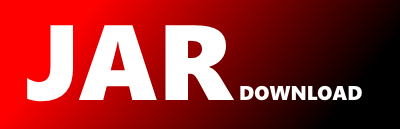
net.gdface.cassdk.BaseCassdk Maven / Gradle / Ivy
package net.gdface.cassdk;
import java.awt.Rectangle;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.atomic.AtomicBoolean;
import net.gdface.image.ImageErrorException;
import net.gdface.image.LazyImage;
import net.gdface.image.UnsupportedFormatException;
import net.gdface.sdk.CodeInfo;
import net.gdface.sdk.FRect;
import net.gdface.sdk.BaseFaceApiLocal;
import net.gdface.sdk.NotFaceDetectedException;
import net.gdface.sdk.RectUtils;
import net.gdface.utils.Assert;
/**
* CASSDK接口抽象类
*
* @author guyadong
*
*/
public abstract class BaseCassdk extends BaseFaceApiLocal {
protected BaseCassdk() {
}
/**
* 检测人脸
* @param imgRGB
* @param width
* @param height
* @param faceInfo
*/
abstract protected void nativeDetectFace(byte[] imgRGB,int width, int height,List faceInfo);
/**
* 提取特征
* @param imgMatrix
* @param width
* @param height
* @param faceInfo
* @return
*/
abstract protected byte[] nativeGetFaceFeature(byte[] imgMatrix,int width, int height,CodeInfo faceInfo);
/**
* 根据检测到的人脸位置{@code facePos},提取一组特征
* @param imgMatrix
* @param width
* @param height
* @param facePos
* @return
*/
abstract protected byte[][] nativeGetFaceFeatures(byte[] imgMatrix,int width, int height,List facePos);
/**
* 检测并提取特征
* @param imgMatrix
* @param width
* @param height
* @return
*/
abstract protected CodeInfo[] nativeDetectAndGetFeatures(byte[] imgMatrix,int width, int height);
@Override
public List detectFace(LazyImage lazyImg, final FRect detectRectangle)
throws UnsupportedFormatException {
concurrentLock.lock();
try {
Assert.notNull(lazyImg, "bufImg");
// detectRectangle为空时,全图提取特征码
Rectangle imgRect = lazyImg.getRectangle();
Rectangle dr;
if (FRect.isNull(detectRectangle)){
dr = imgRect;
}else{
// 如果指定的检测范围范围超出图像尺寸,则抛出异常
RectUtils.assertContains(imgRect, "imgRect", dr = RectUtils.newRectangle(detectRectangle),
"detectRectangle");
}
List faceInfo = new ArrayList();
if (dr.width > 0 && dr.height > 0) {
final byte[] imgRGB =getMatrixData(lazyImg, RectUtils.newFRect(dr));
nativeDetectFace(imgRGB,dr.width, dr.height,faceInfo);
if (!RectUtils.isZeroPoint(dr)) {
// 坐标系转换
for (CodeInfo ci : faceInfo) {
RectUtils.translate(ci, dr.x, dr.y);
}
}
}
return faceInfo;
} finally {
concurrentLock.unlock();
}
}
@Override
protected CodeInfo getCodeFromImageMatrix(final byte[] imgMatrix, final int width, final int height,
CodeInfo faceInfo, final AtomicBoolean hasFail) {
Assert.notEmpty(imgMatrix, "imgMatrix");
if (width <= 0 || height <= 0){
throw new IllegalArgumentException(String.format("INVALID VALUE:the imgMatrix's size(%dW%dH) ", width,
height));
}
Assert.notNull(faceInfo, "faceInfo");
byte[] code = nativeGetFaceFeature(imgMatrix, width, height,faceInfo);
if (null==code && null != hasFail){
hasFail.set(true);
}
faceInfo.setCode(code);
return faceInfo;
}
@Override
protected byte[] getMatrixData(LazyImage lazyImg, FRect rect) throws UnsupportedFormatException {
return lazyImg.getMatrixBGR(
FRect.isNull(rect)
? null
: RectUtils.newRectangle(rect));
}
@Override
public List getCodeInfo(LazyImage lazyImg, int faceNum, List facePos) throws NotFaceDetectedException {
concurrentLock.lock();
// 检测到的人脸数目
int faceCount = 0;
// 提取到特征码的人脸数目
int featureCount=0;
final AtomicBoolean hasFail = new AtomicBoolean(false);
try {
Assert.notNull(lazyImg, "lazyImg");
// facePos为null或空时抛出异常
Assert.notEmpty(facePos, "facePos");
// 人脸位置对象数目小于要求提取的特征码的数目,则抛出异常
if (facePos.size() < faceNum){
throw new NotFaceDetectedException(facePos.size(), 0);
}
byte[] imgData=getMatrixData(lazyImg,null);
byte[][] features = nativeGetFaceFeatures(imgData, lazyImg.getWidth(), lazyImg.getHeight(),facePos);
for(int i = 0 ;i 0)) {
if (featureCount != faceNum){
throw new NotFaceDetectedException(faceCount, featureCount);
}
} else if (0 == featureCount){
throw new NotFaceDetectedException(faceCount, 0);
}
for(int i=0;i0&&faceNum!=codes.length){
throw NOTFACEDETECTED_INSTANCE_DEFAULT;
}
return codes;
}finally{
concurrentLock.unlock();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy