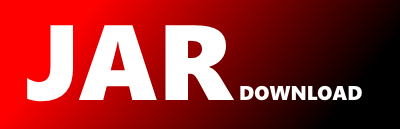
net.gdface.sdk.FaceApiGenericDecorator Maven / Gradle / Ivy
package net.gdface.sdk;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.nio.ByteBuffer;
import java.util.List;
import java.util.Map;
import net.gdface.image.ImageErrorException;
import net.gdface.utils.FaceUtilits;
/**
* decorator pattern 装饰者模式实现基于{@link FaceApiDecorator}的支持泛型方法参数{@link FaceApi}接口
* 所有 byte[] 类型的参数都支持泛型参数,参见 {@link net.gdface.utils.FaceUtilits#getBytesNotEmpty(Object)}
* 注意:
* 参数为{@link java.io.InputStream}的方法返回时会自动执行{@link java.io.InputStream#close()}关闭流
* 参见 {@link net.gdface.utils.FaceUtilits#readBytes(java.io.InputStream)}
* unchecked后缀的方法将所有显式申明的异常封装到{@link RuntimeException}抛出
* 计算机生成代码(generated by automated tools DecoratorGenerator @author guyadong)
* @author guyadong
*
*/
public class FaceApiGenericDecorator extends FaceApiDecorator{
public FaceApiGenericDecorator(FaceApi delegate) {
super(delegate);
}
//1
/**
* {@link FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)}对应的泛型方法
* @param imgData1
* 泛型参数,参见 {@link net.gdface.utils.FaceUtilits#getBytesNotEmpty(Object)}
* @param facePos1
* @param imgData2
* 泛型参数,参见 {@link net.gdface.utils.FaceUtilits#getBytesNotEmpty(Object)}
* @param facePos2
* @return double
* @see FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)
*/
public double compare2Face (T1 imgData1,CodeInfo facePos1,T2 imgData2,CodeInfo facePos2) throws ImageErrorException,NotFaceDetectedException,IOException{
return compare2Face(FaceUtilits.getBytesNotEmpty(imgData1),facePos1,FaceUtilits.getBytesNotEmpty(imgData2),facePos2);
}
//2
/**
* {@link #compare2Face(T1,CodeInfo,T2,CodeInfo)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData1
* @param facePos1
* @param imgData2
* @param facePos2
* @return double
*/
public double compare2FaceUnchecked (T1 imgData1,CodeInfo facePos1,T2 imgData2,CodeInfo facePos2) {
try{
return compare2Face(imgData1,facePos1,imgData2,facePos2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//3
/**
* 参见{@link FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)}
* @param imgData1 InputStream
* @param facePos1
* @param imgData2 InputStream
* @param facePos2
* @return double
* @throws ImageErrorException
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)
*/
public double compare2Face (InputStream imgData1,CodeInfo facePos1,InputStream imgData2,CodeInfo facePos2) throws ImageErrorException,NotFaceDetectedException,IOException{
return compare2Face(FaceUtilits.getBytesNotEmpty(imgData1),facePos1,FaceUtilits.getBytesNotEmpty(imgData2),facePos2);
}
//4
/**
* {@link #compare2Face (InputStream,CodeInfo,InputStream,CodeInfo)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData1
* @param facePos1
* @param imgData2
* @param facePos2
* @return double
*/
public double compare2FaceUnchecked (InputStream imgData1,CodeInfo facePos1,InputStream imgData2,CodeInfo facePos2) {
try{
return compare2Face(imgData1,facePos1,imgData2,facePos2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//5
/**
* 参见{@link FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)}
* @param imgData1 URL
* @param facePos1
* @param imgData2 URL
* @param facePos2
* @return double
* @throws ImageErrorException
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)
*/
public double compare2Face (URL imgData1,CodeInfo facePos1,URL imgData2,CodeInfo facePos2) throws ImageErrorException,NotFaceDetectedException,IOException{
return compare2Face(FaceUtilits.getBytesNotEmpty(imgData1),facePos1,FaceUtilits.getBytesNotEmpty(imgData2),facePos2);
}
//6
/**
* {@link #compare2Face (URL,CodeInfo,URL,CodeInfo)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData1
* @param facePos1
* @param imgData2
* @param facePos2
* @return double
*/
public double compare2FaceUnchecked (URL imgData1,CodeInfo facePos1,URL imgData2,CodeInfo facePos2) {
try{
return compare2Face(imgData1,facePos1,imgData2,facePos2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//7
/**
* 参见{@link FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)}
* @param imgData1 File
* @param facePos1
* @param imgData2 File
* @param facePos2
* @return double
* @throws ImageErrorException
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)
*/
public double compare2Face (File imgData1,CodeInfo facePos1,File imgData2,CodeInfo facePos2) throws ImageErrorException,NotFaceDetectedException,IOException{
return compare2Face(FaceUtilits.getBytesNotEmpty(imgData1),facePos1,FaceUtilits.getBytesNotEmpty(imgData2),facePos2);
}
//8
/**
* {@link #compare2Face (File,CodeInfo,File,CodeInfo)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData1
* @param facePos1
* @param imgData2
* @param facePos2
* @return double
*/
public double compare2FaceUnchecked (File imgData1,CodeInfo facePos1,File imgData2,CodeInfo facePos2) {
try{
return compare2Face(imgData1,facePos1,imgData2,facePos2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//9
/**
* 参见{@link FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)}
* @param imgData1 ByteBuffer
* @param facePos1
* @param imgData2 ByteBuffer
* @param facePos2
* @return double
* @throws ImageErrorException
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)
*/
public double compare2Face (ByteBuffer imgData1,CodeInfo facePos1,ByteBuffer imgData2,CodeInfo facePos2) throws ImageErrorException,NotFaceDetectedException,IOException{
return compare2Face(FaceUtilits.getBytesNotEmpty(imgData1),facePos1,FaceUtilits.getBytesNotEmpty(imgData2),facePos2);
}
//10
/**
* {@link #compare2Face (ByteBuffer,CodeInfo,ByteBuffer,CodeInfo)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData1
* @param facePos1
* @param imgData2
* @param facePos2
* @return double
*/
public double compare2FaceUnchecked (ByteBuffer imgData1,CodeInfo facePos1,ByteBuffer imgData2,CodeInfo facePos2) {
try{
return compare2Face(imgData1,facePos1,imgData2,facePos2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//11
/**
* {@link FaceApi#compareCode(byte[],byte[])}对应的泛型方法
* @param code1
* 泛型参数,参见 {@link net.gdface.utils.FaceUtilits#getBytesNotEmpty(Object)}
* @param code2
* 泛型参数,参见 {@link net.gdface.utils.FaceUtilits#getBytesNotEmpty(Object)}
* @return double
* @throws ImageErrorException
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#compareCode(byte[],byte[])
*/
public double compareCode (T2 code1,T2 code2) throws IOException{
return compareCode(FaceUtilits.getBytesNotEmpty(code1),FaceUtilits.getBytesNotEmpty(code2));
}
//12
/**
* {@link #compareCode(T2,T2)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param code1
* @param code2
* @return double
*/
public double compareCodeUnchecked (T2 code1,T2 code2) {
try{
return compareCode(code1,code2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//13
/**
* {@link FaceApi#compareCodes(byte[],net.gdface.sdk.CodeInfo[])}对应的泛型方法
* @param code1
* 泛型参数,参见 {@link net.gdface.utils.FaceUtilits#getBytesNotEmpty(Object)}
* @param codes
* @return double[]
* @throws IOException
* @see FaceApi#compareCodes(byte[],net.gdface.sdk.CodeInfo[])
*/
public double[] compareCodes (T code1,CodeInfo[] codes) throws IOException{
return compareCodes(FaceUtilits.getBytesNotEmpty(code1),codes);
}
//14
/**
* {@link #compareCodes(T,CodeInfo[])}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param code1
* @param codes
* @return double[]
*/
public double[] compareCodesUnchecked (T code1,CodeInfo[] codes) {
try{
return compareCodes(code1,codes);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//15
/**
* {@link FaceApi#compareFeatures(byte[],List)}对应的泛型方法
* @param code1
* 泛型参数,参见 {@link net.gdface.utils.FaceUtilits#getBytesNotEmpty(Object)}
* @param codes
* @return List
* @throws IOException
* @see FaceApi#compareFeatures(byte[],List)
*/
public List compareFeatures (T code1,List codes) throws IOException{
return compareFeatures(FaceUtilits.getBytesNotEmpty(code1),codes);
}
//16
/**
* {@link #compareFeatures(T,List)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param code1
* @param codes
* @return List
*/
public List compareFeaturesUnchecked (T code1,List codes) {
try{
return compareFeatures(code1,codes);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//17
/**
* {@link FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)}对应的泛型方法
* @param imgData1
* 泛型参数,参见 {@link net.gdface.utils.FaceUtilits#getBytesNotEmpty(Object)}
* @param detectRect1
* @param imgData2
* 泛型参数,参见 {@link net.gdface.utils.FaceUtilits#getBytesNotEmpty(Object)}
* @param detectRect2
* @return double
* @throws IOException
* @see FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)
*/
public double detectAndCompare2Face (T1 imgData1,FRect detectRect1,T2 imgData2,FRect detectRect2) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndCompare2Face(FaceUtilits.getBytesNotEmpty(imgData1),detectRect1,FaceUtilits.getBytesNotEmpty(imgData2),detectRect2);
}
//18
/**
* {@link #detectAndCompare2Face(T1,FRect,T2,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData1
* @param detectRect1
* @param imgData2
* @param detectRect2
* @return double
*/
public double detectAndCompare2FaceUnchecked (T1 imgData1,FRect detectRect1,T2 imgData2,FRect detectRect2) {
try{
return detectAndCompare2Face(imgData1,detectRect1,imgData2,detectRect2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//19
/**
* 参见{@link FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)}
* @param imgData1 InputStream
* @param detectRect1
* @param imgData2 InputStream
* @param detectRect2
* @return double
* @throws ImageErrorException
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)
*/
public double detectAndCompare2Face (InputStream imgData1,FRect detectRect1,InputStream imgData2,FRect detectRect2) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndCompare2Face(FaceUtilits.getBytesNotEmpty(imgData1),detectRect1,FaceUtilits.getBytesNotEmpty(imgData2),detectRect2);
}
//20
/**
* {@link #detectAndCompare2Face (InputStream,FRect,InputStream,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData1
* @param detectRect1
* @param imgData2
* @param detectRect2
* @return double
*/
public double detectAndCompare2FaceUnchecked (InputStream imgData1,FRect detectRect1,InputStream imgData2,FRect detectRect2) {
try{
return detectAndCompare2Face(imgData1,detectRect1,imgData2,detectRect2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//21
/**
* 参见{@link FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)}
* @param imgData1 URL
* @param detectRect1
* @param imgData2 URL
* @param detectRect2
* @return double
* @throws ImageErrorException
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)
*/
public double detectAndCompare2Face (URL imgData1,FRect detectRect1,URL imgData2,FRect detectRect2) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndCompare2Face(FaceUtilits.getBytesNotEmpty(imgData1),detectRect1,FaceUtilits.getBytesNotEmpty(imgData2),detectRect2);
}
//22
/**
* {@link #detectAndCompare2Face (URL,FRect,URL,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData1
* @param detectRect1
* @param imgData2
* @param detectRect2
* @return double
*/
public double detectAndCompare2FaceUnchecked (URL imgData1,FRect detectRect1,URL imgData2,FRect detectRect2) {
try{
return detectAndCompare2Face(imgData1,detectRect1,imgData2,detectRect2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//23
/**
* 参见{@link FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)}
* @param imgData1 File
* @param detectRect1
* @param imgData2 File
* @param detectRect2
* @return double
* @throws ImageErrorException
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)
*/
public double detectAndCompare2Face (File imgData1,FRect detectRect1,File imgData2,FRect detectRect2) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndCompare2Face(FaceUtilits.getBytesNotEmpty(imgData1),detectRect1,FaceUtilits.getBytesNotEmpty(imgData2),detectRect2);
}
//24
/**
* {@link #detectAndCompare2Face (File,FRect,File,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData1
* @param detectRect1
* @param imgData2
* @param detectRect2
* @return double
*/
public double detectAndCompare2FaceUnchecked (File imgData1,FRect detectRect1,File imgData2,FRect detectRect2) {
try{
return detectAndCompare2Face(imgData1,detectRect1,imgData2,detectRect2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//25
/**
* 参见{@link FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)}
* @param imgData1 ByteBuffer
* @param detectRect1
* @param imgData2 ByteBuffer
* @param detectRect2
* @return double
* @throws ImageErrorException
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)
*/
public double detectAndCompare2Face (ByteBuffer imgData1,FRect detectRect1,ByteBuffer imgData2,FRect detectRect2) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndCompare2Face(FaceUtilits.getBytesNotEmpty(imgData1),detectRect1,FaceUtilits.getBytesNotEmpty(imgData2),detectRect2);
}
//26
/**
* {@link #detectAndCompare2Face (ByteBuffer,FRect,ByteBuffer,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData1
* @param detectRect1
* @param imgData2
* @param detectRect2
* @return double
*/
public double detectAndCompare2FaceUnchecked (ByteBuffer imgData1,FRect detectRect1,ByteBuffer imgData2,FRect detectRect2) {
try{
return detectAndCompare2Face(imgData1,detectRect1,imgData2,detectRect2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//27
/**
* {@link FaceApi#detectAndGetCodeInfo(byte[],int,FRect)}对应的泛型方法
* @param imgData
* 泛型参数,参见 {@link net.gdface.utils.FaceUtilits#getBytesNotEmpty(Object)}
* @param faceNum
* @param detectRectangle
* @return CodeInfo[]
* @throws ImageErrorException
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#detectAndGetCodeInfo(byte[],int,FRect)
*/
public CodeInfo[] detectAndGetCodeInfo (T imgData,int faceNum,FRect detectRectangle) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndGetCodeInfo(FaceUtilits.getBytesNotEmpty(imgData),faceNum,detectRectangle);
}
//28
/**
* {@link #detectAndGetCodeInfo(T,int,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param faceNum
* @param detectRectangle
* @return CodeInfo[]
*/
public CodeInfo[] detectAndGetCodeInfoUnchecked (T imgData,int faceNum,FRect detectRectangle) {
try{
return detectAndGetCodeInfo(imgData,faceNum,detectRectangle);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//29
/**
* 参见{@link FaceApi#detectAndGetCodeInfo(byte[],int,FRect)}
* @param imgData InputStream
* @param faceNum
* @param detectRectangle
* @return CodeInfo[]
* @throws ImageErrorException
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#detectAndGetCodeInfo(byte[],int,FRect)
*/
public CodeInfo[] detectAndGetCodeInfo (InputStream imgData,int faceNum,FRect detectRectangle) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndGetCodeInfo(FaceUtilits.getBytesNotEmpty(imgData),faceNum,detectRectangle);
}
//30
/**
* {@link #detectAndGetCodeInfo (InputStream,int,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param faceNum
* @param detectRectangle
* @return CodeInfo[]
*/
public CodeInfo[] detectAndGetCodeInfoUnchecked (InputStream imgData,int faceNum,FRect detectRectangle) {
try{
return detectAndGetCodeInfo(imgData,faceNum,detectRectangle);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//31
/**
* 参见{@link FaceApi#detectAndGetCodeInfo(byte[],int,FRect)}
* @param imgData URL
* @param faceNum
* @param detectRectangle
* @return CodeInfo[]
* @throws ImageErrorException
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#detectAndGetCodeInfo(byte[],int,FRect)
*/
public CodeInfo[] detectAndGetCodeInfo (URL imgData,int faceNum,FRect detectRectangle) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndGetCodeInfo(FaceUtilits.getBytesNotEmpty(imgData),faceNum,detectRectangle);
}
//32
/**
* {@link #detectAndGetCodeInfo (URL,int,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param faceNum
* @param detectRectangle
* @return CodeInfo[]
*/
public CodeInfo[] detectAndGetCodeInfoUnchecked (URL imgData,int faceNum,FRect detectRectangle) {
try{
return detectAndGetCodeInfo(imgData,faceNum,detectRectangle);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//33
/**
* 参见{@link FaceApi#detectAndGetCodeInfo(byte[],int,FRect)}
* @param imgData File
* @param faceNum
* @param detectRectangle
* @return CodeInfo[]
* @throws ImageErrorException
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#detectAndGetCodeInfo(byte[],int,FRect)
*/
public CodeInfo[] detectAndGetCodeInfo (File imgData,int faceNum,FRect detectRectangle) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndGetCodeInfo(FaceUtilits.getBytesNotEmpty(imgData),faceNum,detectRectangle);
}
//34
/**
* {@link #detectAndGetCodeInfo (File,int,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param faceNum
* @param detectRectangle
* @return CodeInfo[]
*/
public CodeInfo[] detectAndGetCodeInfoUnchecked (File imgData,int faceNum,FRect detectRectangle) {
try{
return detectAndGetCodeInfo(imgData,faceNum,detectRectangle);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//35
/**
* 参见{@link FaceApi#detectAndGetCodeInfo(byte[],int,FRect)}
* @param imgData ByteBuffer
* @param faceNum
* @param detectRectangle
* @return CodeInfo[]
* @throws ImageErrorException
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#detectAndGetCodeInfo(byte[],int,FRect)
*/
public CodeInfo[] detectAndGetCodeInfo (ByteBuffer imgData,int faceNum,FRect detectRectangle) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndGetCodeInfo(FaceUtilits.getBytesNotEmpty(imgData),faceNum,detectRectangle);
}
//36
/**
* {@link #detectAndGetCodeInfo (ByteBuffer,int,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param faceNum
* @param detectRectangle
* @return CodeInfo[]
*/
public CodeInfo[] detectAndGetCodeInfoUnchecked (ByteBuffer imgData,int faceNum,FRect detectRectangle) {
try{
return detectAndGetCodeInfo(imgData,faceNum,detectRectangle);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//37
/**
* {@link FaceApi#detectCenterFace(byte[])}对应的泛型方法
* @param imgData
* 泛型参数,参见 {@link net.gdface.utils.FaceUtilits#getBytesNotEmpty(Object)}
* @return CodeInfo
* @throws ImageErrorException
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#detectCenterFace(byte[])
*/
public CodeInfo detectCenterFace (T imgData) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectCenterFace(FaceUtilits.getBytesNotEmpty(imgData));
}
//38
/**
* {@link #detectCenterFace(T)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @return CodeInfo
*/
public CodeInfo detectCenterFaceUnchecked (T imgData) {
try{
return detectCenterFace(imgData);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//39
/**
* {@link FaceApi#detectFace(byte[],FRect)}对应的泛型方法
* @param imgData
* 泛型参数,参见 {@link net.gdface.utils.FaceUtilits#getBytesNotEmpty(Object)}
* @param detectRectangle
* @return CodeInfo[]
* @throws ImageErrorException
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#detectFace(byte[],FRect)
*/
public CodeInfo[] detectFace (T imgData,FRect detectRectangle) throws ImageErrorException,IOException{
return detectFace(FaceUtilits.getBytesNotEmpty(imgData),detectRectangle);
}
//40
/**
* {@link #detectFace(T,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param detectRectangle
* @return CodeInfo[]
*/
public CodeInfo[] detectFaceUnchecked (T imgData,FRect detectRectangle) {
try{
return detectFace(imgData,detectRectangle);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//41
/**
* 参见{@link FaceApi#detectFace(byte[],FRect)}
* @param imgData InputStream
* @param detectRectangle
* @return CodeInfo[]
* @throws ImageErrorException
* @throws IOException
* @see FaceApi#detectFace(byte[],FRect)
*/
public CodeInfo[] detectFace (InputStream imgData,FRect detectRectangle) throws ImageErrorException,IOException{
return detectFace(FaceUtilits.getBytesNotEmpty(imgData),detectRectangle);
}
//42
/**
* {@link #detectFace (InputStream,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param detectRectangle
* @return CodeInfo[]
*/
public CodeInfo[] detectFaceUnchecked (InputStream imgData,FRect detectRectangle) {
try{
return detectFace(imgData,detectRectangle);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//43
/**
* 参见{@link FaceApi#detectFace(byte[],FRect)}
* @param imgData URL
* @param detectRectangle
* @return CodeInfo[]
* @throws ImageErrorException
* @throws IOException
* @see FaceApi#detectFace(byte[],FRect)
*/
public CodeInfo[] detectFace (URL imgData,FRect detectRectangle) throws ImageErrorException,IOException{
return detectFace(FaceUtilits.getBytesNotEmpty(imgData),detectRectangle);
}
//44
/**
* {@link #detectFace (URL,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param detectRectangle
* @return CodeInfo[]
*/
public CodeInfo[] detectFaceUnchecked (URL imgData,FRect detectRectangle) {
try{
return detectFace(imgData,detectRectangle);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//45
/**
* 参见{@link FaceApi#detectFace(byte[],FRect)}
* @param imgData File
* @param detectRectangle
* @return CodeInfo[]
* @throws ImageErrorException
* @throws IOException
* @see FaceApi#detectFace(byte[],FRect)
*/
public CodeInfo[] detectFace (File imgData,FRect detectRectangle) throws ImageErrorException,IOException{
return detectFace(FaceUtilits.getBytesNotEmpty(imgData),detectRectangle);
}
//46
/**
* {@link #detectFace (File,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param detectRectangle
* @return CodeInfo[]
*/
public CodeInfo[] detectFaceUnchecked (File imgData,FRect detectRectangle) {
try{
return detectFace(imgData,detectRectangle);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//47
/**
* 参见{@link FaceApi#detectFace(byte[],FRect)}
* @param imgData ByteBuffer
* @param detectRectangle
* @return CodeInfo[]
* @throws ImageErrorException
* @throws IOException
* @see FaceApi#detectFace(byte[],FRect)
*/
public CodeInfo[] detectFace (ByteBuffer imgData,FRect detectRectangle) throws ImageErrorException,IOException{
return detectFace(FaceUtilits.getBytesNotEmpty(imgData),detectRectangle);
}
//48
/**
* {@link #detectFace (ByteBuffer,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param detectRectangle
* @return CodeInfo[]
*/
public CodeInfo[] detectFaceUnchecked (ByteBuffer imgData,FRect detectRectangle) {
try{
return detectFace(imgData,detectRectangle);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//49
/**
* {@link FaceApi#detectFaceAgain(byte[],net.gdface.sdk.FRect[])}对应的泛型方法
* @param imgData
* 泛型参数,参见 {@link net.gdface.utils.FaceUtilits#getBytesNotEmpty(Object)}
* @param detectRects
* @return CodeInfo[]
* @throws ImageErrorException
* @throws IOException
* @see FaceApi#detectFaceAgain(byte[],net.gdface.sdk.FRect[])
*/
public CodeInfo[] detectFaceAgain (T imgData,FRect[] detectRects) throws IOException{
return detectFaceAgain(FaceUtilits.getBytesNotEmpty(imgData),detectRects);
}
//50
/**
* {@link #detectFaceAgain(T,FRect[])}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param detectRects
* @return CodeInfo[]
*/
public CodeInfo[] detectFaceAgainUnchecked (T imgData,FRect[] detectRects) {
try{
return detectFaceAgain(imgData,detectRects);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//51
/**
* 参见{@link FaceApi#detectFaceAgain(byte[],net.gdface.sdk.FRect[])}
* @param imgData InputStream
* @param detectRects
* @return CodeInfo[]
* @throws IOException
* @see FaceApi#detectFaceAgain(byte[],net.gdface.sdk.FRect[])
*/
public CodeInfo[] detectFaceAgain (InputStream imgData,FRect[] detectRects) throws IOException{
return detectFaceAgain(FaceUtilits.getBytesNotEmpty(imgData),detectRects);
}
//52
/**
* {@link #detectFaceAgain (InputStream,FRect[])}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param detectRects
* @return CodeInfo[]
*/
public CodeInfo[] detectFaceAgainUnchecked (InputStream imgData,FRect[] detectRects) {
try{
return detectFaceAgain(imgData,detectRects);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//53
/**
* 参见{@link FaceApi#detectFaceAgain(byte[],net.gdface.sdk.FRect[])}
* @param imgData URL
* @param detectRects
* @return CodeInfo[]
* @throws IOException
* @see FaceApi#detectFaceAgain(byte[],net.gdface.sdk.FRect[])
*/
public CodeInfo[] detectFaceAgain (URL imgData,FRect[] detectRects) throws IOException{
return detectFaceAgain(FaceUtilits.getBytesNotEmpty(imgData),detectRects);
}
//54
/**
* {@link #detectFaceAgain (URL,FRect[])}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param detectRects
* @return CodeInfo[]
*/
public CodeInfo[] detectFaceAgainUnchecked (URL imgData,FRect[] detectRects) {
try{
return detectFaceAgain(imgData,detectRects);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//55
/**
* 参见{@link FaceApi#detectFaceAgain(byte[],net.gdface.sdk.FRect[])}
* @param imgData File
* @param detectRects
* @return CodeInfo[]
* @throws IOException
* @see FaceApi#detectFaceAgain(byte[],net.gdface.sdk.FRect[])
*/
public CodeInfo[] detectFaceAgain (File imgData,FRect[] detectRects) throws IOException{
return detectFaceAgain(FaceUtilits.getBytesNotEmpty(imgData),detectRects);
}
//56
/**
* {@link #detectFaceAgain (File,FRect[])}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param detectRects
* @return CodeInfo[]
*/
public CodeInfo[] detectFaceAgainUnchecked (File imgData,FRect[] detectRects) {
try{
return detectFaceAgain(imgData,detectRects);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//57
/**
* 参见{@link FaceApi#detectFaceAgain(byte[],net.gdface.sdk.FRect[])}
* @param imgData ByteBuffer
* @param detectRects
* @return CodeInfo[]
* @throws IOException
* @see FaceApi#detectFaceAgain(byte[],net.gdface.sdk.FRect[])
*/
public CodeInfo[] detectFaceAgain (ByteBuffer imgData,FRect[] detectRects) throws IOException{
return detectFaceAgain(FaceUtilits.getBytesNotEmpty(imgData),detectRects);
}
//58
/**
* {@link #detectFaceAgain (ByteBuffer,FRect[])}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param detectRects
* @return CodeInfo[]
*/
public CodeInfo[] detectFaceAgainUnchecked (ByteBuffer imgData,FRect[] detectRects) {
try{
return detectFaceAgain(imgData,detectRects);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//59
/**
* {@link FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])}对应的泛型方法
* @param imgData
* 泛型参数,参见 {@link net.gdface.utils.FaceUtilits#getBytesNotEmpty(Object)}
* @param faceNum
* @param facePos
* @return CodeInfo[]
* @throws IOException
* @see FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])
*/
public CodeInfo[] getCodeInfo (T imgData,int faceNum,CodeInfo[] facePos) throws NotFaceDetectedException,IOException{
return getCodeInfo(FaceUtilits.getBytesNotEmpty(imgData),faceNum,facePos);
}
//60
/**
* {@link #getCodeInfo(T,int,CodeInfo[])}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param faceNum
* @param facePos
* @return CodeInfo[]
*/
public CodeInfo[] getCodeInfoUnchecked (T imgData,int faceNum,CodeInfo[] facePos) {
try{
return getCodeInfo(imgData,faceNum,facePos);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//61
/**
* 参见{@link FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])}
* @param imgData InputStream
* @param faceNum
* @param facePos
* @return CodeInfo[]
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])
*/
public CodeInfo[] getCodeInfo (InputStream imgData,int faceNum,CodeInfo[] facePos) throws NotFaceDetectedException,IOException{
return getCodeInfo(FaceUtilits.getBytesNotEmpty(imgData),faceNum,facePos);
}
//62
/**
* {@link #getCodeInfo (InputStream,int,CodeInfo[])}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param faceNum
* @param facePos
* @return CodeInfo[]
*/
public CodeInfo[] getCodeInfoUnchecked (InputStream imgData,int faceNum,CodeInfo[] facePos) {
try{
return getCodeInfo(imgData,faceNum,facePos);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//63
/**
* 参见{@link FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])}
* @param imgData URL
* @param faceNum
* @param facePos
* @return CodeInfo[]
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])
*/
public CodeInfo[] getCodeInfo (URL imgData,int faceNum,CodeInfo[] facePos) throws NotFaceDetectedException,IOException{
return getCodeInfo(FaceUtilits.getBytesNotEmpty(imgData),faceNum,facePos);
}
//64
/**
* {@link #getCodeInfo (URL,int,CodeInfo[])}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param faceNum
* @param facePos
* @return CodeInfo[]
*/
public CodeInfo[] getCodeInfoUnchecked (URL imgData,int faceNum,CodeInfo[] facePos) {
try{
return getCodeInfo(imgData,faceNum,facePos);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//65
/**
* 参见{@link FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])}
* @param imgData File
* @param faceNum
* @param facePos
* @return CodeInfo[]
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])
*/
public CodeInfo[] getCodeInfo (File imgData,int faceNum,CodeInfo[] facePos) throws NotFaceDetectedException,IOException{
return getCodeInfo(FaceUtilits.getBytesNotEmpty(imgData),faceNum,facePos);
}
//66
/**
* {@link #getCodeInfo (File,int,CodeInfo[])}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param faceNum
* @param facePos
* @return CodeInfo[]
*/
public CodeInfo[] getCodeInfoUnchecked (File imgData,int faceNum,CodeInfo[] facePos) {
try{
return getCodeInfo(imgData,faceNum,facePos);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//67
/**
* 参见{@link FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])}
* @param imgData ByteBuffer
* @param faceNum
* @param facePos
* @return CodeInfo[]
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])
*/
public CodeInfo[] getCodeInfo (ByteBuffer imgData,int faceNum,CodeInfo[] facePos) throws NotFaceDetectedException,IOException{
return getCodeInfo(FaceUtilits.getBytesNotEmpty(imgData),faceNum,facePos);
}
//68
/**
* {@link #getCodeInfo (ByteBuffer,int,CodeInfo[])}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param faceNum
* @param facePos
* @return CodeInfo[]
*/
public CodeInfo[] getCodeInfoUnchecked (ByteBuffer imgData,int faceNum,CodeInfo[] facePos) {
try{
return getCodeInfo(imgData,faceNum,facePos);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//69
/**
* {@link FaceApi#hasFace(byte[],FRect)}对应的泛型方法
* @param imgData
* 泛型参数,参见 {@link net.gdface.utils.FaceUtilits#getBytesNotEmpty(Object)}
* @param detectRectangle
* @return boolean
* @throws NotFaceDetectedException
* @throws IOException
* @see FaceApi#hasFace(byte[],FRect)
*/
public boolean hasFace (T imgData,FRect detectRectangle) throws ImageErrorException,IOException{
return hasFace(FaceUtilits.getBytesNotEmpty(imgData),detectRectangle);
}
//70
/**
* {@link #hasFace(T,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param detectRectangle
* @return boolean
*/
public boolean hasFaceUnchecked (T imgData,FRect detectRectangle) {
try{
return hasFace(imgData,detectRectangle);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//71
/**
* 参见{@link FaceApi#hasFace(byte[],FRect)}
* @param imgData InputStream
* @param detectRectangle
* @return boolean
* @throws ImageErrorException
* @throws IOException
* @see FaceApi#hasFace(byte[],FRect)
*/
public boolean hasFace (InputStream imgData,FRect detectRectangle) throws ImageErrorException,IOException{
return hasFace(FaceUtilits.getBytesNotEmpty(imgData),detectRectangle);
}
//72
/**
* {@link #hasFace (InputStream,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param detectRectangle
* @return boolean
*/
public boolean hasFaceUnchecked (InputStream imgData,FRect detectRectangle) {
try{
return hasFace(imgData,detectRectangle);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//73
/**
* 参见{@link FaceApi#hasFace(byte[],FRect)}
* @param imgData URL
* @param detectRectangle
* @return boolean
* @throws ImageErrorException
* @throws IOException
* @see FaceApi#hasFace(byte[],FRect)
*/
public boolean hasFace (URL imgData,FRect detectRectangle) throws ImageErrorException,IOException{
return hasFace(FaceUtilits.getBytesNotEmpty(imgData),detectRectangle);
}
//74
/**
* {@link #hasFace (URL,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param detectRectangle
* @return boolean
*/
public boolean hasFaceUnchecked (URL imgData,FRect detectRectangle) {
try{
return hasFace(imgData,detectRectangle);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//75
/**
* 参见{@link FaceApi#hasFace(byte[],FRect)}
* @param imgData File
* @param detectRectangle
* @return boolean
* @throws ImageErrorException
* @throws IOException
* @see FaceApi#hasFace(byte[],FRect)
*/
public boolean hasFace (File imgData,FRect detectRectangle) throws ImageErrorException,IOException{
return hasFace(FaceUtilits.getBytesNotEmpty(imgData),detectRectangle);
}
//76
/**
* {@link #hasFace (File,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param detectRectangle
* @return boolean
*/
public boolean hasFaceUnchecked (File imgData,FRect detectRectangle) {
try{
return hasFace(imgData,detectRectangle);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//77
/**
* 参见{@link FaceApi#hasFace(byte[],FRect)}
* @param imgData ByteBuffer
* @param detectRectangle
* @return boolean
* @throws ImageErrorException
* @throws IOException
* @see FaceApi#hasFace(byte[],FRect)
*/
public boolean hasFace (ByteBuffer imgData,FRect detectRectangle) throws ImageErrorException,IOException{
return hasFace(FaceUtilits.getBytesNotEmpty(imgData),detectRectangle);
}
//78
/**
* {@link #hasFace (ByteBuffer,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param imgData
* @param detectRectangle
* @return boolean
*/
public boolean hasFaceUnchecked (ByteBuffer imgData,FRect detectRectangle) {
try{
return hasFace(imgData,detectRectangle);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy