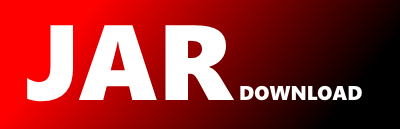
com.gitee.jmash.propertyset.service.AbstractPropertySetRead Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2002-2003 by OpenSymphony
* All rights reserved.
*/
package com.gitee.jmash.propertyset.service;
import com.gitee.jmash.common.utils.Data;
import com.gitee.jmash.propertyset.PropertyException;
import com.gitee.jmash.propertyset.enums.PropertyType;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Date;
import java.util.Iterator;
import java.util.Properties;
import org.w3c.dom.Document;
/**
* Base implementation of PropertySet.
*
*
* Performs necessary casting for get???/set??? methods which wrap around the
* following 2 methods which are declared protected abstract
and
* need to be implemented by subclasses:
*
*
*
* - {#get(int,java.lang.String)}
* - {#setImpl(int,java.lang.String,java.lang.Object)}
*
*
*
* The following methods are declared public abstract
and are the
* remainder of the methods that need to be implemented at the very least:
*
*
*
* - {#exists(java.lang.String)}
* - {#remove(java.lang.String)}
* - {#getType(java.lang.String)}
* - {#getKeys(java.lang.String,int)}
*
*
*
* The supports???
methods are implemented and all return true by
* default. Override if necessary.
*
*
* @author Joe Walnes
* @author Hani Suleiman
* @version $Revision: 151 $
*/
public abstract class AbstractPropertySetRead implements PropertySetRead {
public Object getAsActualType(String key) throws PropertyException {
PropertyType type = getType(key);
Object value = null;
switch (type) {
case BOOLEAN:
value = Boolean.valueOf(getBoolean(key));
break;
case INT:
value = Integer.valueOf(getInt(key));
break;
case LONG:
value = Long.valueOf(getLong(key));
break;
case DOUBLE:
value = Double.valueOf(getDouble(key));
break;
case STRING:
value = getString(key);
break;
case TEXT:
value = getText(key);
break;
case DATE:
value = getDate(key);
break;
case XML:
value = getXML(key);
break;
case DATA:
value = getData(key);
break;
case PROPERTIES:
value = getProperties(key);
break;
case OBJECT:
value = getObject(key);
break;
default:
break;
}
return value;
}
public boolean getBoolean(String key) {
try {
return ((Boolean) get(PropertyType.BOOLEAN, key)).booleanValue();
} catch (NullPointerException e) {
return false;
}
}
/**
* Casts to {com.opensymphony.util.Data} and returns bytes.
*/
public byte[] getData(String key) {
try {
Object data = get(PropertyType.DATA, key);
if (data instanceof Data) {
return ((Data) data).getBytes();
} else if (data instanceof byte[]) {
return (byte[]) data;
}
} catch (NullPointerException e) {
return null;
}
return null;
}
public Date getDate(String key) {
try {
return (Date) get(PropertyType.DATE, key);
} catch (NullPointerException e) {
return null;
}
}
public double getDouble(String key) {
try {
return ((Double) get(PropertyType.DOUBLE, key)).doubleValue();
} catch (NullPointerException e) {
return 0.0;
}
}
public int getInt(String key) {
try {
return ((Integer) get(PropertyType.INT, key)).intValue();
} catch (NullPointerException e) {
return 0;
}
}
/**
* 获取所有的Key值集合
*
* @return
* @throws PropertyException
*/
public Collection getKeys() throws PropertyException {
return getKeys(null, PropertyType.ANY);
}
/**
* 获取Key值集合,指定PropertyType
*
* @return
* @throws PropertyException
*/
public Collection getKeys(PropertyType type) throws PropertyException {
return getKeys(null, type);
}
/**
* 获取Key值集合,指定prefix
*
* @return
* @throws PropertyException
*/
public Collection getKeys(String prefix) throws PropertyException {
return getKeys(prefix, PropertyType.ANY);
}
public long getLong(String key) {
try {
return ((Long) get(PropertyType.LONG, key)).longValue();
} catch (NullPointerException e) {
return 0L;
}
}
public Object getObject(String key) {
try {
return get(PropertyType.OBJECT, key);
} catch (NullPointerException e) {
return null;
}
}
public Properties getProperties(String key) {
try {
return (Properties) get(PropertyType.PROPERTIES, key);
} catch (NullPointerException e) {
return null;
}
}
public String getString(String key) {
try {
return (String) get(PropertyType.STRING, key);
} catch (NullPointerException e) {
return null;
}
}
public String getText(String key) {
try {
return (String) get(PropertyType.TEXT, key);
} catch (NullPointerException e) {
return null;
}
}
public Document getXML(String key) {
try {
return (Document) get(PropertyType.XML, key);
} catch (NullPointerException e) {
return null;
}
}
/**
* Simple human readable representation of contents of PropertySet.
*/
@SuppressWarnings("rawtypes")
public String toString() {
StringBuffer result = new StringBuffer();
result.append(getClass().getName());
result.append(" {\n");
try {
Iterator keys = getKeys().iterator();
while (keys.hasNext()) {
String key = (String) keys.next();
PropertyType type = getType(key);
if (type.ordinal() > 0) {
result.append('\t');
result.append(key);
result.append(" = ");
result.append(get(type, key));
result.append('\n');
}
}
} catch (PropertyException e) {
// toString should never throw an exception.
}
result.append("}\n");
return result.toString();
}
protected abstract Object get(PropertyType type, String key) throws PropertyException;
protected String type(PropertyType type) {
if (type == null) {
return null;
} else {
return type.name().toLowerCase();
}
}
protected PropertyType type(String type) {
if (type == null) {
return PropertyType.ANY;
}
try {
type = type.toUpperCase();
return PropertyType.valueOf(type);
} catch (java.lang.IllegalArgumentException ex) {
return PropertyType.ANY;
}
}
/*
* (non-Javadoc)
*
* @see
* gcloud.propertyset.PropertySet#getMultiValueAsActualType(java.lang.String)
*/
public Object[] getMultiValueAsActualType(String key) throws PropertyException {
String p = String.format("%s_%s", key, MULTI_VALUE);
Collection keys = this.getKeys(p);
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy