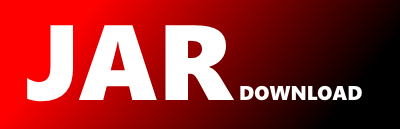
com.gitee.feizns.convert.ConvertUtils Maven / Gradle / Ivy
package com.gitee.feizns.convert;
import com.gitee.feizns.convert.impl.DateConverter;
import com.gitee.feizns.convert.impl.NumberConverter;
import com.gitee.feizns.convert.impl.StringConverter;
import com.gitee.feizns.convert.method.MethodConverter;
import com.gitee.feizns.convert.method.valueof.SingleParameterValueOfConverter;
import com.gitee.feizns.convert.method.valueof.TwoParametersValueOfConverter;
import com.gitee.feizns.reflect.TypeUtils;
import java.util.Comparator;
import java.util.List;
import java.util.TreeSet;
import java.util.stream.Collectors;
/**
* @author feizns
* @since 2019/5/19 0019
*/
public abstract class ConvertUtils {
/**
* 常规转换器
*/
private static final TreeSet COMMONS_CONVERTERS = new TreeSet<>(priority());
/**
* 方法转换器
*/
private static final TreeSet METHOD_CONVERTERS = new TreeSet<>(priority());
static {
registry(new NumberConverter());
registry(new DateConverter());
registry(new StringConverter());
registry(new SingleParameterValueOfConverter());
registry(new TwoParametersValueOfConverter());
}
public static T to(Object original, Class targetType){
if ( original != null ) {
List converters = COMMONS_CONVERTERS.stream().filter(converter -> converter.support(targetType)).collect(Collectors.toList());
if ( converters.isEmpty() )
return (T) to(METHOD_CONVERTERS, original, TypeUtils.getWrapper(targetType));
else
return to(converters, original, targetType);
}
return null;
}
public static final T to(Object original, Class targetType, T defaultVal) {
T ret = null;
return (ret = to(original, targetType)) != null
? ret
: defaultVal;
}
/**
* 任意类型转换
* @param converters
* @param original
* @param targetType
* @return
*/
private static T to(Iterable converters, Object original, Class targetType) {
for (Converter converter : converters) {
if ( converter.support(targetType) ) {
Object result = converter.to(original, targetType);
if ( result != null )
return (T) result;
}
}
return null;
}
/**
* 注册转换器
* @param converter
*/
public static final void registry(Converter converter) {
if ( converter instanceof MethodConverter ) {
MethodConverter methodConverter = (MethodConverter) converter;
methodConverter.setConverters(COMMONS_CONVERTERS);
METHOD_CONVERTERS.add(methodConverter);
} else {
COMMONS_CONVERTERS.add(converter);
}
}
/**
* 排序规则
* @return
*/
private static final Comparator super Converter> priority() {
return (o1, o2) -> o1.priority() < o2.priority() ? -1 : 1;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy