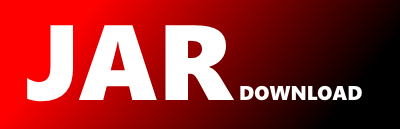
commonMain.com.giancarlobuenaflor.kflogger.backend.KLogData.kt Maven / Gradle / Ivy
package com.giancarlobuenaflor.kflogger.backend
import com.giancarlobuenaflor.kflogger.KLevel
import com.giancarlobuenaflor.kflogger.KLogSite
/**
* A backend API for determining metadata associated with a log statement.
*
* Some metadata is expected to be available for all log statements (such as the log level or a
* timestamp) whereas other data is optional (class/method name for example). As well providing the
* common logging metadata, customized loggers can choose to add arbitrary key/value pairs to the
* log data. It is up to each logging backend implementation to decide how it interprets this data
* using the hierarchical key. See [Metadata].
*/
public expect interface KLogData {
/**
* Returns whether this log statement should be emitted regardless of its log level or any other
* properties.
*
* This allows extensions of `LogContext` or `LoggingBackend` which implement additional filtering
* or rate-limiting fluent methods to easily check whether a log statement was forced. Forced log
* statements should behave exactly as if none of the filtering or rate-limiting occurred,
* including argument validity checks.
*
* Thus the idiomatic use of `wasForced()` is:
* `public API someFilteringMethod(int value) {
* if (wasForced()) {
* return api();
* }
* if (value < 0) {
* throw new IllegalArgumentException("Bad things ...");
* }
* // rest of method...
* }
* `
*
*
* Checking for forced log statements before checking the validity of arguments provides a
* last-resort means to mitigate cases in which syntactically incorrect log statements are only
* discovered when they are enabled.
*/
public fun wasForced(): Boolean
/** Returns the log level for the current log statement. */
public fun getLevel(): KLevel
/**
* Returns any additional metadata for this log statement. If no additional metadata is present,
* the immutable empty metadata instance is returned.
*
* IMPORTANT: The returned instance is restricted to metadata added at the log site, and will not
* include any scoped metadata to be applied to the log statement. To process combined log site
* and scoped metadata, obtain or create a [MetadataProcessor].
*/
public fun getMetadata(): KMetadata?
/** Returns a microsecond timestamp for the current log statement. */
@Deprecated("Use timestampNanos") public fun getTimestampMicros(): Long
/** Returns a nanosecond timestamp for the current log statement. */
public fun getTimestampNanos(): Long
/**
* Returns the logger name (which is usually a canonicalized class name) or `null` if not given.
*/
public fun getLoggerName(): String?
/**
* Returns the log site data for the current log statement.
*
* @throws IllegalStateException if called prior to the postProcess() method being called.
*/
public fun getLogSite(): KLogSite?
/**
* Returns the arguments to be formatted with the message. Arguments exist when a `log()` method
* with a format message and separate arguments was invoked.
*
* @throws IllegalStateException if no arguments are available (ie, when there is no template
* context).
*/
public fun getArguments(): Array?
/**
* Returns the single argument to be logged directly when no arguments were provided.
*
* @throws IllegalStateException if no single literal argument is available (ie, when a template
* context exists).
*/
public fun getLiteralArgument(): Any?
/**
* Returns a template key for this log statement, or `null` if the statement does not require
* formatting (in which case the message to be logged can be determined by calling
* [.getLiteralArgument]).
*/
public fun getTemplateContext(): KTemplateContext?
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy