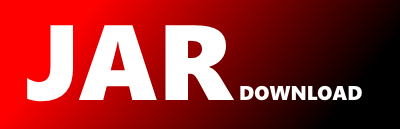
com.gitee.beiding.template_excel.RegexExtractCell Maven / Gradle / Ivy
package com.gitee.beiding.template_excel;
import java.util.*;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
class RegexExtractCell extends ExtractCell {
//正则型提取
private Pattern matchPattern;
private Set variables;
void setVariables(Set variables) {
this.variables = variables;
}
private Set transmutativeGroups;
void setTransmutativeGroups(Set transmutativeGroups) {
this.transmutativeGroups = transmutativeGroups;
}
RegexExtractCell() {
}
void setMatchPattern(Pattern matchPattern) {
this.matchPattern = matchPattern;
}
//根据表达式编译生成一个提取单元格
static RegexExtractCell compile(String exp) {
return ExtractCellCompileUtils.compile(exp);
}
public Map extract(Object object, Merge merge) {
if (object == null) {
return null;
}
//默认只要一行满足
int rowNumber = 1;
if (this.merge != merge) {
//如果列不相同直接返回不匹配
if (this.merge.getCol() != merge.getCol()) {
return null;
}
if (this.merge.getRow() == 1) {
rowNumber = merge.getRow();
} else {
return null;
}
}
//将对象转换为字符串类型
String text = object.toString();
//TODO 只有正则表达式才能提取出来结果,而且提取出来的结果一定时字符串类型
Matcher matcher = matchPattern.matcher(text);
if (matcher.find()) {
Map map = new HashMap<>();
int i = matcher.groupCount();
if (variables != null) {
for (String variable : variables) {
if (transmutativeGroups.contains(variable)) {
map.put(variable.replace("0BRACKET0PRE0", "[").replace("0BRACKET0POST0", "]").replace("0POINT0", "."), new ValueHolder(matcher.group(variable), rowNumber));
this.blankToken = rowNumber - 1;
} else {
map.put(variable, new ValueHolder(matcher.group(variable), rowNumber));
this.blankToken = rowNumber - 1;
}
}
}
return map;
} else {
//如果不匹配则返回空
return null;
}
}
@Override
public String toString() {
String s = "正则表达式\t提取的变量\n";
s += matchPattern == null ? "-\t" : matchPattern.pattern() + "\t";
s += variables == null ? "-\t" : variables + "\t";
return s;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy