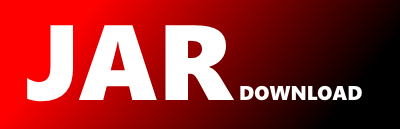
com.gitee.feizns.dynamic.decrypt.Aes Maven / Gradle / Ivy
package com.gitee.feizns.dynamic.decrypt;
import com.gitee.feizns.dynamic.Strings;
import lombok.SneakyThrows;
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.security.SecureRandom;
import java.util.Base64;
/**
* aes加密
* @link java加密-AES加解密
* @author feizns
* @since 2023/04/04
*/
public class Aes {
private static final String KEY_ALGORITHM = "AES";
/**
* 默认的加密算法
*/
private static final String DEFAULT_CIPHER_ALGORITHM = "AES/ECB/PKCS5Padding";
/**
* AES 加密操作
* @param content 待加密内容
* @param key 加密密钥
* @return 返回Base64转码后的加密数据
*/
public static String encrypt(String content, String key) throws Exception {
// 创建密码器
Cipher cipher = Cipher.getInstance(DEFAULT_CIPHER_ALGORITHM);
byte[] byteContent = Strings.bytes(content);
// 初始化为加密模式的密码器
cipher.init(Cipher.ENCRYPT_MODE, generateSecretKey(key));
// 加密
byte[] result = cipher.doFinal(byteContent);
//通过Base64转码返回
return byte2Base64(result);
}
/**
* AES 解密操作
* @param content 内容
* @param key 关键
* @return {@link String}
*/
@SneakyThrows
public static String decrypt(String content, String key) {
//实例化
Cipher cipher = Cipher.getInstance(DEFAULT_CIPHER_ALGORITHM);
//使用密钥初始化,设置为解密模式
cipher.init(Cipher.DECRYPT_MODE, generateSecretKey(key));
//执行操作
byte[] result = cipher.doFinal(base642Byte(content));
return new String(result, StandardCharsets.UTF_8);
}
/**
* 生成加密秘钥
* @param key 关键
* @return {@link SecretKeySpec}
*/
@SneakyThrows
private static SecretKeySpec generateSecretKey(final String key) {
//返回生成指定算法密钥生成器的 KeyGenerator 对象
KeyGenerator kg = KeyGenerator.getInstance(KEY_ALGORITHM);
// 此类提供加密的强随机数生成器 (RNG),该实现在windows上每次生成的key都相同,但是在部分linux或solaris系统上则不同。
// SecureRandom random = new SecureRandom(key.getBytes());
// 指定算法名称,不同的系统上生成的key是相同的。
SecureRandom random = SecureRandom.getInstance("SHA1PRNG");
random.setSeed(Strings.bytes(key));
//AES 要求密钥长度为 128
kg.init(128, random);
//生成一个密钥
SecretKey secretKey = kg.generateKey();
// 转换为AES专用密钥
return new SecretKeySpec(secretKey.getEncoded(), KEY_ALGORITHM);
}
/**
* 字节数组转Base64编码
* @param bytes 字节
* @return {@link String}
*/
public static String byte2Base64(byte[] bytes) {
return Base64.getEncoder().encodeToString(bytes);
}
/**
* Base64编码转字节数组
* @param base64Key base64关键
* @return {@code byte[]}
*/
public static byte[] base642Byte(String base64Key) {;
return Base64.getDecoder().decode(Strings.bytes(base64Key));
}
@SneakyThrows
public static void main(String[] args) {
System.out.println(encrypt("hello", "123456"));
System.out.println(encrypt("hello", "123456"));
System.out.println(decrypt("NvS+en33crrh4tP9RdE//A==", "123456"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy