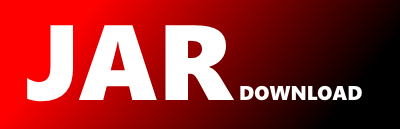
com.genexus.util.GxJsonReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gxandroid Show documentation
Show all versions of gxandroid Show documentation
Core classes for the runtime used by Java and Android apps generated with GeneXus
package com.genexus.util;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.UnsupportedEncodingException;
import org.apache.commons.lang.CharEncoding;
import com.genexus.internet.StringCollection;
import com.google.gson.stream.JsonReader;
public class GxJsonReader {
private JsonReader reader;
public GxJsonReader(InputStream stream) {
try {
reader = new JsonReader(new InputStreamReader(stream, CharEncoding.UTF_8));
} catch (UnsupportedEncodingException e) {
//TODO
}
}
public boolean readBeginArray() {
boolean result;
try {
reader.beginArray();
result = true;
} catch (IOException e) {
result = false;
}
return result;
}
public boolean readEndArray() {
boolean result;
try {
reader.endArray();
result = true;
} catch (IOException e) {
result = false;
}
return result;
}
public boolean endOfArray() {
boolean result;
try {
result = !reader.hasNext();
} catch (IOException e) {
result = true;
}
return result;
}
public StringCollection readNextStringCollection() {
StringCollection stringCollection = new StringCollection();
try {
reader.beginArray();
while (reader.hasNext()) {
String field = reader.nextString();
stringCollection.add(field);
}
reader.endArray();
} catch (IOException e) {
// TODO
}
return stringCollection;
}
public StringCollection parseStringCollection() {
StringCollection stringCollection = new StringCollection();
try {
reader.beginArray();
while (reader.hasNext()) {
String field = reader.nextString();
stringCollection.add(field);
}
reader.endArray();
} catch (IOException e) {
// TODO
}
return stringCollection;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy