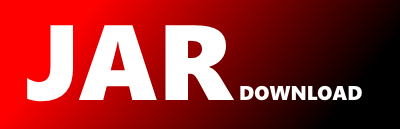
com.genexus.db.driver.DataSourceConnectionPool Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gxclassR Show documentation
Show all versions of gxclassR Show documentation
Core classes for the runtime used by Java and Android apps generated with GeneXus
package com.genexus.db.driver;
import com.genexus.ModelContext;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.Enumeration;
import java.util.concurrent.ConcurrentHashMap;
public final class DataSourceConnectionPool implements IConnectionPool
{
private DataSource dataSource;
private ConcurrentHashMap readOnlyPools ;
private ConcurrentHashMap readWritePools;
private boolean readOnlyPoolEnabled;
DataSourceConnectionPool(DataSource dataSource)
{
this.dataSource = dataSource;
readWritePools = new ConcurrentHashMap();
readOnlyPoolEnabled = dataSource.roPoolEnabled;
readOnlyPools = new ConcurrentHashMap();
}
public Enumeration getROPools()
{
return readOnlyPools.keys();
}
public Enumeration getRWPools()
{
return readWritePools.keys();
}
public ConnectionPool getROConnectionPool(String user)
{
return (ConnectionPool) readOnlyPools.get(user);
}
public ConnectionPool getRWConnectionPool(String user)
{
return (ConnectionPool) readWritePools.get(user);
}
private ConnectionPool newConnectionPool(DataSource dataSource1, String user, String password, boolean readOnly) {
ConnectionPool pool;
if (dataSource1 == null)
dataSource1 = dataSource;
if (readOnly)
pool = new ReadOnlyConnectionPool(dataSource1, user, password);
else
pool = new ReadWriteConnectionPool(dataSource1, user, password);
return pool;
}
public Connection checkOut(ModelContext context, DataSource dataSource1, int handle, String user, String password, boolean readOnly, boolean sticky) throws SQLException
{
ConnectionPool pool = null;
String key = user + password;
ConcurrentHashMap connectionPools = (readOnlyPoolEnabled && readOnly)? readOnlyPools : readWritePools;
if ((pool = (ConnectionPool) connectionPools.get(key)) == null)
{
pool = newConnectionPool(dataSource1, user, password, (readOnlyPoolEnabled && readOnly));
ConnectionPool previousPool = connectionPools.putIfAbsent(key, pool);
if (previousPool != null)
pool = previousPool;
}
return pool.checkOut(context, handle, sticky);
}
public void disconnectOnException(int handle) throws SQLException
{
for (Enumeration en = readWritePools.elements(); en.hasMoreElements(); )
((ConnectionPool) en.nextElement()).disconnectOnException(handle);
for (Enumeration en = readOnlyPools.elements(); en.hasMoreElements(); )
((ConnectionPool) en.nextElement()).disconnectOnException(handle);
}
public void disconnect(int handle) throws SQLException
{
SQLException disconnectException = null;
for (Enumeration en = readWritePools.elements(); en.hasMoreElements(); )
{
try
{
( (ConnectionPool) en.nextElement()).disconnect(handle);
}
catch (SQLException e)
{
disconnectException = e;
}
}
for (Enumeration en = readOnlyPools.elements(); en.hasMoreElements(); )
{
try
{
( (ConnectionPool) en.nextElement()).disconnect(handle);
}
catch (SQLException e)
{
disconnectException = e;
}
}
if (disconnectException != null)
throw disconnectException;
}
public void disconnect() throws SQLException
{
for (Enumeration en = readWritePools.elements(); en.hasMoreElements(); )
en.nextElement().disconnect();
for (Enumeration en = readOnlyPools.elements(); en.hasMoreElements(); )
en.nextElement().disconnect();
}
@Override
public void flushBuffers(int handle, java.lang.Object o) throws SQLException
{
for (Enumeration en = readWritePools.elements(); en.hasMoreElements(); )
{
ConnectionPool connPool = en.nextElement();
for (Enumeration en1 = connPool.getConnections(); en1.hasMoreElements(); ) {
GXConnection conn = (GXConnection) en1.nextElement();
if (conn.getHandle() == handle) {
conn.flushBatchCursors(o);
}
}
}
for (Enumeration en = readOnlyPools.elements(); en.hasMoreElements(); )
{
ConnectionPool connPool = en.nextElement();
for (Enumeration en1 = connPool.getConnections(); en1.hasMoreElements(); ) {
GXConnection conn = (GXConnection) en1.nextElement();
if (conn.getHandle() == handle) {
conn.flushBatchCursors(o);
}
}
}
}
@Override
public void runWithLock(Runnable runnable) {
for (Enumeration en = readWritePools.elements(); en.hasMoreElements(); )
((ConnectionPool) en.nextElement()).runWithLock(runnable);
for (Enumeration en = readOnlyPools.elements(); en.hasMoreElements(); )
((ConnectionPool) en.nextElement()).runWithLock(runnable);
}
@Override
public void removeElement(GXConnection con) {
for (Enumeration en = readWritePools.elements(); en.hasMoreElements(); )
((ConnectionPool) en.nextElement()).removeElement(con);
for (Enumeration en = readOnlyPools.elements(); en.hasMoreElements(); )
((ConnectionPool) en.nextElement()).removeElement(con);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy