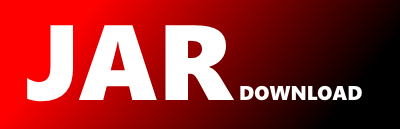
net.gdface.thrift.EnumThriftCodec Maven / Gradle / Ivy
The newest version!
package net.gdface.thrift;
import com.facebook.swift.codec.ThriftCodec;
import com.facebook.swift.codec.internal.UnknownEnumValueException;
import com.facebook.swift.codec.metadata.ThriftCatalogWithTransformer;
import com.facebook.swift.codec.metadata.ThriftEnumMetadata;
import com.facebook.swift.codec.metadata.ThriftType;
import com.google.common.base.Preconditions;
import org.apache.thrift.protocol.TProtocol;
import javax.annotation.concurrent.Immutable;
/**
* 支持负值的枚举类型编解码器
* 在默认枚举类型编解码器{@link com.facebook.swift.codec.internal.EnumThriftCodec}基础上删除非负值判断
*/
@Immutable
public class EnumThriftCodec> implements ThriftCodec
{
private final ThriftType type;
private final ThriftEnumMetadata enumMetadata;
@SuppressWarnings("unchecked")
public EnumThriftCodec(ThriftType type)
{
this.type = type;
enumMetadata = (ThriftEnumMetadata) type.getEnumMetadata();
}
@Override
public ThriftType getType()
{
return type;
}
@Override
public T read(TProtocol protocol)
throws Exception
{
int enumValue = protocol.readI32();
if (enumMetadata.hasExplicitThriftValue()) {
T enumConstant = enumMetadata.getByEnumValue().get(enumValue);
if (enumConstant != null) {
return enumConstant;
}
}
else {
T[] enumConstants = enumMetadata.getEnumClass().getEnumConstants();
if (enumValue < enumConstants.length) {
return enumConstants[enumValue];
}
}
// unknown, throw unknown value exception
throw new UnknownEnumValueException(
String.format(
"Enum %s does not have a value for %s",
enumMetadata.getEnumClass(),
enumValue
)
);
}
@Override
public void write(T enumConstant, TProtocol protocol)
throws Exception
{
Preconditions.checkNotNull(enumConstant, "enumConstant is null");
int enumValue;
if (enumMetadata.hasExplicitThriftValue()) {
enumValue = enumMetadata.getByEnumConstant().get(enumConstant);
}
else {
enumValue = enumConstant.ordinal();
}
protocol.writeI32(enumValue);
}
/**
* 生成指定枚举类型的编解码器
* @param type
* @return EnumThriftCodec 实例
*/
public static > EnumThriftCodec getCodec(Class type){
ThriftType thriftType = ThriftCatalogWithTransformer.CATALOG.getThriftType(type);
return new EnumThriftCodec(thriftType);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy