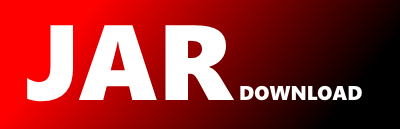
com.genexus.db.odata.ModelInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gxodata Show documentation
Show all versions of gxodata Show documentation
Core classes for the runtime used by Java and Android apps generated with GeneXus
The newest version!
package com.genexus.db.odata;
import java.lang.reflect.Field;
import java.net.URI;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import org.apache.olingo.client.api.http.HttpClientFactory;
import org.apache.olingo.client.core.edm.xml.ClientCsdlAnnotation;
import org.apache.olingo.commons.api.edm.Edm;
import org.apache.olingo.commons.api.edm.EdmAnnotation;
import org.apache.olingo.commons.api.edm.EdmEntityContainer;
import org.apache.olingo.commons.api.edm.EdmEntitySet;
import org.apache.olingo.commons.api.edm.EdmEntityType;
import org.apache.olingo.commons.api.edm.EdmNavigationProperty;
import org.apache.olingo.commons.api.format.ContentType;
public class ModelInfo
{
private static final HashMap serviceModels = new HashMap<>();
static ModelInfo getModel(String connUrl)
{
return serviceModels.get(connUrl);
}
static void addModel(String connUrl, ModelInfo modelInfo)
{
serviceModels.put(connUrl, modelInfo);
}
final String url;
final Edm model;
boolean useChunked;
ContentType defaultContentType = ContentType.JSON_FULL_METADATA;
HashSet recordNotFoundServiceCodes;
HashSet recordAlreadyExistsServiceCodes;
HttpClientFactory handlerFactory = null;
ModelInfo(String url, Edm model, String checkOptimisticConcurrency)
{
this.url = url;
this.model = model;
initializeOptimisticConcurrency(checkOptimisticConcurrency);
initializeEntityMapper(model);
}
public Edm getModel()
{
return model;
}
static final HashMap>> entityMappers = new HashMap<>();
private static final HashMap NIL_MAPPER = new HashMap<>();
private void initializeEntityMapper(Edm model)
{
HashMap> entityMapper = entityMappers.computeIfAbsent(url, k -> new HashMap<>());
HashMap rootMapper = entityMapper.computeIfAbsent(null, k -> new HashMap<>());
EdmEntityContainer container = model.getEntityContainer();
HashMap> entitySetTypes = new HashMap<>();
for(EdmEntitySet entitySet:container.getEntitySets())
{
List entitySets = entitySetTypes.computeIfAbsent(entitySet.getEntityType(), k -> new ArrayList<>());
entitySets.add(entitySet.getName());
}
for(EdmEntitySet entitySet:container.getEntitySets())
{
EdmEntityType type = entitySet.getEntityType();
initializeEntityMapper(entityMapper, type, entitySetTypes);
rootMapper.put(entitySet.getName(), type.getName());
if(!needsCheckOptimisticConcurrencyAll)
{
for(EdmAnnotation annotation:entitySet.getAnnotations())
{ // @hack intento obtener si la actualizacion de este entitySet requiere IfMatch
try
{
Field annotationField = annotation.getClass().getDeclaredField("annotation");
annotationField.setAccessible(true);
ClientCsdlAnnotation csdlAnnotation = (ClientCsdlAnnotation)annotationField.get(annotation);
if(csdlAnnotation.getTerm().endsWith("OptimisticConcurrency"))
{
if(checkOptimisticConcurrencyEntities == null)
checkOptimisticConcurrencyEntities = new HashSet<>();
checkOptimisticConcurrencyEntities.add(entitySet.getName().toLowerCase());
}
}catch(IllegalAccessException | IllegalArgumentException | NoSuchFieldException | SecurityException ignored)
{
}
}
}
}
}
public void initializeEntityMapper(HashMap> entityMapper, EdmEntityType type, HashMap> entitySetTypesMap)
{
HashMap currentMapper = entityMapper.computeIfAbsent(type, k -> new HashMap<>());
for(String navPropName : type.getNavigationPropertyNames())
{
EdmNavigationProperty navProp = type.getNavigationProperty(navPropName);
EdmEntityType navPropType = navProp.getType();
if(!currentMapper.containsKey(navProp.getName()))
{
currentMapper.put(navProp.getName(), navProp.getName()); // Navego por el nombre de la propiedad
if(entitySetTypesMap.containsKey(navPropType))
{ // Si es un entity set en gx se ve como tal
for(String entitySetName:entitySetTypesMap.get(navPropType))
currentMapper.put(entitySetName, navPropName);
}else
{ // si no es un enityset en gx se ve con el tipo de la entidad
currentMapper.put(navPropType.getName(), navProp.getName());
}
if(!navPropType.equals(type))
initializeEntityMapper(entityMapper, navPropType, entitySetTypesMap);
}
}
}
private void initializeOptimisticConcurrency(String checkOptimisticConcurrency)
{
if(checkOptimisticConcurrency != null)
{
checkOptimisticConcurrency = checkOptimisticConcurrency.trim().toLowerCase();
if(checkOptimisticConcurrency.equals("true") || checkOptimisticConcurrency.equals("all"))
needsCheckOptimisticConcurrencyAll = true;
else
{
checkOptimisticConcurrencyEntities = new HashSet<>();
for(String entity:checkOptimisticConcurrency.split(","))
{
checkOptimisticConcurrencyEntities.add(entity.trim());
}
}
}
}
private boolean needsCheckOptimisticConcurrencyAll = false;
private HashSet checkOptimisticConcurrencyEntities = null;
boolean needsCheckOptimisticConcurrency(URI updURI)
{
try
{
if(needsCheckOptimisticConcurrencyAll)
return true;
if(checkOptimisticConcurrencyEntities != null)
{
String path = updURI.getPath().toLowerCase();
path = path.substring(path.lastIndexOf('/')+1);
if(path.contains("("))
path = path.substring(0, path.indexOf('('));
return checkOptimisticConcurrencyEntities.contains(path);
}
}catch(Exception ignored){}
return false;
}
public String entity(String name)
{
HashMap> entityMapper = entityMappers.get(url);
return entityMapper == null ? name : entityMapper.getOrDefault(null, NIL_MAPPER).getOrDefault(name, name);
}
public String entity(EdmEntityType fromEntity, String name)
{
HashMap> entityMapper = entityMappers.get(url);
return entityMapper == null ? name : entityMapper.getOrDefault(fromEntity, NIL_MAPPER).get(name);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy