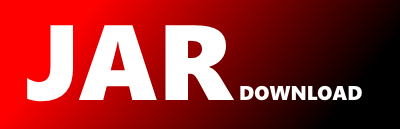
com.gitee.beiding.template_excel.DoubleActingIndex Maven / Gradle / Ivy
package com.gitee.beiding.template_excel;
/*
双动指针
该对象中维护两个指针
使用条件决定指针的移动策略
*/
class DoubleActingIndex {
interface Handle {
//是否具有更多
boolean next();
//是否应该换边
boolean shouldChange();
//换边完成后
void afterChange();
//被换到该边以后
void afterChangeTo();
}
private static Handle empty = new Handle() {
@Override
public boolean shouldChange() {
return false;
}
@Override
public boolean next() {
return false;
}
@Override
public void afterChange() {
}
@Override
public void afterChangeTo() {
}
};
private class Index {
private Handle handle = empty;
private Index another;
}
//左侧指针
private Index left = new Index();
//右侧指针
private Index right = new Index();
{
left.another = right;
right.another = left;
}
private static Integer LEFT = 0;
private static Integer RIGHT = 1;
public void setStart(int start) {
if (start == LEFT) {
this.current = left;
} else if (start == RIGHT) {
this.current = right;
}
}
//当前移动的边
private Index current = right;
//触发下一个
public boolean next() {
//直接返回
if (!this.current.handle.next()) {
return false;
}
if (this.current.handle.shouldChange()) {
//换边的行为
this.current.handle.afterChange();
this.current = this.current.another;
//被换到以后
this.current.handle.afterChangeTo();
}
return true;
}
public void setRight(Handle handle) {
this.right.handle = handle;
}
public void setLeft(Handle handle) {
this.left.handle = handle;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy