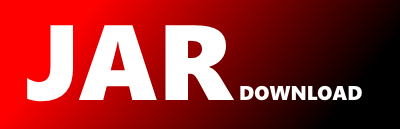
com.gitee.feizns.reflect.MethodUtils Maven / Gradle / Ivy
package com.gitee.feizns.reflect;
import java.lang.reflect.Method;
import java.util.Arrays;
import java.util.function.Predicate;
import java.util.stream.Stream;
/**
* 方法.
* @author feizns
* @since 2019/5/15
*/
public abstract class MethodUtils {
/**
* 获取方法
* @param obj
* @param name
* @return
*/
public static final Method getDeclared(Object obj, String name, Class>... parameterTypes) {
return getDeclared(obj.getClass(), name, parameterTypes);
}
/**
* 筛选所有的方法
* @param type
* @param filter
* @return
*/
public static final Stream getDeclared(Class> type, Predicate filter) {
Stream.Builder builder = Stream.builder();
while ( type != null ) {
Arrays.stream(type.getDeclaredMethods()).forEach(method -> {
if ( filter.test( TypeUtils.accessible(method) ) ) {
builder.add(method);
}
});
type = type.getSuperclass();
}
return builder.build();
}
/**
* 获取方法
* @param type
* @param name
* @param parameterTypes
* @return
*/
public static final Method getDeclared(Class> type, String name, Class>... parameterTypes) {
while ( type != null ) {
try {
return TypeUtils.accessible(type.getDeclaredMethod(name, parameterTypes));
} catch (NoSuchMethodException e) {
type = type.getSuperclass();
}
}
return null;
}
/**
* 获取公共方法
* @param obj
* @param name
* @param parameterTypes
* @return
*/
public static final Method get(Object obj, String name, Class>... parameterTypes) {
return get(obj.getClass(), name, parameterTypes);
}
/**
* 获取公共方法
* @param type
* @param name
* @param parameterTypes
* @return
*/
public static final Method get(Class> type, String name, Class>... parameterTypes) {
try {
return TypeUtils.accessible(type.getMethod(name, parameterTypes));
} catch (NoSuchMethodException e) {
return null;
}
}
/**
* 自定义查找规则
* @param type
* @param filter
* @return
*/
public static final Stream get(Class> type, Predicate filter) {
return Arrays.stream(type.getMethods()).filter(filter);
}
/**
* 调用方法
* @param obj
* @param name
* @param params
* @return
*/
public static final Object invoke(Object obj, String name, Object... params) {
try {
return TypeUtils.accessible(obj.getClass().getMethod(name, ClassUtils.getClasses(params)))
.invoke(obj, params);
} catch (ReflectiveOperationException e) {
return null;
}
}
/**
* 调用方法
* @param method
* @param params
* @return
*/
public static final Object invoke(Method method, Object... params) {
try {
return TypeUtils.accessible(method).invoke(null, params);
} catch (ReflectiveOperationException e) {
return null;
}
}
/**
* 调用方法
* @param method
* @param params
* @return
*/
public static final Object invoke(Object obj, Method method, Object... params) {
try {
return TypeUtils.accessible(method).invoke(obj, params);
} catch (ReflectiveOperationException e) {
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy