWithHttpInfo(mediatype, id, userData);
return resp.getData();
}
/**
* Remove key/value pairs from user data.
* Delete data with the specified keys from the interaction's user data.
* @param mediatype The media channel. (required)
* @param id The ID of the interaction. (required)
* @param userData The keys of the data to remove. (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteMediaUserDataWithHttpInfo(String mediatype, String id, UserData2 userData) throws ApiException {
com.squareup.okhttp.Call call = deleteMediaUserDataValidateBeforeCall(mediatype, id, userData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Remove key/value pairs from user data. (asynchronously)
* Delete data with the specified keys from the interaction's user data.
* @param mediatype The media channel. (required)
* @param id The ID of the interaction. (required)
* @param userData The keys of the data to remove. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call deleteMediaUserDataAsync(String mediatype, String id, UserData2 userData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = deleteMediaUserDataValidateBeforeCall(mediatype, id, userData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for dndOff
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call dndOffCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/media/dnd-off";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call dndOffValidateBeforeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = dndOffCall(progressListener, progressRequestListener);
return call;
}
/**
* Turn off Do Not Disturb.
* Turn off Do Not Disturb for the current agent on all media channels.
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse dndOff() throws ApiException {
ApiResponse resp = dndOffWithHttpInfo();
return resp.getData();
}
/**
* Turn off Do Not Disturb.
* Turn off Do Not Disturb for the current agent on all media channels.
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse dndOffWithHttpInfo() throws ApiException {
com.squareup.okhttp.Call call = dndOffValidateBeforeCall(null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Turn off Do Not Disturb. (asynchronously)
* Turn off Do Not Disturb for the current agent on all media channels.
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call dndOffAsync(final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = dndOffValidateBeforeCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for dndOn
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call dndOnCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/media/dnd-on";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call dndOnValidateBeforeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = dndOnCall(progressListener, progressRequestListener);
return call;
}
/**
* Set the agent state to Do Not Disturb.
* Set the current agent's state to Do Not Disturb on all media channels.
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse dndOn() throws ApiException {
ApiResponse resp = dndOnWithHttpInfo();
return resp.getData();
}
/**
* Set the agent state to Do Not Disturb.
* Set the current agent's state to Do Not Disturb on all media channels.
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse dndOnWithHttpInfo() throws ApiException {
com.squareup.okhttp.Call call = dndOnValidateBeforeCall(null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Set the agent state to Do Not Disturb. (asynchronously)
* Set the current agent's state to Do Not Disturb on all media channels.
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call dndOnAsync(final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = dndOnValidateBeforeCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for logoutAgentState
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call logoutAgentStateCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/media/logout";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call logoutAgentStateValidateBeforeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = logoutAgentStateCall(progressListener, progressRequestListener);
return call;
}
/**
* Log out of all media channels.
* Log out the current agent on all media channels. You can make a `/media/{mediatype}/ready` or `/media/{mediatype}/not-ready` request to log in to the media channel again.
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse logoutAgentState() throws ApiException {
ApiResponse resp = logoutAgentStateWithHttpInfo();
return resp.getData();
}
/**
* Log out of all media channels.
* Log out the current agent on all media channels. You can make a `/media/{mediatype}/ready` or `/media/{mediatype}/not-ready` request to log in to the media channel again.
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse logoutAgentStateWithHttpInfo() throws ApiException {
com.squareup.okhttp.Call call = logoutAgentStateValidateBeforeCall(null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Log out of all media channels. (asynchronously)
* Log out the current agent on all media channels. You can make a `/media/{mediatype}/ready` or `/media/{mediatype}/not-ready` request to log in to the media channel again.
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call logoutAgentStateAsync(final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = logoutAgentStateValidateBeforeCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for mediaStartMonitoring
* @param mediatype The media channel. (required)
* @param mediaStartMonitoringData Request parameters. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call mediaStartMonitoringCall(String mediatype, MediaStartMonitoringData mediaStartMonitoringData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = mediaStartMonitoringData;
// create path and map variables
String localVarPath = "/media/{mediatype}/start-monitoring"
.replaceAll("\\{" + "mediatype" + "\\}", apiClient.escapeString(mediatype.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call mediaStartMonitoringValidateBeforeCall(String mediatype, MediaStartMonitoringData mediaStartMonitoringData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'mediatype' is set
if (mediatype == null) {
throw new ApiException("Missing the required parameter 'mediatype' when calling mediaStartMonitoring(Async)");
}
com.squareup.okhttp.Call call = mediaStartMonitoringCall(mediatype, mediaStartMonitoringData, progressListener, progressRequestListener);
return call;
}
/**
* Start monitoring an agent.
* Start supervisor monitoring of an agent on the specified media channel. When an agent being monitored accepts a chat, the supervisor also receives the chat and all related notifications. If the agent is currently in a chat, the supervisor is added to the agent's next chat. The supervisor can't send messages in this mode and only another supervisor can see that the monitoring supervisor joined the chat. If the monitored agent leaves the chat but another agent is still present, the supervisor continues monitoring the chat until it's completed or placed in a queue. Once you've enabled monitoring, you can change the monitoring mode using `/media/{mediatype}/interactions/{id}/switch-to-barge-in`, `/media/{mediatype}/interactions/{id}/switch-to-coach`, and `/media/{mediatype}/interactions/{id}/switch-to-monitor`.
* @param mediatype The media channel. (required)
* @param mediaStartMonitoringData Request parameters. (optional)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse mediaStartMonitoring(String mediatype, MediaStartMonitoringData mediaStartMonitoringData) throws ApiException {
ApiResponse resp = mediaStartMonitoringWithHttpInfo(mediatype, mediaStartMonitoringData);
return resp.getData();
}
/**
* Start monitoring an agent.
* Start supervisor monitoring of an agent on the specified media channel. When an agent being monitored accepts a chat, the supervisor also receives the chat and all related notifications. If the agent is currently in a chat, the supervisor is added to the agent's next chat. The supervisor can't send messages in this mode and only another supervisor can see that the monitoring supervisor joined the chat. If the monitored agent leaves the chat but another agent is still present, the supervisor continues monitoring the chat until it's completed or placed in a queue. Once you've enabled monitoring, you can change the monitoring mode using `/media/{mediatype}/interactions/{id}/switch-to-barge-in`, `/media/{mediatype}/interactions/{id}/switch-to-coach`, and `/media/{mediatype}/interactions/{id}/switch-to-monitor`.
* @param mediatype The media channel. (required)
* @param mediaStartMonitoringData Request parameters. (optional)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse mediaStartMonitoringWithHttpInfo(String mediatype, MediaStartMonitoringData mediaStartMonitoringData) throws ApiException {
com.squareup.okhttp.Call call = mediaStartMonitoringValidateBeforeCall(mediatype, mediaStartMonitoringData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Start monitoring an agent. (asynchronously)
* Start supervisor monitoring of an agent on the specified media channel. When an agent being monitored accepts a chat, the supervisor also receives the chat and all related notifications. If the agent is currently in a chat, the supervisor is added to the agent's next chat. The supervisor can't send messages in this mode and only another supervisor can see that the monitoring supervisor joined the chat. If the monitored agent leaves the chat but another agent is still present, the supervisor continues monitoring the chat until it's completed or placed in a queue. Once you've enabled monitoring, you can change the monitoring mode using `/media/{mediatype}/interactions/{id}/switch-to-barge-in`, `/media/{mediatype}/interactions/{id}/switch-to-coach`, and `/media/{mediatype}/interactions/{id}/switch-to-monitor`.
* @param mediatype The media channel. (required)
* @param mediaStartMonitoringData Request parameters. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call mediaStartMonitoringAsync(String mediatype, MediaStartMonitoringData mediaStartMonitoringData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = mediaStartMonitoringValidateBeforeCall(mediatype, mediaStartMonitoringData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for mediaStopMonitoring
* @param mediatype The media channel. (required)
* @param mediaStopMonitoringData Request parameters. (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call mediaStopMonitoringCall(String mediatype, MediaStopMonitoringData mediaStopMonitoringData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = mediaStopMonitoringData;
// create path and map variables
String localVarPath = "/media/{mediatype}/stop-monitoring"
.replaceAll("\\{" + "mediatype" + "\\}", apiClient.escapeString(mediatype.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call mediaStopMonitoringValidateBeforeCall(String mediatype, MediaStopMonitoringData mediaStopMonitoringData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'mediatype' is set
if (mediatype == null) {
throw new ApiException("Missing the required parameter 'mediatype' when calling mediaStopMonitoring(Async)");
}
com.squareup.okhttp.Call call = mediaStopMonitoringCall(mediatype, mediaStopMonitoringData, progressListener, progressRequestListener);
return call;
}
/**
* Stop monitoring an agent.
* Stop supervisor monitoring of an agent on the specified media channel.
* @param mediatype The media channel. (required)
* @param mediaStopMonitoringData Request parameters. (optional)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse mediaStopMonitoring(String mediatype, MediaStopMonitoringData mediaStopMonitoringData) throws ApiException {
ApiResponse resp = mediaStopMonitoringWithHttpInfo(mediatype, mediaStopMonitoringData);
return resp.getData();
}
/**
* Stop monitoring an agent.
* Stop supervisor monitoring of an agent on the specified media channel.
* @param mediatype The media channel. (required)
* @param mediaStopMonitoringData Request parameters. (optional)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse mediaStopMonitoringWithHttpInfo(String mediatype, MediaStopMonitoringData mediaStopMonitoringData) throws ApiException {
com.squareup.okhttp.Call call = mediaStopMonitoringValidateBeforeCall(mediatype, mediaStopMonitoringData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Stop monitoring an agent. (asynchronously)
* Stop supervisor monitoring of an agent on the specified media channel.
* @param mediatype The media channel. (required)
* @param mediaStopMonitoringData Request parameters. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call mediaStopMonitoringAsync(String mediatype, MediaStopMonitoringData mediaStopMonitoringData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = mediaStopMonitoringValidateBeforeCall(mediatype, mediaStopMonitoringData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for mediaSwicthToBargeIn
* @param mediatype The media channel. (required)
* @param id The ID of the chat interaction. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call mediaSwicthToBargeInCall(String mediatype, String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/media/{mediatype}/interactions/{id}/switch-to-barge-in"
.replaceAll("\\{" + "mediatype" + "\\}", apiClient.escapeString(mediatype.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call mediaSwicthToBargeInValidateBeforeCall(String mediatype, String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'mediatype' is set
if (mediatype == null) {
throw new ApiException("Missing the required parameter 'mediatype' when calling mediaSwicthToBargeIn(Async)");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling mediaSwicthToBargeIn(Async)");
}
com.squareup.okhttp.Call call = mediaSwicthToBargeInCall(mediatype, id, progressListener, progressRequestListener);
return call;
}
/**
* Switch to the barge-in monitoring mode.
* Switch to the barge-in monitoring mode for the specified chat. Both the agent and the customer can see the supervisor's messages.
* @param mediatype The media channel. (required)
* @param id The ID of the chat interaction. (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse mediaSwicthToBargeIn(String mediatype, String id) throws ApiException {
ApiResponse resp = mediaSwicthToBargeInWithHttpInfo(mediatype, id);
return resp.getData();
}
/**
* Switch to the barge-in monitoring mode.
* Switch to the barge-in monitoring mode for the specified chat. Both the agent and the customer can see the supervisor's messages.
* @param mediatype The media channel. (required)
* @param id The ID of the chat interaction. (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse mediaSwicthToBargeInWithHttpInfo(String mediatype, String id) throws ApiException {
com.squareup.okhttp.Call call = mediaSwicthToBargeInValidateBeforeCall(mediatype, id, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Switch to the barge-in monitoring mode. (asynchronously)
* Switch to the barge-in monitoring mode for the specified chat. Both the agent and the customer can see the supervisor's messages.
* @param mediatype The media channel. (required)
* @param id The ID of the chat interaction. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call mediaSwicthToBargeInAsync(String mediatype, String id, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = mediaSwicthToBargeInValidateBeforeCall(mediatype, id, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for mediaSwicthToCoach
* @param mediatype The media channel. (required)
* @param id The ID of the chat interaction. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call mediaSwicthToCoachCall(String mediatype, String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/media/{mediatype}/interactions/{id}/switch-to-coach"
.replaceAll("\\{" + "mediatype" + "\\}", apiClient.escapeString(mediatype.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call mediaSwicthToCoachValidateBeforeCall(String mediatype, String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'mediatype' is set
if (mediatype == null) {
throw new ApiException("Missing the required parameter 'mediatype' when calling mediaSwicthToCoach(Async)");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling mediaSwicthToCoach(Async)");
}
com.squareup.okhttp.Call call = mediaSwicthToCoachCall(mediatype, id, progressListener, progressRequestListener);
return call;
}
/**
* Switch to the coach monitoring mode.
* Switch to the coach monitoring mode for the specified chat. Only the agent can see the supervisor's messages.
* @param mediatype The media channel. (required)
* @param id The ID of the chat interaction. (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse mediaSwicthToCoach(String mediatype, String id) throws ApiException {
ApiResponse resp = mediaSwicthToCoachWithHttpInfo(mediatype, id);
return resp.getData();
}
/**
* Switch to the coach monitoring mode.
* Switch to the coach monitoring mode for the specified chat. Only the agent can see the supervisor's messages.
* @param mediatype The media channel. (required)
* @param id The ID of the chat interaction. (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse mediaSwicthToCoachWithHttpInfo(String mediatype, String id) throws ApiException {
com.squareup.okhttp.Call call = mediaSwicthToCoachValidateBeforeCall(mediatype, id, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Switch to the coach monitoring mode. (asynchronously)
* Switch to the coach monitoring mode for the specified chat. Only the agent can see the supervisor's messages.
* @param mediatype The media channel. (required)
* @param id The ID of the chat interaction. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call mediaSwicthToCoachAsync(String mediatype, String id, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = mediaSwicthToCoachValidateBeforeCall(mediatype, id, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for mediaSwicthToMonitor
* @param mediatype The media channel. (required)
* @param id The ID of the chat interaction. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call mediaSwicthToMonitorCall(String mediatype, String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/media/{mediatype}/interactions/{id}/switch-to-monitor"
.replaceAll("\\{" + "mediatype" + "\\}", apiClient.escapeString(mediatype.toString()))
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call mediaSwicthToMonitorValidateBeforeCall(String mediatype, String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'mediatype' is set
if (mediatype == null) {
throw new ApiException("Missing the required parameter 'mediatype' when calling mediaSwicthToMonitor(Async)");
}
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException("Missing the required parameter 'id' when calling mediaSwicthToMonitor(Async)");
}
com.squareup.okhttp.Call call = mediaSwicthToMonitorCall(mediatype, id, progressListener, progressRequestListener);
return call;
}
/**
* Switch to the monitor mode.
* Switch to the monitor mode for the specified chat. The supervisor can't send messages in this mode and only another supervisor can see that the monitoring supervisor joined the chat.
* @param mediatype The media channel. (required)
* @param id The ID of the chat interaction. (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse mediaSwicthToMonitor(String mediatype, String id) throws ApiException {
ApiResponse resp = mediaSwicthToMonitorWithHttpInfo(mediatype, id);
return resp.getData();
}
/**
* Switch to the monitor mode.
* Switch to the monitor mode for the specified chat. The supervisor can't send messages in this mode and only another supervisor can see that the monitoring supervisor joined the chat.
* @param mediatype The media channel. (required)
* @param id The ID of the chat interaction. (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse mediaSwicthToMonitorWithHttpInfo(String mediatype, String id) throws ApiException {
com.squareup.okhttp.Call call = mediaSwicthToMonitorValidateBeforeCall(mediatype, id, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Switch to the monitor mode. (asynchronously)
* Switch to the monitor mode for the specified chat. The supervisor can't send messages in this mode and only another supervisor can see that the monitoring supervisor joined the chat.
* @param mediatype The media channel. (required)
* @param id The ID of the chat interaction. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call mediaSwicthToMonitorAsync(String mediatype, String id, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = mediaSwicthToMonitorValidateBeforeCall(mediatype, id, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for notReadyAgentState
* @param notReadyForAgentData (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call notReadyAgentStateCall(NotReadyForAgentData notReadyForAgentData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = notReadyForAgentData;
// create path and map variables
String localVarPath = "/media/not-ready";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call notReadyAgentStateValidateBeforeCall(NotReadyForAgentData notReadyForAgentData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'notReadyForAgentData' is set
if (notReadyForAgentData == null) {
throw new ApiException("Missing the required parameter 'notReadyForAgentData' when calling notReadyAgentState(Async)");
}
com.squareup.okhttp.Call call = notReadyAgentStateCall(notReadyForAgentData, progressListener, progressRequestListener);
return call;
}
/**
* Set the agent state to Not Ready.
* Set the current agent's state to Not Ready on all media channels.
* @param notReadyForAgentData (required)
* @return ApiSuccessResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiSuccessResponse notReadyAgentState(NotReadyForAgentData notReadyForAgentData) throws ApiException {
ApiResponse resp = notReadyAgentStateWithHttpInfo(notReadyForAgentData);
return resp.getData();
}
/**
* Set the agent state to Not Ready.
* Set the current agent's state to Not Ready on all media channels.
* @param notReadyForAgentData (required)
* @return ApiResponse<ApiSuccessResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse notReadyAgentStateWithHttpInfo(NotReadyForAgentData notReadyForAgentData) throws ApiException {
com.squareup.okhttp.Call call = notReadyAgentStateValidateBeforeCall(notReadyForAgentData, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Set the agent state to Not Ready. (asynchronously)
* Set the current agent's state to Not Ready on all media channels.
* @param notReadyForAgentData (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call notReadyAgentStateAsync(NotReadyForAgentData notReadyForAgentData, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = notReadyAgentStateValidateBeforeCall(notReadyForAgentData, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for notReadyForMedia
* @param mediatype The media channel. (required)
* @param notReadyForMediaData (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call notReadyForMediaCall(String mediatype, NotReadyForMediaData notReadyForMediaData, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = notReadyForMediaData;
// create path and map variables
String localVarPath = "/media/{mediatype}/not-ready"
.replaceAll("\\{" + "mediatype" + "\\}", apiClient.escapeString(mediatype.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList