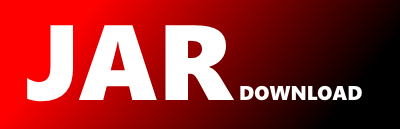
com.genexus.gxserver.client.services.helper.IServerHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gxserver-client Show documentation
Show all versions of gxserver-client Show documentation
Java client for GeneXus Server services
The newest version!
package com.genexus.gxserver.client.services.helper;
import javax.xml.datatype.XMLGregorianCalendar;
import com.genexus.gxserver.client.services.contracts.ArrayOfServerMessage;
import com.genexus.gxserver.client.services.contracts.ArrayOfTransferProp;
import com.genexus.gxserver.client.services.serialization.arrays.ArrayOfstring;
import jakarta.jws.HandlerChain;
import jakarta.jws.WebMethod;
import jakarta.jws.WebParam;
import jakarta.jws.WebResult;
import jakarta.jws.WebService;
import jakarta.jws.soap.SOAPBinding;
import jakarta.xml.bind.annotation.XmlSeeAlso;
import jakarta.xml.ws.Holder;
import jakarta.xml.ws.RequestWrapper;
import jakarta.xml.ws.ResponseWrapper;
/**
* This class was generated by the JAX-WS RI.
* JAX-WS RI 3.0.1
* Generated source version: 3.0
*
*/
@WebService(name = "IServerHelper", targetNamespace = "http://tempuri.org/")
@HandlerChain(file = "IServerHelper_handler.xml")
@XmlSeeAlso({
com.genexus.gxserver.client.services.contracts.ObjectFactory.class,
com.genexus.gxserver.client.services.helper.ObjectFactory.class,
com.genexus.gxserver.client.services.serialization.ObjectFactory.class,
com.genexus.gxserver.client.services.serialization.arrays.ObjectFactory.class
})
public interface IServerHelper {
/**
*
* @param clientVersion
* @return
* returns java.lang.Boolean
* @throws IServerHelperIsServerAliveGXServerExceptionFaultFaultMessage
*/
@WebMethod(operationName = "IsServerAlive", action = "http://tempuri.org/IServerHelper/IsServerAlive")
@WebResult(name = "IsServerAliveResult", targetNamespace = "http://tempuri.org/")
@RequestWrapper(localName = "IsServerAlive", targetNamespace = "http://tempuri.org/", className = "com.genexus.gxserver.client.services.helper.IsServerAlive")
@ResponseWrapper(localName = "IsServerAliveResponse", targetNamespace = "http://tempuri.org/", className = "com.genexus.gxserver.client.services.helper.IsServerAliveResponse")
public Boolean isServerAlive(
@WebParam(name = "clientVersion", targetNamespace = "http://tempuri.org/")
String clientVersion)
throws IServerHelperIsServerAliveGXServerExceptionFaultFaultMessage
;
/**
*
* @param kbName
* @return
* returns java.lang.Boolean
* @throws IServerHelperIsKBHostedGXServerExceptionFaultFaultMessage
*/
@WebMethod(operationName = "IsKBHosted", action = "http://tempuri.org/IServerHelper/IsKBHosted")
@WebResult(name = "IsKBHostedResult", targetNamespace = "http://tempuri.org/")
@RequestWrapper(localName = "IsKBHosted", targetNamespace = "http://tempuri.org/", className = "com.genexus.gxserver.client.services.helper.IsKBHosted")
@ResponseWrapper(localName = "IsKBHostedResponse", targetNamespace = "http://tempuri.org/", className = "com.genexus.gxserver.client.services.helper.IsKBHostedResponse")
public Boolean isKBHosted(
@WebParam(name = "kbName", targetNamespace = "http://tempuri.org/")
String kbName)
throws IServerHelperIsKBHostedGXServerExceptionFaultFaultMessage
;
/**
*
* @return
* returns java.lang.Boolean
* @throws IServerHelperIsServerSecureGXServerExceptionFaultFaultMessage
*/
@WebMethod(operationName = "IsServerSecure", action = "http://tempuri.org/IServerHelper/IsServerSecure")
@WebResult(name = "IsServerSecureResult", targetNamespace = "http://tempuri.org/")
@RequestWrapper(localName = "IsServerSecure", targetNamespace = "http://tempuri.org/", className = "com.genexus.gxserver.client.services.helper.IsServerSecure")
@ResponseWrapper(localName = "IsServerSecureResponse", targetNamespace = "http://tempuri.org/", className = "com.genexus.gxserver.client.services.helper.IsServerSecureResponse")
public Boolean isServerSecure()
throws IServerHelperIsServerSecureGXServerExceptionFaultFaultMessage
;
/**
*
* @return
* returns com.genexus.gxserver.client.services.serialization.arrays.ArrayOfstring
* @throws IServerHelperAuthenticationTypesGXServerExceptionFaultFaultMessage
*/
@WebMethod(operationName = "AuthenticationTypes", action = "http://tempuri.org/IServerHelper/AuthenticationTypes")
@WebResult(name = "AuthenticationTypesResult", targetNamespace = "http://tempuri.org/")
@RequestWrapper(localName = "AuthenticationTypes", targetNamespace = "http://tempuri.org/", className = "com.genexus.gxserver.client.services.helper.AuthenticationTypes")
@ResponseWrapper(localName = "AuthenticationTypesResponse", targetNamespace = "http://tempuri.org/", className = "com.genexus.gxserver.client.services.helper.AuthenticationTypesResponse")
public ArrayOfstring authenticationTypes()
throws IServerHelperAuthenticationTypesGXServerExceptionFaultFaultMessage
;
/**
*
* @param messages
* @param parameters
* @param properties
* @throws IServerHelperServerInfoGXServerExceptionFaultFaultMessage
*/
@WebMethod(operationName = "ServerInfo", action = "http://tempuri.org/IServerHelper/ServerInfo")
@SOAPBinding(parameterStyle = SOAPBinding.ParameterStyle.BARE)
public void serverInfo(
@WebParam(name = "SimpleTransfer", targetNamespace = "http://tempuri.org/", mode = WebParam.Mode.INOUT, partName = "parameters")
Holder parameters,
@WebParam(name = "Messages", targetNamespace = "http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", header = true, mode = WebParam.Mode.INOUT, partName = "Messages")
Holder messages,
@WebParam(name = "Properties", targetNamespace = "http://schemas.datacontract.org/2004/07/GeneXus.Server.Contracts", header = true, mode = WebParam.Mode.INOUT, partName = "Properties")
Holder properties)
throws IServerHelperServerInfoGXServerExceptionFaultFaultMessage
;
/**
*
* @return
* returns javax.xml.datatype.XMLGregorianCalendar
* @throws IServerHelperServerUTCTimeGXServerExceptionFaultFaultMessage
*/
@WebMethod(operationName = "ServerUTCTime", action = "http://tempuri.org/IServerHelper/ServerUTCTime")
@WebResult(name = "ServerUTCTimeResult", targetNamespace = "http://tempuri.org/")
@RequestWrapper(localName = "ServerUTCTime", targetNamespace = "http://tempuri.org/", className = "com.genexus.gxserver.client.services.helper.ServerUTCTime")
@ResponseWrapper(localName = "ServerUTCTimeResponse", targetNamespace = "http://tempuri.org/", className = "com.genexus.gxserver.client.services.helper.ServerUTCTimeResponse")
public XMLGregorianCalendar serverUTCTime()
throws IServerHelperServerUTCTimeGXServerExceptionFaultFaultMessage
;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy