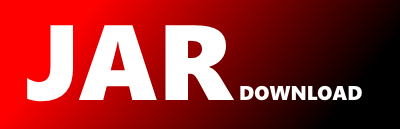
com.geotab.model.entity.user.User Maven / Gradle / Ivy
Show all versions of java-sdk Show documentation
package com.geotab.model.entity.user;
import static com.geotab.model.entity.user.NoDriver.NO_DRIVER_ID;
import static com.geotab.model.entity.user.NoUser.NO_USER_ID;
import static com.geotab.model.entity.user.SystemUser.SYSTEM_USER_ID;
import static com.geotab.model.entity.user.UnknownDriver.UNKNOWN_DRIVER_ID;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.geotab.model.Id;
import com.geotab.model.entity.NameEntityWithVersion;
import com.geotab.model.entity.certificate.Certificate;
import com.geotab.model.entity.fuel.FuelEconomyUnit;
import com.geotab.model.entity.group.Group;
import com.geotab.model.entity.groupfilter.GroupFilter;
import com.geotab.model.entity.worktime.DayOfWeek;
import com.geotab.model.serialization.serdes.EntityCollectionAsIdCollectionSerializer;
import com.geotab.model.serialization.serdes.UserDeserializer;
import com.geotab.model.settings.GoogleMapStyle;
import com.geotab.model.settings.HereMapStyle;
import com.geotab.model.settings.MapView;
import com.geotab.model.settings.OpenStreetMapStyle;
import com.geotab.util.Util;
import java.time.LocalDateTime;
import java.util.List;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
import lombok.experimental.SuperBuilder;
/**
* A user of the system. A user can be a MyGeotab user or a user that is a {@link Driver}.
*/
@Getter @Setter
@NoArgsConstructor
@SuperBuilder(builderMethodName = "userBuilder")
@JsonDeserialize(using = UserDeserializer.class)
@SuppressWarnings("AbbreviationAsWordInName")
public class User extends NameEntityWithVersion {
/**
* A value indicating the user accepted EULA revision number. Default [0].
*/
protected Integer acceptedEULA;
/**
* The version of the last drive what's new guide viewed by the user. Default [0].
*/
protected Integer driveGuideVersion;
/**
* Value indicating accepted WiFi-specific EULA version. Default [0].
*/
protected Integer wifiEULA;
/**
* The active dashboard reports. Default [empty].
*/
protected List activeDashboardReports;
/**
* The list of bookmarked pages. Default [empty].
*/
protected List bookmarks;
/**
* The date the user is active from. Default [UtcNow].
*/
protected LocalDateTime activeFrom;
/**
* The date the user is active to. Default [MaxDate].
*/
protected LocalDateTime activeTo;
/**
* List of all available dashboard reports to the user. Default [empty].
*/
protected List availableDashboardReports;
/**
* The user's stored list of custom response options to choose from when sending a TextMessage. Each item is a set of
* predefined response options. Default [empty].
*/
protected String[][] cannedResponseOptions;
/**
* Flag indicating whether the user's password requires resetting. If [true], the user will be forced to change their
* password on next login. Default [false].
*/
@JsonProperty("changePassword")
protected Boolean changePassword;
/**
* Free text field where any user information can be stored and referenced for this entity. Default [""].
*/
protected String comment;
/**
* List of organization {@link Group}(s) that the user belongs to.
*/
@JsonSerialize(converter = EntityCollectionAsIdCollectionSerializer.class)
protected List companyGroups;
/**
* The group filter {@link GroupFilter} that the user belongs to, which is used to determine what the user can see within the database.
*/
@JsonProperty("accessGroupFilter")
protected GroupFilter accessGroupFilter;
/**
* A value to nullify accessGroupFilter.
*/
@JsonProperty("nullifyAccessGroupFilter")
protected Boolean nullifyAccessGroupFilter;
/**
* The format dates will be displayed to this user. Default ["MM/dd/yy HH:mm:ss"].
*/
protected String dateFormat;
/**
* The user phone number with space separated country phone code. Example +1 5555555555. Maximum length [20] Default
* [""]
*/
protected String phoneNumber;
/**
* Gets or sets the user's preferred currency for display in the UI.
*/
protected Currency displayCurrency;
/**
* The user two symbols country ISO code (https://www.iso.org/iso-3166-country-codes.html). Maximum length [2] Default
* [""]
*/
protected String countryCode;
/**
* The user phone number without formatting. Maximum length [5] Default [""]
*/
protected String phoneNumberExtension;
/**
* The default map style for Google maps. Default [Roadmap].
*/
protected GoogleMapStyle defaultGoogleMapStyle;
/**
* The default map engine to use for this user. System map engines are:
*
* -
* GoogleMaps
*
* -
* HereMap
*
* -
* MapBox
*
*
*
* Default ["MapBox"].
*/
protected String defaultMapEngine;
/**
* The default map style for Open Street Maps. Default [MapBox].
*/
protected OpenStreetMapStyle defaultOpenStreetMapStyle;
/**
* The default map style for Here Maps. Default [Roadmap].
*/
protected HereMapStyle defaultHereMapStyle;
/**
* Indicates the default page to view when login complete. Maps to the hash portion of the web site URL
* (https://url/enpoint/[#page]). Default [helpGuide].
*/
protected String defaultPage;
/**
* The designation or title of the employee. Maximum length [50] Default [""].
*/
protected String designation;
/**
* The employee number or external identifier. Maximum length [50] Default [""].
*/
protected String employeeNo;
/**
* The first name of the user. Maximum length [255].
*/
protected String firstName;
/**
* The user's preferred {@link FuelEconomyUnit} for viewing fuel economy. Default [{@link
* FuelEconomyUnit#LITERS_PER_100_KM}].
*/
protected FuelEconomyUnit fuelEconomyUnit;
/**
* The user's preferred {@link ElectricEnergyEconomyUnit} for viewing fuel economy. Default [{@link
* ElectricEnergyEconomyUnit#LITERS_E_PER_100_KM}].
*/
protected ElectricEnergyEconomyUnit electricEnergyEconomyUnit;
/**
* The {@link HosRuleSet} the user follows. Default [{@link HosRuleSet#NONE}].
*/
protected HosRuleSet hosRuleSet;
/**
* Value indicating whether the user is allowed to HOS yard move. Default [false].
*/
@JsonProperty("isYardMoveEnabled")
protected Boolean isYardMoveEnabled;
/**
* Value indicating whether the user is allowed to HOS personal conveyance. Default [false].
*/
@JsonProperty("isPersonalConveyanceEnabled")
protected Boolean isPersonalConveyanceEnabled;
/**
* Value indicating whether the user is allowed to HOS personal conveyance. Default [false].
*/
@JsonProperty("isExemptHOSEnabled")
protected Boolean isExemptHOSEnabled;
/**
* Value indicating the maximum personal conveyance distance per day in meters. Default [0].
*/
protected Double maxPCDistancePerDay;
/**
* The HOS authority name of the user. Default [""].
*/
protected String authorityName;
/**
* The HOS authority address of the user. Default [""].
*/
protected String authorityAddress;
/**
* Value indicating whether the old EULA has been accepted by the end user. Default [false].
*/
@JsonProperty("isEULAAccepted")
protected Boolean isEULAAccepted;
/**
* Value that indicates whether news notifications are enabled for this user. Default [true].
*/
@JsonProperty("isNewsEnabled")
protected Boolean isNewsEnabled = true;
/**
* Value indicating whether are service update notifications enabled for this user. Default [false].
*/
@JsonProperty("isServiceUpdatesEnabled")
protected Boolean isServiceUpdatesEnabled;
/**
* Value indicating whether labs are enabled for this user. When set to true this will enable experimental features
* that are still in the process of being developed. Default [false].
*/
@JsonProperty("isLabsEnabled")
protected Boolean isLabsEnabled;
/**
* Whether the current regional settings is in metric units of measurement (or US/Imperial). Default [true].
*/
@JsonProperty("isMetric")
protected Boolean isMetric = true;
/**
* The user's language. Default: ["en"] for English.
*/
protected String language;
/**
* The user's preferred day to represent the start of the week. Default ["Sunday"].
*/
protected DayOfWeek firstDayOfWeek;
/**
* The last name of the user. Maximum length [255].
*/
protected String lastName;
/**
* The list of the of the available {@link MapView}s from the live map. Default [continent of the user's selected time
* zone].
*/
protected List mapViews;
/**
* The user's password.
*/
protected String password;
/**
* The private {@link Group}(s) that the user belongs to.
*/
@JsonSerialize(converter = EntityCollectionAsIdCollectionSerializer.class)
protected List privateUserGroups;
/**
* The report {@link Group}(s) for reporting that this user belongs to. The selected reporting groups will allow the
* user to sort entities that are children of the selected groups. It will not allow them to see entities that are
* outside of their data access. Default [empty].
*/
@JsonSerialize(converter = EntityCollectionAsIdCollectionSerializer.class)
protected List reportGroups;
/**
* The security {@link Group}(s) this user belongs to; which define the user's access.
*/
@JsonSerialize(converter = EntityCollectionAsIdCollectionSerializer.class)
protected List securityGroups;
/**
* A flag indicating whether to show ClickOnce support warning as the default page. (legacy) Default [false].
*/
@JsonProperty("showClickOnceWarning")
protected Boolean showClickOnceWarning;
/**
* The IANA time zone Id of the user. All data will be displayed in this time zone. Default ["America/New_York"].
*/
protected String timeZoneId;
/**
* The user authentication type. Default [Basic].
*/
protected UserAuthenticationType userAuthenticationType;
/**
* The default zone display mode used on the map. Default [Default].
*/
protected ZoneDisplayMode zoneDisplayMode;
/**
* The issuer certificate for the user. Will only be populated for users not using basic authentication.
*/
protected Certificate issuerCertificate;
/**
* The name of the company for the user. Default [""].
*/
protected String companyName;
/**
* The company address for the user. Default [""].
*/
protected String companyAddress;
/**
* The carrier number. Default [""].
*/
protected String carrierNumber;
/**
* The user's Last access date of the system.
*/
protected LocalDateTime lastAccessDate;
/**
* The is driver toggle, if [true] the user is a driver, otherwise [false]. Default [false].
*/
@JsonProperty("isDriver")
protected Boolean isDriver;
/**
* If [true] the user will receive the emailed report, otherwise [false]. Default [true].
*/
@JsonProperty("isEmailReportEnabled")
protected Boolean isEmailReportEnabled = true;
public User(String id) {
setId(new Id(id));
setName(id);
}
public static User fromSystem(String id) {
if (Util.isEmpty(id)) return null;
if (NO_DRIVER_ID.equals(id)) return NoDriver.getInstance();
if (UNKNOWN_DRIVER_ID.equals(id)) return UnknownDriver.getInstance();
if (NO_USER_ID.equals(id)) return NoUser.getInstance();
if (SYSTEM_USER_ID.equals(id)) return SystemUser.getInstance();
return null;
}
}