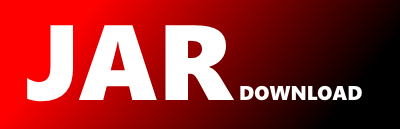
com.gettyio.gim.packet.MessageClass Maven / Gradle / Ivy
package com.gettyio.gim.packet;// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: Message.proto
public final class MessageClass {
private MessageClass() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface MessageOrBuilder extends
// @@protoc_insertion_point(interface_extends:Message)
com.google.protobuf.MessageOrBuilder {
/**
*
*消息唯一ID
*
*
* string id = 1;
* @return The id.
*/
String getId();
/**
*
*消息唯一ID
*
*
* string id = 1;
* @return The bytes for id.
*/
com.google.protobuf.ByteString
getIdBytes();
/**
*
*服务器Id
*
*
* string serverId = 2;
* @return The serverId.
*/
String getServerId();
/**
*
*服务器Id
*
*
* string serverId = 2;
* @return The bytes for serverId.
*/
com.google.protobuf.ByteString
getServerIdBytes();
/**
*
* 请求类型
*
*
* int32 reqType = 3;
* @return The reqType.
*/
int getReqType();
/**
*
*消息时间
*
*
* int64 msgTime = 4;
* @return The msgTime.
*/
long getMsgTime();
/**
*
*同步标志,标记消息在传输哪个阶段
*
*
* int32 syn = 5;
* @return The syn.
*/
int getSyn();
/**
*
*ack
*
*
* string ack = 6;
* @return The ack.
*/
String getAck();
/**
*
*ack
*
*
* string ack = 6;
* @return The bytes for ack.
*/
com.google.protobuf.ByteString
getAckBytes();
/**
*
* 发送用户ID
*
*
* string fromId = 7;
* @return The fromId.
*/
String getFromId();
/**
*
* 发送用户ID
*
*
* string fromId = 7;
* @return The bytes for fromId.
*/
com.google.protobuf.ByteString
getFromIdBytes();
/**
*
*接收用户ID
*
*
* string toId = 8;
* @return The toId.
*/
String getToId();
/**
*
*接收用户ID
*
*
* string toId = 8;
* @return The bytes for toId.
*/
com.google.protobuf.ByteString
getToIdBytes();
/**
*
*群id
*
*
* string groupId = 9;
* @return The groupId.
*/
String getGroupId();
/**
*
*群id
*
*
* string groupId = 9;
* @return The bytes for groupId.
*/
com.google.protobuf.ByteString
getGroupIdBytes();
/**
*
*消息体
*
*
* string body = 10;
* @return The body.
*/
String getBody();
/**
*
*消息体
*
*
* string body = 10;
* @return The bytes for body.
*/
com.google.protobuf.ByteString
getBodyBytes();
/**
*
*状态
*
*
* int32 status = 11;
* @return The status.
*/
int getStatus();
}
/**
* Protobuf type {@code Message}
*/
public static final class Message extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:Message)
MessageOrBuilder {
private static final long serialVersionUID = 0L;
// Use Message.newBuilder() to construct.
private Message(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Message() {
id_ = "";
serverId_ = "";
ack_ = "";
fromId_ = "";
toId_ = "";
groupId_ = "";
body_ = "";
}
@Override
@SuppressWarnings({"unused"})
protected Object newInstance(
UnusedPrivateParameter unused) {
return new Message();
}
@Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Message(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
String s = input.readStringRequireUtf8();
id_ = s;
break;
}
case 18: {
String s = input.readStringRequireUtf8();
serverId_ = s;
break;
}
case 24: {
reqType_ = input.readInt32();
break;
}
case 32: {
msgTime_ = input.readInt64();
break;
}
case 40: {
syn_ = input.readInt32();
break;
}
case 50: {
String s = input.readStringRequireUtf8();
ack_ = s;
break;
}
case 58: {
String s = input.readStringRequireUtf8();
fromId_ = s;
break;
}
case 66: {
String s = input.readStringRequireUtf8();
toId_ = s;
break;
}
case 74: {
String s = input.readStringRequireUtf8();
groupId_ = s;
break;
}
case 82: {
String s = input.readStringRequireUtf8();
body_ = s;
break;
}
case 88: {
status_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return MessageClass.internal_static_Message_descriptor;
}
@Override
protected FieldAccessorTable
internalGetFieldAccessorTable() {
return MessageClass.internal_static_Message_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Message.class, Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
private volatile Object id_;
/**
*
*消息唯一ID
*
*
* string id = 1;
* @return The id.
*/
@Override
public String getId() {
Object ref = id_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
*
*消息唯一ID
*
*
* string id = 1;
* @return The bytes for id.
*/
@Override
public com.google.protobuf.ByteString
getIdBytes() {
Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SERVERID_FIELD_NUMBER = 2;
private volatile Object serverId_;
/**
*
*服务器Id
*
*
* string serverId = 2;
* @return The serverId.
*/
@Override
public String getServerId() {
Object ref = serverId_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
serverId_ = s;
return s;
}
}
/**
*
*服务器Id
*
*
* string serverId = 2;
* @return The bytes for serverId.
*/
@Override
public com.google.protobuf.ByteString
getServerIdBytes() {
Object ref = serverId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
serverId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REQTYPE_FIELD_NUMBER = 3;
private int reqType_;
/**
*
* 请求类型
*
*
* int32 reqType = 3;
* @return The reqType.
*/
@Override
public int getReqType() {
return reqType_;
}
public static final int MSGTIME_FIELD_NUMBER = 4;
private long msgTime_;
/**
*
*消息时间
*
*
* int64 msgTime = 4;
* @return The msgTime.
*/
@Override
public long getMsgTime() {
return msgTime_;
}
public static final int SYN_FIELD_NUMBER = 5;
private int syn_;
/**
*
*同步标志,标记消息在传输哪个阶段
*
*
* int32 syn = 5;
* @return The syn.
*/
@Override
public int getSyn() {
return syn_;
}
public static final int ACK_FIELD_NUMBER = 6;
private volatile Object ack_;
/**
*
*ack
*
*
* string ack = 6;
* @return The ack.
*/
@Override
public String getAck() {
Object ref = ack_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
ack_ = s;
return s;
}
}
/**
*
*ack
*
*
* string ack = 6;
* @return The bytes for ack.
*/
@Override
public com.google.protobuf.ByteString
getAckBytes() {
Object ref = ack_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
ack_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FROMID_FIELD_NUMBER = 7;
private volatile Object fromId_;
/**
*
* 发送用户ID
*
*
* string fromId = 7;
* @return The fromId.
*/
@Override
public String getFromId() {
Object ref = fromId_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
fromId_ = s;
return s;
}
}
/**
*
* 发送用户ID
*
*
* string fromId = 7;
* @return The bytes for fromId.
*/
@Override
public com.google.protobuf.ByteString
getFromIdBytes() {
Object ref = fromId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
fromId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TOID_FIELD_NUMBER = 8;
private volatile Object toId_;
/**
*
*接收用户ID
*
*
* string toId = 8;
* @return The toId.
*/
@Override
public String getToId() {
Object ref = toId_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
toId_ = s;
return s;
}
}
/**
*
*接收用户ID
*
*
* string toId = 8;
* @return The bytes for toId.
*/
@Override
public com.google.protobuf.ByteString
getToIdBytes() {
Object ref = toId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
toId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int GROUPID_FIELD_NUMBER = 9;
private volatile Object groupId_;
/**
*
*群id
*
*
* string groupId = 9;
* @return The groupId.
*/
@Override
public String getGroupId() {
Object ref = groupId_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
groupId_ = s;
return s;
}
}
/**
*
*群id
*
*
* string groupId = 9;
* @return The bytes for groupId.
*/
@Override
public com.google.protobuf.ByteString
getGroupIdBytes() {
Object ref = groupId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
groupId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BODY_FIELD_NUMBER = 10;
private volatile Object body_;
/**
*
*消息体
*
*
* string body = 10;
* @return The body.
*/
@Override
public String getBody() {
Object ref = body_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
body_ = s;
return s;
}
}
/**
*
*消息体
*
*
* string body = 10;
* @return The bytes for body.
*/
@Override
public com.google.protobuf.ByteString
getBodyBytes() {
Object ref = body_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
body_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUS_FIELD_NUMBER = 11;
private int status_;
/**
*
*状态
*
*
* int32 status = 11;
* @return The status.
*/
@Override
public int getStatus() {
return status_;
}
private byte memoizedIsInitialized = -1;
@Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, id_);
}
if (!getServerIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, serverId_);
}
if (reqType_ != 0) {
output.writeInt32(3, reqType_);
}
if (msgTime_ != 0L) {
output.writeInt64(4, msgTime_);
}
if (syn_ != 0) {
output.writeInt32(5, syn_);
}
if (!getAckBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, ack_);
}
if (!getFromIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, fromId_);
}
if (!getToIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, toId_);
}
if (!getGroupIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, groupId_);
}
if (!getBodyBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, body_);
}
if (status_ != 0) {
output.writeInt32(11, status_);
}
unknownFields.writeTo(output);
}
@Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, id_);
}
if (!getServerIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, serverId_);
}
if (reqType_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, reqType_);
}
if (msgTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, msgTime_);
}
if (syn_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, syn_);
}
if (!getAckBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, ack_);
}
if (!getFromIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, fromId_);
}
if (!getToIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, toId_);
}
if (!getGroupIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, groupId_);
}
if (!getBodyBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, body_);
}
if (status_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(11, status_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@Override
public boolean equals(final Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof Message)) {
return super.equals(obj);
}
Message other = (Message) obj;
if (!getId()
.equals(other.getId())) return false;
if (!getServerId()
.equals(other.getServerId())) return false;
if (getReqType()
!= other.getReqType()) return false;
if (getMsgTime()
!= other.getMsgTime()) return false;
if (getSyn()
!= other.getSyn()) return false;
if (!getAck()
.equals(other.getAck())) return false;
if (!getFromId()
.equals(other.getFromId())) return false;
if (!getToId()
.equals(other.getToId())) return false;
if (!getGroupId()
.equals(other.getGroupId())) return false;
if (!getBody()
.equals(other.getBody())) return false;
if (getStatus()
!= other.getStatus()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
hash = (37 * hash) + SERVERID_FIELD_NUMBER;
hash = (53 * hash) + getServerId().hashCode();
hash = (37 * hash) + REQTYPE_FIELD_NUMBER;
hash = (53 * hash) + getReqType();
hash = (37 * hash) + MSGTIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMsgTime());
hash = (37 * hash) + SYN_FIELD_NUMBER;
hash = (53 * hash) + getSyn();
hash = (37 * hash) + ACK_FIELD_NUMBER;
hash = (53 * hash) + getAck().hashCode();
hash = (37 * hash) + FROMID_FIELD_NUMBER;
hash = (53 * hash) + getFromId().hashCode();
hash = (37 * hash) + TOID_FIELD_NUMBER;
hash = (53 * hash) + getToId().hashCode();
hash = (37 * hash) + GROUPID_FIELD_NUMBER;
hash = (53 * hash) + getGroupId().hashCode();
hash = (37 * hash) + BODY_FIELD_NUMBER;
hash = (53 * hash) + getBody().hashCode();
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static Message parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Message parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Message parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Message parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Message parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Message parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Message parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static Message parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static Message parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static Message parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static Message parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static Message parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(Message prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@Override
protected Builder newBuilderForType(
BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Message}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:Message)
MessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return MessageClass.internal_static_Message_descriptor;
}
@Override
protected FieldAccessorTable
internalGetFieldAccessorTable() {
return MessageClass.internal_static_Message_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Message.class, Builder.class);
}
// Construct using MessageClass.Message.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@Override
public Builder clear() {
super.clear();
id_ = "";
serverId_ = "";
reqType_ = 0;
msgTime_ = 0L;
syn_ = 0;
ack_ = "";
fromId_ = "";
toId_ = "";
groupId_ = "";
body_ = "";
status_ = 0;
return this;
}
@Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return MessageClass.internal_static_Message_descriptor;
}
@Override
public Message getDefaultInstanceForType() {
return Message.getDefaultInstance();
}
@Override
public Message build() {
Message result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@Override
public Message buildPartial() {
Message result = new Message(this);
result.id_ = id_;
result.serverId_ = serverId_;
result.reqType_ = reqType_;
result.msgTime_ = msgTime_;
result.syn_ = syn_;
result.ack_ = ack_;
result.fromId_ = fromId_;
result.toId_ = toId_;
result.groupId_ = groupId_;
result.body_ = body_;
result.status_ = status_;
onBuilt();
return result;
}
@Override
public Builder clone() {
return super.clone();
}
@Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return super.setField(field, value);
}
@Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return super.setRepeatedField(field, index, value);
}
@Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return super.addRepeatedField(field, value);
}
@Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof Message) {
return mergeFrom((Message)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(Message other) {
if (other == Message.getDefaultInstance()) return this;
if (!other.getId().isEmpty()) {
id_ = other.id_;
onChanged();
}
if (!other.getServerId().isEmpty()) {
serverId_ = other.serverId_;
onChanged();
}
if (other.getReqType() != 0) {
setReqType(other.getReqType());
}
if (other.getMsgTime() != 0L) {
setMsgTime(other.getMsgTime());
}
if (other.getSyn() != 0) {
setSyn(other.getSyn());
}
if (!other.getAck().isEmpty()) {
ack_ = other.ack_;
onChanged();
}
if (!other.getFromId().isEmpty()) {
fromId_ = other.fromId_;
onChanged();
}
if (!other.getToId().isEmpty()) {
toId_ = other.toId_;
onChanged();
}
if (!other.getGroupId().isEmpty()) {
groupId_ = other.groupId_;
onChanged();
}
if (!other.getBody().isEmpty()) {
body_ = other.body_;
onChanged();
}
if (other.getStatus() != 0) {
setStatus(other.getStatus());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@Override
public final boolean isInitialized() {
return true;
}
@Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Message parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (Message) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private Object id_ = "";
/**
*
*消息唯一ID
*
*
* string id = 1;
* @return The id.
*/
public String getId() {
Object ref = id_;
if (!(ref instanceof String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (String) ref;
}
}
/**
*
*消息唯一ID
*
*
* string id = 1;
* @return The bytes for id.
*/
public com.google.protobuf.ByteString
getIdBytes() {
Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*消息唯一ID
*
*
* string id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(
String value) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
return this;
}
/**
*
*消息唯一ID
*
*
* string id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
*
*消息唯一ID
*
*
* string id = 1;
* @param value The bytes for id to set.
* @return This builder for chaining.
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
id_ = value;
onChanged();
return this;
}
private Object serverId_ = "";
/**
*
*服务器Id
*
*
* string serverId = 2;
* @return The serverId.
*/
public String getServerId() {
Object ref = serverId_;
if (!(ref instanceof String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
serverId_ = s;
return s;
} else {
return (String) ref;
}
}
/**
*
*服务器Id
*
*
* string serverId = 2;
* @return The bytes for serverId.
*/
public com.google.protobuf.ByteString
getServerIdBytes() {
Object ref = serverId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
serverId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*服务器Id
*
*
* string serverId = 2;
* @param value The serverId to set.
* @return This builder for chaining.
*/
public Builder setServerId(
String value) {
if (value == null) {
throw new NullPointerException();
}
serverId_ = value;
onChanged();
return this;
}
/**
*
*服务器Id
*
*
* string serverId = 2;
* @return This builder for chaining.
*/
public Builder clearServerId() {
serverId_ = getDefaultInstance().getServerId();
onChanged();
return this;
}
/**
*
*服务器Id
*
*
* string serverId = 2;
* @param value The bytes for serverId to set.
* @return This builder for chaining.
*/
public Builder setServerIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
serverId_ = value;
onChanged();
return this;
}
private int reqType_ ;
/**
*
* 请求类型
*
*
* int32 reqType = 3;
* @return The reqType.
*/
@Override
public int getReqType() {
return reqType_;
}
/**
*
* 请求类型
*
*
* int32 reqType = 3;
* @param value The reqType to set.
* @return This builder for chaining.
*/
public Builder setReqType(int value) {
reqType_ = value;
onChanged();
return this;
}
/**
*
* 请求类型
*
*
* int32 reqType = 3;
* @return This builder for chaining.
*/
public Builder clearReqType() {
reqType_ = 0;
onChanged();
return this;
}
private long msgTime_ ;
/**
*
*消息时间
*
*
* int64 msgTime = 4;
* @return The msgTime.
*/
@Override
public long getMsgTime() {
return msgTime_;
}
/**
*
*消息时间
*
*
* int64 msgTime = 4;
* @param value The msgTime to set.
* @return This builder for chaining.
*/
public Builder setMsgTime(long value) {
msgTime_ = value;
onChanged();
return this;
}
/**
*
*消息时间
*
*
* int64 msgTime = 4;
* @return This builder for chaining.
*/
public Builder clearMsgTime() {
msgTime_ = 0L;
onChanged();
return this;
}
private int syn_ ;
/**
*
*同步标志,标记消息在传输哪个阶段
*
*
* int32 syn = 5;
* @return The syn.
*/
@Override
public int getSyn() {
return syn_;
}
/**
*
*同步标志,标记消息在传输哪个阶段
*
*
* int32 syn = 5;
* @param value The syn to set.
* @return This builder for chaining.
*/
public Builder setSyn(int value) {
syn_ = value;
onChanged();
return this;
}
/**
*
*同步标志,标记消息在传输哪个阶段
*
*
* int32 syn = 5;
* @return This builder for chaining.
*/
public Builder clearSyn() {
syn_ = 0;
onChanged();
return this;
}
private Object ack_ = "";
/**
*
*ack
*
*
* string ack = 6;
* @return The ack.
*/
public String getAck() {
Object ref = ack_;
if (!(ref instanceof String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
ack_ = s;
return s;
} else {
return (String) ref;
}
}
/**
*
*ack
*
*
* string ack = 6;
* @return The bytes for ack.
*/
public com.google.protobuf.ByteString
getAckBytes() {
Object ref = ack_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
ack_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*ack
*
*
* string ack = 6;
* @param value The ack to set.
* @return This builder for chaining.
*/
public Builder setAck(
String value) {
if (value == null) {
throw new NullPointerException();
}
ack_ = value;
onChanged();
return this;
}
/**
*
*ack
*
*
* string ack = 6;
* @return This builder for chaining.
*/
public Builder clearAck() {
ack_ = getDefaultInstance().getAck();
onChanged();
return this;
}
/**
*
*ack
*
*
* string ack = 6;
* @param value The bytes for ack to set.
* @return This builder for chaining.
*/
public Builder setAckBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ack_ = value;
onChanged();
return this;
}
private Object fromId_ = "";
/**
*
* 发送用户ID
*
*
* string fromId = 7;
* @return The fromId.
*/
public String getFromId() {
Object ref = fromId_;
if (!(ref instanceof String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
fromId_ = s;
return s;
} else {
return (String) ref;
}
}
/**
*
* 发送用户ID
*
*
* string fromId = 7;
* @return The bytes for fromId.
*/
public com.google.protobuf.ByteString
getFromIdBytes() {
Object ref = fromId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
fromId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* 发送用户ID
*
*
* string fromId = 7;
* @param value The fromId to set.
* @return This builder for chaining.
*/
public Builder setFromId(
String value) {
if (value == null) {
throw new NullPointerException();
}
fromId_ = value;
onChanged();
return this;
}
/**
*
* 发送用户ID
*
*
* string fromId = 7;
* @return This builder for chaining.
*/
public Builder clearFromId() {
fromId_ = getDefaultInstance().getFromId();
onChanged();
return this;
}
/**
*
* 发送用户ID
*
*
* string fromId = 7;
* @param value The bytes for fromId to set.
* @return This builder for chaining.
*/
public Builder setFromIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
fromId_ = value;
onChanged();
return this;
}
private Object toId_ = "";
/**
*
*接收用户ID
*
*
* string toId = 8;
* @return The toId.
*/
public String getToId() {
Object ref = toId_;
if (!(ref instanceof String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
toId_ = s;
return s;
} else {
return (String) ref;
}
}
/**
*
*接收用户ID
*
*
* string toId = 8;
* @return The bytes for toId.
*/
public com.google.protobuf.ByteString
getToIdBytes() {
Object ref = toId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
toId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*接收用户ID
*
*
* string toId = 8;
* @param value The toId to set.
* @return This builder for chaining.
*/
public Builder setToId(
String value) {
if (value == null) {
throw new NullPointerException();
}
toId_ = value;
onChanged();
return this;
}
/**
*
*接收用户ID
*
*
* string toId = 8;
* @return This builder for chaining.
*/
public Builder clearToId() {
toId_ = getDefaultInstance().getToId();
onChanged();
return this;
}
/**
*
*接收用户ID
*
*
* string toId = 8;
* @param value The bytes for toId to set.
* @return This builder for chaining.
*/
public Builder setToIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
toId_ = value;
onChanged();
return this;
}
private Object groupId_ = "";
/**
*
*群id
*
*
* string groupId = 9;
* @return The groupId.
*/
public String getGroupId() {
Object ref = groupId_;
if (!(ref instanceof String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
groupId_ = s;
return s;
} else {
return (String) ref;
}
}
/**
*
*群id
*
*
* string groupId = 9;
* @return The bytes for groupId.
*/
public com.google.protobuf.ByteString
getGroupIdBytes() {
Object ref = groupId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
groupId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*群id
*
*
* string groupId = 9;
* @param value The groupId to set.
* @return This builder for chaining.
*/
public Builder setGroupId(
String value) {
if (value == null) {
throw new NullPointerException();
}
groupId_ = value;
onChanged();
return this;
}
/**
*
*群id
*
*
* string groupId = 9;
* @return This builder for chaining.
*/
public Builder clearGroupId() {
groupId_ = getDefaultInstance().getGroupId();
onChanged();
return this;
}
/**
*
*群id
*
*
* string groupId = 9;
* @param value The bytes for groupId to set.
* @return This builder for chaining.
*/
public Builder setGroupIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
groupId_ = value;
onChanged();
return this;
}
private Object body_ = "";
/**
*
*消息体
*
*
* string body = 10;
* @return The body.
*/
public String getBody() {
Object ref = body_;
if (!(ref instanceof String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
body_ = s;
return s;
} else {
return (String) ref;
}
}
/**
*
*消息体
*
*
* string body = 10;
* @return The bytes for body.
*/
public com.google.protobuf.ByteString
getBodyBytes() {
Object ref = body_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
body_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*消息体
*
*
* string body = 10;
* @param value The body to set.
* @return This builder for chaining.
*/
public Builder setBody(
String value) {
if (value == null) {
throw new NullPointerException();
}
body_ = value;
onChanged();
return this;
}
/**
*
*消息体
*
*
* string body = 10;
* @return This builder for chaining.
*/
public Builder clearBody() {
body_ = getDefaultInstance().getBody();
onChanged();
return this;
}
/**
*
*消息体
*
*
* string body = 10;
* @param value The bytes for body to set.
* @return This builder for chaining.
*/
public Builder setBodyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
body_ = value;
onChanged();
return this;
}
private int status_ ;
/**
*
*状态
*
*
* int32 status = 11;
* @return The status.
*/
@Override
public int getStatus() {
return status_;
}
/**
*
*状态
*
*
* int32 status = 11;
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
*状态
*
*
* int32 status = 11;
* @return This builder for chaining.
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
@Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:Message)
}
// @@protoc_insertion_point(class_scope:Message)
private static final Message DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new Message();
}
public static Message getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@Override
public Message parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Message(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@Override
public Message getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_Message_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_Message_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
String[] descriptorData = {
"\n\rMessage.proto\"\260\001\n\007Message\022\n\n\002id\030\001 \001(\t\022" +
"\020\n\010serverId\030\002 \001(\t\022\017\n\007reqType\030\003 \001(\005\022\017\n\007ms" +
"gTime\030\004 \001(\003\022\013\n\003syn\030\005 \001(\005\022\013\n\003ack\030\006 \001(\t\022\016\n" +
"\006fromId\030\007 \001(\t\022\014\n\004toId\030\010 \001(\t\022\017\n\007groupId\030\t" +
" \001(\t\022\014\n\004body\030\n \001(\t\022\016\n\006status\030\013 \001(\005B\016B\014Me" +
"ssageClassb\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
});
internal_static_Message_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_Message_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_Message_descriptor,
new String[] { "Id", "ServerId", "ReqType", "MsgTime", "Syn", "Ack", "FromId", "ToId", "GroupId", "Body", "Status", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy