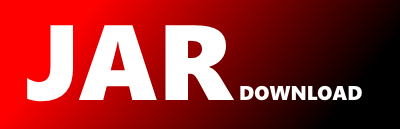
net.gdface.codegen.generator.GeneratorConfiguration Maven / Gradle / Ivy
package net.gdface.codegen.generator;
import static com.google.common.base.Preconditions.checkNotNull;
import java.io.File;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.Options;
import org.apache.commons.cli.ParseException;
import com.google.common.collect.Maps;
import com.google.common.collect.Sets;
import net.gdface.cli.AbstractConfiguration;
import net.gdface.cli.Context;
import net.gdface.codegen.CreateInterfaceSourceConstants;
import net.gdface.codegen.DuplicatedConstants;
import net.gdface.utils.MiscellaneousUtils;
import net.gdface.utils.SimpleLog;
/**
* 代码生成器通用参数对象
* @author guyadong
*
*/
public class GeneratorConfiguration extends AbstractConfiguration implements CreateInterfaceSourceConstants,
DuplicatedConstants {
public static final String DEFAULT_OUT = ".";
public static final String DEFAULT_ROOT = "./templates";
public static final String DEFAULT_FOLDER = ".";
public static final String DEFAULT_INCLUDE = "includes";
public static final String DEFAULT_LOADER= "class";
private File outputLocation;
private String templateRoot;
private String templateFolder;
private String includeFolder;
private Set excludeVms;
private Set includeVms;
private String sourcePackage;
private String resourceLoaderType;
protected final Context defaultValue = Context.builder()
.addProperty(OUTPUT_LOCATION_OPTION_LONG, new File(DEFAULT_OUT))
.addProperty(TEMPLATE_ROOT_OPTION_LONG, DEFAULT_ROOT)
.addProperty(TEMPLATE_FOLDER_OPTION_LONG, DEFAULT_FOLDER)
.addProperty(TEMPLATE_INCLUDE_OPTION_LONG, DEFAULT_INCLUDE)
.addProperty(EXCLUDE_VMS_OPTION_LONG, "")
.addProperty(INCLUDE_VMS_OPTION_LONG, "")
.addProperty(RESOURCE_LOADER_OPTION_LONG, DEFAULT_LOADER)
.build();
@Override
protected Map getDefaultValueMap() {
return defaultValue.getContext();
}
@Override
public void loadConfig(Options options, CommandLine cmd) throws ParseException {
super.loadConfig(options, cmd);
outputLocation = getProperty(OUTPUT_LOCATION_OPTION_LONG);
templateRoot = getProperty(TEMPLATE_ROOT_OPTION_LONG);
templateFolder = getProperty(TEMPLATE_FOLDER_OPTION_LONG);
includeFolder = getProperty(TEMPLATE_INCLUDE_OPTION_LONG);
String evms = getProperty(EXCLUDE_VMS_OPTION_LONG);
excludeVms = Sets.newHashSet(MiscellaneousUtils.elementsOf(evms));
String ivms = getProperty(INCLUDE_VMS_OPTION_LONG);
includeVms = Sets.newHashSet(MiscellaneousUtils.elementsOf(ivms));
sourcePackage = getProperty(PACKAGE_OPTION_LONG);
resourceLoaderType = getProperty(RESOURCE_LOADER_OPTION_LONG);
}
/**
* @return outputLocation
*/
public File getOutputLocation() {
return outputLocation;
}
/**
* @return templateRoot
*/
public String getTemplateRoot() {
return templateRoot;
}
/**
* @return templateFolder
*/
public String getTemplateFolder() {
return templateFolder;
}
/**
* @return includeFolder
*/
public String getIncludeFolder() {
return includeFolder;
}
/**
* @return excludeVms
*/
public Set getExcludeVms() {
return excludeVms;
}
/**
* @return includeVms
*/
public Set getIncludeVms() {
return includeVms;
}
/**
* @return sourcePackage
*/
public String getSourcePackage() {
return sourcePackage;
}
/**
* @return defaultValue
*/
public Context getDefaultValue() {
return defaultValue;
}
/**
* @param templateFolder 要设置的 templateFolder
*/
public void setTemplateFolder(String templateFolder) {
this.templateFolder = templateFolder;
}
public String getResourceLoaderType() {
return resourceLoaderType;
}
public boolean useClasspathResourceLoader(){
return DEFAULT_LOADER.equals(getResourceLoaderType());
}
public List getListProperty(String name){
return MiscellaneousUtils.elementsOf((String)getProperty(name));
}
public HashSet getSetProperty(String name){
return Sets.newHashSet(MiscellaneousUtils.elementsOf((String)getProperty(name)));
}
public void readClassEntry(String propertyName, Map, List> input) {
String[] entries = getProperty(propertyName);
if(entries != null){
for(String str : entries){
String[] entry = str.split(":");
try {
List names = MiscellaneousUtils.elementsOf(entry[1]);
if(!names.isEmpty()){
checkNotNull(input,"input is null").put(Class.forName(entry[0],false,null),names);
}
} catch (ClassNotFoundException e) {
SimpleLog.log(e.toString());
}
}
}
}
public Map, List> getClassEntry(String propertyName) {
Map, List> input = Maps.newHashMap();
readClassEntry(propertyName,input);
return input;
}
/**
* @return excludeMethods
*/
public Map, List> getExcludeMethods() {
return Collections.emptyMap();
}
/**
* @return includeMethods
*/
public Map, List> getIncludeMethods() {
return Collections.emptyMap();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy