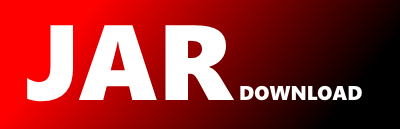
com.gitee.beiding.template_excel.TemplateCell Maven / Gradle / Ivy
package com.gitee.beiding.template_excel;
//模板单元
import java.util.*;
class TemplateCell {
static TemplateCell parse(Object s) {
TemplateCell r = new TemplateCell();
if (s instanceof String) {
TemplateCellParseUtils.Result array = TemplateCellParseUtils.parse((String) s);
LinkedHashMap> map = array.getCheckJsMap();
if (map.size() > 0) {
//初始化
r.arrayIndex = new ArrayIndex();
r.arrayIndex.init(map);
r.indexSet = map.keySet();
//初始化?
r.indexMax = new HashMap<>();
for (String index : r.indexSet) {
r.indexMax.put(index, 0);
}
}
//放入表达式
r.expression = array.getExpression();
r.commands = array.getCommands();
} else {
r.singleResult = s;
}
return r;
}
private TemplateCell() {
}
private List commands;
//单元格表达式
private String expression;
//数组索引
private ArrayIndex arrayIndex;
private Set indexSet;
private Map indexMax;
private Object singleResult;
private DB db;
Map getIndexMax() {
return indexMax;
}
Object getSingleResult() {
return singleResult;
}
//运算
void exe() {
if (expression != null) {
Map condition = new HashMap<>();
if (arrayIndex != null) {
db = new DB(indexSet);
//TODO 数字的自增问题??
while (arrayIndex.autoIncrement()) {
for (String s : indexMax.keySet()) {
int v = (Integer) Js.get(s);
Integer currentMax = indexMax.get(s);
if (currentMax == null || v > currentMax) {
indexMax.put(s, v);
}
condition.put(s, v);
}
//放入结果集
try {
db.putValue(condition, Js.exe(expression));
} catch (Exception e) {//TODO 这里可能会数组越界,直接忽略即可
// System.err.println(condition + " " + expression);
//System.err.println(expression);
// db.putValue(condition, null);
}
}
//清空临时变量
arrayIndex.clear();
} else {
try {
singleResult = Js.exe(expression);
} catch (Exception e) {
// System.err.println(expression);
}
}
}
}
/*
建立索引
*/
Object get(Map index) {
Object r;
if (arrayIndex == null) {
r = singleResult;
} else {
r = db.andValue(index);
}
if (commands != null) {
if (r == null) {
return null;
}
//TODO 断言 r一定为字符串类型
String s = (String) r;
Iterator iterator = commands.iterator();
if (iterator.hasNext()) {
String command = iterator.next();
try {
Object exe = Js.exe(command);
if ((s.replace(command, "").trim().equals(""))) {
return exe;
}
if (exe == null) {
s = s.replace(command, "");
} else {
s = s.replace(command, exe.toString());
}
while (iterator.hasNext()) {
try {
command = iterator.next();
exe = Js.exe(command);
if (exe == null) {
s = s.replace(command, "");
} else {
s = s.replace(command, exe.toString());
}
} catch (Exception ignore) {
}
}
} catch (Exception ignore) {
}
}
return s;
}
return r;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy