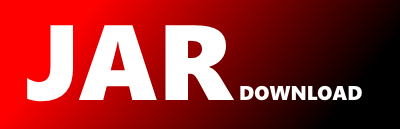
net.gdface.sdk.RectUtils Maven / Gradle / Ivy
package net.gdface.sdk;
import java.awt.Point;
import java.awt.Rectangle;
import net.gdface.utils.Assert;
import net.gdface.utils.Judge;
public class RectUtils {
public static final FRect copyFRect(FRect to,FRect from){
Assert.notNull(from, "from");
return setFRect(to,from.getLeft(),from.getTop(),from.getWidth(),from.getHeight());
}
public static final FRect copyFRect(FRect to,Rectangle from){
Assert.notNull(from, "from");
return setFRect(to,from.x,from.y,from.width,from.height);
}
public static final FRect newFRect(int left,int top,int width,int height){
FRect rect = new FRect();
rect.setLeft(left);
rect.setTop(top);
rect.setWidth(width);
rect.setHeight(height);
return rect;
}
public static final FRect setFRect(FRect frect,int left,int top,int width,int height){
Assert.notNull(frect, "frect");
frect.setLeft(left);
frect.setTop(top);
frect.setWidth(width);
frect.setHeight(height);
return frect;
}
public static final FRect newFRect(Rectangle rectangle){
Assert.notNull(rectangle, "rectangle");
return newFRect(rectangle.x,rectangle.y,rectangle.width,rectangle.height);
}
public static final Rectangle newRectangle(FRect rect){
Assert.notNull(rect,"rect");
return new Rectangle(rect.getLeft(),rect.getTop(),rect.getWidth(),rect.getHeight());
}
public static final FRect translate(FRect frect, int x, int y) {
Rectangle rectangle = newRectangle(frect);
rectangle.translate(x, y);
RectUtils.setFRect(frect, rectangle.x,rectangle.y,rectangle.width,rectangle.height);
return frect;
}
public static final EyeInfo translate(EyeInfo eyeInfo, int x, int y) {
eyeInfo.setLeftAndRight(eyeInfo.getLeftx()+x, eyeInfo.getLefty()+y, eyeInfo.getRightx()+x, eyeInfo.getRighty()+y);
return eyeInfo;
}
public static final FInt2 translate(FInt2 point, int x, int y) {
point.setX(point.getX()+x);
point.setY(point.getY()+y);
return point;
}
public static final CodeInfo translateRelative(Rectangle rect,CodeInfo ci) {
return translate(rect,ci,false);
}
public static final CodeInfo translateAbsolute(Rectangle rect,CodeInfo ci) {
return translate(rect,ci,true);
}
public static final CodeInfo translate(Rectangle rect,CodeInfo ci,boolean toAbs) {
if (!rect.getLocation().equals(ZERO_POINT)) {// 坐标系转换
return toAbs?translate(ci,rect.x,rect.y):translate(ci,-rect.x,-rect.y);
}
return ci;
}
public static final CodeInfo translate(CodeInfo ci, int x, int y) {
if(null!=ci){
if (null != ci.getPos())
translate(ci.getPos(), x, y);
if (null != ci.getEi())
translate(ci.getEi(), x, y);
if(null!=ci.getMouth())
translate(ci.getMouth(),x,y);
if(null!=ci.getNose())
translate(ci.getNose(),x,y);
}
return ci;
}
/**
* @param frect
* @param x
* @param y
* @return
* @see Rectangle#grow(int, int)
*/
public static final FRect grow(FRect frect, int x, int y) {
Rectangle rectangle = newRectangle(frect);
rectangle.grow(x, y);
RectUtils.setFRect(frect, rectangle.x,rectangle.y,rectangle.width,rectangle.height);
return frect;
}
public static final FRect intersection(FRect r1,Rectangle r2) {
Assert.notNull(r1, "r1");
Assert.notNull(r2, "r2");
return newFRect(newRectangle(r1).intersection(r2));
}
public static final FRect intersection(FRect r1,FRect r2) {
return intersection(r1,newRectangle(r2));
}
public static final void assertContains(final Rectangle parent, String argParent, final Rectangle sub, final String argSub)
throws IllegalArgumentException {
if(!parent.contains(sub))
throw new IllegalArgumentException(String.format(
"the %s(X%d,Y%d,W%d,H%d) not contained by %s(X%d,Y%d,W%d,H%d)",
argSub,sub.x, sub.y,sub.width, sub.height, argParent,parent.x,parent.y,parent.width, parent.height));
}
public static final boolean equals(FRect fr1,FRect fr2){
return !Judge.hasNull(fr1, fr2) && newRectangle(fr1).equals(newRectangle(fr2));
}
public static final boolean equals(EyeInfo ei1,EyeInfo ei2){
return !Judge.hasNull(ei1, ei2) && ei1.getLeftx() == ei2.getLeftx() && ei1.getLefty() == ei2.getLefty()
&& ei1.getRightx() == ei2.getRightx() && ei1.getRighty() == ei2.getRighty();
//return newRectangle(fr1).equals(newRectangle(fr2));
}
private static final Point ZERO_POINT = new Point(0, 0);
public static final boolean isZeroPoint(Rectangle r){
return r.getLocation().equals(ZERO_POINT);
}
/**
* 计算两点间距离
* @param p1
* @param p2
* @return
*/
public static final double distance(FInt2 p1,FInt2 p2){
int x=p1.getX()-p2.getY(),y=p1.getY()-p2.getY();
return Math.sqrt(x*x+y*y);
}
/**
* 计算矩形r中心点到p的距离
* @param r
* @param p
* @return
* @see #distance(FInt2, FInt2)
*/
public static final double distance(FRect r,FInt2 p){
return distance(new FInt2(r.getLeft()+(r.getWidth()>>1),r.getTop()+(r.getHeight()>>1)),p);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy