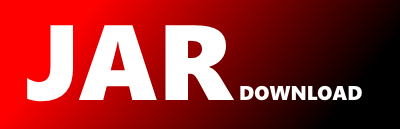
com.geotab.model.entity.addins.AddInConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-sdk Show documentation
Show all versions of java-sdk Show documentation
Legacy Geotab Java SDK to be replaced by the NewGeneration API.
package com.geotab.model.entity.addins;
import com.geotab.model.entity.NameEntityWithVersion;
import lombok.Getter;
import lombok.Setter;
import lombok.experimental.SuperBuilder;
/**
* Represents populated {@link AddIn} with configuration.
*/
@Getter @Setter
@SuperBuilder
public class AddInConfiguration extends NameEntityWithVersion {
/**
* Gets or sets the email address for support related to this Add-In.
*/
private String supportEmail;
/**
* Gets or sets the digital signature of the Add-In.
*/
private String signature;
/**
* Gets or sets an array of custom pages and/or buttons (External references).
*/
private AddInItem[] items;
/**
* Gets or sets custom pages and/or buttons (Embedded code).
*/
private Object files;
/**
* Gets or sets the unique MyGeotab Marketplace Add-In key assigned by Geotab.
* If there’s no plan to get your Add-In to the Marketplace, you can leave out the key/value pair from the configuration.
*/
private String key;
/**
* Gets or sets the Add-In solution Id.
*/
private String solutionId;
/**
* Gets or sets a value indicating whether the Add-In is signed.
*/
private Boolean isSigned;
/**
* Gets or sets the install callback URL.
*/
private String installCallbackUrl;
/**
* Gets or sets the uninstall callback URL.
*/
private String uninstallCallbackUrl;
/**
* Gets or sets a value indicating whether Add-In is executed initially on start up within the Drive App.
*/
private Boolean onStartup;
/**
* Gets or sets a value indicating whether Add-In is executed upon log out within the Drive App.
*/
private Boolean onShutdown;
/**
* Gets or sets an array of {@link CustomSecurityIdDefinition} that are added to the list of features available when editing clearances.
*
* @deprecated Use the {@link #customSecurityIds} parameter
*/
@Deprecated
private CustomSecurityIdDefinition[] securityIds;
/**
* Gets or sets an array of {@link CustomSecurityId} that are added to the list of features available when editing clearances.
*/
private CustomSecurityId[] customSecurityIds;
/**
* Gets or sets a value indicating whether Add-In is going to setup the securityIds for viewing support.
*/
private Boolean enableViewSecurityId;
/**
* Initializes a new instance of the {@link AddInConfiguration} class.
*/
public AddInConfiguration() {
this.setName("");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy