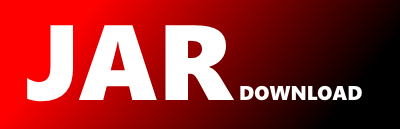
energyml.prodml2_0.DasFbe Maven / Gradle / Ivy
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.0
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2022.10.13 at 08:02:05 PM UTC
//
package energyml.prodml2_0;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlAttribute;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import energyml.common2_1.FrequencyMeasure;
import energyml.common2_1.LengthMeasure;
/**
* This object contains the attributes of FBE processed data. This includes the FBE data unit, location of the FBE data along the fiber optical path, information about times, (optional) filter related parameters, and UUIDs of the original raw and/or spectra files from which the files were processed. Note that the actual FBE data samples and times arrays are not present in the XML files but only in the HDF5 files because of their size. The XML files only contain references to locate the corresponding HDF files containing the actual FBE samples and times.
*
* Java class for DasFbe complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="DasFbe">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="FbeDataUnit" type="energyml.common2_1.String64"/>
* <element name="FbeDescription" type="energyml.common2_1.String2000" maxOccurs="unbounded" minOccurs="0"/>
* <element name="FbeIndex" type="energyml.common2_1.NonNegativeLong" maxOccurs="unbounded" minOccurs="0"/>
* <element name="FilterType" type="energyml.common2_1.String64" maxOccurs="unbounded" minOccurs="0"/>
* <element name="NumberOfLoci" type="energyml.common2_1.NonNegativeLong"/>
* <element name="OutputDataRate" type="energyml.common2_1.FrequencyMeasure"/>
* <element name="RawReference" type="energyml.common2_1.UuidString" maxOccurs="unbounded" minOccurs="0"/>
* <element name="SpatialSamplingInterval" type="energyml.common2_1.LengthMeasure" maxOccurs="unbounded" minOccurs="0"/>
* <element name="SpatialSamplingIntervalUnit" type="energyml.common2_1.String64" maxOccurs="unbounded" minOccurs="0"/>
* <element name="SpectraReference" type="energyml.common2_1.UuidString" maxOccurs="unbounded" minOccurs="0"/>
* <element name="StartLocusIndex" type="energyml.common2_1.NonNegativeLong"/>
* <element name="TransformSize" type="energyml.common2_1.NonNegativeLong" maxOccurs="unbounded" minOccurs="0"/>
* <element name="TransformType" type="energyml.common2_1.String64" maxOccurs="unbounded" minOccurs="0"/>
* <element name="WindowFunction" type="energyml.common2_1.String64" maxOccurs="unbounded" minOccurs="0"/>
* <element name="WindowOverlap" type="energyml.common2_1.NonNegativeLong" maxOccurs="unbounded" minOccurs="0"/>
* <element name="WindowSize" type="energyml.common2_1.NonNegativeLong" maxOccurs="unbounded" minOccurs="0"/>
* <element name="Custom" type="energyml.prodml2_0.DasCustom" minOccurs="0"/>
* <element name="FbeData" type="energyml.prodml2_0.DasFbeData" maxOccurs="unbounded"/>
* <element name="FbeDataTime" type="energyml.prodml2_0.DasTimeArray"/>
* </sequence>
* <attribute name="uuid" use="required" type="energyml.common2_1.UuidString" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "DasFbe", propOrder = {
"fbeDataUnit",
"fbeDescription",
"fbeIndex",
"filterType",
"numberOfLoci",
"outputDataRate",
"rawReference",
"spatialSamplingInterval",
"spatialSamplingIntervalUnit",
"spectraReference",
"startLocusIndex",
"transformSize",
"transformType",
"windowFunction",
"windowOverlap",
"windowSize",
"custom",
"fbeData",
"fbeDataTime"
})
public class DasFbe {
@XmlElement(name = "FbeDataUnit", required = true)
protected String fbeDataUnit;
@XmlElement(name = "FbeDescription")
protected List fbeDescription;
@XmlElement(name = "FbeIndex", type = Long.class)
protected List fbeIndex;
@XmlElement(name = "FilterType")
protected List filterType;
@XmlElement(name = "NumberOfLoci")
protected long numberOfLoci;
@XmlElement(name = "OutputDataRate", required = true)
protected FrequencyMeasure outputDataRate;
@XmlElement(name = "RawReference")
protected List rawReference;
@XmlElement(name = "SpatialSamplingInterval")
protected List spatialSamplingInterval;
@XmlElement(name = "SpatialSamplingIntervalUnit")
protected List spatialSamplingIntervalUnit;
@XmlElement(name = "SpectraReference")
protected List spectraReference;
@XmlElement(name = "StartLocusIndex")
protected long startLocusIndex;
@XmlElement(name = "TransformSize", type = Long.class)
protected List transformSize;
@XmlElement(name = "TransformType")
protected List transformType;
@XmlElement(name = "WindowFunction")
protected List windowFunction;
@XmlElement(name = "WindowOverlap", type = Long.class)
protected List windowOverlap;
@XmlElement(name = "WindowSize", type = Long.class)
protected List windowSize;
@XmlElement(name = "Custom")
protected DasCustom custom;
@XmlElement(name = "FbeData", required = true)
protected List fbeData;
@XmlElement(name = "FbeDataTime", required = true)
protected DasTimeArray fbeDataTime;
@XmlAttribute(name = "uuid", required = true)
protected String uuid;
/**
* Gets the value of the fbeDataUnit property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFbeDataUnit() {
return fbeDataUnit;
}
/**
* Sets the value of the fbeDataUnit property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFbeDataUnit(String value) {
this.fbeDataUnit = value;
}
/**
* Gets the value of the fbeDescription property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the fbeDescription property.
*
*
* For example, to add a new item, do as follows:
*
* getFbeDescription().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getFbeDescription() {
if (fbeDescription == null) {
fbeDescription = new ArrayList();
}
return this.fbeDescription;
}
/**
* Gets the value of the fbeIndex property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the fbeIndex property.
*
*
* For example, to add a new item, do as follows:
*
* getFbeIndex().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Long }
*
*
*/
public List getFbeIndex() {
if (fbeIndex == null) {
fbeIndex = new ArrayList();
}
return this.fbeIndex;
}
/**
* Gets the value of the filterType property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the filterType property.
*
*
* For example, to add a new item, do as follows:
*
* getFilterType().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getFilterType() {
if (filterType == null) {
filterType = new ArrayList();
}
return this.filterType;
}
/**
* Gets the value of the numberOfLoci property.
*
*/
public long getNumberOfLoci() {
return numberOfLoci;
}
/**
* Sets the value of the numberOfLoci property.
*
*/
public void setNumberOfLoci(long value) {
this.numberOfLoci = value;
}
/**
* Gets the value of the outputDataRate property.
*
* @return
* possible object is
* {@link FrequencyMeasure }
*
*/
public FrequencyMeasure getOutputDataRate() {
return outputDataRate;
}
/**
* Sets the value of the outputDataRate property.
*
* @param value
* allowed object is
* {@link FrequencyMeasure }
*
*/
public void setOutputDataRate(FrequencyMeasure value) {
this.outputDataRate = value;
}
/**
* Gets the value of the rawReference property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the rawReference property.
*
*
* For example, to add a new item, do as follows:
*
* getRawReference().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getRawReference() {
if (rawReference == null) {
rawReference = new ArrayList();
}
return this.rawReference;
}
/**
* Gets the value of the spatialSamplingInterval property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the spatialSamplingInterval property.
*
*
* For example, to add a new item, do as follows:
*
* getSpatialSamplingInterval().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link LengthMeasure }
*
*
*/
public List getSpatialSamplingInterval() {
if (spatialSamplingInterval == null) {
spatialSamplingInterval = new ArrayList();
}
return this.spatialSamplingInterval;
}
/**
* Gets the value of the spatialSamplingIntervalUnit property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the spatialSamplingIntervalUnit property.
*
*
* For example, to add a new item, do as follows:
*
* getSpatialSamplingIntervalUnit().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getSpatialSamplingIntervalUnit() {
if (spatialSamplingIntervalUnit == null) {
spatialSamplingIntervalUnit = new ArrayList();
}
return this.spatialSamplingIntervalUnit;
}
/**
* Gets the value of the spectraReference property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the spectraReference property.
*
*
* For example, to add a new item, do as follows:
*
* getSpectraReference().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getSpectraReference() {
if (spectraReference == null) {
spectraReference = new ArrayList();
}
return this.spectraReference;
}
/**
* Gets the value of the startLocusIndex property.
*
*/
public long getStartLocusIndex() {
return startLocusIndex;
}
/**
* Sets the value of the startLocusIndex property.
*
*/
public void setStartLocusIndex(long value) {
this.startLocusIndex = value;
}
/**
* Gets the value of the transformSize property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the transformSize property.
*
*
* For example, to add a new item, do as follows:
*
* getTransformSize().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Long }
*
*
*/
public List getTransformSize() {
if (transformSize == null) {
transformSize = new ArrayList();
}
return this.transformSize;
}
/**
* Gets the value of the transformType property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the transformType property.
*
*
* For example, to add a new item, do as follows:
*
* getTransformType().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getTransformType() {
if (transformType == null) {
transformType = new ArrayList();
}
return this.transformType;
}
/**
* Gets the value of the windowFunction property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the windowFunction property.
*
*
* For example, to add a new item, do as follows:
*
* getWindowFunction().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getWindowFunction() {
if (windowFunction == null) {
windowFunction = new ArrayList();
}
return this.windowFunction;
}
/**
* Gets the value of the windowOverlap property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the windowOverlap property.
*
*
* For example, to add a new item, do as follows:
*
* getWindowOverlap().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Long }
*
*
*/
public List getWindowOverlap() {
if (windowOverlap == null) {
windowOverlap = new ArrayList();
}
return this.windowOverlap;
}
/**
* Gets the value of the windowSize property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the windowSize property.
*
*
* For example, to add a new item, do as follows:
*
* getWindowSize().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Long }
*
*
*/
public List getWindowSize() {
if (windowSize == null) {
windowSize = new ArrayList();
}
return this.windowSize;
}
/**
* Gets the value of the custom property.
*
* @return
* possible object is
* {@link DasCustom }
*
*/
public DasCustom getCustom() {
return custom;
}
/**
* Sets the value of the custom property.
*
* @param value
* allowed object is
* {@link DasCustom }
*
*/
public void setCustom(DasCustom value) {
this.custom = value;
}
/**
* Gets the value of the fbeData property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the fbeData property.
*
*
* For example, to add a new item, do as follows:
*
* getFbeData().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DasFbeData }
*
*
*/
public List getFbeData() {
if (fbeData == null) {
fbeData = new ArrayList();
}
return this.fbeData;
}
/**
* Gets the value of the fbeDataTime property.
*
* @return
* possible object is
* {@link DasTimeArray }
*
*/
public DasTimeArray getFbeDataTime() {
return fbeDataTime;
}
/**
* Sets the value of the fbeDataTime property.
*
* @param value
* allowed object is
* {@link DasTimeArray }
*
*/
public void setFbeDataTime(DasTimeArray value) {
this.fbeDataTime = value;
}
/**
* Gets the value of the uuid property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUuid() {
return uuid;
}
/**
* Sets the value of the uuid property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUuid(String value) {
this.uuid = value;
}
}