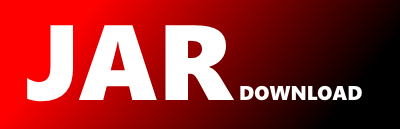
com.gitee.feizns.reflect.FieldUtils Maven / Gradle / Ivy
package com.gitee.feizns.reflect;
import java.lang.reflect.Field;
/**
* @author feizns
* @since 2019/5/12 0012
*/
public abstract class FieldUtils {
/**
* 获取属性
* @param obj
* @param name
* @return
*/
public static final Field get(Object obj, String name) {
return get(obj.getClass(), name);
}
/**
* 获取属性
* @param type
* @param name
* @return
*/
public static final Field get(Class> type, String name) {
while ( type != null ) {
try {
Field field = type.getDeclaredField(name);
field.setAccessible(true);
return field;
} catch (NoSuchFieldException e) {
type = type.getSuperclass();
}
}
return null;
}
/**
* 获取字段的值
* @param obj
* @param field
* @param
* @return
*/
public static final T getData(Object obj, Field field) {
try {
field.setAccessible(true);
return (T) field.get(obj);
} catch (IllegalAccessException e) {
return null;
}
}
/**
* 获取属性的值
* @param obj
* @param name
* @param
* @return
*/
public static final T getData(Object obj, String name) {
Field field = get(obj, name);
return field != null ? getData(obj, field) : null;
}
/**
* 获取字段的值
* @param obj
* @param name
* @param resultType
* @param
* @return
*/
public static final T getData(Object obj, String name, Class resultType) {
Field field = get(obj, name);
if ( field != null ) {
Object data = getData(obj, field);
if ( data != null && resultType.isAssignableFrom(data.getClass()) ) {
return (T) data;
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy