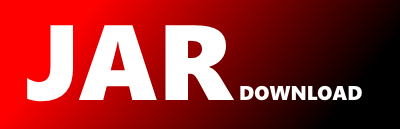
org.nico.cat.server.request.buddy.RequestContent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nocat Show documentation
Show all versions of nocat Show documentation
Java-based service container
package org.nico.cat.server.request.buddy;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.nico.cat.server.request.parameter.RequestMethod;
import org.nico.cat.server.util.UriUtils;
/**
* Request content
* @author nico
* @version createTime:2018年1月7日 下午7:08:12
*/
public class RequestContent {
/**
* Request uri
*/
private String uri;
/**
* Request method
*/
private RequestMethod method;
/**
* Request version
*/
private String version;
/**
* SessionId
*/
private String sessionId;
/**
* Request host
*/
private String host;
/**
* Request prot
*/
private String port;
/**
* Request body
*/
private String body;
/**
* Uri Redirect
*/
private String uriRedirect;
/**
* Api Redirect
*/
private String apiRedirect;
/**
* Request url properties
*/
private Map properties;
/**
* Request headers
*/
private Map headers;
{
properties = new HashMap();
}
public Map getProperties() {
return properties;
}
public void setProperties(Map properties) {
this.properties = properties;
}
/**
* Get the attribute value
*
* @param name
* The property name
*
* @return {@link String}
*/
public String getProperty(String name){
if(this.properties.get(name) != null){
return this.properties.get(name).toString();
}else{
return null;
}
}
public void setProperty(String key, String value){
this.properties.put(key, value);
}
public Map getHeaders() {
return headers;
}
public String getSessionId() {
return sessionId;
}
public void setSessionId(String sessionId) {
this.sessionId = sessionId;
}
public String getHeader(String name){
return headers != null ? (String) headers.get(name) : null;
}
public void setHeaders(Map headers) {
this.headers = headers;
}
/**
* Access to flow
*
* @param name
* The property name
*
* @return {@link InputStream}
*/
public InputStream getStream(String name){
Object obj = getProperty(name);
if(obj != null){
if(obj instanceof InputStream){
return (InputStream)obj;
}
}
return null;
}
public String getBody() {
return body;
}
public void setBody(String body) {
this.body = body;
}
public String getUri() {
return uri;
}
public void setUri(String uri) {
this.uri = UriUtils.getUri(uri);
Map properties = UriUtils.getProperties(uri);
if(properties != null){
this.properties.putAll(properties);
}
}
public RequestMethod getMethod() {
return method;
}
public void setMethod(RequestMethod method) {
this.method = method;
}
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
public String getHost() {
return host;
}
public void setHost(String host) {
this.host = host;
}
public String getPort() {
return port;
}
public void setPort(String port) {
this.port = port;
}
public String getUriRedirect() {
return uriRedirect;
}
public void setUriRedirect(String uriRedirect) {
this.uriRedirect = uriRedirect;
}
public String getApiRedirect() {
return apiRedirect;
}
public void setApiRedirect(String apiRedirect) {
this.apiRedirect = apiRedirect;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy