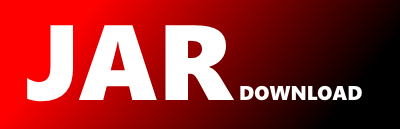
net.gdface.sdk.FaceApiGenericDecorator Maven / Gradle / Ivy
// ______________________________________________________
// Generated by codegen - https://gitee.com/l0km/codegen
// template: decorator/decorator.generic.class.vm
// ______________________________________________________
package net.gdface.sdk;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.nio.ByteBuffer;
import java.util.List;
import java.util.Map;
import net.gdface.image.ImageErrorException;
import net.gdface.image.MatType;
import net.gdface.utils.BinaryUtils;
/**
* decorator pattern 装饰者模式实现基于{@link FaceApiDecorator}的支持泛型方法参数{@link FaceApi}接口
* 所有 byte[] 类型的参数都支持泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* 注意:
* 参数为{@link java.io.InputStream}的方法返回时会自动执行{@link java.io.InputStream#close()}关闭流
* 参见 {@link net.gdface.utils.BinaryUtils#readBytes(java.io.InputStream)}
* unchecked后缀的方法将所有显式申明的异常封装到{@link RuntimeException}抛出
* 计算机生成代码(generated by automated tools DecoratorGenerator @author guyadong)
* @author guyadong
*
*/
public class FaceApiGenericDecorator extends FaceApiDecorator{
public FaceApiGenericDecorator(FaceApi delegate) {
super(delegate);
}
//1
/**
* {@link FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)}对应的泛型方法
* @param T1
* @param T2
* @param imgData1 imgData1
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param facePos1 facePos1
* @param imgData2 imgData2
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param facePos2 facePos2
* @return double
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)
*/
public double compare2Face (T1 imgData1,CodeInfo facePos1,T2 imgData2,CodeInfo facePos2) throws ImageErrorException,NotFaceDetectedException,IOException{
return compare2Face(BinaryUtils.getBytesNotEmpty(imgData1),facePos1,BinaryUtils.getBytesNotEmpty(imgData2),facePos2);
}
//2
/**
* {@link FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T1
* @param T2
* @param imgData1 imgData1
* @param facePos1 facePos1
* @param imgData2 imgData2
* @param facePos2 facePos2
* @return double
*/
public double compare2FaceUnchecked (T1 imgData1,CodeInfo facePos1,T2 imgData2,CodeInfo facePos2) {
try{
return compare2Face(imgData1,facePos1,imgData2,facePos2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//3
/**
* 参见{@link FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)}
* @param imgData1 imgData1 InputStream
* @param facePos1 facePos1
* @param imgData2 imgData2 InputStream
* @param facePos2 facePos2
* @return double
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)
*/
public double compare2Face (InputStream imgData1,CodeInfo facePos1,InputStream imgData2,CodeInfo facePos2) throws ImageErrorException,NotFaceDetectedException,IOException{
return compare2Face(BinaryUtils.getBytesNotEmpty(imgData1),facePos1,BinaryUtils.getBytesNotEmpty(imgData2),facePos2);
}
//4
/**
* {@link #compare2Face (InputStream,CodeInfo,InputStream,CodeInfo)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T1
* @param T2
* @param imgData1 imgData1
* @param facePos1 facePos1
* @param imgData2 imgData2
* @param facePos2 facePos2
* @return double
*/
public double compare2FaceUnchecked (InputStream imgData1,CodeInfo facePos1,InputStream imgData2,CodeInfo facePos2) {
try{
return compare2Face(imgData1,facePos1,imgData2,facePos2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//5
/**
* 参见{@link FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)}
* @param imgData1 imgData1 URL
* @param facePos1 facePos1
* @param imgData2 imgData2 URL
* @param facePos2 facePos2
* @return double
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)
*/
public double compare2Face (URL imgData1,CodeInfo facePos1,URL imgData2,CodeInfo facePos2) throws ImageErrorException,NotFaceDetectedException,IOException{
return compare2Face(BinaryUtils.getBytesNotEmpty(imgData1),facePos1,BinaryUtils.getBytesNotEmpty(imgData2),facePos2);
}
//6
/**
* {@link #compare2Face (URL,CodeInfo,URL,CodeInfo)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T1
* @param T2
* @param imgData1 imgData1
* @param facePos1 facePos1
* @param imgData2 imgData2
* @param facePos2 facePos2
* @return double
*/
public double compare2FaceUnchecked (URL imgData1,CodeInfo facePos1,URL imgData2,CodeInfo facePos2) {
try{
return compare2Face(imgData1,facePos1,imgData2,facePos2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//7
/**
* 参见{@link FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)}
* @param imgData1 imgData1 File
* @param facePos1 facePos1
* @param imgData2 imgData2 File
* @param facePos2 facePos2
* @return double
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)
*/
public double compare2Face (File imgData1,CodeInfo facePos1,File imgData2,CodeInfo facePos2) throws ImageErrorException,NotFaceDetectedException,IOException{
return compare2Face(BinaryUtils.getBytesNotEmpty(imgData1),facePos1,BinaryUtils.getBytesNotEmpty(imgData2),facePos2);
}
//8
/**
* {@link #compare2Face (File,CodeInfo,File,CodeInfo)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T1
* @param T2
* @param imgData1 imgData1
* @param facePos1 facePos1
* @param imgData2 imgData2
* @param facePos2 facePos2
* @return double
*/
public double compare2FaceUnchecked (File imgData1,CodeInfo facePos1,File imgData2,CodeInfo facePos2) {
try{
return compare2Face(imgData1,facePos1,imgData2,facePos2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//9
/**
* 参见{@link FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)}
* @param imgData1 imgData1 ByteBuffer
* @param facePos1 facePos1
* @param imgData2 imgData2 ByteBuffer
* @param facePos2 facePos2
* @return double
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#compare2Face(byte[],CodeInfo,byte[],CodeInfo)
*/
public double compare2Face (ByteBuffer imgData1,CodeInfo facePos1,ByteBuffer imgData2,CodeInfo facePos2) throws ImageErrorException,NotFaceDetectedException,IOException{
return compare2Face(BinaryUtils.getBytesNotEmpty(imgData1),facePos1,BinaryUtils.getBytesNotEmpty(imgData2),facePos2);
}
//10
/**
* {@link #compare2Face (ByteBuffer,CodeInfo,ByteBuffer,CodeInfo)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T1
* @param T2
* @param imgData1 imgData1
* @param facePos1 facePos1
* @param imgData2 imgData2
* @param facePos2 facePos2
* @return double
*/
public double compare2FaceUnchecked (ByteBuffer imgData1,CodeInfo facePos1,ByteBuffer imgData2,CodeInfo facePos2) {
try{
return compare2Face(imgData1,facePos1,imgData2,facePos2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//11
/**
* {@link FaceApi#compareCode(byte[],byte[])}对应的泛型方法
* @param T1
* @param T2
* @param code1 code1
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param code2 code2
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @return double
* @throws IOException IOException
* @see FaceApi#compareCode(byte[],byte[])
*/
public double compareCode (T1 code1,T2 code2) throws IOException{
return compareCode(BinaryUtils.getBytesNotEmpty(code1),BinaryUtils.getBytesNotEmpty(code2));
}
//12
/**
* {@link FaceApi#compareCode(byte[],byte[])}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T1
* @param T2
* @param code1 code1
* @param code2 code2
* @return double
*/
public double compareCodeUnchecked (T1 code1,T2 code2) {
try{
return compareCode(code1,code2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//13
/**
* {@link FaceApi#compareCodes(byte[],net.gdface.sdk.CodeInfo[])}对应的泛型方法
* @param T
* @param code1 code1
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param codes codes
* @return double[]
* @throws IOException IOException
* @see FaceApi#compareCodes(byte[],net.gdface.sdk.CodeInfo[])
*/
public double[] compareCodes (T code1,CodeInfo[] codes) throws IOException{
return compareCodes(BinaryUtils.getBytesNotEmpty(code1),codes);
}
//14
/**
* {@link FaceApi#compareCodes(byte[],net.gdface.sdk.CodeInfo[])}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param code1 code1
* @param codes codes
* @return double[]
*/
public double[] compareCodesUnchecked (T code1,CodeInfo[] codes) {
try{
return compareCodes(code1,codes);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//15
/**
* {@link FaceApi#compareFaces(byte[],byte[],int)}对应的泛型方法
* @param T1
* @param T2
* @param code code
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param imgData imgData
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param faceNum faceNum
* @return CompareResult
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#compareFaces(byte[],byte[],int)
*/
public CompareResult compareFaces (T1 code,T2 imgData,int faceNum) throws ImageErrorException,NotFaceDetectedException,IOException{
return compareFaces(BinaryUtils.getBytesNotEmpty(code),BinaryUtils.getBytesNotEmpty(imgData),faceNum);
}
//16
/**
* {@link FaceApi#compareFaces(byte[],byte[],int)}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T1
* @param T2
* @param code code
* @param imgData imgData
* @param faceNum faceNum
* @return CompareResult
*/
public CompareResult compareFacesUnchecked (T1 code,T2 imgData,int faceNum) {
try{
return compareFaces(code,imgData,faceNum);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//17
/**
* 参见{@link FaceApi#compareFaces(byte[],byte[],int)}
* @param code code InputStream
* @param imgData imgData InputStream
* @param faceNum faceNum
* @return CompareResult
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#compareFaces(byte[],byte[],int)
*/
public CompareResult compareFaces (InputStream code,InputStream imgData,int faceNum) throws ImageErrorException,NotFaceDetectedException,IOException{
return compareFaces(BinaryUtils.getBytesNotEmpty(code),BinaryUtils.getBytesNotEmpty(imgData),faceNum);
}
//18
/**
* {@link #compareFaces (InputStream,InputStream,int)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T1
* @param T2
* @param code code
* @param imgData imgData
* @param faceNum faceNum
* @return CompareResult
*/
public CompareResult compareFacesUnchecked (InputStream code,InputStream imgData,int faceNum) {
try{
return compareFaces(code,imgData,faceNum);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//19
/**
* 参见{@link FaceApi#compareFaces(byte[],byte[],int)}
* @param code code URL
* @param imgData imgData URL
* @param faceNum faceNum
* @return CompareResult
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#compareFaces(byte[],byte[],int)
*/
public CompareResult compareFaces (URL code,URL imgData,int faceNum) throws ImageErrorException,NotFaceDetectedException,IOException{
return compareFaces(BinaryUtils.getBytesNotEmpty(code),BinaryUtils.getBytesNotEmpty(imgData),faceNum);
}
//20
/**
* {@link #compareFaces (URL,URL,int)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T1
* @param T2
* @param code code
* @param imgData imgData
* @param faceNum faceNum
* @return CompareResult
*/
public CompareResult compareFacesUnchecked (URL code,URL imgData,int faceNum) {
try{
return compareFaces(code,imgData,faceNum);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//21
/**
* 参见{@link FaceApi#compareFaces(byte[],byte[],int)}
* @param code code File
* @param imgData imgData File
* @param faceNum faceNum
* @return CompareResult
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#compareFaces(byte[],byte[],int)
*/
public CompareResult compareFaces (File code,File imgData,int faceNum) throws ImageErrorException,NotFaceDetectedException,IOException{
return compareFaces(BinaryUtils.getBytesNotEmpty(code),BinaryUtils.getBytesNotEmpty(imgData),faceNum);
}
//22
/**
* {@link #compareFaces (File,File,int)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T1
* @param T2
* @param code code
* @param imgData imgData
* @param faceNum faceNum
* @return CompareResult
*/
public CompareResult compareFacesUnchecked (File code,File imgData,int faceNum) {
try{
return compareFaces(code,imgData,faceNum);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//23
/**
* 参见{@link FaceApi#compareFaces(byte[],byte[],int)}
* @param code code ByteBuffer
* @param imgData imgData ByteBuffer
* @param faceNum faceNum
* @return CompareResult
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#compareFaces(byte[],byte[],int)
*/
public CompareResult compareFaces (ByteBuffer code,ByteBuffer imgData,int faceNum) throws ImageErrorException,NotFaceDetectedException,IOException{
return compareFaces(BinaryUtils.getBytesNotEmpty(code),BinaryUtils.getBytesNotEmpty(imgData),faceNum);
}
//24
/**
* {@link #compareFaces (ByteBuffer,ByteBuffer,int)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T1
* @param T2
* @param code code
* @param imgData imgData
* @param faceNum faceNum
* @return CompareResult
*/
public CompareResult compareFacesUnchecked (ByteBuffer code,ByteBuffer imgData,int faceNum) {
try{
return compareFaces(code,imgData,faceNum);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//25
/**
* {@link FaceApi#compareFeatures(byte[],List)}对应的泛型方法
* @param T
* @param code1 code1
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param codes codes
* @return List
* @throws IOException IOException
* @see FaceApi#compareFeatures(byte[],List)
*/
public List compareFeatures (T code1,List codes) throws IOException{
return compareFeatures(BinaryUtils.getBytesNotEmpty(code1),codes);
}
//26
/**
* {@link FaceApi#compareFeatures(byte[],List)}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param code1 code1
* @param codes codes
* @return List
*/
public List compareFeaturesUnchecked (T code1,List codes) {
try{
return compareFeatures(code1,codes);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//27
/**
* {@link FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)}对应的泛型方法
* @param T1
* @param T2
* @param imgData1 imgData1
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param detectRect1 detectRect1
* @param imgData2 imgData2
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param detectRect2 detectRect2
* @return double
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)
*/
public double detectAndCompare2Face (T1 imgData1,FRect detectRect1,T2 imgData2,FRect detectRect2) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndCompare2Face(BinaryUtils.getBytesNotEmpty(imgData1),detectRect1,BinaryUtils.getBytesNotEmpty(imgData2),detectRect2);
}
//28
/**
* {@link FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T1
* @param T2
* @param imgData1 imgData1
* @param detectRect1 detectRect1
* @param imgData2 imgData2
* @param detectRect2 detectRect2
* @return double
*/
public double detectAndCompare2FaceUnchecked (T1 imgData1,FRect detectRect1,T2 imgData2,FRect detectRect2) {
try{
return detectAndCompare2Face(imgData1,detectRect1,imgData2,detectRect2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//29
/**
* 参见{@link FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)}
* @param imgData1 imgData1 InputStream
* @param detectRect1 detectRect1
* @param imgData2 imgData2 InputStream
* @param detectRect2 detectRect2
* @return double
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)
*/
public double detectAndCompare2Face (InputStream imgData1,FRect detectRect1,InputStream imgData2,FRect detectRect2) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndCompare2Face(BinaryUtils.getBytesNotEmpty(imgData1),detectRect1,BinaryUtils.getBytesNotEmpty(imgData2),detectRect2);
}
//30
/**
* {@link #detectAndCompare2Face (InputStream,FRect,InputStream,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T1
* @param T2
* @param imgData1 imgData1
* @param detectRect1 detectRect1
* @param imgData2 imgData2
* @param detectRect2 detectRect2
* @return double
*/
public double detectAndCompare2FaceUnchecked (InputStream imgData1,FRect detectRect1,InputStream imgData2,FRect detectRect2) {
try{
return detectAndCompare2Face(imgData1,detectRect1,imgData2,detectRect2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//31
/**
* 参见{@link FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)}
* @param imgData1 imgData1 URL
* @param detectRect1 detectRect1
* @param imgData2 imgData2 URL
* @param detectRect2 detectRect2
* @return double
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)
*/
public double detectAndCompare2Face (URL imgData1,FRect detectRect1,URL imgData2,FRect detectRect2) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndCompare2Face(BinaryUtils.getBytesNotEmpty(imgData1),detectRect1,BinaryUtils.getBytesNotEmpty(imgData2),detectRect2);
}
//32
/**
* {@link #detectAndCompare2Face (URL,FRect,URL,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T1
* @param T2
* @param imgData1 imgData1
* @param detectRect1 detectRect1
* @param imgData2 imgData2
* @param detectRect2 detectRect2
* @return double
*/
public double detectAndCompare2FaceUnchecked (URL imgData1,FRect detectRect1,URL imgData2,FRect detectRect2) {
try{
return detectAndCompare2Face(imgData1,detectRect1,imgData2,detectRect2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//33
/**
* 参见{@link FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)}
* @param imgData1 imgData1 File
* @param detectRect1 detectRect1
* @param imgData2 imgData2 File
* @param detectRect2 detectRect2
* @return double
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)
*/
public double detectAndCompare2Face (File imgData1,FRect detectRect1,File imgData2,FRect detectRect2) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndCompare2Face(BinaryUtils.getBytesNotEmpty(imgData1),detectRect1,BinaryUtils.getBytesNotEmpty(imgData2),detectRect2);
}
//34
/**
* {@link #detectAndCompare2Face (File,FRect,File,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T1
* @param T2
* @param imgData1 imgData1
* @param detectRect1 detectRect1
* @param imgData2 imgData2
* @param detectRect2 detectRect2
* @return double
*/
public double detectAndCompare2FaceUnchecked (File imgData1,FRect detectRect1,File imgData2,FRect detectRect2) {
try{
return detectAndCompare2Face(imgData1,detectRect1,imgData2,detectRect2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//35
/**
* 参见{@link FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)}
* @param imgData1 imgData1 ByteBuffer
* @param detectRect1 detectRect1
* @param imgData2 imgData2 ByteBuffer
* @param detectRect2 detectRect2
* @return double
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#detectAndCompare2Face(byte[],FRect,byte[],FRect)
*/
public double detectAndCompare2Face (ByteBuffer imgData1,FRect detectRect1,ByteBuffer imgData2,FRect detectRect2) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndCompare2Face(BinaryUtils.getBytesNotEmpty(imgData1),detectRect1,BinaryUtils.getBytesNotEmpty(imgData2),detectRect2);
}
//36
/**
* {@link #detectAndCompare2Face (ByteBuffer,FRect,ByteBuffer,FRect)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T1
* @param T2
* @param imgData1 imgData1
* @param detectRect1 detectRect1
* @param imgData2 imgData2
* @param detectRect2 detectRect2
* @return double
*/
public double detectAndCompare2FaceUnchecked (ByteBuffer imgData1,FRect detectRect1,ByteBuffer imgData2,FRect detectRect2) {
try{
return detectAndCompare2Face(imgData1,detectRect1,imgData2,detectRect2);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//37
/**
* {@link FaceApi#detectAndGetCodeInfo(byte[],int)}对应的泛型方法
* @param T
* @param imgData imgData
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param faceNum faceNum
* @return CodeInfo[]
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#detectAndGetCodeInfo(byte[],int)
*/
public CodeInfo[] detectAndGetCodeInfo (T imgData,int faceNum) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndGetCodeInfo(BinaryUtils.getBytesNotEmpty(imgData),faceNum);
}
//38
/**
* {@link FaceApi#detectAndGetCodeInfo(byte[],int)}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param faceNum faceNum
* @return CodeInfo[]
*/
public CodeInfo[] detectAndGetCodeInfoUnchecked (T imgData,int faceNum) {
try{
return detectAndGetCodeInfo(imgData,faceNum);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//39
/**
* 参见{@link FaceApi#detectAndGetCodeInfo(byte[],int)}
* @param imgData imgData InputStream
* @param faceNum faceNum
* @return CodeInfo[]
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#detectAndGetCodeInfo(byte[],int)
*/
public CodeInfo[] detectAndGetCodeInfo (InputStream imgData,int faceNum) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndGetCodeInfo(BinaryUtils.getBytesNotEmpty(imgData),faceNum);
}
//40
/**
* {@link #detectAndGetCodeInfo (InputStream,int)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param faceNum faceNum
* @return CodeInfo[]
*/
public CodeInfo[] detectAndGetCodeInfoUnchecked (InputStream imgData,int faceNum) {
try{
return detectAndGetCodeInfo(imgData,faceNum);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//41
/**
* 参见{@link FaceApi#detectAndGetCodeInfo(byte[],int)}
* @param imgData imgData URL
* @param faceNum faceNum
* @return CodeInfo[]
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#detectAndGetCodeInfo(byte[],int)
*/
public CodeInfo[] detectAndGetCodeInfo (URL imgData,int faceNum) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndGetCodeInfo(BinaryUtils.getBytesNotEmpty(imgData),faceNum);
}
//42
/**
* {@link #detectAndGetCodeInfo (URL,int)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param faceNum faceNum
* @return CodeInfo[]
*/
public CodeInfo[] detectAndGetCodeInfoUnchecked (URL imgData,int faceNum) {
try{
return detectAndGetCodeInfo(imgData,faceNum);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//43
/**
* 参见{@link FaceApi#detectAndGetCodeInfo(byte[],int)}
* @param imgData imgData File
* @param faceNum faceNum
* @return CodeInfo[]
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#detectAndGetCodeInfo(byte[],int)
*/
public CodeInfo[] detectAndGetCodeInfo (File imgData,int faceNum) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndGetCodeInfo(BinaryUtils.getBytesNotEmpty(imgData),faceNum);
}
//44
/**
* {@link #detectAndGetCodeInfo (File,int)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param faceNum faceNum
* @return CodeInfo[]
*/
public CodeInfo[] detectAndGetCodeInfoUnchecked (File imgData,int faceNum) {
try{
return detectAndGetCodeInfo(imgData,faceNum);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//45
/**
* 参见{@link FaceApi#detectAndGetCodeInfo(byte[],int)}
* @param imgData imgData ByteBuffer
* @param faceNum faceNum
* @return CodeInfo[]
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#detectAndGetCodeInfo(byte[],int)
*/
public CodeInfo[] detectAndGetCodeInfo (ByteBuffer imgData,int faceNum) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectAndGetCodeInfo(BinaryUtils.getBytesNotEmpty(imgData),faceNum);
}
//46
/**
* {@link #detectAndGetCodeInfo (ByteBuffer,int)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param faceNum faceNum
* @return CodeInfo[]
*/
public CodeInfo[] detectAndGetCodeInfoUnchecked (ByteBuffer imgData,int faceNum) {
try{
return detectAndGetCodeInfo(imgData,faceNum);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//47
/**
* {@link FaceApi#detectCenterFace(byte[])}对应的泛型方法
* @param T
* @param imgData imgData
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @return CodeInfo
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#detectCenterFace(byte[])
*/
public CodeInfo detectCenterFace (T imgData) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectCenterFace(BinaryUtils.getBytesNotEmpty(imgData));
}
//48
/**
* {@link FaceApi#detectCenterFace(byte[])}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @return CodeInfo
*/
public CodeInfo detectCenterFaceUnchecked (T imgData) {
try{
return detectCenterFace(imgData);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//49
/**
* {@link FaceApi#detectFace(byte[])}对应的泛型方法
* @param T
* @param imgData imgData
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @return CodeInfo[]
* @throws ImageErrorException ImageErrorException
* @throws IOException IOException
* @see FaceApi#detectFace(byte[])
*/
public CodeInfo[] detectFace (T imgData) throws ImageErrorException,IOException{
return detectFace(BinaryUtils.getBytesNotEmpty(imgData));
}
//50
/**
* {@link FaceApi#detectFace(byte[])}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @return CodeInfo[]
*/
public CodeInfo[] detectFaceUnchecked (T imgData) {
try{
return detectFace(imgData);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//51
/**
* 参见{@link FaceApi#detectFace(byte[])}
* @param imgData imgData InputStream
* @return CodeInfo[]
* @throws ImageErrorException ImageErrorException
* @throws IOException IOException
* @see FaceApi#detectFace(byte[])
*/
public CodeInfo[] detectFace (InputStream imgData) throws ImageErrorException,IOException{
return detectFace(BinaryUtils.getBytesNotEmpty(imgData));
}
//52
/**
* {@link #detectFace (InputStream)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @return CodeInfo[]
*/
public CodeInfo[] detectFaceUnchecked (InputStream imgData) {
try{
return detectFace(imgData);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//53
/**
* 参见{@link FaceApi#detectFace(byte[])}
* @param imgData imgData URL
* @return CodeInfo[]
* @throws ImageErrorException ImageErrorException
* @throws IOException IOException
* @see FaceApi#detectFace(byte[])
*/
public CodeInfo[] detectFace (URL imgData) throws ImageErrorException,IOException{
return detectFace(BinaryUtils.getBytesNotEmpty(imgData));
}
//54
/**
* {@link #detectFace (URL)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @return CodeInfo[]
*/
public CodeInfo[] detectFaceUnchecked (URL imgData) {
try{
return detectFace(imgData);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//55
/**
* 参见{@link FaceApi#detectFace(byte[])}
* @param imgData imgData File
* @return CodeInfo[]
* @throws ImageErrorException ImageErrorException
* @throws IOException IOException
* @see FaceApi#detectFace(byte[])
*/
public CodeInfo[] detectFace (File imgData) throws ImageErrorException,IOException{
return detectFace(BinaryUtils.getBytesNotEmpty(imgData));
}
//56
/**
* {@link #detectFace (File)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @return CodeInfo[]
*/
public CodeInfo[] detectFaceUnchecked (File imgData) {
try{
return detectFace(imgData);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//57
/**
* 参见{@link FaceApi#detectFace(byte[])}
* @param imgData imgData ByteBuffer
* @return CodeInfo[]
* @throws ImageErrorException ImageErrorException
* @throws IOException IOException
* @see FaceApi#detectFace(byte[])
*/
public CodeInfo[] detectFace (ByteBuffer imgData) throws ImageErrorException,IOException{
return detectFace(BinaryUtils.getBytesNotEmpty(imgData));
}
//58
/**
* {@link #detectFace (ByteBuffer)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @return CodeInfo[]
*/
public CodeInfo[] detectFaceUnchecked (ByteBuffer imgData) {
try{
return detectFace(imgData);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//59
/**
* {@link FaceApi#detectMaxFace(byte[])}对应的泛型方法
* @param T
* @param imgData imgData
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @return CodeInfo
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#detectMaxFace(byte[])
*/
public CodeInfo detectMaxFace (T imgData) throws ImageErrorException,NotFaceDetectedException,IOException{
return detectMaxFace(BinaryUtils.getBytesNotEmpty(imgData));
}
//60
/**
* {@link FaceApi#detectMaxFace(byte[])}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @return CodeInfo
*/
public CodeInfo detectMaxFaceUnchecked (T imgData) {
try{
return detectMaxFace(imgData);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//61
/**
* {@link FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])}对应的泛型方法
* @param T
* @param imgData imgData
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param faceNum faceNum
* @param facePos facePos
* @return CodeInfo[]
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])
*/
public CodeInfo[] getCodeInfo (T imgData,int faceNum,CodeInfo[] facePos) throws NotFaceDetectedException,IOException{
return getCodeInfo(BinaryUtils.getBytesNotEmpty(imgData),faceNum,facePos);
}
//62
/**
* {@link FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param faceNum faceNum
* @param facePos facePos
* @return CodeInfo[]
*/
public CodeInfo[] getCodeInfoUnchecked (T imgData,int faceNum,CodeInfo[] facePos) {
try{
return getCodeInfo(imgData,faceNum,facePos);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//63
/**
* 参见{@link FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])}
* @param imgData imgData InputStream
* @param faceNum faceNum
* @param facePos facePos
* @return CodeInfo[]
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])
*/
public CodeInfo[] getCodeInfo (InputStream imgData,int faceNum,CodeInfo[] facePos) throws NotFaceDetectedException,IOException{
return getCodeInfo(BinaryUtils.getBytesNotEmpty(imgData),faceNum,facePos);
}
//64
/**
* {@link #getCodeInfo (InputStream,int,CodeInfo[])}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param faceNum faceNum
* @param facePos facePos
* @return CodeInfo[]
*/
public CodeInfo[] getCodeInfoUnchecked (InputStream imgData,int faceNum,CodeInfo[] facePos) {
try{
return getCodeInfo(imgData,faceNum,facePos);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//65
/**
* 参见{@link FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])}
* @param imgData imgData URL
* @param faceNum faceNum
* @param facePos facePos
* @return CodeInfo[]
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])
*/
public CodeInfo[] getCodeInfo (URL imgData,int faceNum,CodeInfo[] facePos) throws NotFaceDetectedException,IOException{
return getCodeInfo(BinaryUtils.getBytesNotEmpty(imgData),faceNum,facePos);
}
//66
/**
* {@link #getCodeInfo (URL,int,CodeInfo[])}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param faceNum faceNum
* @param facePos facePos
* @return CodeInfo[]
*/
public CodeInfo[] getCodeInfoUnchecked (URL imgData,int faceNum,CodeInfo[] facePos) {
try{
return getCodeInfo(imgData,faceNum,facePos);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//67
/**
* 参见{@link FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])}
* @param imgData imgData File
* @param faceNum faceNum
* @param facePos facePos
* @return CodeInfo[]
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])
*/
public CodeInfo[] getCodeInfo (File imgData,int faceNum,CodeInfo[] facePos) throws NotFaceDetectedException,IOException{
return getCodeInfo(BinaryUtils.getBytesNotEmpty(imgData),faceNum,facePos);
}
//68
/**
* {@link #getCodeInfo (File,int,CodeInfo[])}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param faceNum faceNum
* @param facePos facePos
* @return CodeInfo[]
*/
public CodeInfo[] getCodeInfoUnchecked (File imgData,int faceNum,CodeInfo[] facePos) {
try{
return getCodeInfo(imgData,faceNum,facePos);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//69
/**
* 参见{@link FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])}
* @param imgData imgData ByteBuffer
* @param faceNum faceNum
* @param facePos facePos
* @return CodeInfo[]
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])
*/
public CodeInfo[] getCodeInfo (ByteBuffer imgData,int faceNum,CodeInfo[] facePos) throws NotFaceDetectedException,IOException{
return getCodeInfo(BinaryUtils.getBytesNotEmpty(imgData),faceNum,facePos);
}
//70
/**
* {@link #getCodeInfo (ByteBuffer,int,CodeInfo[])}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param faceNum faceNum
* @param facePos facePos
* @return CodeInfo[]
*/
public CodeInfo[] getCodeInfoUnchecked (ByteBuffer imgData,int faceNum,CodeInfo[] facePos) {
try{
return getCodeInfo(imgData,faceNum,facePos);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//71
/**
* {@link FaceApi#getCodeInfo(byte[],CodeInfo)}对应的泛型方法
* @param T
* @param imgData imgData
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param facePos facePos
* @return CodeInfo
* @throws IOException IOException
* @see FaceApi#getCodeInfo(byte[],CodeInfo)
*/
public CodeInfo getCodeInfo (T imgData,CodeInfo facePos) throws IOException{
return getCodeInfo(BinaryUtils.getBytesNotEmpty(imgData),facePos);
}
//72
/**
* {@link FaceApi#getCodeInfo(byte[],CodeInfo)}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param facePos facePos
* @return CodeInfo
*/
public CodeInfo getCodeInfoUnchecked (T imgData,CodeInfo facePos) {
try{
return getCodeInfo(imgData,facePos);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//73
/**
* 参见{@link FaceApi#getCodeInfo(byte[],CodeInfo)}
* @param imgData imgData InputStream
* @param facePos facePos
* @return CodeInfo
* @throws IOException IOException
* @see FaceApi#getCodeInfo(byte[],CodeInfo)
*/
public CodeInfo getCodeInfo (InputStream imgData,CodeInfo facePos) throws IOException{
return getCodeInfo(BinaryUtils.getBytesNotEmpty(imgData),facePos);
}
//74
/**
* {@link #getCodeInfo (InputStream,CodeInfo)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param facePos facePos
* @return CodeInfo
*/
public CodeInfo getCodeInfoUnchecked (InputStream imgData,CodeInfo facePos) {
try{
return getCodeInfo(imgData,facePos);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//75
/**
* 参见{@link FaceApi#getCodeInfo(byte[],CodeInfo)}
* @param imgData imgData URL
* @param facePos facePos
* @return CodeInfo
* @throws IOException IOException
* @see FaceApi#getCodeInfo(byte[],CodeInfo)
*/
public CodeInfo getCodeInfo (URL imgData,CodeInfo facePos) throws IOException{
return getCodeInfo(BinaryUtils.getBytesNotEmpty(imgData),facePos);
}
//76
/**
* {@link #getCodeInfo (URL,CodeInfo)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param facePos facePos
* @return CodeInfo
*/
public CodeInfo getCodeInfoUnchecked (URL imgData,CodeInfo facePos) {
try{
return getCodeInfo(imgData,facePos);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//77
/**
* 参见{@link FaceApi#getCodeInfo(byte[],CodeInfo)}
* @param imgData imgData File
* @param facePos facePos
* @return CodeInfo
* @throws IOException IOException
* @see FaceApi#getCodeInfo(byte[],CodeInfo)
*/
public CodeInfo getCodeInfo (File imgData,CodeInfo facePos) throws IOException{
return getCodeInfo(BinaryUtils.getBytesNotEmpty(imgData),facePos);
}
//78
/**
* {@link #getCodeInfo (File,CodeInfo)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param facePos facePos
* @return CodeInfo
*/
public CodeInfo getCodeInfoUnchecked (File imgData,CodeInfo facePos) {
try{
return getCodeInfo(imgData,facePos);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//79
/**
* 参见{@link FaceApi#getCodeInfo(byte[],CodeInfo)}
* @param imgData imgData ByteBuffer
* @param facePos facePos
* @return CodeInfo
* @throws IOException IOException
* @see FaceApi#getCodeInfo(byte[],CodeInfo)
*/
public CodeInfo getCodeInfo (ByteBuffer imgData,CodeInfo facePos) throws IOException{
return getCodeInfo(BinaryUtils.getBytesNotEmpty(imgData),facePos);
}
//80
/**
* {@link #getCodeInfo (ByteBuffer,CodeInfo)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param facePos facePos
* @return CodeInfo
*/
public CodeInfo getCodeInfoUnchecked (ByteBuffer imgData,CodeInfo facePos) {
try{
return getCodeInfo(imgData,facePos);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//81
/**
* {@link FaceApi#hasFace(byte[])}对应的泛型方法
* @param T
* @param imgData imgData
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @return boolean
* @throws ImageErrorException ImageErrorException
* @throws IOException IOException
* @see FaceApi#hasFace(byte[])
*/
public boolean hasFace (T imgData) throws ImageErrorException,IOException{
return hasFace(BinaryUtils.getBytesNotEmpty(imgData));
}
//82
/**
* {@link FaceApi#hasFace(byte[])}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @return boolean
*/
public boolean hasFaceUnchecked (T imgData) {
try{
return hasFace(imgData);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//83
/**
* 参见{@link FaceApi#hasFace(byte[])}
* @param imgData imgData InputStream
* @return boolean
* @throws ImageErrorException ImageErrorException
* @throws IOException IOException
* @see FaceApi#hasFace(byte[])
*/
public boolean hasFace (InputStream imgData) throws ImageErrorException,IOException{
return hasFace(BinaryUtils.getBytesNotEmpty(imgData));
}
//84
/**
* {@link #hasFace (InputStream)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @return boolean
*/
public boolean hasFaceUnchecked (InputStream imgData) {
try{
return hasFace(imgData);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//85
/**
* 参见{@link FaceApi#hasFace(byte[])}
* @param imgData imgData URL
* @return boolean
* @throws ImageErrorException ImageErrorException
* @throws IOException IOException
* @see FaceApi#hasFace(byte[])
*/
public boolean hasFace (URL imgData) throws ImageErrorException,IOException{
return hasFace(BinaryUtils.getBytesNotEmpty(imgData));
}
//86
/**
* {@link #hasFace (URL)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @return boolean
*/
public boolean hasFaceUnchecked (URL imgData) {
try{
return hasFace(imgData);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//87
/**
* 参见{@link FaceApi#hasFace(byte[])}
* @param imgData imgData File
* @return boolean
* @throws ImageErrorException ImageErrorException
* @throws IOException IOException
* @see FaceApi#hasFace(byte[])
*/
public boolean hasFace (File imgData) throws ImageErrorException,IOException{
return hasFace(BinaryUtils.getBytesNotEmpty(imgData));
}
//88
/**
* {@link #hasFace (File)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @return boolean
*/
public boolean hasFaceUnchecked (File imgData) {
try{
return hasFace(imgData);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//89
/**
* 参见{@link FaceApi#hasFace(byte[])}
* @param imgData imgData ByteBuffer
* @return boolean
* @throws ImageErrorException ImageErrorException
* @throws IOException IOException
* @see FaceApi#hasFace(byte[])
*/
public boolean hasFace (ByteBuffer imgData) throws ImageErrorException,IOException{
return hasFace(BinaryUtils.getBytesNotEmpty(imgData));
}
//90
/**
* {@link #hasFace (ByteBuffer)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @return boolean
*/
public boolean hasFaceUnchecked (ByteBuffer imgData) {
try{
return hasFace(imgData);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//91
/**
* {@link FaceApi#matDetectAndGetCodeInfo(MatType,byte[],int,int,int)}对应的泛型方法
* @param T
* @param matType matType
* @param matData matData
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param width width
* @param height height
* @param faceNum faceNum
* @return CodeInfo[]
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#matDetectAndGetCodeInfo(MatType,byte[],int,int,int)
*/
public CodeInfo[] matDetectAndGetCodeInfo (MatType matType,T matData,int width,int height,int faceNum) throws NotFaceDetectedException,IOException{
return matDetectAndGetCodeInfo(matType,BinaryUtils.getBytesNotEmpty(matData),width,height,faceNum);
}
//92
/**
* {@link FaceApi#matDetectAndGetCodeInfo(MatType,byte[],int,int,int)}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param matType matType
* @param matData matData
* @param width width
* @param height height
* @param faceNum faceNum
* @return CodeInfo[]
*/
public CodeInfo[] matDetectAndGetCodeInfoUnchecked (MatType matType,T matData,int width,int height,int faceNum) {
try{
return matDetectAndGetCodeInfo(matType,matData,width,height,faceNum);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//93
/**
* 参见{@link FaceApi#matDetectAndGetCodeInfo(MatType,byte[],int,int,int)}
* @param matType matType
* @param matData matData ByteBuffer
* @param width width
* @param height height
* @param faceNum faceNum
* @return CodeInfo[]
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#matDetectAndGetCodeInfo(MatType,byte[],int,int,int)
*/
public CodeInfo[] matDetectAndGetCodeInfo (MatType matType,ByteBuffer matData,int width,int height,int faceNum) throws NotFaceDetectedException,IOException{
return matDetectAndGetCodeInfo(matType,BinaryUtils.getBytesNotEmpty(matData),width,height,faceNum);
}
//94
/**
* {@link #matDetectAndGetCodeInfo (MatType,ByteBuffer,int,int,int)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param matType matType
* @param matData matData
* @param width width
* @param height height
* @param faceNum faceNum
* @return CodeInfo[]
*/
public CodeInfo[] matDetectAndGetCodeInfoUnchecked (MatType matType,ByteBuffer matData,int width,int height,int faceNum) {
try{
return matDetectAndGetCodeInfo(matType,matData,width,height,faceNum);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//95
/**
* {@link FaceApi#matDetectFace(MatType,byte[],int,int)}对应的泛型方法
* @param T
* @param matType matType
* @param matData matData
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param width width
* @param height height
* @return CodeInfo[]
* @throws IOException IOException
* @see FaceApi#matDetectFace(MatType,byte[],int,int)
*/
public CodeInfo[] matDetectFace (MatType matType,T matData,int width,int height) throws IOException{
return matDetectFace(matType,BinaryUtils.getBytesNotEmpty(matData),width,height);
}
//96
/**
* {@link FaceApi#matDetectFace(MatType,byte[],int,int)}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param matType matType
* @param matData matData
* @param width width
* @param height height
* @return CodeInfo[]
*/
public CodeInfo[] matDetectFaceUnchecked (MatType matType,T matData,int width,int height) {
try{
return matDetectFace(matType,matData,width,height);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//97
/**
* 参见{@link FaceApi#matDetectFace(MatType,byte[],int,int)}
* @param matType matType
* @param matData matData ByteBuffer
* @param width width
* @param height height
* @return CodeInfo[]
* @throws IOException IOException
* @see FaceApi#matDetectFace(MatType,byte[],int,int)
*/
public CodeInfo[] matDetectFace (MatType matType,ByteBuffer matData,int width,int height) throws IOException{
return matDetectFace(matType,BinaryUtils.getBytesNotEmpty(matData),width,height);
}
//98
/**
* {@link #matDetectFace (MatType,ByteBuffer,int,int)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param matType matType
* @param matData matData
* @param width width
* @param height height
* @return CodeInfo[]
*/
public CodeInfo[] matDetectFaceUnchecked (MatType matType,ByteBuffer matData,int width,int height) {
try{
return matDetectFace(matType,matData,width,height);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//99
/**
* {@link FaceApi#matDetectMaxFace(MatType,byte[],int,int)}对应的泛型方法
* @param T
* @param matType matType
* @param matData matData
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param width width
* @param height height
* @return CodeInfo
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#matDetectMaxFace(MatType,byte[],int,int)
*/
public CodeInfo matDetectMaxFace (MatType matType,T matData,int width,int height) throws NotFaceDetectedException,IOException{
return matDetectMaxFace(matType,BinaryUtils.getBytesNotEmpty(matData),width,height);
}
//100
/**
* {@link FaceApi#matDetectMaxFace(MatType,byte[],int,int)}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param matType matType
* @param matData matData
* @param width width
* @param height height
* @return CodeInfo
*/
public CodeInfo matDetectMaxFaceUnchecked (MatType matType,T matData,int width,int height) {
try{
return matDetectMaxFace(matType,matData,width,height);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//101
/**
* 参见{@link FaceApi#matDetectMaxFace(MatType,byte[],int,int)}
* @param matType matType
* @param matData matData ByteBuffer
* @param width width
* @param height height
* @return CodeInfo
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#matDetectMaxFace(MatType,byte[],int,int)
*/
public CodeInfo matDetectMaxFace (MatType matType,ByteBuffer matData,int width,int height) throws NotFaceDetectedException,IOException{
return matDetectMaxFace(matType,BinaryUtils.getBytesNotEmpty(matData),width,height);
}
//102
/**
* {@link #matDetectMaxFace (MatType,ByteBuffer,int,int)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param matType matType
* @param matData matData
* @param width width
* @param height height
* @return CodeInfo
*/
public CodeInfo matDetectMaxFaceUnchecked (MatType matType,ByteBuffer matData,int width,int height) {
try{
return matDetectMaxFace(matType,matData,width,height);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//103
/**
* {@link FaceApi#matGetCodeInfo(MatType,byte[],int,int,int,net.gdface.sdk.CodeInfo[])}对应的泛型方法
* @param T
* @param matType matType
* @param matData matData
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param width width
* @param height height
* @param facenum facenum
* @param facePos facePos
* @return CodeInfo[]
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#matGetCodeInfo(MatType,byte[],int,int,int,net.gdface.sdk.CodeInfo[])
*/
public CodeInfo[] matGetCodeInfo (MatType matType,T matData,int width,int height,int facenum,CodeInfo[] facePos) throws NotFaceDetectedException,IOException{
return matGetCodeInfo(matType,BinaryUtils.getBytesNotEmpty(matData),width,height,facenum,facePos);
}
//104
/**
* {@link FaceApi#matGetCodeInfo(MatType,byte[],int,int,int,net.gdface.sdk.CodeInfo[])}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param matType matType
* @param matData matData
* @param width width
* @param height height
* @param facenum facenum
* @param facePos facePos
* @return CodeInfo[]
*/
public CodeInfo[] matGetCodeInfoUnchecked (MatType matType,T matData,int width,int height,int facenum,CodeInfo[] facePos) {
try{
return matGetCodeInfo(matType,matData,width,height,facenum,facePos);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//105
/**
* 参见{@link FaceApi#matGetCodeInfo(MatType,byte[],int,int,int,net.gdface.sdk.CodeInfo[])}
* @param matType matType
* @param matData matData ByteBuffer
* @param width width
* @param height height
* @param facenum facenum
* @param facePos facePos
* @return CodeInfo[]
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#matGetCodeInfo(MatType,byte[],int,int,int,net.gdface.sdk.CodeInfo[])
*/
public CodeInfo[] matGetCodeInfo (MatType matType,ByteBuffer matData,int width,int height,int facenum,CodeInfo[] facePos) throws NotFaceDetectedException,IOException{
return matGetCodeInfo(matType,BinaryUtils.getBytesNotEmpty(matData),width,height,facenum,facePos);
}
//106
/**
* {@link #matGetCodeInfo (MatType,ByteBuffer,int,int,int,CodeInfo[])}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param matType matType
* @param matData matData
* @param width width
* @param height height
* @param facenum facenum
* @param facePos facePos
* @return CodeInfo[]
*/
public CodeInfo[] matGetCodeInfoUnchecked (MatType matType,ByteBuffer matData,int width,int height,int facenum,CodeInfo[] facePos) {
try{
return matGetCodeInfo(matType,matData,width,height,facenum,facePos);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//107
/**
* {@link FaceApi#matGetCodeInfo(MatType,byte[],int,int,CodeInfo)}对应的泛型方法
* @param T
* @param matType matType
* @param matData matData
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param width width
* @param height height
* @param facePos facePos
* @return CodeInfo
* @throws IOException IOException
* @see FaceApi#matGetCodeInfo(MatType,byte[],int,int,CodeInfo)
*/
public CodeInfo matGetCodeInfo (MatType matType,T matData,int width,int height,CodeInfo facePos) throws IOException{
return matGetCodeInfo(matType,BinaryUtils.getBytesNotEmpty(matData),width,height,facePos);
}
//108
/**
* {@link FaceApi#matGetCodeInfo(MatType,byte[],int,int,CodeInfo)}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param matType matType
* @param matData matData
* @param width width
* @param height height
* @param facePos facePos
* @return CodeInfo
*/
public CodeInfo matGetCodeInfoUnchecked (MatType matType,T matData,int width,int height,CodeInfo facePos) {
try{
return matGetCodeInfo(matType,matData,width,height,facePos);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//109
/**
* 参见{@link FaceApi#matGetCodeInfo(MatType,byte[],int,int,CodeInfo)}
* @param matType matType
* @param matData matData ByteBuffer
* @param width width
* @param height height
* @param facePos facePos
* @return CodeInfo
* @throws IOException IOException
* @see FaceApi#matGetCodeInfo(MatType,byte[],int,int,CodeInfo)
*/
public CodeInfo matGetCodeInfo (MatType matType,ByteBuffer matData,int width,int height,CodeInfo facePos) throws IOException{
return matGetCodeInfo(matType,BinaryUtils.getBytesNotEmpty(matData),width,height,facePos);
}
//110
/**
* {@link #matGetCodeInfo (MatType,ByteBuffer,int,int,CodeInfo)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param matType matType
* @param matData matData
* @param width width
* @param height height
* @param facePos facePos
* @return CodeInfo
*/
public CodeInfo matGetCodeInfoUnchecked (MatType matType,ByteBuffer matData,int width,int height,CodeInfo facePos) {
try{
return matGetCodeInfo(matType,matData,width,height,facePos);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//111
/**
* {@link FaceApi#matHasFace(MatType,byte[],int,int)}对应的泛型方法
* @param T
* @param matType matType
* @param matData matData
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param width width
* @param height height
* @return boolean
* @throws IOException IOException
* @see FaceApi#matHasFace(MatType,byte[],int,int)
*/
public boolean matHasFace (MatType matType,T matData,int width,int height) throws IOException{
return matHasFace(matType,BinaryUtils.getBytesNotEmpty(matData),width,height);
}
//112
/**
* {@link FaceApi#matHasFace(MatType,byte[],int,int)}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param matType matType
* @param matData matData
* @param width width
* @param height height
* @return boolean
*/
public boolean matHasFaceUnchecked (MatType matType,T matData,int width,int height) {
try{
return matHasFace(matType,matData,width,height);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//113
/**
* 参见{@link FaceApi#matHasFace(MatType,byte[],int,int)}
* @param matType matType
* @param matData matData ByteBuffer
* @param width width
* @param height height
* @return boolean
* @throws IOException IOException
* @see FaceApi#matHasFace(MatType,byte[],int,int)
*/
public boolean matHasFace (MatType matType,ByteBuffer matData,int width,int height) throws IOException{
return matHasFace(matType,BinaryUtils.getBytesNotEmpty(matData),width,height);
}
//114
/**
* {@link #matHasFace (MatType,ByteBuffer,int,int)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param matType matType
* @param matData matData
* @param width width
* @param height height
* @return boolean
*/
public boolean matHasFaceUnchecked (MatType matType,ByteBuffer matData,int width,int height) {
try{
return matHasFace(matType,matData,width,height);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//115
/**
* {@link FaceApi#matSearchFaces(MatType,byte[],int,int,CodeInfo,double,int)}对应的泛型方法
* @param T
* @param matType matType
* @param matData matData
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param width width
* @param height height
* @param facePos facePos
* @param similarty similarty
* @param rows rows
* @return FseResult[]
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#matSearchFaces(MatType,byte[],int,int,CodeInfo,double,int)
*/
public FseResult[] matSearchFaces (MatType matType,T matData,int width,int height,CodeInfo facePos,double similarty,int rows) throws ImageErrorException,NotFaceDetectedException,IOException{
return matSearchFaces(matType,BinaryUtils.getBytesNotEmpty(matData),width,height,facePos,similarty,rows);
}
//116
/**
* {@link FaceApi#matSearchFaces(MatType,byte[],int,int,CodeInfo,double,int)}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param matType matType
* @param matData matData
* @param width width
* @param height height
* @param facePos facePos
* @param similarty similarty
* @param rows rows
* @return FseResult[]
*/
public FseResult[] matSearchFacesUnchecked (MatType matType,T matData,int width,int height,CodeInfo facePos,double similarty,int rows) {
try{
return matSearchFaces(matType,matData,width,height,facePos,similarty,rows);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//117
/**
* 参见{@link FaceApi#matSearchFaces(MatType,byte[],int,int,CodeInfo,double,int)}
* @param matType matType
* @param matData matData ByteBuffer
* @param width width
* @param height height
* @param facePos facePos
* @param similarty similarty
* @param rows rows
* @return FseResult[]
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#matSearchFaces(MatType,byte[],int,int,CodeInfo,double,int)
*/
public FseResult[] matSearchFaces (MatType matType,ByteBuffer matData,int width,int height,CodeInfo facePos,double similarty,int rows) throws ImageErrorException,NotFaceDetectedException,IOException{
return matSearchFaces(matType,BinaryUtils.getBytesNotEmpty(matData),width,height,facePos,similarty,rows);
}
//118
/**
* {@link #matSearchFaces (MatType,ByteBuffer,int,int,CodeInfo,double,int)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param matType matType
* @param matData matData
* @param width width
* @param height height
* @param facePos facePos
* @param similarty similarty
* @param rows rows
* @return FseResult[]
*/
public FseResult[] matSearchFacesUnchecked (MatType matType,ByteBuffer matData,int width,int height,CodeInfo facePos,double similarty,int rows) {
try{
return matSearchFaces(matType,matData,width,height,facePos,similarty,rows);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//119
/**
* {@link FaceApi#matWearMask(MatType,byte[],int,int,CodeInfo)}对应的泛型方法
* @param T
* @param matType matType
* @param matData matData
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param width width
* @param height height
* @param faceInfo faceInfo
* @return Boolean
* @throws IOException IOException
* @see FaceApi#matWearMask(MatType,byte[],int,int,CodeInfo)
*/
public Boolean matWearMask (MatType matType,T matData,int width,int height,CodeInfo faceInfo) throws IOException{
return matWearMask(matType,BinaryUtils.getBytesNotEmpty(matData),width,height,faceInfo);
}
//120
/**
* {@link FaceApi#matWearMask(MatType,byte[],int,int,CodeInfo)}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param matType matType
* @param matData matData
* @param width width
* @param height height
* @param faceInfo faceInfo
* @return Boolean
*/
public Boolean matWearMaskUnchecked (MatType matType,T matData,int width,int height,CodeInfo faceInfo) {
try{
return matWearMask(matType,matData,width,height,faceInfo);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//121
/**
* 参见{@link FaceApi#matWearMask(MatType,byte[],int,int,CodeInfo)}
* @param matType matType
* @param matData matData ByteBuffer
* @param width width
* @param height height
* @param faceInfo faceInfo
* @return Boolean
* @throws IOException IOException
* @see FaceApi#matWearMask(MatType,byte[],int,int,CodeInfo)
*/
public Boolean matWearMask (MatType matType,ByteBuffer matData,int width,int height,CodeInfo faceInfo) throws IOException{
return matWearMask(matType,BinaryUtils.getBytesNotEmpty(matData),width,height,faceInfo);
}
//122
/**
* {@link #matWearMask (MatType,ByteBuffer,int,int,CodeInfo)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param matType matType
* @param matData matData
* @param width width
* @param height height
* @param faceInfo faceInfo
* @return Boolean
*/
public Boolean matWearMaskUnchecked (MatType matType,ByteBuffer matData,int width,int height,CodeInfo faceInfo) {
try{
return matWearMask(matType,matData,width,height,faceInfo);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//123
/**
* {@link FaceApi#searchFaces(byte[],CodeInfo,double,int)}对应的泛型方法
* @param T
* @param imgData imgData
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param facePos facePos
* @param similarty similarty
* @param rows rows
* @return FseResult[]
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#searchFaces(byte[],CodeInfo,double,int)
*/
public FseResult[] searchFaces (T imgData,CodeInfo facePos,double similarty,int rows) throws ImageErrorException,NotFaceDetectedException,IOException{
return searchFaces(BinaryUtils.getBytesNotEmpty(imgData),facePos,similarty,rows);
}
//124
/**
* {@link FaceApi#searchFaces(byte[],CodeInfo,double,int)}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param facePos facePos
* @param similarty similarty
* @param rows rows
* @return FseResult[]
*/
public FseResult[] searchFacesUnchecked (T imgData,CodeInfo facePos,double similarty,int rows) {
try{
return searchFaces(imgData,facePos,similarty,rows);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//125
/**
* 参见{@link FaceApi#searchFaces(byte[],CodeInfo,double,int)}
* @param imgData imgData InputStream
* @param facePos facePos
* @param similarty similarty
* @param rows rows
* @return FseResult[]
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#searchFaces(byte[],CodeInfo,double,int)
*/
public FseResult[] searchFaces (InputStream imgData,CodeInfo facePos,double similarty,int rows) throws ImageErrorException,NotFaceDetectedException,IOException{
return searchFaces(BinaryUtils.getBytesNotEmpty(imgData),facePos,similarty,rows);
}
//126
/**
* {@link #searchFaces (InputStream,CodeInfo,double,int)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param facePos facePos
* @param similarty similarty
* @param rows rows
* @return FseResult[]
*/
public FseResult[] searchFacesUnchecked (InputStream imgData,CodeInfo facePos,double similarty,int rows) {
try{
return searchFaces(imgData,facePos,similarty,rows);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//127
/**
* 参见{@link FaceApi#searchFaces(byte[],CodeInfo,double,int)}
* @param imgData imgData URL
* @param facePos facePos
* @param similarty similarty
* @param rows rows
* @return FseResult[]
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#searchFaces(byte[],CodeInfo,double,int)
*/
public FseResult[] searchFaces (URL imgData,CodeInfo facePos,double similarty,int rows) throws ImageErrorException,NotFaceDetectedException,IOException{
return searchFaces(BinaryUtils.getBytesNotEmpty(imgData),facePos,similarty,rows);
}
//128
/**
* {@link #searchFaces (URL,CodeInfo,double,int)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param facePos facePos
* @param similarty similarty
* @param rows rows
* @return FseResult[]
*/
public FseResult[] searchFacesUnchecked (URL imgData,CodeInfo facePos,double similarty,int rows) {
try{
return searchFaces(imgData,facePos,similarty,rows);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//129
/**
* 参见{@link FaceApi#searchFaces(byte[],CodeInfo,double,int)}
* @param imgData imgData File
* @param facePos facePos
* @param similarty similarty
* @param rows rows
* @return FseResult[]
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#searchFaces(byte[],CodeInfo,double,int)
*/
public FseResult[] searchFaces (File imgData,CodeInfo facePos,double similarty,int rows) throws ImageErrorException,NotFaceDetectedException,IOException{
return searchFaces(BinaryUtils.getBytesNotEmpty(imgData),facePos,similarty,rows);
}
//130
/**
* {@link #searchFaces (File,CodeInfo,double,int)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param facePos facePos
* @param similarty similarty
* @param rows rows
* @return FseResult[]
*/
public FseResult[] searchFacesUnchecked (File imgData,CodeInfo facePos,double similarty,int rows) {
try{
return searchFaces(imgData,facePos,similarty,rows);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//131
/**
* 参见{@link FaceApi#searchFaces(byte[],CodeInfo,double,int)}
* @param imgData imgData ByteBuffer
* @param facePos facePos
* @param similarty similarty
* @param rows rows
* @return FseResult[]
* @throws ImageErrorException ImageErrorException
* @throws NotFaceDetectedException NotFaceDetectedException
* @throws IOException IOException
* @see FaceApi#searchFaces(byte[],CodeInfo,double,int)
*/
public FseResult[] searchFaces (ByteBuffer imgData,CodeInfo facePos,double similarty,int rows) throws ImageErrorException,NotFaceDetectedException,IOException{
return searchFaces(BinaryUtils.getBytesNotEmpty(imgData),facePos,similarty,rows);
}
//132
/**
* {@link #searchFaces (ByteBuffer,CodeInfo,double,int)}对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param facePos facePos
* @param similarty similarty
* @param rows rows
* @return FseResult[]
*/
public FseResult[] searchFacesUnchecked (ByteBuffer imgData,CodeInfo facePos,double similarty,int rows) {
try{
return searchFaces(imgData,facePos,similarty,rows);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//133
/**
* {@link FaceApi#searchFeatures(byte[],double,int)}对应的泛型方法
* @param T
* @param feature feature
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param similarty similarty
* @param rows rows
* @return FseResult[]
* @throws IOException IOException
* @see FaceApi#searchFeatures(byte[],double,int)
*/
public FseResult[] searchFeatures (T feature,double similarty,int rows) throws IOException{
return searchFeatures(BinaryUtils.getBytesNotEmpty(feature),similarty,rows);
}
//134
/**
* {@link FaceApi#searchFeatures(byte[],double,int)}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param feature feature
* @param similarty similarty
* @param rows rows
* @return FseResult[]
*/
public FseResult[] searchFeaturesUnchecked (T feature,double similarty,int rows) {
try{
return searchFeatures(feature,similarty,rows);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
//135
/**
* {@link FaceApi#wearMask(byte[],CodeInfo)}对应的泛型方法
* @param T
* @param imgData imgData
* 泛型参数,参见 {@link net.gdface.utils.BinaryUtils#getBytesNotEmpty(Object)}
* @param faceInfo faceInfo
* @return Boolean
* @throws ImageErrorException ImageErrorException
* @throws IOException IOException
* @see FaceApi#wearMask(byte[],CodeInfo)
*/
public Boolean wearMask (T imgData,CodeInfo faceInfo) throws ImageErrorException,IOException{
return wearMask(BinaryUtils.getBytesNotEmpty(imgData),faceInfo);
}
//136
/**
* {@link FaceApi#wearMask(byte[],CodeInfo)}泛型方法对应的unchecked方法,
* 所有显式申明的异常都被封装到{@link RuntimeException}抛出
* @param T
* @param imgData imgData
* @param faceInfo faceInfo
* @return Boolean
*/
public Boolean wearMaskUnchecked (T imgData,CodeInfo faceInfo) {
try{
return wearMask(imgData,faceInfo);
} catch(RuntimeException e){
throw e;
} catch(Exception e){
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy