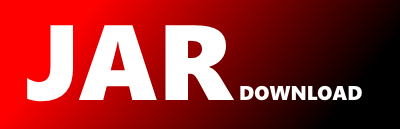
com.genexus.gxserver.client.services.contracts.ObjectFactory Maven / Gradle / Ivy
package com.genexus.gxserver.client.services.contracts;
import javax.xml.namespace.QName;
import jakarta.xml.bind.JAXBElement;
import jakarta.xml.bind.annotation.XmlElementDecl;
import jakarta.xml.bind.annotation.XmlRegistry;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.genexus.gxserver.client.services.contracts package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _ArrayOfServerMessage_QNAME = new QName("http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", "ArrayOfServerMessage");
private final static QName _ServerMessage_QNAME = new QName("http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", "ServerMessage");
private final static QName _MessageType_QNAME = new QName("http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", "MessageType");
private final static QName _Messages_QNAME = new QName("http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", "Messages");
private final static QName _ArrayOfTransferProp_QNAME = new QName("http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", "ArrayOfTransferProp");
private final static QName _TransferProp_QNAME = new QName("http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", "TransferProp");
private final static QName _DateTimeProp_QNAME = new QName("http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", "DateTimeProp");
private final static QName _GuidProp_QNAME = new QName("http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", "GuidProp");
private final static QName _IntProp_QNAME = new QName("http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", "IntProp");
private final static QName _StringProp_QNAME = new QName("http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", "StringProp");
private final static QName _BoolProp_QNAME = new QName("http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", "BoolProp");
private final static QName _XmlProp_QNAME = new QName("http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", "XmlProp");
private final static QName _LongProp_QNAME = new QName("http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", "LongProp");
private final static QName _Properties_QNAME = new QName("http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", "Properties");
private final static QName _FileByteStream_QNAME = new QName("http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", "FileByteStream");
private final static QName _GXServerException_QNAME = new QName("http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", "GXServerException");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.genexus.gxserver.client.services.contracts
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link ArrayOfServerMessage }
*
*/
public ArrayOfServerMessage createArrayOfServerMessage() {
return new ArrayOfServerMessage();
}
/**
* Create an instance of {@link ServerMessage }
*
*/
public ServerMessage createServerMessage() {
return new ServerMessage();
}
/**
* Create an instance of {@link ArrayOfTransferProp }
*
*/
public ArrayOfTransferProp createArrayOfTransferProp() {
return new ArrayOfTransferProp();
}
/**
* Create an instance of {@link TransferProp }
*
*/
public TransferProp createTransferProp() {
return new TransferProp();
}
/**
* Create an instance of {@link DateTimeProp }
*
*/
public DateTimeProp createDateTimeProp() {
return new DateTimeProp();
}
/**
* Create an instance of {@link GuidProp }
*
*/
public GuidProp createGuidProp() {
return new GuidProp();
}
/**
* Create an instance of {@link IntProp }
*
*/
public IntProp createIntProp() {
return new IntProp();
}
/**
* Create an instance of {@link StringProp }
*
*/
public StringProp createStringProp() {
return new StringProp();
}
/**
* Create an instance of {@link BoolProp }
*
*/
public BoolProp createBoolProp() {
return new BoolProp();
}
/**
* Create an instance of {@link XmlProp }
*
*/
public XmlProp createXmlProp() {
return new XmlProp();
}
/**
* Create an instance of {@link LongProp }
*
*/
public LongProp createLongProp() {
return new LongProp();
}
/**
* Create an instance of {@link GXServerException }
*
*/
public GXServerException createGXServerException() {
return new GXServerException();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ArrayOfServerMessage }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ArrayOfServerMessage }{@code >}
*/
@XmlElementDecl(namespace = "http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", name = "ArrayOfServerMessage")
public JAXBElement createArrayOfServerMessage(ArrayOfServerMessage value) {
return new JAXBElement(_ArrayOfServerMessage_QNAME, ArrayOfServerMessage.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ServerMessage }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ServerMessage }{@code >}
*/
@XmlElementDecl(namespace = "http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", name = "ServerMessage")
public JAXBElement createServerMessage(ServerMessage value) {
return new JAXBElement(_ServerMessage_QNAME, ServerMessage.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link MessageType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link MessageType }{@code >}
*/
@XmlElementDecl(namespace = "http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", name = "MessageType")
public JAXBElement createMessageType(MessageType value) {
return new JAXBElement(_MessageType_QNAME, MessageType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ArrayOfServerMessage }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ArrayOfServerMessage }{@code >}
*/
@XmlElementDecl(namespace = "http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", name = "Messages")
public JAXBElement createMessages(ArrayOfServerMessage value) {
return new JAXBElement(_Messages_QNAME, ArrayOfServerMessage.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ArrayOfTransferProp }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ArrayOfTransferProp }{@code >}
*/
@XmlElementDecl(namespace = "http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", name = "ArrayOfTransferProp")
public JAXBElement createArrayOfTransferProp(ArrayOfTransferProp value) {
return new JAXBElement(_ArrayOfTransferProp_QNAME, ArrayOfTransferProp.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link TransferProp }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link TransferProp }{@code >}
*/
@XmlElementDecl(namespace = "http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", name = "TransferProp")
public JAXBElement createTransferProp(TransferProp value) {
return new JAXBElement(_TransferProp_QNAME, TransferProp.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link DateTimeProp }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link DateTimeProp }{@code >}
*/
@XmlElementDecl(namespace = "http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", name = "DateTimeProp")
public JAXBElement createDateTimeProp(DateTimeProp value) {
return new JAXBElement(_DateTimeProp_QNAME, DateTimeProp.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link GuidProp }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link GuidProp }{@code >}
*/
@XmlElementDecl(namespace = "http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", name = "GuidProp")
public JAXBElement createGuidProp(GuidProp value) {
return new JAXBElement(_GuidProp_QNAME, GuidProp.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link IntProp }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link IntProp }{@code >}
*/
@XmlElementDecl(namespace = "http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", name = "IntProp")
public JAXBElement createIntProp(IntProp value) {
return new JAXBElement(_IntProp_QNAME, IntProp.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StringProp }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link StringProp }{@code >}
*/
@XmlElementDecl(namespace = "http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", name = "StringProp")
public JAXBElement createStringProp(StringProp value) {
return new JAXBElement(_StringProp_QNAME, StringProp.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BoolProp }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BoolProp }{@code >}
*/
@XmlElementDecl(namespace = "http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", name = "BoolProp")
public JAXBElement createBoolProp(BoolProp value) {
return new JAXBElement(_BoolProp_QNAME, BoolProp.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link XmlProp }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link XmlProp }{@code >}
*/
@XmlElementDecl(namespace = "http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", name = "XmlProp")
public JAXBElement createXmlProp(XmlProp value) {
return new JAXBElement(_XmlProp_QNAME, XmlProp.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link LongProp }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link LongProp }{@code >}
*/
@XmlElementDecl(namespace = "http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", name = "LongProp")
public JAXBElement createLongProp(LongProp value) {
return new JAXBElement(_LongProp_QNAME, LongProp.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ArrayOfTransferProp }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ArrayOfTransferProp }{@code >}
*/
@XmlElementDecl(namespace = "http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", name = "Properties")
public JAXBElement createProperties(ArrayOfTransferProp value) {
return new JAXBElement(_Properties_QNAME, ArrayOfTransferProp.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link byte[]}{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link byte[]}{@code >}
*/
@XmlElementDecl(namespace = "http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", name = "FileByteStream")
public JAXBElement createFileByteStream(byte[] value) {
return new JAXBElement(_FileByteStream_QNAME, byte[].class, null, ((byte[]) value));
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link GXServerException }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link GXServerException }{@code >}
*/
@XmlElementDecl(namespace = "http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", name = "GXServerException")
public JAXBElement createGXServerException(GXServerException value) {
return new JAXBElement(_GXServerException_QNAME, GXServerException.class, null, value);
}
}