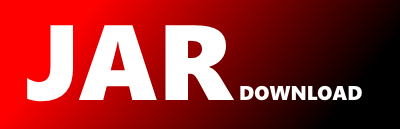
com.gitee.jmash.propertyset.service.PropertySetReadBean Maven / Gradle / Ivy
/*
* Copyright (c) 2002-2003 by OpenSymphony All rights reserved.
*/
package com.gitee.jmash.propertyset.service;
import com.gitee.jmash.core.orm.cdi.JpaStatefulService;
import com.gitee.jmash.core.transaction.JakartaTransaction;
import com.gitee.jmash.propertyset.PropertyException;
import com.gitee.jmash.propertyset.PropertyImplementationException;
import com.gitee.jmash.propertyset.entity.PropertyPK;
import com.gitee.jmash.propertyset.entity.PropertySetEntity;
import com.gitee.jmash.propertyset.enums.PropertyType;
import jakarta.enterprise.inject.Typed;
import jakarta.enterprise.inject.spi.CDI;
import jakarta.persistence.EntityManager;
import jakarta.persistence.PersistenceContext;
import jakarta.persistence.Query;
import jakarta.transaction.Transactional;
import jakarta.transaction.Transactional.TxType;
import jakarta.validation.executable.ValidateOnExecution;
import java.io.StringReader;
import java.util.Collection;
import javax.xml.XMLConstants;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Document;
import org.xml.sax.InputSource;
/**
* EJB3 propertyset implementation. This implementation requires a couple of extra init args:
* manager
: Entity manager to use. transaction
: Can be either JTA or
* RESOURCE_LOCAL. Note that this class can also be deployed as a stateful EJB3 session bean. In
* that case, no configuration is required. It should also not be obtained via PropertySetManager,
* but should instead be looked up in the container. The name the bean is deployed under is
* 'OSPropertySet'. Before any operations are called on the stateful bean, {#setEntityId(Long)} and
* {#setEntityName(String)} must be called.
*
* @author Hani Suleiman Date: Nov 8, 2005 Time: 4:51:53 PM
*/
@Typed(PropertySetRead.class)
@Transactional(TxType.SUPPORTS)
@JpaStatefulService
@ValidateOnExecution
public class PropertySetReadBean extends AbstractPropertySetRead
implements PropertySetRead, JakartaTransaction {
private EntityManager entityManager;
private String entityId;
private String entityName;
@PersistenceContext(unitName = "ReadPropertySet")
public void setEntityManager(EntityManager em) {
this.entityManager = em;
}
public PropertySetReadBean() {}
public PropertySetReadBean(EntityManager entityManager) {
this.entityManager = entityManager;
}
public PropertySetReadBean(EntityManager entityManager, String entityId, String entityName) {
this.entityManager = entityManager;
this.entityId = entityId;
this.entityName = entityName;
}
// ~ Methods
// ////////////////////////////////////////////////////////////////
public void setEntityId(String entityId) {
this.entityId = entityId;
}
public String getEntityId() {
return entityId;
}
public EntityManager getEntityManager() {
return entityManager;
}
public void setEntityName(String entityName) {
this.entityName = entityName;
}
public String getEntityName() {
return entityName;
}
public void clear() {
entityManager.clear();
}
public Collection getKeys(String prefix, PropertyType type) throws PropertyException {
return getKeys(entityName, entityId, prefix, type);
}
@SuppressWarnings("unchecked")
public Collection getKeys(String entityName, String entityId, String prefix,
PropertyType type) throws PropertyException {
Query q;
if ((type.ordinal() == 0) && (prefix == null)) {
q = entityManager.createNamedQuery("os_keys");
}
// all types with the specified prefix
else if ((type.ordinal() == 0) && (prefix != null)) {
q = entityManager.createNamedQuery("os_keys.prefix");
q.setParameter("prefix", prefix + '%');
}
// type and prefix
else if ((prefix == null) && (type.ordinal() != 0)) {
q = entityManager.createNamedQuery("os_keys.type");
q.setParameter("type", type);
} else {
q = entityManager.createNamedQuery("os_keys.prefixAndType");
q.setParameter("prefix", prefix + '%');
q.setParameter("type", type);
}
q.setParameter("entityId", entityId);
q.setParameter("entityName", entityName);
return q.getResultList();
}
public PropertyType getType(String key) throws PropertyException {
return getType(entityName, entityId, key);
}
public PropertyType getType(String entityName, String entityId, String key)
throws PropertyException {
PropertyPK pk = new PropertyPK(entityName, entityId, key);
PropertySetEntity entry = entityManager.find(PropertySetEntity.class, pk);
if (entry == null) {
return PropertyType.ANY;
}
return entry.getType();
}
public boolean exists(String key) throws PropertyException {
return exists(entityName, entityId, key);
}
public boolean exists(String entityName, String entityId, String key) throws PropertyException {
PropertyPK pk = new PropertyPK(entityName, entityId, key);
PropertySetEntity entry = entityManager.find(PropertySetEntity.class, pk);
return entry != null;
}
protected Object get(PropertyType type, String key) throws PropertyException {
return get(entityName, entityId, type, key);
}
protected Object get(String entityName, String entityId, PropertyType type, String key)
throws PropertyException {
PropertyPK pk = new PropertyPK(entityName, entityId, key);
PropertySetEntity entry = entityManager.find(PropertySetEntity.class, pk);
if (entry == null) {
return null;
}
if (entry.getType() != type) {
throw new PropertyException("key '" + key + "' does not have matching type of " + type(type)
+ ", but is of type " + type(entry.getType()));
}
switch (type) {
case BOOLEAN:
return Boolean.valueOf(entry.getBoolValue());
case DOUBLE:
return entry.getDoubleValue();
case STRING:
return entry.getStringValue();
case TEXT:
return entry.getTextValue();
case LONG:
return entry.getLongValue();
case INT:
return entry.getIntValue();
case DATE:
return entry.getDateValue();
case OBJECT:
return entry.getSerialized();
case DATA:
return entry.getData();
case XML:
return readXML(entry.getTextValue());
default:
break;
}
throw new PropertyException("type " + type(type) + " not supported");
}
/**
* Parse XML document from String in byte array.
*/
private Document readXML(String data) {
try {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
factory.setFeature("http://apache.org/xml/features/disallow-doctype-decl", true);
factory.setFeature("http://xml.org/sax/features/external-general-entities", false);
factory.setFeature("http://xml.org/sax/features/external-parameter-entities", false);
factory.setFeature("http://apache.org/xml/features/nonvalidating/load-external-dtd", false);
factory.setFeature(XMLConstants.FEATURE_SECURE_PROCESSING, true);
factory.setXIncludeAware(false);
factory.setExpandEntityReferences(false);
DocumentBuilder builder = factory.newDocumentBuilder();
return builder.parse(new InputSource(new StringReader(data)));
} catch (Exception e) {
throw new PropertyImplementationException(data + "Cannot parse XML data" + e.getMessage(), e);
}
}
public String toString() {
return "PropertySetReadBean#" + hashCode() + "{entityManager=" + entityManager + ", entityId="
+ entityId + ", entityName='" + entityName + '\'' + '}';
}
@Override
public void close() throws Exception {
CDI.current().destroy(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy