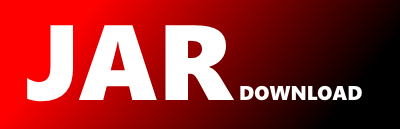
com.gitee.jmash.propertyset.entity.PropertySetEntity Maven / Gradle / Ivy
The newest version!
package com.gitee.jmash.propertyset.entity;
import com.gitee.jmash.propertyset.enums.PropertyType;
import jakarta.persistence.Cacheable;
import jakarta.persistence.Column;
import jakarta.persistence.EmbeddedId;
import jakarta.persistence.Entity;
import jakarta.persistence.Lob;
import jakarta.persistence.NamedQueries;
import jakarta.persistence.NamedQuery;
import jakarta.persistence.Table;
import jakarta.persistence.Transient;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.Serializable;
import java.time.LocalDateTime;
import java.util.Date;
/**
* @author Hani Suleiman Date: Nov 8, 2005 Time: 4:57:44 PM
*/
@Entity
@Table(name = "os_properties")
@NamedQueries({
@NamedQuery(name = "os_entries", query = "select p from PropertySetEntity p where p.primaryKey.entityName=:entityName and p.primaryKey.entityId=:entityId"),
@NamedQuery(name = "os_keys", query = "select p.primaryKey.key from PropertySetEntity p where p.primaryKey.entityName=:entityName and p.primaryKey.entityId=:entityId"),
@NamedQuery(name = "os_keys.prefix", query = "select p.primaryKey.key from PropertySetEntity p where p.primaryKey.entityName=:entityName and p.primaryKey.entityId=:entityId and p.primaryKey.key like :prefix"),
@NamedQuery(name = "os_keys.type", query = "select p.primaryKey.key from PropertySetEntity p where p.primaryKey.entityName=:entityName and p.primaryKey.entityId=:entityId and p.type=:type"),
@NamedQuery(name = "os_keys.prefixAndType", query = "select p.primaryKey.key from PropertySetEntity p where p.primaryKey.entityName=:entityName and p.primaryKey.entityId=:entityId and p.type=:type and p.primaryKey.key like :prefix") })
@Cacheable
public class PropertySetEntity {
private PropertyPK primaryKey;
private boolean boolValue;
private int intValue;
private long longValue;
private double doubleValue;
private String stringValue;
private String textValue;
private Date dateValue;
private PropertyType type;
private byte[] data;
private transient Serializable serialized;
private byte[] objectData;
private LocalDateTime createTime=LocalDateTime.now();
private LocalDateTime updateTime=LocalDateTime.now();
@EmbeddedId
public PropertyPK getPrimaryKey() {
return primaryKey;
}
public void setPrimaryKey(PropertyPK primaryKey) {
this.primaryKey = primaryKey;
}
@Column(name = "bool_value")
public boolean getBoolValue() {
return boolValue;
}
public void setBoolValue(boolean boolValue) {
this.boolValue = boolValue;
}
@Transient
public Serializable getSerialized() {
if (serialized == null) {
try {
serialized = (Serializable) deserialize(getObjectData());
} catch (Exception e) {
// can't happen hopefully
throw new RuntimeException("Error deserializing object", e);
}
}
return serialized;
}
public void setSerialized(Serializable serialized) {
this.serialized = serialized;
setObjectData(getSerialized(serialized));
}
@Column(name = "int_value")
public int getIntValue() {
return intValue;
}
public void setIntValue(int intValue) {
this.intValue = intValue;
}
@Column(name = "long_value")
public long getLongValue() {
return longValue;
}
public void setLongValue(long longValue) {
this.longValue = longValue;
}
@Column(name = "double_value")
public double getDoubleValue() {
return doubleValue;
}
public void setDoubleValue(double doubleValue) {
this.doubleValue = doubleValue;
}
@Column(name = "string_value")
public String getStringValue() {
return stringValue;
}
public void setStringValue(String stringValue) {
this.stringValue = stringValue;
}
@Lob
@Column(name = "text_value")
public String getTextValue() {
return textValue;
}
public void setTextValue(String textValue) {
this.textValue = textValue;
}
@Column(name = "date_value")
public Date getDateValue() {
return dateValue;
}
public void setDateValue(Date dateValue) {
this.dateValue = dateValue;
}
@Column(name = "type_")
public PropertyType getType() {
return type;
}
public void setType(PropertyType type) {
this.type = type;
}
@Lob
@Column(name = "data_")
public byte[] getData() {
return data;
}
public void setData(byte[] data) {
this.data = data;
}
@Lob
@Column(name = "object_data")
public byte[] getObjectData() {
return objectData;
}
public void setObjectData(byte[] objectData) {
this.objectData = objectData;
}
@Column(name = "create_time")
public LocalDateTime getCreateTime() {
return createTime;
}
public void setCreateTime(LocalDateTime createTime) {
this.createTime = createTime;
}
@Column(name = "update_time")
public LocalDateTime getUpdateTime() {
return updateTime;
}
public void setUpdateTime(LocalDateTime updateTime) {
this.updateTime = updateTime;
}
private static byte[] getSerialized(Object object) {
ByteArrayOutputStream byteOut = new ByteArrayOutputStream();
try {
ObjectOutputStream out = new ObjectOutputStream(byteOut);
out.writeObject(object);
out.flush();
out.close();
} catch (IOException e) {
throw new RuntimeException("Error serializing " + object, e);
}
return byteOut.toByteArray();
}
private static Object deserialize(byte[] data) throws IOException, ClassNotFoundException {
ObjectInputStream in = new ObjectInputStream(new ByteArrayInputStream(data));
return in.readObject();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy