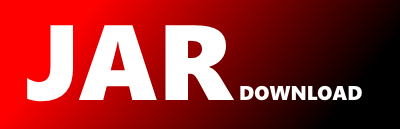
com.getperka.cli.logging.model.Thrown Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cli Show documentation
Show all versions of cli Show documentation
Utility code used by various Perka projects
package com.getperka.cli.logging.model;
/**
* Represents a Throwable. The name is a bit awkward, but was chosen so as to
* not conflict with built-in names.
*/
@javax.annotation.Generated(value="org.jsonddl.generator.industrial.IndustrialDialect", date="2012-07-11T15:04:56")
public interface Thrown
extends org.jsonddl.JsonDdlObject
{
/**
* Constructs instances of {@link Thrown}. Instances of Builder should not be
* reused after calling {@link #build()}.
*/
@javax.annotation.Generated(value="org.jsonddl.generator.industrial.IndustrialDialect", date="2012-07-11T15:04:56")
public static class Builder
implements org.jsonddl.JsonDdlObject.Builder, org.jsonddl.impl.Traversable, org.jsonddl.impl.Digested, Thrown {
private Thrown built;
private ThrownImpl obj;
public Builder() {
this(new ThrownImpl());
}
Builder(ThrownImpl instance) {
this.obj = instance;
}
public Thrown.Builder accept(org.jsonddl.JsonDdlVisitor visitor) {
obj = new org.jsonddl.impl.ContextImpl.ObjectContext.Builder()
.withValue(this)
.withKind(org.jsonddl.model.Kind.DDL)
.withMutability(true)
.build().traverse(visitor).builder().obj;
return this;
}
public Thrown build() {
// Prevent build() loops from causing NPE's
if (built != null) {
return built;
}
ThrownImpl toReturn = obj;
built = toReturn;
obj = null;
toReturn.Cause = org.jsonddl.impl.Protected.object(toReturn.Cause);
toReturn.StackTrace = org.jsonddl.impl.Protected.object(toReturn.StackTrace);
return toReturn;
}
public Thrown.Builder builder() {
return this;
}
public Thrown.Builder from(Thrown copyFrom) {
withCause(copyFrom.getCause());
withMessage(copyFrom.getMessage());
withStackTrace(copyFrom.getStackTrace());
withType(copyFrom.getType());
return this;
}
public Thrown.Builder from(java.util.Map map) {
accept(org.jsonddl.impl.JsonMapVisitor.fromJsonMap(map));
return this;
}
public Class getDdlObjectType() {
return Thrown.class;
}
public Thrown.Builder newInstance() {
return new Thrown.Builder();
}
public java.util.Map toJsonObject() {
return obj.toJsonObject();
}
public Thrown.Builder traverse(org.jsonddl.JsonDdlVisitor visitor) {
withCause(
new org.jsonddl.impl.ContextImpl.ObjectContext.Builder()
.withKind(org.jsonddl.model.Kind.DDL)
.withLeafType(Thrown.class)
.withMutability(true)
.withProperty("cause")
.withValue(obj.Cause)
.build().traverse(visitor));
withMessage(
new org.jsonddl.impl.ContextImpl.ValueContext.Builder()
.withKind(org.jsonddl.model.Kind.STRING)
.withLeafType(java.lang.String.class)
.withMutability(true)
.withProperty("message")
.withValue(obj.Message)
.build().traverse(visitor));
withStackTrace(
new org.jsonddl.impl.ContextImpl.ListContext.Builder()
.withKind(org.jsonddl.model.Kind.LIST)
.withNestedKinds(java.util.Arrays.asList(org.jsonddl.model.Kind.DDL))
.withLeafType(StackFrame.class)
.withMutability(true)
.withProperty("stackTrace")
.withValue(obj.StackTrace)
.build().traverse(visitor));
withType(
new org.jsonddl.impl.ContextImpl.ValueContext.Builder()
.withKind(org.jsonddl.model.Kind.STRING)
.withLeafType(java.lang.String.class)
.withMutability(true)
.withProperty("type")
.withValue(obj.Type)
.build().traverse(visitor));
return this;
}
public byte[] computeDigest() {
byte[] digest;
org.jsonddl.impl.DigestVisitor v = new org.jsonddl.impl.DigestVisitor();
accept(v);
digest = v.getDigest();
return digest;
}
public int hashCode() {
byte[] digest = computeDigest();
return (int)((digest[0] << 3) | (digest[1] << 2) | (digest[18] << 1) | digest[19]);
}
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (!(o instanceof org.jsonddl.impl.Digested)) {
return false;
}
byte[] d1 = computeDigest();
byte[] d2 = ((org.jsonddl.impl.Digested) o).computeDigest();
for (int i = 0, j = d1.length; i();
}
try {
obj.StackTrace.add(element);
} catch (java.lang.UnsupportedOperationException e) {
obj.StackTrace = new java.util.ArrayList(obj.StackTrace);
obj.StackTrace.add(element);
}
return this;
}
public Thrown.Builder getCause() {
Thrown.Builder toReturn = obj.Cause.builder();
obj.Cause = toReturn;
return toReturn;
}
public java.lang.String getMessage() {
return obj.Message;
}
public java.util.List getStackTrace() {
return obj.StackTrace;
}
public java.lang.String getType() {
return obj.Type;
}
public void setCause(Thrown value) {
withCause(value);
}
public void setMessage(java.lang.String value) {
withMessage(value);
}
public void setStackTrace(java.util.List value) {
withStackTrace(value);
}
/** The exception's original type. */
public void setType(java.lang.String value) {
withType(value);
}
public Thrown.Builder withCause(Thrown value) {
obj.Cause = value;
return this;
}
public Thrown.Builder withMessage(java.lang.String value) {
obj.Message = value;
return this;
}
public Thrown.Builder withStackTrace(java.util.List value) {
obj.StackTrace = value;
return this;
}
/** The exception's original type. */
public Thrown.Builder withType(java.lang.String value) {
obj.Type = value;
return this;
}
}
Builder builder();
Thrown getCause();
java.lang.String getMessage();
java.util.List getStackTrace();
/** The exception's original type. */
java.lang.String getType();
Builder newInstance();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy