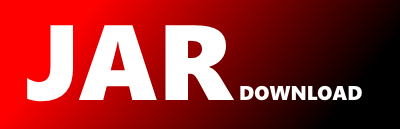
com.genexus.util.LDAPClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gxclassR Show documentation
Show all versions of gxclassR Show documentation
Core classes for the runtime used by Java and Android apps generated with GeneXus
package com.genexus.util;
import com.genexus.diagnostics.core.ILogger;
import com.genexus.diagnostics.core.LogManager;
import java.util.Hashtable;
import java.util.Vector;
import javax.naming.Context;
import javax.naming.NamingEnumeration;
import javax.naming.NamingException;
import javax.naming.directory.Attribute;
import javax.naming.directory.Attributes;
import javax.naming.directory.BasicAttributes;
import javax.naming.directory.DirContext;
import javax.naming.directory.InitialDirContext;
import javax.naming.directory.SearchControls;
import javax.naming.directory.SearchResult;
public class LDAPClient {
private static final ILogger logger = LogManager.getLogger(LDAPClient.class);
String ldapHost;
int port;
String authentication;
String user;
String password;
byte secure;
DirContext ctx;
public LDAPClient() {
port = 389;
authentication = "simple";
user = "";
password = "";
}
public void setHost(String host)
{
ldapHost = host;
}
public void setPort(int port)
{
this.port = port;
}
public void setAuthenticationMethod(String authentication)
{
this.authentication = authentication;
}
public void setUser(String user)
{
this.user = user;
}
public void setPassword(String password)
{
this.password = password;
}
public void setSecure(byte secure)
{
this.secure = secure;
}
public byte connect()
{
String host;
Hashtable env = new Hashtable<>(5);
if (ldapHost.equals(""))
{
return 0;
}
else
{
env.put(Context.INITIAL_CONTEXT_FACTORY,
"com.sun.jndi.ldap.LdapCtxFactory");
host = "ldap://" + ldapHost + ":" + port;
env.put(Context.PROVIDER_URL, host);
env.put(Context.SECURITY_AUTHENTICATION, authentication);
env.put(Context.SECURITY_PRINCIPAL, user);
env.put(Context.SECURITY_CREDENTIALS, password);
if (secure == 1)
{
env.put(Context.SECURITY_PROTOCOL, "ssl");
}
try {
// Create initial context
ctx = new InitialDirContext(env);
}
catch (NamingException e) {
logger.error("Error in connect", e);
return 0;
}
return 1;
}
}
public void disconnect()
{
try
{
ctx.close();
}
catch (NamingException e)
{
logger.error("Error in disconnect", e);
}
}
public Vector getAttribute(String attName, String context, GXProperties ldapAttributes)
{
Vector strResult = new Vector<>();
Attributes matchAttrs = new BasicAttributes(true);
String searchFilter = "";
if (ldapAttributes.count() > 0)
{
searchFilter = "(&";
for(int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy