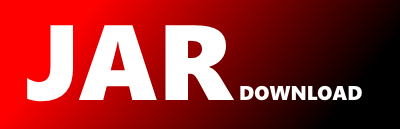
com.gitee.jmash.propertyset.service.PropertySetWriteBean Maven / Gradle / Ivy
/*
* Copyright (c) 2002-2003 by OpenSymphony
* All rights reserved.
*/
package com.gitee.jmash.propertyset.service;
import com.gitee.jmash.common.utils.Data;
import com.gitee.jmash.core.orm.cdi.JpaStatefulService;
import com.gitee.jmash.core.transaction.JakartaTransaction;
import com.gitee.jmash.propertyset.PropertyException;
import com.gitee.jmash.propertyset.PropertyImplementationException;
import com.gitee.jmash.propertyset.entity.PropertyPK;
import com.gitee.jmash.propertyset.entity.PropertySetEntity;
import com.gitee.jmash.propertyset.enums.PropertyType;
import jakarta.enterprise.context.Dependent;
import jakarta.enterprise.inject.Typed;
import jakarta.enterprise.inject.spi.CDI;
import jakarta.persistence.EntityManager;
import jakarta.persistence.PersistenceContext;
import jakarta.persistence.Query;
import jakarta.persistence.spi.PersistenceUnitTransactionType;
import jakarta.transaction.Transactional;
import jakarta.transaction.Transactional.TxType;
import jakarta.validation.executable.ValidateOnExecution;
import java.io.Serializable;
import java.io.StringWriter;
import java.util.Collection;
import java.util.Date;
import java.util.List;
import java.util.Properties;
import javax.xml.transform.Result;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerConfigurationException;
import javax.xml.transform.TransformerException;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.TransformerFactoryConfigurationError;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import org.w3c.dom.Document;
/**
* EJB3 propertyset implementation. This implementation requires a couple of
* extra init args:
* manager
: Entity manager to use.
* transaction
: Can be either JTA or RESOURCE_LOCAL.
* Note that this class can also be deployed as a stateful EJB3 session bean. In
* that case, no configuration is required. It should also not be obtained via
* PropertySetManager, but should instead be looked up in the container. The
* name the bean is deployed under is 'OSPropertySet'. Before any operations are
* called on the stateful bean, {#setEntityId(Long)} and
* {#setEntityName(String)} must be called.
*
* @author Hani Suleiman Date: Nov 8, 2005 Time: 4:51:53 PM
*/
@Typed(PropertySetWrite.class)
@Transactional(TxType.REQUIRED)
@JpaStatefulService
@ValidateOnExecution
@Dependent
public class PropertySetWriteBean extends AbstractPropertySetWrite
implements PropertySetWrite, JakartaTransaction {
private EntityManager entityManager;
private String entityId;
private PersistenceUnitTransactionType transactionType;
private String entityName;
@PersistenceContext(unitName = "WritePropertySet")
public void setEntityManager(EntityManager em) {
this.entityManager = em;
}
public PropertySetWriteBean() {
}
public PropertySetWriteBean(EntityManager entityManager,
PersistenceUnitTransactionType transactionType) {
this.entityManager = entityManager;
this.transactionType = transactionType;
}
public PropertySetWriteBean(EntityManager entityManager,
PersistenceUnitTransactionType transactionType, String entityId, String entityName) {
this.entityManager = entityManager;
this.entityId = entityId;
this.entityName = entityName;
this.transactionType = transactionType;
}
// ~ Methods
// ////////////////////////////////////////////////////////////////
public void setEntityId(String entityId) {
this.entityId = entityId;
}
public String getEntityId() {
return entityId;
}
public EntityManager getEntityManager() {
return entityManager;
}
public void setEntityName(String entityName) {
this.entityName = entityName;
}
public String getEntityName() {
return entityName;
}
public void clear() {
entityManager.clear();
}
public void setTransactionType(PersistenceUnitTransactionType transactionType) {
this.transactionType = transactionType;
}
public PersistenceUnitTransactionType getTransactionType() {
return transactionType;
}
public void remove(String key) throws PropertyException {
remove(entityName, entityId, key);
}
public void remove() throws PropertyException {
remove(entityName, entityId);
}
public void remove(String entityName, String entityId, String key) throws PropertyException {
PropertyPK pk = new PropertyPK(entityName, entityId, key);
PropertySetEntity entry = entityManager.find(PropertySetEntity.class, pk);
if (entry != null) {
entityManager.remove(entry);
}
}
@SuppressWarnings("unchecked")
public void remove(String entityName, String entityId) throws PropertyException {
Query q = entityManager.createNamedQuery("os_entries");
q.setParameter("entityId", entityId);
q.setParameter("entityName", entityName);
// idiot jalopy blows up on a real man's for loop, so we have to use
// jdk14 wanky version
List l = q.getResultList();
for (PropertySetEntity o : l) {
entityManager.remove(o);
}
}
public boolean supportsType(PropertyType type) {
if (type == PropertyType.PROPERTIES) {
return false;
}
return true;
}
protected void setImpl(PropertyType type, String key, Object value) throws PropertyException {
setImpl(entityName, entityId, type, key, value);
}
protected void setImpl(String entityName, String entityId, PropertyType type, String key,
Object value) throws PropertyException {
PropertyPK pk = new PropertyPK(entityName, entityId, key);
PropertySetEntity item;
item = entityManager.find(PropertySetEntity.class, pk);
if (item == null) {
item = new PropertySetEntity();
item.setPrimaryKey(pk);
item.setType(type);
} else if (item.getType() != type) {
throw new PropertyException(
"Existing key '" + key + "' does not have matching type of " + type);
}
switch (type) {
case BOOLEAN:
item.setBoolValue(((Boolean) value).booleanValue());
break;
case INT:
item.setIntValue(((Number) value).intValue());
break;
case LONG:
item.setLongValue(((Number) value).longValue());
break;
case DOUBLE:
item.setDoubleValue(((Number) value).doubleValue());
break;
case STRING:
item.setStringValue((String) value);
break;
case TEXT:
item.setTextValue((String) value);
break;
case DATE:
item.setDateValue((Date) value);
case OBJECT:
item.setSerialized((Serializable) value);
break;
case DATA:
if (value instanceof Data) {
item.setData(((Data) value).getBytes());
} else {
item.setData((byte[]) value);
}
break;
case XML:
String text = writeXML((Document) value);
item.setTextValue(text);
break;
default:
throw new PropertyException("type " + type + " not supported");
}
entityManager.merge(item);
}
public Collection getKeys(String prefix, PropertyType type) throws PropertyException {
return getKeys(entityName, entityId, prefix, type);
}
@SuppressWarnings("unchecked")
public Collection getKeys(String entityName, String entityId, String prefix,
PropertyType type) throws PropertyException {
Query q;
if ((type.ordinal() == 0) && (prefix == null)) {
q = entityManager.createNamedQuery("os_keys");
}
// all types with the specified prefix
else if ((type.ordinal() == 0) && (prefix != null)) {
q = entityManager.createNamedQuery("os_keys.prefix");
q.setParameter("prefix", prefix + '%');
}
// type and prefix
else if ((prefix == null) && (type.ordinal() != 0)) {
q = entityManager.createNamedQuery("os_keys.type");
q.setParameter("type", type);
} else {
q = entityManager.createNamedQuery("os_keys.prefixAndType");
q.setParameter("prefix", prefix + '%');
q.setParameter("type", type);
}
q.setParameter("entityId", entityId);
q.setParameter("entityName", entityName);
return q.getResultList();
}
public void setAsActualType(String key, Object value) throws PropertyException {
super.setAsActualType(key, value);
}
public void setBoolean(String key, boolean value) {
super.setBoolean(key, value);
}
public void setDate(String key, Date value) {
super.setDate(key, value);
}
public void setData(String key, byte[] value) {
super.setData(key, value);
}
public void setDouble(String key, double value) {
super.setDouble(key, value);
}
public void setInt(String key, int value) {
super.setInt(key, value);
}
public void setLong(String key, long value) {
super.setLong(key, value);
}
public void setObject(String key, Object value) {
super.setObject(key, value);
}
public void setProperties(String key, Properties value) {
super.setProperties(key, value);
}
public void setText(String key, String value) {
super.setText(key, value);
}
public void setString(String key, String value) {
super.setString(key, value);
}
public void setXML(String key, Document value) {
super.setXML(key, value);
}
/**
* Serialize (print) XML document to byte array (as String).
*/
private String writeXML(Document doc) {
try {
DOMSource source = new DOMSource(doc);
StringWriter writer = new StringWriter();
Result result = new StreamResult(writer);
Transformer transformer = TransformerFactory.newInstance().newTransformer();
transformer.transform(source, result);
// log.debug();
return writer.getBuffer().toString();
} catch (TransformerConfigurationException e) {
throw new PropertyImplementationException("Cannot serialize XML" + e.getMessage(), e);
} catch (TransformerFactoryConfigurationError e) {
throw new PropertyImplementationException("Cannot serialize XML" + e.getMessage(), e);
} catch (TransformerException e) {
throw new PropertyImplementationException("Cannot serialize XML" + e.getMessage(), e);
}
}
public String toString() {
return "PropertySetWriteBean#" + hashCode() + "{entityManager=" + entityManager + ", entityId="
+ entityId + ", entityName='" + entityName + '\'' + '}';
}
@Override
public void close() throws Exception {
CDI.current().destroy(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy