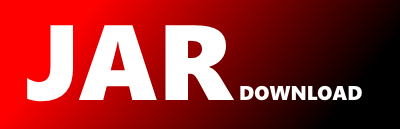
jmash.file.FileGrpc Maven / Gradle / Ivy
package jmash.file;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*
*File Service
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.60.1)",
comments = "Source: jmash/file/file_rpc.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class FileGrpc {
private FileGrpc() {}
public static final java.lang.String SERVICE_NAME = "jmash.file.File";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getFindEnumListMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "findEnumList",
requestType = com.google.protobuf.StringValue.class,
responseType = jmash.protobuf.EnumValueList.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getFindEnumListMethod() {
io.grpc.MethodDescriptor getFindEnumListMethod;
if ((getFindEnumListMethod = FileGrpc.getFindEnumListMethod) == null) {
synchronized (FileGrpc.class) {
if ((getFindEnumListMethod = FileGrpc.getFindEnumListMethod) == null) {
FileGrpc.getFindEnumListMethod = getFindEnumListMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "findEnumList"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.StringValue.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
jmash.protobuf.EnumValueList.getDefaultInstance()))
.setSchemaDescriptor(new FileMethodDescriptorSupplier("findEnumList"))
.build();
}
}
}
return getFindEnumListMethod;
}
private static volatile io.grpc.MethodDescriptor getFindEnumMapMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "findEnumMap",
requestType = com.google.protobuf.StringValue.class,
responseType = jmash.protobuf.CustomEnumValueMap.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getFindEnumMapMethod() {
io.grpc.MethodDescriptor getFindEnumMapMethod;
if ((getFindEnumMapMethod = FileGrpc.getFindEnumMapMethod) == null) {
synchronized (FileGrpc.class) {
if ((getFindEnumMapMethod = FileGrpc.getFindEnumMapMethod) == null) {
FileGrpc.getFindEnumMapMethod = getFindEnumMapMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "findEnumMap"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.StringValue.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
jmash.protobuf.CustomEnumValueMap.getDefaultInstance()))
.setSchemaDescriptor(new FileMethodDescriptorSupplier("findEnumMap"))
.build();
}
}
}
return getFindEnumMapMethod;
}
private static volatile io.grpc.MethodDescriptor getFindJmashFilePageMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "findJmashFilePage",
requestType = jmash.file.protobuf.JmashFileReq.class,
responseType = jmash.file.protobuf.JmashFilePage.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getFindJmashFilePageMethod() {
io.grpc.MethodDescriptor getFindJmashFilePageMethod;
if ((getFindJmashFilePageMethod = FileGrpc.getFindJmashFilePageMethod) == null) {
synchronized (FileGrpc.class) {
if ((getFindJmashFilePageMethod = FileGrpc.getFindJmashFilePageMethod) == null) {
FileGrpc.getFindJmashFilePageMethod = getFindJmashFilePageMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "findJmashFilePage"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
jmash.file.protobuf.JmashFileReq.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
jmash.file.protobuf.JmashFilePage.getDefaultInstance()))
.setSchemaDescriptor(new FileMethodDescriptorSupplier("findJmashFilePage"))
.build();
}
}
}
return getFindJmashFilePageMethod;
}
private static volatile io.grpc.MethodDescriptor getFindJmashFileListMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "findJmashFileList",
requestType = jmash.file.protobuf.JmashFileReq.class,
responseType = jmash.file.protobuf.JmashFileList.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getFindJmashFileListMethod() {
io.grpc.MethodDescriptor getFindJmashFileListMethod;
if ((getFindJmashFileListMethod = FileGrpc.getFindJmashFileListMethod) == null) {
synchronized (FileGrpc.class) {
if ((getFindJmashFileListMethod = FileGrpc.getFindJmashFileListMethod) == null) {
FileGrpc.getFindJmashFileListMethod = getFindJmashFileListMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "findJmashFileList"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
jmash.file.protobuf.JmashFileReq.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
jmash.file.protobuf.JmashFileList.getDefaultInstance()))
.setSchemaDescriptor(new FileMethodDescriptorSupplier("findJmashFileList"))
.build();
}
}
}
return getFindJmashFileListMethod;
}
private static volatile io.grpc.MethodDescriptor getFindJmashFileByIdMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "findJmashFileById",
requestType = jmash.file.protobuf.JmashFileKey.class,
responseType = jmash.file.protobuf.JmashFileModel.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getFindJmashFileByIdMethod() {
io.grpc.MethodDescriptor getFindJmashFileByIdMethod;
if ((getFindJmashFileByIdMethod = FileGrpc.getFindJmashFileByIdMethod) == null) {
synchronized (FileGrpc.class) {
if ((getFindJmashFileByIdMethod = FileGrpc.getFindJmashFileByIdMethod) == null) {
FileGrpc.getFindJmashFileByIdMethod = getFindJmashFileByIdMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "findJmashFileById"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
jmash.file.protobuf.JmashFileKey.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
jmash.file.protobuf.JmashFileModel.getDefaultInstance()))
.setSchemaDescriptor(new FileMethodDescriptorSupplier("findJmashFileById"))
.build();
}
}
}
return getFindJmashFileByIdMethod;
}
private static volatile io.grpc.MethodDescriptor getDeleteJmashFileMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "deleteJmashFile",
requestType = jmash.file.protobuf.JmashFileKey.class,
responseType = jmash.file.protobuf.JmashFileModel.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getDeleteJmashFileMethod() {
io.grpc.MethodDescriptor getDeleteJmashFileMethod;
if ((getDeleteJmashFileMethod = FileGrpc.getDeleteJmashFileMethod) == null) {
synchronized (FileGrpc.class) {
if ((getDeleteJmashFileMethod = FileGrpc.getDeleteJmashFileMethod) == null) {
FileGrpc.getDeleteJmashFileMethod = getDeleteJmashFileMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "deleteJmashFile"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
jmash.file.protobuf.JmashFileKey.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
jmash.file.protobuf.JmashFileModel.getDefaultInstance()))
.setSchemaDescriptor(new FileMethodDescriptorSupplier("deleteJmashFile"))
.build();
}
}
}
return getDeleteJmashFileMethod;
}
private static volatile io.grpc.MethodDescriptor getBatchDeleteJmashFileMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "batchDeleteJmashFile",
requestType = jmash.file.protobuf.JmashFileKeyList.class,
responseType = com.google.protobuf.Int32Value.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getBatchDeleteJmashFileMethod() {
io.grpc.MethodDescriptor getBatchDeleteJmashFileMethod;
if ((getBatchDeleteJmashFileMethod = FileGrpc.getBatchDeleteJmashFileMethod) == null) {
synchronized (FileGrpc.class) {
if ((getBatchDeleteJmashFileMethod = FileGrpc.getBatchDeleteJmashFileMethod) == null) {
FileGrpc.getBatchDeleteJmashFileMethod = getBatchDeleteJmashFileMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "batchDeleteJmashFile"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
jmash.file.protobuf.JmashFileKeyList.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Int32Value.getDefaultInstance()))
.setSchemaDescriptor(new FileMethodDescriptorSupplier("batchDeleteJmashFile"))
.build();
}
}
}
return getBatchDeleteJmashFileMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static FileStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public FileStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new FileStub(channel, callOptions);
}
};
return FileStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static FileBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public FileBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new FileBlockingStub(channel, callOptions);
}
};
return FileBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static FileFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public FileFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new FileFutureStub(channel, callOptions);
}
};
return FileFutureStub.newStub(factory, channel);
}
/**
*
*File Service
*
*/
public interface AsyncService {
/**
*
* 枚举值列表
*
*/
default void findEnumList(com.google.protobuf.StringValue request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getFindEnumListMethod(), responseObserver);
}
/**
*
* 枚举值Map
*
*/
default void findEnumMap(com.google.protobuf.StringValue request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getFindEnumMapMethod(), responseObserver);
}
/**
*
* 查询翻页信息
*
*/
default void findJmashFilePage(jmash.file.protobuf.JmashFileReq request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getFindJmashFilePageMethod(), responseObserver);
}
/**
*
* 查询列表信息
*
*/
default void findJmashFileList(jmash.file.protobuf.JmashFileReq request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getFindJmashFileListMethod(), responseObserver);
}
/**
*
* 查询
*
*/
default void findJmashFileById(jmash.file.protobuf.JmashFileKey request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getFindJmashFileByIdMethod(), responseObserver);
}
/**
*
* 删除
*
*/
default void deleteJmashFile(jmash.file.protobuf.JmashFileKey request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getDeleteJmashFileMethod(), responseObserver);
}
/**
*
* 批量删除
*
*/
default void batchDeleteJmashFile(jmash.file.protobuf.JmashFileKeyList request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getBatchDeleteJmashFileMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service File.
*
*File Service
*
*/
public static abstract class FileImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return FileGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service File.
*
*File Service
*
*/
public static final class FileStub
extends io.grpc.stub.AbstractAsyncStub {
private FileStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected FileStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new FileStub(channel, callOptions);
}
/**
*
* 枚举值列表
*
*/
public void findEnumList(com.google.protobuf.StringValue request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getFindEnumListMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* 枚举值Map
*
*/
public void findEnumMap(com.google.protobuf.StringValue request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getFindEnumMapMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* 查询翻页信息
*
*/
public void findJmashFilePage(jmash.file.protobuf.JmashFileReq request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getFindJmashFilePageMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* 查询列表信息
*
*/
public void findJmashFileList(jmash.file.protobuf.JmashFileReq request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getFindJmashFileListMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* 查询
*
*/
public void findJmashFileById(jmash.file.protobuf.JmashFileKey request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getFindJmashFileByIdMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* 删除
*
*/
public void deleteJmashFile(jmash.file.protobuf.JmashFileKey request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getDeleteJmashFileMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* 批量删除
*
*/
public void batchDeleteJmashFile(jmash.file.protobuf.JmashFileKeyList request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getBatchDeleteJmashFileMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service File.
*
*File Service
*
*/
public static final class FileBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private FileBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected FileBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new FileBlockingStub(channel, callOptions);
}
/**
*
* 枚举值列表
*
*/
public jmash.protobuf.EnumValueList findEnumList(com.google.protobuf.StringValue request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getFindEnumListMethod(), getCallOptions(), request);
}
/**
*
* 枚举值Map
*
*/
public jmash.protobuf.CustomEnumValueMap findEnumMap(com.google.protobuf.StringValue request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getFindEnumMapMethod(), getCallOptions(), request);
}
/**
*
* 查询翻页信息
*
*/
public jmash.file.protobuf.JmashFilePage findJmashFilePage(jmash.file.protobuf.JmashFileReq request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getFindJmashFilePageMethod(), getCallOptions(), request);
}
/**
*
* 查询列表信息
*
*/
public jmash.file.protobuf.JmashFileList findJmashFileList(jmash.file.protobuf.JmashFileReq request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getFindJmashFileListMethod(), getCallOptions(), request);
}
/**
*
* 查询
*
*/
public jmash.file.protobuf.JmashFileModel findJmashFileById(jmash.file.protobuf.JmashFileKey request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getFindJmashFileByIdMethod(), getCallOptions(), request);
}
/**
*
* 删除
*
*/
public jmash.file.protobuf.JmashFileModel deleteJmashFile(jmash.file.protobuf.JmashFileKey request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getDeleteJmashFileMethod(), getCallOptions(), request);
}
/**
*
* 批量删除
*
*/
public com.google.protobuf.Int32Value batchDeleteJmashFile(jmash.file.protobuf.JmashFileKeyList request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getBatchDeleteJmashFileMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service File.
*
*File Service
*
*/
public static final class FileFutureStub
extends io.grpc.stub.AbstractFutureStub {
private FileFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected FileFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new FileFutureStub(channel, callOptions);
}
/**
*
* 枚举值列表
*
*/
public com.google.common.util.concurrent.ListenableFuture findEnumList(
com.google.protobuf.StringValue request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getFindEnumListMethod(), getCallOptions()), request);
}
/**
*
* 枚举值Map
*
*/
public com.google.common.util.concurrent.ListenableFuture findEnumMap(
com.google.protobuf.StringValue request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getFindEnumMapMethod(), getCallOptions()), request);
}
/**
*
* 查询翻页信息
*
*/
public com.google.common.util.concurrent.ListenableFuture findJmashFilePage(
jmash.file.protobuf.JmashFileReq request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getFindJmashFilePageMethod(), getCallOptions()), request);
}
/**
*
* 查询列表信息
*
*/
public com.google.common.util.concurrent.ListenableFuture findJmashFileList(
jmash.file.protobuf.JmashFileReq request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getFindJmashFileListMethod(), getCallOptions()), request);
}
/**
*
* 查询
*
*/
public com.google.common.util.concurrent.ListenableFuture findJmashFileById(
jmash.file.protobuf.JmashFileKey request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getFindJmashFileByIdMethod(), getCallOptions()), request);
}
/**
*
* 删除
*
*/
public com.google.common.util.concurrent.ListenableFuture deleteJmashFile(
jmash.file.protobuf.JmashFileKey request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getDeleteJmashFileMethod(), getCallOptions()), request);
}
/**
*
* 批量删除
*
*/
public com.google.common.util.concurrent.ListenableFuture batchDeleteJmashFile(
jmash.file.protobuf.JmashFileKeyList request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getBatchDeleteJmashFileMethod(), getCallOptions()), request);
}
}
private static final int METHODID_FIND_ENUM_LIST = 0;
private static final int METHODID_FIND_ENUM_MAP = 1;
private static final int METHODID_FIND_JMASH_FILE_PAGE = 2;
private static final int METHODID_FIND_JMASH_FILE_LIST = 3;
private static final int METHODID_FIND_JMASH_FILE_BY_ID = 4;
private static final int METHODID_DELETE_JMASH_FILE = 5;
private static final int METHODID_BATCH_DELETE_JMASH_FILE = 6;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_FIND_ENUM_LIST:
serviceImpl.findEnumList((com.google.protobuf.StringValue) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_FIND_ENUM_MAP:
serviceImpl.findEnumMap((com.google.protobuf.StringValue) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_FIND_JMASH_FILE_PAGE:
serviceImpl.findJmashFilePage((jmash.file.protobuf.JmashFileReq) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_FIND_JMASH_FILE_LIST:
serviceImpl.findJmashFileList((jmash.file.protobuf.JmashFileReq) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_FIND_JMASH_FILE_BY_ID:
serviceImpl.findJmashFileById((jmash.file.protobuf.JmashFileKey) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_DELETE_JMASH_FILE:
serviceImpl.deleteJmashFile((jmash.file.protobuf.JmashFileKey) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_BATCH_DELETE_JMASH_FILE:
serviceImpl.batchDeleteJmashFile((jmash.file.protobuf.JmashFileKeyList) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getFindEnumListMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.google.protobuf.StringValue,
jmash.protobuf.EnumValueList>(
service, METHODID_FIND_ENUM_LIST)))
.addMethod(
getFindEnumMapMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.google.protobuf.StringValue,
jmash.protobuf.CustomEnumValueMap>(
service, METHODID_FIND_ENUM_MAP)))
.addMethod(
getFindJmashFilePageMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
jmash.file.protobuf.JmashFileReq,
jmash.file.protobuf.JmashFilePage>(
service, METHODID_FIND_JMASH_FILE_PAGE)))
.addMethod(
getFindJmashFileListMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
jmash.file.protobuf.JmashFileReq,
jmash.file.protobuf.JmashFileList>(
service, METHODID_FIND_JMASH_FILE_LIST)))
.addMethod(
getFindJmashFileByIdMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
jmash.file.protobuf.JmashFileKey,
jmash.file.protobuf.JmashFileModel>(
service, METHODID_FIND_JMASH_FILE_BY_ID)))
.addMethod(
getDeleteJmashFileMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
jmash.file.protobuf.JmashFileKey,
jmash.file.protobuf.JmashFileModel>(
service, METHODID_DELETE_JMASH_FILE)))
.addMethod(
getBatchDeleteJmashFileMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
jmash.file.protobuf.JmashFileKeyList,
com.google.protobuf.Int32Value>(
service, METHODID_BATCH_DELETE_JMASH_FILE)))
.build();
}
private static abstract class FileBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
FileBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return jmash.file.FileProto.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("File");
}
}
private static final class FileFileDescriptorSupplier
extends FileBaseDescriptorSupplier {
FileFileDescriptorSupplier() {}
}
private static final class FileMethodDescriptorSupplier
extends FileBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final java.lang.String methodName;
FileMethodDescriptorSupplier(java.lang.String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (FileGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new FileFileDescriptorSupplier())
.addMethod(getFindEnumListMethod())
.addMethod(getFindEnumMapMethod())
.addMethod(getFindJmashFilePageMethod())
.addMethod(getFindJmashFileListMethod())
.addMethod(getFindJmashFileByIdMethod())
.addMethod(getDeleteJmashFileMethod())
.addMethod(getBatchDeleteJmashFileMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy