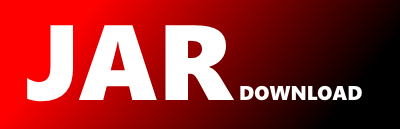
com.gitee.cn9750wang.webtools.query.LimitPage Maven / Gradle / Ivy
/*
* Copyright 2021 wwy
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gitee.cn9750wang.webtools.query;
import com.gitee.cn9750wang.webtools.error.P21Exception;
import java.io.Serializable;
/**
* 分页查询对象
*
* @author wwy
*/
public class LimitPage implements Serializable {
private static final long serialVersionUID = 1L;
/** 默认 每页大小 */
public static final int DEFAULT_SIZE = 10;
/** 默认 每页最大200页 */
public static final int DEFAULT_MAX_SIZE = 200;
/** 当前所要查询的页码 */
private long page = 1;
/** 每页的大小 */
private long size = DEFAULT_SIZE;
/**
* 构造函数
* 通过一定格式的字符串方式查询
* @param queryStr 按格式排布的字符串
*/
public LimitPage(String queryStr){
// 缺少必要参数
if(queryStr == null || queryStr.isEmpty()){
return;
}
// 按逗号分割字符串
var split = queryStr.split(",");
switch (split.length){
case 1:page = Long.parseLong(split[0]);break;
case 2:
page = Long.parseLong(split[0]);
size = Long.parseLong(split[1]);
break;
default:throw new P21Exception("分页查询参数格式不正确");
}
// 合理化参数
this.rationalization();
}
/**
* 构造函数
* @param page 第几页
*/
public LimitPage(long page){
this(page,DEFAULT_SIZE);
}
/**
* 构造函数
* @param page 第几页
* @param size 每页大小
*/
public LimitPage(long page,long size){
this.page = page;
this.size = size;
}
public long getPage() {
return page;
}
public long getSize() {
return size;
}
/**
* 数据库查询语句中 limit [limit] offset [offset] 的limit
* @return 每页大小
*/
public long getLimit() {
return size;
}
/**
* 数据库查询语句中 limit [index] offset [offset] 的offset
* @return 分页偏移量
*/
public long getOffset() {
return (page - 1) * size;
}
/**
* 合理化分页参数,默认每页不超过200条数据
* @return LimitPage分页对象
*/
public LimitPage rationalization(){
return this.rationalization(DEFAULT_MAX_SIZE);
}
/**
* 合理化分页参数
* @param maxSize 自定义每页最大分页参数
* @return LimitPage分页对象
*/
public LimitPage rationalization(final long maxSize){
// 页码应为正整数,否则默认从第一页开始
page = page >= 1 ? page : 1;
// 每页大小是否合理,否则默认每页10条数据
size = size >= 1 && size <= maxSize ? size : DEFAULT_SIZE;
return this;
}
/**
* 限制每页大小为excel2007(xls格式)最大行数
* @return LimitPage分页对象
*/
public LimitPage xlsMaxRow(){
return this.rationalization(256*256);
}
/**
* 限制每页大小为excel2010(xlsx格式)最大行数
* @return LimitPage分页对象
*/
public LimitPage xlsxMaxRow(){
return this.rationalization(1024*1024);
}
/**
* 生成mysql limit查询语句
* @return mysql limit查询语句
*/
public String mysql(){
return "limit " + getLimit() + " offset "+ getOffset();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy