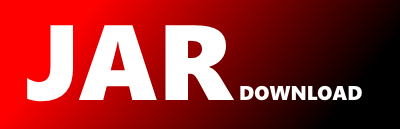
com.gettyio.gim.utils.FastJsonUtil Maven / Gradle / Ivy
/*
* Copyright 2019 The Getty Project
*
* The Getty Project licenses this file to you under the Apache License,
* version 2.0 (the "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package com.gettyio.gim.utils;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import com.alibaba.fastjson.TypeReference;
import com.alibaba.fastjson.serializer.SerializeConfig;
import com.alibaba.fastjson.serializer.SerializerFeature;
import com.alibaba.fastjson.serializer.SimpleDateFormatSerializer;
import java.util.List;
import java.util.Map;
/**
* Created by gogym on 2017/8/15.
* Result obj = (Result) JSON.parseObject(js, new
* TypeReference>(){});
*/
public class FastJsonUtil {
private static final SerializeConfig config;
static {
String dateFormat = "yyyy-MM-dd HH:mm:ss";
config = new SerializeConfig();
// 使用和json-lib兼容的日期输出格式
config.put(java.util.Date.class, new SimpleDateFormatSerializer(dateFormat));
// 使用和json-lib兼容的日期输出格式
config.put(java.sql.Date.class, new SimpleDateFormatSerializer(dateFormat));
}
private static final SerializerFeature[] features = {
// 输出空置字段
SerializerFeature.WriteMapNullValue,
// list字段如果为null,输出为[],而不是null
SerializerFeature.WriteNullListAsEmpty,
// 数值字段如果为null,输出为0,而不是null
SerializerFeature.WriteNullNumberAsZero,
// Boolean字段如果为null,输出为false,而不是null
SerializerFeature.WriteNullBooleanAsFalse,
// 字符类型字段如果为null,输出为"",而不是null
SerializerFeature.WriteNullStringAsEmpty
};
public static String toJSONString(Object object) {
return JSON.toJSONString(object, config, features);
}
public static String toJSONNoFeatures(Object object) {
return JSON.toJSONString(object, config);
}
public static Object toBean(String text) {
return JSON.parse(text);
}
public static T toBean(String text, Class clazz) {
return JSON.parseObject(text, clazz);
}
public static T toBean(String str, TypeReference type) {
return JSON.parseObject(str, type);
}
/**
* 转换为数组
*
* @param text
* @param
* @return
*/
public static Object[] toArray(String text) {
return toArray(text, null);
}
/**
* 转换为数组
*
* @param text
* @param clazz
* @param
* @return
*/
public static Object[] toArray(String text, Class clazz) {
return JSON.parseArray(text, clazz).toArray();
}
/**
* 转换为List
*
* @param text
* @param clazz
* @param
* @return
*/
public static List toList(String text, Class clazz) {
return JSON.parseArray(text, clazz);
}
/**
* 将javabean转化为序列化的json字符串
*
* @param keyvalue
* @return
*/
public static Object beanToJson(Map keyvalue) {
String textJson = JSON.toJSONString(keyvalue);
Object objectJson = JSON.parse(textJson);
return objectJson;
}
/**
* 将string转化为序列化的json字符串
*
* @param text
* @return
*/
public static JSONObject textToJson(String text) {
JSONObject objectJson = JSON.parseObject(text);
return objectJson;
}
/**
* json字符串转化为map
*
* @param s
* @return
*/
public static Map stringToCollect(String s) {
Map m = JSONObject.parseObject(s);
return m;
}
/**
* 将map转化为string
*
* @param m
* @return
*/
public static String collectToString(Map m) {
String s = JSONObject.toJSONString(m);
return s;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy