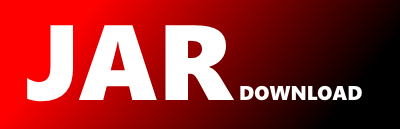
energyml.prodml2_0.FluidCharacterization Maven / Gradle / Ivy
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.0
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2022.10.13 at 08:02:05 PM UTC
//
package energyml.prodml2_0;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import energyml.common2_1.AbstractObject;
import energyml.common2_1.AbstractTemperaturePressure;
import energyml.common2_1.DataObjectReference;
/**
* Fluid characterization.
*
* Java class for FluidCharacterization complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="FluidCharacterization">
* <complexContent>
* <extension base="{http://www.energistics.org/energyml/data/commonv2}AbstractObject">
* <sequence>
* <element name="FluidComponentCatalog" type="energyml.prodml2_0.FluidComponentCatalog" minOccurs="0"/>
* <element name="FluidSystem" type="energyml.common2_1.DataObjectReference" maxOccurs="unbounded" minOccurs="0"/>
* <element name="FluidSystemCharacterizationType" type="energyml.common2_1.String64"/>
* <element name="IntendedUsage" type="energyml.common2_1.String64" maxOccurs="unbounded" minOccurs="0"/>
* <element name="Remark" type="energyml.common2_1.String2000" maxOccurs="unbounded" minOccurs="0"/>
* <element name="RockFluidUnitFeatureReference" type="energyml.common2_1.DataObjectReference" maxOccurs="unbounded" minOccurs="0"/>
* <element name="StandardConditions" type="energyml.common2_1.AbstractTemperaturePressure" maxOccurs="unbounded" minOccurs="0"/>
* <element name="ApplicationSource" type="energyml.prodml2_0.ApplicationInfo" minOccurs="0"/>
* <element name="ApplicationTarget" type="energyml.prodml2_0.ApplicationInfo" maxOccurs="unbounded" minOccurs="0"/>
* <element name="FluidCharacterizationModel" type="energyml.prodml2_0.FluidCharacterizationModel" maxOccurs="unbounded" minOccurs="0"/>
* <element name="FluidCharacterizationSource" type="energyml.prodml2_0.FluidCharacterizationSource" maxOccurs="unbounded" minOccurs="0"/>
* <element name="FluidCharacterizationTableFormatSet" type="energyml.prodml2_0.FluidCharacterizationTableFormatSet" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "FluidCharacterization", propOrder = {
"fluidComponentCatalog",
"fluidSystem",
"fluidSystemCharacterizationType",
"intendedUsage",
"remark",
"rockFluidUnitFeatureReference",
"standardConditions",
"applicationSource",
"applicationTarget",
"fluidCharacterizationModel",
"fluidCharacterizationSource",
"fluidCharacterizationTableFormatSet"
})
public class FluidCharacterization
extends AbstractObject
{
@XmlElement(name = "FluidComponentCatalog")
protected FluidComponentCatalog fluidComponentCatalog;
@XmlElement(name = "FluidSystem")
protected List fluidSystem;
@XmlElement(name = "FluidSystemCharacterizationType", required = true)
protected String fluidSystemCharacterizationType;
@XmlElement(name = "IntendedUsage")
protected List intendedUsage;
@XmlElement(name = "Remark")
protected List remark;
@XmlElement(name = "RockFluidUnitFeatureReference")
protected List rockFluidUnitFeatureReference;
@XmlElement(name = "StandardConditions")
protected List standardConditions;
@XmlElement(name = "ApplicationSource")
protected ApplicationInfo applicationSource;
@XmlElement(name = "ApplicationTarget")
protected List applicationTarget;
@XmlElement(name = "FluidCharacterizationModel")
protected List fluidCharacterizationModel;
@XmlElement(name = "FluidCharacterizationSource")
protected List fluidCharacterizationSource;
@XmlElement(name = "FluidCharacterizationTableFormatSet")
protected FluidCharacterizationTableFormatSet fluidCharacterizationTableFormatSet;
/**
* Gets the value of the fluidComponentCatalog property.
*
* @return
* possible object is
* {@link FluidComponentCatalog }
*
*/
public FluidComponentCatalog getFluidComponentCatalog() {
return fluidComponentCatalog;
}
/**
* Sets the value of the fluidComponentCatalog property.
*
* @param value
* allowed object is
* {@link FluidComponentCatalog }
*
*/
public void setFluidComponentCatalog(FluidComponentCatalog value) {
this.fluidComponentCatalog = value;
}
/**
* Gets the value of the fluidSystem property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the fluidSystem property.
*
*
* For example, to add a new item, do as follows:
*
* getFluidSystem().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DataObjectReference }
*
*
*/
public List getFluidSystem() {
if (fluidSystem == null) {
fluidSystem = new ArrayList();
}
return this.fluidSystem;
}
/**
* Gets the value of the fluidSystemCharacterizationType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFluidSystemCharacterizationType() {
return fluidSystemCharacterizationType;
}
/**
* Sets the value of the fluidSystemCharacterizationType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFluidSystemCharacterizationType(String value) {
this.fluidSystemCharacterizationType = value;
}
/**
* Gets the value of the intendedUsage property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the intendedUsage property.
*
*
* For example, to add a new item, do as follows:
*
* getIntendedUsage().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getIntendedUsage() {
if (intendedUsage == null) {
intendedUsage = new ArrayList();
}
return this.intendedUsage;
}
/**
* Gets the value of the remark property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the remark property.
*
*
* For example, to add a new item, do as follows:
*
* getRemark().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getRemark() {
if (remark == null) {
remark = new ArrayList();
}
return this.remark;
}
/**
* Gets the value of the rockFluidUnitFeatureReference property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the rockFluidUnitFeatureReference property.
*
*
* For example, to add a new item, do as follows:
*
* getRockFluidUnitFeatureReference().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DataObjectReference }
*
*
*/
public List getRockFluidUnitFeatureReference() {
if (rockFluidUnitFeatureReference == null) {
rockFluidUnitFeatureReference = new ArrayList();
}
return this.rockFluidUnitFeatureReference;
}
/**
* Gets the value of the standardConditions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the standardConditions property.
*
*
* For example, to add a new item, do as follows:
*
* getStandardConditions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AbstractTemperaturePressure }
*
*
*/
public List getStandardConditions() {
if (standardConditions == null) {
standardConditions = new ArrayList();
}
return this.standardConditions;
}
/**
* Gets the value of the applicationSource property.
*
* @return
* possible object is
* {@link ApplicationInfo }
*
*/
public ApplicationInfo getApplicationSource() {
return applicationSource;
}
/**
* Sets the value of the applicationSource property.
*
* @param value
* allowed object is
* {@link ApplicationInfo }
*
*/
public void setApplicationSource(ApplicationInfo value) {
this.applicationSource = value;
}
/**
* Gets the value of the applicationTarget property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the applicationTarget property.
*
*
* For example, to add a new item, do as follows:
*
* getApplicationTarget().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ApplicationInfo }
*
*
*/
public List getApplicationTarget() {
if (applicationTarget == null) {
applicationTarget = new ArrayList();
}
return this.applicationTarget;
}
/**
* Gets the value of the fluidCharacterizationModel property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the fluidCharacterizationModel property.
*
*
* For example, to add a new item, do as follows:
*
* getFluidCharacterizationModel().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FluidCharacterizationModel }
*
*
*/
public List getFluidCharacterizationModel() {
if (fluidCharacterizationModel == null) {
fluidCharacterizationModel = new ArrayList();
}
return this.fluidCharacterizationModel;
}
/**
* Gets the value of the fluidCharacterizationSource property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the fluidCharacterizationSource property.
*
*
* For example, to add a new item, do as follows:
*
* getFluidCharacterizationSource().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FluidCharacterizationSource }
*
*
*/
public List getFluidCharacterizationSource() {
if (fluidCharacterizationSource == null) {
fluidCharacterizationSource = new ArrayList();
}
return this.fluidCharacterizationSource;
}
/**
* Gets the value of the fluidCharacterizationTableFormatSet property.
*
* @return
* possible object is
* {@link FluidCharacterizationTableFormatSet }
*
*/
public FluidCharacterizationTableFormatSet getFluidCharacterizationTableFormatSet() {
return fluidCharacterizationTableFormatSet;
}
/**
* Sets the value of the fluidCharacterizationTableFormatSet property.
*
* @param value
* allowed object is
* {@link FluidCharacterizationTableFormatSet }
*
*/
public void setFluidCharacterizationTableFormatSet(FluidCharacterizationTableFormatSet value) {
this.fluidCharacterizationTableFormatSet = value;
}
}