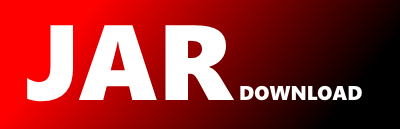
com.gitee.beiding.template_excel.AutoIncrementNumber Maven / Gradle / Ivy
package com.gitee.beiding.template_excel;
import java.util.*;
import java.util.stream.Collectors;
class AutoIncrementNumber {
private Map maxValues;
private W last;
//
void init(Map maxValues) {
this.maxValues = maxValues;
W f = last = new W();
List list = maxValues.keySet().stream().sorted(Comparator.reverseOrder()).collect(Collectors.toList());
f.name = list.get(0);
last.value = -1;
values.put(last.name, last.value);
for (int i = 1; i < list.size(); i++) {//构建链结构
W w = new W();
w.name = list.get(i);
w.next = f;
f.pre = w;
f = w;
values.put(f.name, f.value);
}
}
private Map values = new HashMap<>();
boolean autoIncrement() {
return autoIncrement(last);
}
//自增
private boolean autoIncrement(W current) {
current.value++;
values.put(current.name, current.value);
if (current.value <= maxValues.get(current.name)) {
return true;
} else {//自增已到达数当前位边界
//已经到最大
if (current.pre == null) {
return false;
} else {
//重置当前位
current.value = 0;//重置后
values.put(current.name, current.value);
//上一位自增
return autoIncrement(current.pre);
}
}
}
Map getValues() {
return values;
}
//位
private class W {
//名称
String name;
int value = 0;
//下一位
W next;
//上一位
W pre;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy