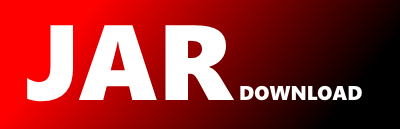
gu.dtalk.CheckOption Maven / Gradle / Ivy
package gu.dtalk;
import java.io.ByteArrayOutputStream;
import java.io.PrintStream;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import com.alibaba.fastjson.TypeReference;
import com.alibaba.fastjson.annotation.JSONField;
import com.google.common.base.Function;
import com.google.common.base.MoreObjects;
import com.google.common.base.Predicate;
import com.google.common.base.Predicates;
import com.google.common.collect.Iterables;
import com.google.common.collect.Lists;
import com.google.common.collect.Sets;
import static com.google.common.base.Preconditions.*;
/**
* 多选列表选项
* @author guyadong
*
* @param OPTION数据类型
*/
public class CheckOption extends BaseOption> {
@JSONField(serialize = false,deserialize = false)
private final Predicate> checkValidator = new Predicate>() {
@Override
public boolean apply(Set set) {
boolean findInvalid = Iterables.tryFind(set, new Predicate() {
@Override
public boolean apply(Integer index) {
return index < 0 || index >= options.size();
}
}).isPresent();
return !findInvalid;
}
};
protected final LinkedHashMap options = new LinkedHashMap<>();
public CheckOption() {
super(new TypeReference>() {}.getType());
super.setValidator(checkValidator);
}
public CheckOption addOption(E opt,String desc){
options.put(checkNotNull(opt), MoreObjects.firstNonNull(desc, ""));
return this;
}
@Override
public OptionType getType() {
return OptionType.MULTICHECK;
}
@Override
public BaseOption> setValidator(Predicate> validator) {
return super.setValidator(Predicates.and(checkValidator, validator));
}
@JSONField(serialize = false,deserialize = false)
public List getSelected(){
ArrayList indexs = Lists.newArrayList((Set) getValue());
final ArrayList opts = Lists.newArrayList(options.keySet());
return Lists.transform(indexs, new Function() {
@Override
public E apply(Integer input) {
return opts.get(input);
}
});
}
@JSONField(serialize = false,deserialize = false)
public CheckOption setSelected(List sel){
sel = MoreObjects.firstNonNull(sel, Collections.emptyList());
Iterable itor = Iterables.filter(sel, new Predicate() {
@Override
public boolean apply(E input) {
return options.containsKey(input);
}
});
final ArrayList opts = Lists.newArrayList(options.keySet());
HashSet indexSet = Sets.newHashSet(Iterables.transform(itor, new Function(){
@Override
public Integer apply(E input) {
return opts.indexOf(input);
}}));
setValue(indexSet);
return this;
}
@SuppressWarnings("unchecked")
public CheckOption setSelected(E... sel){
return setSelected(Arrays.asList(sel));
}
public String contentOfOptions(){
ByteArrayOutputStream bytestream = new ByteArrayOutputStream();
PrintStream stream = new PrintStream(bytestream);
int index=0;
stream.printf("\tavailable options:\n");
for(Entry entry:options.entrySet()){
String desc = entry.getValue();
stream.printf("\t<%d>%s -- %s\n", index++,entry.getKey().toString(), desc.isEmpty() ? "NO DESC" : desc);
}
return bytestream.toString();
}
public Map getOptions() {
return options;
}
public void setOptions(Map options) {
this.options.clear();
this.options.putAll(options);
}
public BaseOption> setValue(Integer ...selects){
return setValue(Sets.newHashSet(selects));
}
@Override
public List> getAvailable() {
return Collections.emptyList();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy