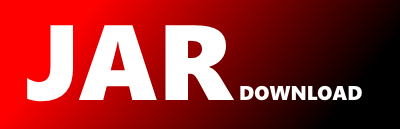
cn.yangjunda.util.XMLReader Maven / Gradle / Ivy
package cn.yangjunda.util;
import org.dom4j.Attribute;
import org.dom4j.Document;
import org.dom4j.DocumentException;
import org.dom4j.Element;
import org.dom4j.io.SAXReader;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* Created by juanda on 12/9/17.
*/
public class XMLReader {
private Configuration configuration = new Configuration();
private Set> classes;
/**
* 读取Bean配置文件
* @param
* @return
*/
private Element element;
@SuppressWarnings("unchecked")
public void readXML() {
Document document = null;
SAXReader saxReader = new SAXReader();
try {
ClassLoader classLoader =
Thread.currentThread().getContextClassLoader();
document = saxReader.read(classLoader.getResourceAsStream("juanda-mvc.xml"));
Element beans = document.getRootElement();
if("beans".equals(beans.getName())){
getBeans(beans);
}
} catch (DocumentException e) {
// log.info("读取配置文件出错....");
}
}
private void getBeans(Element node){
System.out.println("--------------------");
if ("context".equals(node.getName())){
List listAttr=node.attributes();//当前节点的所有属性的list
for(Attribute attr:listAttr){//遍历当前节点的所有属性
if("base-package".equals(attr.getName())){
configuration.setPackagePath(attr.getValue());
}else if("autowire-open".equals(attr.getName())){
try {
configuration.setAutowire(Boolean.valueOf(attr.getValue()));
}catch (Exception e){
configuration.setAutowire(true);
}
}
}
}else if("bean".equals(node.getName())){
Map map = configuration.getBeans();
List listAttr=node.attributes();//当前节点的所有属性的list
String id = null;
String className = null;
for(Attribute attr:listAttr){//遍历当前节点的所有属性
if(".*".equals(attr.getValue().substring(attr.getValue().length()-2))){
classes = ClassTools.getClasses(attr.getValue().substring(0,attr.getValue().length()-2));
for (Class> clasz : classes) {
map.put(clasz.getName(),firstLowerName(clasz.getName().substring((clasz.getName().lastIndexOf(".") + 1), clasz.getName().length())));
}
configuration.setBeans(map);
}else {
if("id".equals(attr.getName())){
id=attr.getValue();
}else if("class".equals(attr.getName())){
className = attr.getValue();
}
if(StringUtils.isNotBlank(id)&&StringUtils.isNotBlank(className)){
map.put(className,id);
configuration.setBeans(map);
}
}
}
}
//递归遍历当前节点所有的子节点
List listElement=node.elements();//所有一级子节点的list
for(Element e:listElement){//遍历所有一级子节点
getBeans(e);//递归
}
}
public Configuration getConfiguration() {
return configuration;
}
public void setConfiguration(Configuration configuration) {
this.configuration = configuration;
}
public Element getElement() {
return element;
}
public void setElement(Element element) {
this.element = element;
}
private String firstLowerName(String name) {
name = name.substring(0, 1).toLowerCase() + name.substring(1);
return name;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy