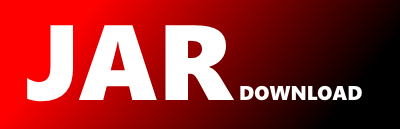
net.gdface.sdk.FaceApiSpringController Maven / Gradle / Ivy
// ______________________________________________________
// Generated by codegen - https://gitee.com/l0km/codegen
// template: decorator/spring.controller.class.vm
// ______________________________________________________
/**
* decorator pattern 装饰者模式代理{@link FaceApi}接口
* 将{@link FaceApi}实例封装为一个spring controler
* 计算机生成代码(generated by automated tools DecoratorGenerator @author guyadong)
* @author guyadong
*
*/
package net.gdface.sdk;
import java.io.File;
import java.io.InputStream;
import java.net.URL;
import java.nio.ByteBuffer;
import java.util.List;
import java.util.Map;
import net.gdface.image.ImageErrorException;
import net.gdface.image.MatType;
import java.util.ServiceLoader;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.util.Iterator;
import java.util.Objects;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.web.bind.annotation.*;
import io.swagger.annotations.*;
/**
* 人脸识别SDK核心接口
* 此接口中mat前缀的方法图像数据参数为图像矩阵(要求图像宽高必须为偶数),
* 其他方法中所有byte[]类型的图像参数都指未解码的图像格式(如jpg,bmp,png...),
* 目前支持的图像格式类型依赖于JDK的支持能力
* @author guyadong
*/
@RestController
@Api(value="FaceApi",tags={"FaceApi Controller"})
public class FaceApiSpringController {
private static final Logger logger = LoggerFactory.getLogger(FaceApiSpringController.class);
private static InstanceSupplier instanceSupplier = getInstanceSupplier();
private final ResponseFactory responseFactory = loadResponseFactory();
/**
* SPI(Service Provider Interface)机制加载 {@link InstanceSupplier}实例,没有找到则返回{@code null},
* 返回{@link InstanceSupplier}提供的{@link FaceApi}实例
* @return 返回{@link FaceApi}实例
*/
private static final InstanceSupplier getInstanceSupplier() {
/* SPI(Service Provider Interface)机制加载 {@link InstanceSupplier}实例,没有找到则抛出异常 */
ServiceLoader providers = ServiceLoader.load(InstanceSupplier.class);
Iterator itor = providers.iterator();
return itor.hasNext() ? itor.next() : null;
}
/**
* @param instanceSupplier 要设置的 instanceSupplier
*/
public static void setInstanceSupplier(InstanceSupplier instanceSupplier) {
FaceApiSpringController.instanceSupplier = instanceSupplier;
}
/**
* SPI(Service Provider Interface)加载{@link ResponseFactory}接口实例,
* 没有找到则返回{@link DefaultResponseFactory}实例
* @return 返回{@link ResponseFactory}实例
*/
private static final ResponseFactory loadResponseFactory() {
ServiceLoader providers = ServiceLoader.load(ResponseFactory.class);
Iterator itor = providers.iterator();
return itor.hasNext() ? itor.next() : new DefaultResponseFactory();
}
public FaceApiSpringController() {
}
/**
* @return 返回被装饰的{@link FaceApi}实例
*/
protected FaceApi delegate() {
return Objects.requireNonNull(
instanceSupplier == null ? null : instanceSupplier.instanceOfFaceApi(),
"FaceApi instance is null" );
}
// port-1
/**
* 对人脸图像提取特征码,返回比较相似度结果
* imgData1和imgData2相等或imgData2为{@code null}时,即比较同一张图像中的两张人脸的相似度
* 调用该方法时假设图像({@code imgData1}和{@code imgData2})能正常解码,
* 所以当对图像解码出现异常时,将{@link ImageErrorException}异常对象封装到{@link RuntimeException}抛出
* 任何参数为{@code null}则抛出{@link IllegalArgumentException}
* @param imgData1
* 图像1数据(jpg,png...)字节数组
* @param facePos1
* 检测到的人脸/眼睛位置
* @param imgData2
* 图像1数据(jpg,png...)字节数组
* @param facePos2
* 检测到的人脸/眼睛位置
* @return 两张人脸之间的相似度(0.0~1)
* @throws NotFaceDetectedException
* @throws ImageErrorException
* @see #getCodeInfo(byte[], int, CodeInfo[])
* @see #compareCode(byte[], byte[])
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/compare2Face", method = RequestMethod.POST)
@ApiOperation(value = "对人脸图像提取特征码,返回比较相似度结果
", notes = "对人脸图像提取特征码,返回比较相似度结果
\n"
+" imgData1和imgData2相等或imgData2为{@code null}时,即比较同一张图像中的两张人脸的相似度 \n"
+" 调用该方法时假设图像({@code imgData1}和{@code imgData2})能正常解码,
\n"
+" 所以当对图像解码出现异常时,将{@link ImageErrorException}异常对象封装到{@link RuntimeException}抛出
\n"
+" 任何参数为{@code null}则抛出{@link IllegalArgumentException}",httpMethod="POST")
public Response compare2Face( @RequestBody Compare2FaceArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().compare2Face(args.imgData1,args.facePos1,args.imgData2,args.facePos2));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-2
/**
* 特征码比对
* 参数为{@code null}或长度不一致则抛出{@link IllegalArgumentException}异常,返回两个特征码之间的相似度(0.0~1)
* @param code1 待比对的特征码
* @param code2 待比对的特征码
* @return 相似度(0.0~1)
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/compareCode", method = RequestMethod.POST)
@ApiOperation(value = "特征码比对
", notes = "特征码比对
\n"
+" 参数为{@code null}或长度不一致则抛出{@link IllegalArgumentException}异常,返回两个特征码之间的相似度(0.0~1)",httpMethod="POST")
public Response compareCode( @RequestBody CompareCodeArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().compareCode(args.code1,args.code2));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-3
/**
* 特征码比对1:N
* 返回对应的特征码相似度数组
* @param code1 待比对的特征码
* @param codes 包含人脸特征的{@link CodeInfo }数组
* @return 特征码相似度数组
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/compareCodes", method = RequestMethod.POST)
@ApiOperation(value = "特征码比对1:N
", notes = "特征码比对1:N
\n"
+" 返回对应的特征码相似度数组",httpMethod="POST")
public Response compareCodes( @RequestBody CompareCodesArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().compareCodes(args.code1,args.codes));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-4
/**
* 特征码比对1:N
* 对{@code imgData}图像数据检测人脸并提取特征,然后与{@code code}特征进行比对,
* 返回包含比对相似度结果的{@link CompareResult}实例
* @param code 人脸特征数据
* @param imgData 待比对的图像(jpg,png...)(可能有多张人脸)
* @param faceNum 参见 {@link #getCodeInfo(byte[], int, CodeInfo[])}
* @return {@link CompareResult}实例
* @throws NotFaceDetectedException 没有检测到人脸
* @throws ImageErrorException
* @see #compareCodes(byte[], CodeInfo[])
* @see #detectAndGetCodeInfo(byte[], int)
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/compareFaces", method = RequestMethod.POST)
@ApiOperation(value = "特征码比对1:N
", notes = "特征码比对1:N
\n"
+" 对{@code imgData}图像数据检测人脸并提取特征,然后与{@code code}特征进行比对, \n"
+" 返回包含比对相似度结果的{@link CompareResult}实例",httpMethod="POST")
public Response compareFaces( @RequestBody CompareFacesArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().compareFaces(args.code,args.imgData,args.faceNum));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-5
/**
* 特征码比对1:N
* @param code1 人脸特征数据
* @param codes 一组人脸特征
* @return 返回对应的特征码相似度列表
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/compareFeatures", method = RequestMethod.POST)
@ApiOperation(value = "特征码比对1:N
", notes = "特征码比对1:N
",httpMethod="POST")
public Response compareFeatures( @RequestBody CompareFeaturesArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().compareFeatures(args.code1,args.codes));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-6
/**
* 对两个图像指定范围({@link FRect})进行人脸检测,找到并提取唯一的人脸特征码,然后比较相似度,返回相似度结果
* imgData1和imgData2相等或imgData2为{@code null}时,
* 即比较同一张图像中的两张人脸的相似度,这种情况下detectRect1和detectRect2都不能为{@code null}
* imgData1和imgData2不相等且都不为{@code null}时,detectRect1和detectRect2被忽略
* @param imgData1
* 图像1数据(jpg,png...)字节数组,为{@code null}则抛出{@link IllegalArgumentException}
* @param detectRect1
* 图片检测范围,为null时全图检测
* @param imgData2
* 图像2数据(jpg,png...)字节数组
* @param detectRect2
* 图片检测范围,为null时全图检测
* @return 两张人脸之间的相似度(0.0~1)
* @throws ImageErrorException
* @throws NotFaceDetectedException
* @see #detectAndGetCodeInfo(byte[], int)
* @see #compareCode(byte[], byte[])
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/detectAndCompare2Face", method = RequestMethod.POST)
@ApiOperation(value = "对两个图像指定范围({@link FRect})进行人脸检测,找到并提取唯一的人脸特征码,然后比较相似度,返回相似度结果
", notes = "对两个图像指定范围({@link FRect})进行人脸检测,找到并提取唯一的人脸特征码,然后比较相似度,返回相似度结果
\n"
+" imgData1和imgData2相等或imgData2为{@code null}时, \n"
+" 即比较同一张图像中的两张人脸的相似度,这种情况下detectRect1和detectRect2都不能为{@code null}
\n"
+" imgData1和imgData2不相等且都不为{@code null}时,detectRect1和detectRect2被忽略",httpMethod="POST")
public Response detectAndCompare2Face( @RequestBody DetectAndCompare2FaceArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().detectAndCompare2Face(args.imgData1,args.detectRect1,args.imgData2,args.detectRect2));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-7
/**
* 先对图像数据{@code imgData}进行人脸检测,然后提取人脸特征码
* 返回所有成功提取特征码的{@link CodeInfo}数组,
* 与{@link #getCodeInfo(byte[], int, CodeInfo[])}的返回结果有差别,返回结果中不包含检测到人脸但提取特征码失败的对象
* @param imgData
* 图像数据(jpg,png...)字节数组,为{@code null}则抛出{@link IllegalArgumentException}
* @param faceNum
* 参见 {@link #getCodeInfo(byte[], int, CodeInfo[])}
* @return {@link CodeInfo}数组
* @throws ImageErrorException
* @throws NotFaceDetectedException 没有检测人脸或提取到特征码的人脸数目不是要求的人脸数目(faceNum>0)
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/detectAndGetCodeInfo", method = RequestMethod.POST)
@ApiOperation(value = "先对图像数据{@code imgData}进行人脸检测,然后提取人脸特征码
", notes = "先对图像数据{@code imgData}进行人脸检测,然后提取人脸特征码
\n"
+" 返回所有成功提取特征码的{@link CodeInfo}数组, \n"
+" 与{@link #getCodeInfo(byte[], int, CodeInfo[])}的返回结果有差别,返回结果中不包含检测到人脸但提取特征码失败的对象",httpMethod="POST")
public Response detectAndGetCodeInfo( @RequestBody DetectAndGetCodeInfoArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().detectAndGetCodeInfo(args.imgData,args.faceNum));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-8
/**
* 检测最中心的人脸
* 返回人脸位置数据对象{@link CodeInfo}
* @param imgData 图像数据(jpg,png...)字节数组,为{@code null}则抛出{@link IllegalArgumentException}
* @return {@link CodeInfo}
* @throws NotFaceDetected 没有检测到人脸
* @throws ImageError 图像读取错误
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/detectCenterFace", method = RequestMethod.POST)
@ApiOperation(value = "检测最中心的人脸
", notes = "检测最中心的人脸
\n"
+" 返回人脸位置数据对象{@link CodeInfo}",httpMethod="POST")
public Response detectCenterFace( @RequestBody DetectCenterFaceArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().detectCenterFace(args.imgData));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-9
/**
* 对图像({@code imgData})进行人脸检测,返回人脸位置数据对象{@link CodeInfo}数组
* 没有检测到人脸则返回空数组
* @param imgData
* 图像数据(jpg,png...)字节数组,为{@code null}则抛出{@link IllegalArgumentException}
* @return {@link CodeInfo}数组
* @throws ImageErrorException 图像读取错误
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/detectFace", method = RequestMethod.POST)
@ApiOperation(value = "对图像({@code imgData})进行人脸检测,返回人脸位置数据对象{@link CodeInfo}数组
", notes = "对图像({@code imgData})进行人脸检测,返回人脸位置数据对象{@link CodeInfo}数组
\n"
+" 没有检测到人脸则返回空数组",httpMethod="POST")
public Response detectFace( @RequestBody DetectFaceArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().detectFace(args.imgData));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-10
/**
* 检测最大的人脸
* 返回人脸位置数据对象{@link CodeInfo}
* @param imgData 图像数据(jpg,png...)字节数组,为{@code null}则抛出{@link IllegalArgumentException}
* @return {@link CodeInfo}
* @throws NotFaceDetected 没有检测到人脸
* @throws ImageError 图像读取错误
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/detectMaxFace", method = RequestMethod.POST)
@ApiOperation(value = "检测最大的人脸
", notes = "检测最大的人脸
\n"
+" 返回人脸位置数据对象{@link CodeInfo}",httpMethod="POST")
public Response detectMaxFace( @RequestBody DetectMaxFaceArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().detectMaxFace(args.imgData));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-11
/**
* 根据{@code facePos}提供的人脸信息位置, 在{@code imgData}图像中提取特征码
* 与 {@link #detectAndGetCodeInfo(byte[], int)}不同, 本方法不对图像数据{@code imgData}进行人脸检测,
* 假设{@code facePos}是 {@link #detectFace(byte[])}或 {@link #detectFaceAgain(byte[], FRect[])} 的返回结果
* 返回facePos,如果没有提取到特征码,则对应元素{@link CodeInfo#getCode()}返回{@code null}
* @param imgData
* 图像数据(jpg,png...)字节数组,为{@code null}则抛出 {@link IllegalArgumentException}
* @param faceNum
* 要求返回的人脸特征码数目
* 大于0时,如果实际提取到的人脸特征码数目不等于{@code faceNum},则抛出{@link NotFaceDetectedException}
* 小于等于0时,返回所有提取到到人脸特征码
* @param facePos
* 检测到的人脸位置对象列表
* 为{@code null}或数组长度为0时抛出{@link IllegalArgumentException}
* 如果元素为{@code null},则跳过
* @return 返回facePos
* @throws NotFaceDetectedException 提取特征码的人脸数目为0或没有提取到指定数目(faceNum大于0时)的特征码
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/getCodeInfo", method = RequestMethod.POST)
@ApiOperation(value = "根据{@code facePos}提供的人脸信息位置, 在{@code imgData}图像中提取特征码
", notes = "根据{@code facePos}提供的人脸信息位置, 在{@code imgData}图像中提取特征码
\n"
+" 与 {@link #detectAndGetCodeInfo(byte[], int)}不同, 本方法不对图像数据{@code imgData}进行人脸检测,
\n"
+" 假设{@code facePos}是 {@link #detectFace(byte[])}或 {@link #detectFaceAgain(byte[], FRect[])} 的返回结果
\n"
+" 返回facePos,如果没有提取到特征码,则对应元素{@link CodeInfo#getCode()}返回{@code null}",httpMethod="POST")
public Response getCodeInfo( @RequestBody GetCodeInfoArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().getCodeInfo(args.imgData,args.faceNum,args.facePos));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-12
/**
* 根据{@code facePos}提供的人脸信息位置, 在{@code imgData}图像中提取特征码
* 返回包含人脸特征的{@link CodeInfo}对象,提取特征失败则返回{@code null}
* @param imgData 图像数据(jpg,png...)字节数组,为{@code null}则抛出 {@link IllegalArgumentException}
* @param facePos 人脸位置信息对象,为{@code null}则抛出异常
* @return {@link CodeInfo} or {@code null}
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/getCodeInfoSingle", method = RequestMethod.POST)
@ApiOperation(value = "根据{@code facePos}提供的人脸信息位置, 在{@code imgData}图像中提取特征码
", notes = "根据{@code facePos}提供的人脸信息位置, 在{@code imgData}图像中提取特征码
\n"
+" 返回包含人脸特征的{@link CodeInfo}对象,提取特征失败则返回{@code null}",httpMethod="POST")
public Response getCodeInfo( @RequestBody GetCodeInfoSingleArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().getCodeInfo(args.imgData,args.facePos));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-13
/**
* 多张人脸提取特征(用于多张人脸合成一个特征的算法)
* 返回人脸特征数据
* {@link #multiFaceFeature()}返回{@code false}时代表此方法未被实现,执行会抛出异常
* @param faces 人脸图像数据(jpg,png...)与人脸位置信息对象的映射
* @return 人脸特征数据
* @throws NotFaceDetectedException
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/getFeature", method = RequestMethod.POST)
@ApiOperation(value = "多张人脸提取特征(用于多张人脸合成一个特征的算法)
", notes = "多张人脸提取特征(用于多张人脸合成一个特征的算法)
\n"
+" 返回人脸特征数据 \n"
+" {@link #multiFaceFeature()}返回{@code false}时代表此方法未被实现,执行会抛出异常",httpMethod="POST")
public Response getFeature( @RequestBody GetFeatureArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().getFeature(args.faces));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-14
/**
* 判断图像是否能提取到人脸特征码
* 为{@code true}则能检测到人脸并能提取特征码
* @param imgData
* 图像数据(jpg,png...)字节数组,为{@code null}则抛出{@link IllegalArgumentException}
* @return 为{@code true}则能检测到人脸并能提取特征码
* @throws ImageErrorException
* @see #detectAndGetCodeInfo(byte[], int)
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/hasFace", method = RequestMethod.POST)
@ApiOperation(value = "判断图像是否能提取到人脸特征码
", notes = "判断图像是否能提取到人脸特征码
\n"
+" 为{@code true}则能检测到人脸并能提取特征码",httpMethod="POST")
public Response hasFace( @RequestBody HasFaceArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().hasFace(args.imgData));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-15
/**
* 返回当前接口实现方式
* false WebService类型
* true 本地实现
* @return boolean
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/isLocal", method = RequestMethod.POST)
@ApiOperation(value = "返回当前接口实现方式
", notes = "返回当前接口实现方式
\n"
+" false WebService类型
\n"
+" true 本地实现",httpMethod="POST")
public Response isLocal()
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(false);
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-16
/**
* 先对图像矩阵数据{@code imgData}进行人脸检测,然后提取人脸特征码
* 返回包含人脸特征数据的{@code CodeInfo}数组
* @param matType 图像矩阵类型
* @param matData 图像矩阵
* @param width 图像宽度
* @param height 图像高度
* @param faceNum 参见 {@link #getCodeInfo(byte[], int, CodeInfo[])}
* @return {@code CodeInfo}数组
* @throws NotFaceDetectedException
* @since 2.1.9
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/matDetectAndGetCodeInfo", method = RequestMethod.POST)
@ApiOperation(value = "先对图像矩阵数据{@code imgData}进行人脸检测,然后提取人脸特征码
", notes = "先对图像矩阵数据{@code imgData}进行人脸检测,然后提取人脸特征码
\n"
+" 返回包含人脸特征数据的{@code CodeInfo}数组",httpMethod="POST")
public Response matDetectAndGetCodeInfo( @RequestBody MatDetectAndGetCodeInfoArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().matDetectAndGetCodeInfo(args.matType,args.matData,args.width,args.height,args.faceNum));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-17
/**
* 对图像矩阵进行人脸检测
* 返回人脸信息对象列表,没有检测到人脸返回空表
* @param matType 图像矩阵类型
* @param matData 图像矩阵
* @param width 图像宽度
* @param height 图像高度
* @return 人脸信息对象列表
* @since 2.1.9
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/matDetectFace", method = RequestMethod.POST)
@ApiOperation(value = "对图像矩阵进行人脸检测
", notes = "对图像矩阵进行人脸检测
\n"
+" 返回人脸信息对象列表,没有检测到人脸返回空表",httpMethod="POST")
public Response matDetectFace( @RequestBody MatDetectFaceArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().matDetectFace(args.matType,args.matData,args.width,args.height));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-18
/**
* 检测最大的人脸
* 返回人脸位置数据对象{@link CodeInfo}
* @param imgData 图像数据(jpg,png...)字节数组,为{@code null}则抛出{@link IllegalArgumentException}
* @return {@link CodeInfo}
* @throws NotFaceDetected 没有检测到人脸
* @since 2.1.9
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/matDetectMaxFace", method = RequestMethod.POST)
@ApiOperation(value = "检测最大的人脸
", notes = "检测最大的人脸
\n"
+" 返回人脸位置数据对象{@link CodeInfo}",httpMethod="POST")
public Response matDetectMaxFace( @RequestBody MatDetectMaxFaceArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().matDetectMaxFace(args.matType,args.matData,args.width,args.height));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-19
/**
* 根据facePos提供的人脸信息位置, 在图像矩阵中提取特征码
* 包含人脸特征数据的facePos
* @param matType 图像矩阵类型
* @param matData 图像矩阵
* @param width 图像宽度
* @param height 图像高度
* @param facenum
* 要求返回的人脸特征码数目
* 大于0时,如果实际提取到的人脸特征码数目不等于{@code faceNum},则抛出{@link NotFaceDetectedException}
* 小于等于0时,返回所有提取到到人脸特征码
* @param facePos 人脸位置信息对象,不可为{@code null}
* @return always facePos
* @throws NotFaceDetectedException 提取特征码的人脸数目为0或没有提取到指定数目(faceNum大于0时)的特征码
* @since 2.1.9
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/matGetCodeInfo", method = RequestMethod.POST)
@ApiOperation(value = "根据facePos提供的人脸信息位置, 在图像矩阵中提取特征码
", notes = "根据facePos提供的人脸信息位置, 在图像矩阵中提取特征码
\n"
+" 包含人脸特征数据的facePos",httpMethod="POST")
public Response matGetCodeInfo( @RequestBody MatGetCodeInfoArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().matGetCodeInfo(args.matType,args.matData,args.width,args.height,args.facenum,args.facePos));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-20
/**
* 根据{@code facePos}提供的人脸信息位置, 在图像矩阵中提取特征码
* 返回包含人脸特征的{@link CodeInfo}对象,提取特征失败则返回{@code null}
* @param matType 图像矩阵类型
* @param matData 图像矩阵
* @param width 图像宽度
* @param height 图像高度
* @param facePos 人脸位置信息对象,为{@code null}则抛出异常
* @return {@link CodeInfo} or {@code null}
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/matGetCodeInfoSingle", method = RequestMethod.POST)
@ApiOperation(value = "根据{@code facePos}提供的人脸信息位置, 在图像矩阵中提取特征码
", notes = "根据{@code facePos}提供的人脸信息位置, 在图像矩阵中提取特征码
\n"
+" 返回包含人脸特征的{@link CodeInfo}对象,提取特征失败则返回{@code null}",httpMethod="POST")
public Response matGetCodeInfo( @RequestBody MatGetCodeInfoSingleArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().matGetCodeInfo(args.matType,args.matData,args.width,args.height,args.facePos));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-21
/**
* 判断图像矩阵是否能提取到人脸特征码
* 返回为{@code true}则能检测到人脸并能提取特征码
* @param matType 图像矩阵类型
* @param matData 图像矩阵,为{@code null}则抛出{@link IllegalArgumentException}
* @param width 图像宽度
* @param height 图像高度
* @return 为{@code true}则能检测到人脸并能提取特征码
* @since 2.1.9
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/matHasFace", method = RequestMethod.POST)
@ApiOperation(value = "判断图像矩阵是否能提取到人脸特征码
", notes = "判断图像矩阵是否能提取到人脸特征码
\n"
+" 返回为{@code true}则能检测到人脸并能提取特征码",httpMethod="POST")
public Response matHasFace( @RequestBody MatHasFaceArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().matHasFace(args.matType,args.matData,args.width,args.height));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-22
/**
* 1:N 人脸图像搜索
* 对{@code facePos}指定的人脸位置提取特征码,然后在数据库中搜索相似的人脸返回搜索结果。
* 返回包含相似度计算结果的FseResult数组
* 相似度值通过 FseResult.getSimilartys() 获取
* 对应的人脸特征ID由 FseResult.getFeatureIds()获取
* @param matType 图像矩阵类型
* @param matData 图像矩阵
* @param width 图像宽度
* @param facePos
* 人脸位置对象
* 为{@code null}时,先做人脸检测再提取特征码
* 不为{@code null}时,直接在指定的位置提取特征码
* @param similarty 相似度阀值
* @param rows 最多返回的记录数目
* @return 包含相似度计算结果的{@link FseResult}数组
* @since 3.0.0
* @throws NotFaceDetectedException -
* @throws ImageErrorException -
* @throws UnsupportedOperationException 没有人脸识别算法(FaceApi实例)支持
* @see #getCodeInfo(byte[], int, CodeInfo[])
* @see #searchFeatures(byte[], double, int)
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/matSearchFaces", method = RequestMethod.POST)
@ApiOperation(value = "1:N 人脸图像搜索
", notes = "1:N 人脸图像搜索
\n"
+" 对{@code facePos}指定的人脸位置提取特征码,然后在数据库中搜索相似的人脸返回搜索结果。
\n"
+" 返回包含相似度计算结果的FseResult数组
\n"
+" 相似度值通过 FseResult.getSimilartys() 获取
\n"
+" 对应的人脸特征ID由 FseResult.getFeatureIds()获取
",httpMethod="POST")
public Response matSearchFaces( @RequestBody MatSearchFacesArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().matSearchFaces(args.matType,args.matData,args.width,args.height,args.facePos,args.similarty,args.rows));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-23
/**
* 检测指定的人脸是否戴口罩
* 返回值有三种状态:
*
* - {@code true} 戴口罩返回
* - {@code false} 未戴口罩返回
* - {@code null} 不知道
*
* @param matType 图像矩阵类型
* @param matData 图像矩阵
* @param width 图像宽度
* @param height 图像高度
* @param faceInfo 人脸位置信息
* @return 返回口罩检测结果
* @since 2.1.9
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/matWearMask", method = RequestMethod.POST)
@ApiOperation(value = "检测指定的人脸是否戴口罩
", notes = "检测指定的人脸是否戴口罩
\n"
+" 返回值有三种状态: \n"
+" \n"
+" - {@code true} 戴口罩返回
\n"
+" - {@code false} 未戴口罩返回
\n"
+" - {@code null} 不知道
\n"
+"
",httpMethod="POST")
public Response matWearMask( @RequestBody MatWearMaskArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().matWearMask(args.matType,args.matData,args.width,args.height,args.faceInfo));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-24
/**
* 返回当前SDK特性(能力)描述,已定义字段:
*
* - SDK_VERSION [string]SDK版本号
* - MULTI_FACE_FEATURE [boolean]算法是否支持多人脸合成特征
* - FACE_LIVE [boolean]是否支持活体检测
* - WEAR_MASK [boolean]是否支持口罩检测
* - FDDATA_SIZE [int]人脸检测数据的(byte)长度
* - FEATURE_SIZE [int]人脸特征数据(byte)长度
* - MAX_FACE_COUNT [int]最大检测人脸数目
* - FSE_ENABLE [boolean]是否支持特征内存搜索引擎
* - CODEINFO_RELOCATE [boolean]是否支持CodeInfo对象重定位
* - LOCAL_DETECT [boolean] 人脸检测是否为本地实现,未定义则为false
*
* 以上字段名常量定义参见{@link CapacityFieldConstant}
* @return key-value 对描述算法能力的映射
* @since 2.2.7
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/sdkCapacity", method = RequestMethod.POST)
@ApiOperation(value = "返回当前SDK特性(能力)描述,已定义字段:
", notes = "返回当前SDK特性(能力)描述,已定义字段:
\n"
+" \n"
+" - SDK_VERSION [string]SDK版本号
\n"
+" - MULTI_FACE_FEATURE [boolean]算法是否支持多人脸合成特征
\n"
+" - FACE_LIVE [boolean]是否支持活体检测
\n"
+" - WEAR_MASK [boolean]是否支持口罩检测
\n"
+" - FDDATA_SIZE [int]人脸检测数据的(byte)长度
\n"
+" - FEATURE_SIZE [int]人脸特征数据(byte)长度
\n"
+" - MAX_FACE_COUNT [int]最大检测人脸数目
\n"
+" - FSE_ENABLE [boolean]是否支持特征内存搜索引擎
\n"
+" - CODEINFO_RELOCATE [boolean]是否支持CodeInfo对象重定位
\n"
+" - LOCAL_DETECT [boolean] 人脸检测是否为本地实现,未定义则为false
\n"
+"
\n"
+" 以上字段名常量定义参见{@link CapacityFieldConstant}",httpMethod="POST")
public Response sdkCapacity()
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().sdkCapacity());
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-25
/**
* 1:N 人脸图像搜索
* 对{@code facePos}指定的人脸位置提取特征码,然后在数据库中搜索相似的人脸返回搜索结果。
* 返回包含相似度计算结果的FseResult数组
* 相似度值通过 FseResult.getSimilartys() 获取
* 对应的人脸特征ID由 FseResult.getFeatureIds()获取
* @param imgData
* 图片字节数组
* @param facePos
* 人脸位置对象
* 为{@code null}时,先做人脸检测再提取特征码
* 不为{@code null}时,直接在指定的位置提取特征码
* @param similarty 相似度阀值
* @param rows 最多返回的记录数目
* @return 包含相似度计算结果的{@link FseResult}数组
* @since 3.0.0
* @throws NotFaceDetectedException -
* @throws ImageErrorException -
* @throws UnsupportedOperationException 没有人脸识别算法(FaceApi实例)支持
* @see #getCodeInfo(byte[], int, CodeInfo[])
* @see #searchFeatures(byte[], double, int)
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/searchFaces", method = RequestMethod.POST)
@ApiOperation(value = "1:N 人脸图像搜索
", notes = "1:N 人脸图像搜索
\n"
+" 对{@code facePos}指定的人脸位置提取特征码,然后在数据库中搜索相似的人脸返回搜索结果。
\n"
+" 返回包含相似度计算结果的FseResult数组
\n"
+" 相似度值通过 FseResult.getSimilartys() 获取
\n"
+" 对应的人脸特征ID由 FseResult.getFeatureIds()获取
",httpMethod="POST")
public Response searchFaces( @RequestBody SearchFacesArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().searchFaces(args.imgData,args.facePos,args.similarty,args.rows));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-26
/**
* 1:N 人脸特征搜索
* 搜索与{@code code}相似度大于{@code similarty}的记录(最多返回前{@code rows}个结果)
* 返回包含相似度计算结果的{@link FseResult}数组,返回结果以相似度降序排列
* 相似度值通过 {@link FseResult#getSimilartys()} 获取
* 对应的人脸特征ID由 {@link FseResult#getFeatureIds()}获取
* @param feature
* 要搜索的特征码
* @param similarty
* 相似度阀值
* @param rows
* 最多返回的记录数目
* @return 包含相似度计算结果的{@link FseResult}数组
* @since 3.0.0
* @throws UnsupportedOperationException 没有人脸识别算法(FaceApi实例)支持
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/searchFeatures", method = RequestMethod.POST)
@ApiOperation(value = "1:N 人脸特征搜索
", notes = "1:N 人脸特征搜索
\n"
+" 搜索与{@code code}相似度大于{@code similarty}的记录(最多返回前{@code rows}个结果)
\n"
+" 返回包含相似度计算结果的{@link FseResult}数组,返回结果以相似度降序排列
\n"
+" 相似度值通过 {@link FseResult#getSimilartys()} 获取
\n"
+" 对应的人脸特征ID由 {@link FseResult#getFeatureIds()}获取",httpMethod="POST")
public Response searchFeatures( @RequestBody SearchFeaturesArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().searchFeatures(args.feature,args.similarty,args.rows));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
// port-27
/**
* 检测指定的人脸是否戴口罩
* 返回值有三种状态:
*
* - {@code true} 戴口罩返回
* - {@code false} 未戴口罩返回
* - {@code null} 不知道
*
* @param imgData 图像数据(jpg,png...)字节数组,为{@code null}则抛出 {@link IllegalArgumentException}
* @param faceInfo 可见光图像人脸信息
* @return 返回口罩检测结果
* @throws ImageErrorException
* @since 2.1.9
*/
@ResponseBody
@RequestMapping(value = "/FaceApi/wearMask", method = RequestMethod.POST)
@ApiOperation(value = "检测指定的人脸是否戴口罩
", notes = "检测指定的人脸是否戴口罩
\n"
+" 返回值有三种状态: \n"
+" \n"
+" - {@code true} 戴口罩返回
\n"
+" - {@code false} 未戴口罩返回
\n"
+" - {@code null} 不知道
\n"
+"
",httpMethod="POST")
public Response wearMask( @RequestBody WearMaskArgs args)
{
Response response = responseFactory.newFaceApiResponse();
try{
response.onComplete(delegate().wearMask(args.imgData,args.faceInfo));
}
catch(Exception e){
logger.error(e.getMessage(),e);
response.onError(e);
}
return response;
}
/**
* argClass-1
* wrap arguments for method {@link #compare2Face(Compare2FaceArgs)}
*/
public static class Compare2FaceArgs{
@ApiModelProperty(value ="图像1数据(jpg,png...)字节数组" ,required=true ,dataType="byte[]")
public byte[] imgData1;
@ApiModelProperty(value ="检测到的人脸/眼睛位置" ,required=true ,dataType="CodeInfo")
public CodeInfo facePos1;
@ApiModelProperty(value ="图像1数据(jpg,png...)字节数组" ,required=true ,dataType="byte[]")
public byte[] imgData2;
@ApiModelProperty(value ="检测到的人脸/眼睛位置" ,required=true ,dataType="CodeInfo")
public CodeInfo facePos2;
}
/**
* argClass-2
* wrap arguments for method {@link #compareCode(CompareCodeArgs)}
*/
public static class CompareCodeArgs{
@ApiModelProperty(value ="待比对的特征码" ,required=true ,dataType="byte[]")
public byte[] code1;
@ApiModelProperty(value ="待比对的特征码" ,required=true ,dataType="byte[]")
public byte[] code2;
}
/**
* argClass-3
* wrap arguments for method {@link #compareCodes(CompareCodesArgs)}
*/
public static class CompareCodesArgs{
@ApiModelProperty(value ="待比对的特征码" ,required=true ,dataType="byte[]")
public byte[] code1;
@ApiModelProperty(value ="包含人脸特征的{@link CodeInfo }数组" ,required=true ,dataType="CodeInfo[]")
public CodeInfo[] codes;
}
/**
* argClass-4
* wrap arguments for method {@link #compareFaces(CompareFacesArgs)}
*/
public static class CompareFacesArgs{
@ApiModelProperty(value ="人脸特征数据" ,required=true ,dataType="byte[]")
public byte[] code;
@ApiModelProperty(value ="待比对的图像(jpg,png...)(可能有多张人脸)" ,required=true ,dataType="byte[]")
public byte[] imgData;
@ApiModelProperty(value ="参见 {@link #getCodeInfo(byte[], int, CodeInfo[])}" ,required=true ,dataType="int")
public int faceNum;
}
/**
* argClass-5
* wrap arguments for method {@link #compareFeatures(CompareFeaturesArgs)}
*/
public static class CompareFeaturesArgs{
@ApiModelProperty(value ="人脸特征数据" ,required=true ,dataType="byte[]")
public byte[] code1;
@ApiModelProperty(value ="一组人脸特征" ,required=true ,dataType="List")
public List codes;
}
/**
* argClass-6
* wrap arguments for method {@link #detectAndCompare2Face(DetectAndCompare2FaceArgs)}
*/
public static class DetectAndCompare2FaceArgs{
@ApiModelProperty(value ="图像1数据(jpg,png...)字节数组,为{@code null}则抛出{@link IllegalArgumentException}" ,required=true ,dataType="byte[]")
public byte[] imgData1;
@ApiModelProperty(value ="图片检测范围,为null时全图检测" ,required=true ,dataType="FRect")
public FRect detectRect1;
@ApiModelProperty(value ="图像2数据(jpg,png...)字节数组" ,required=true ,dataType="byte[]")
public byte[] imgData2;
@ApiModelProperty(value ="图片检测范围,为null时全图检测" ,required=true ,dataType="FRect")
public FRect detectRect2;
}
/**
* argClass-7
* wrap arguments for method {@link #detectAndGetCodeInfo(DetectAndGetCodeInfoArgs)}
*/
public static class DetectAndGetCodeInfoArgs{
@ApiModelProperty(value ="图像数据(jpg,png...)字节数组,为{@code null}则抛出{@link IllegalArgumentException}" ,required=true ,dataType="byte[]")
public byte[] imgData;
@ApiModelProperty(value ="参见 {@link #getCodeInfo(byte[], int, CodeInfo[])}" ,required=true ,dataType="int")
public int faceNum;
}
/**
* argClass-8
* wrap arguments for method {@link #detectCenterFace(DetectCenterFaceArgs)}
*/
public static class DetectCenterFaceArgs{
@ApiModelProperty(value ="图像数据(jpg,png...)字节数组,为{@code null}则抛出{@link IllegalArgumentException}" ,required=true ,dataType="byte[]")
public byte[] imgData;
}
/**
* argClass-9
* wrap arguments for method {@link #detectFace(DetectFaceArgs)}
*/
public static class DetectFaceArgs{
@ApiModelProperty(value ="图像数据(jpg,png...)字节数组,为{@code null}则抛出{@link IllegalArgumentException}" ,required=true ,dataType="byte[]")
public byte[] imgData;
}
/**
* argClass-10
* wrap arguments for method {@link #detectMaxFace(DetectMaxFaceArgs)}
*/
public static class DetectMaxFaceArgs{
@ApiModelProperty(value ="图像数据(jpg,png...)字节数组,为{@code null}则抛出{@link IllegalArgumentException}" ,required=true ,dataType="byte[]")
public byte[] imgData;
}
/**
* argClass-11
* wrap arguments for method {@link #getCodeInfo(GetCodeInfoArgs)}
*/
public static class GetCodeInfoArgs{
@ApiModelProperty(value ="图像数据(jpg,png...)字节数组,为{@code null}则抛出 {@link IllegalArgumentException}" ,required=true ,dataType="byte[]")
public byte[] imgData;
@ApiModelProperty(value ="要求返回的人脸特征码数目
\n"
+" 大于0时,如果实际提取到的人脸特征码数目不等于{@code faceNum},则抛出{@link NotFaceDetectedException}
\n"
+" 小于等于0时,返回所有提取到到人脸特征码" ,required=true ,dataType="int")
public int faceNum;
@ApiModelProperty(value ="检测到的人脸位置对象列表
\n"
+" 为{@code null}或数组长度为0时抛出{@link IllegalArgumentException}
\n"
+" 如果元素为{@code null},则跳过
" ,required=true ,dataType="CodeInfo[]")
public CodeInfo[] facePos;
}
/**
* argClass-12
* wrap arguments for method {@link #getCodeInfo(GetCodeInfoSingleArgs)}
*/
public static class GetCodeInfoSingleArgs{
@ApiModelProperty(value ="图像数据(jpg,png...)字节数组,为{@code null}则抛出 {@link IllegalArgumentException}" ,required=true ,dataType="byte[]")
public byte[] imgData;
@ApiModelProperty(value ="人脸位置信息对象,为{@code null}则抛出异常" ,required=true ,dataType="CodeInfo")
public CodeInfo facePos;
}
/**
* argClass-13
* wrap arguments for method {@link #getFeature(GetFeatureArgs)}
*/
public static class GetFeatureArgs{
@ApiModelProperty(value ="人脸图像数据(jpg,png...)与人脸位置信息对象的映射" ,required=true ,dataType="Map")
public Map faces;
}
/**
* argClass-14
* wrap arguments for method {@link #hasFace(HasFaceArgs)}
*/
public static class HasFaceArgs{
@ApiModelProperty(value ="图像数据(jpg,png...)字节数组,为{@code null}则抛出{@link IllegalArgumentException}" ,required=true ,dataType="byte[]")
public byte[] imgData;
}
/**
* argClass-16
* wrap arguments for method {@link #matDetectAndGetCodeInfo(MatDetectAndGetCodeInfoArgs)}
*/
public static class MatDetectAndGetCodeInfoArgs{
@ApiModelProperty(value ="图像矩阵类型" ,required=true ,dataType="MatType")
public MatType matType;
@ApiModelProperty(value ="图像矩阵" ,required=true ,dataType="byte[]")
public byte[] matData;
@ApiModelProperty(value ="图像宽度" ,required=true ,dataType="int")
public int width;
@ApiModelProperty(value ="图像高度" ,required=true ,dataType="int")
public int height;
@ApiModelProperty(value ="参见 {@link #getCodeInfo(byte[], int, CodeInfo[])}" ,required=true ,dataType="int")
public int faceNum;
}
/**
* argClass-17
* wrap arguments for method {@link #matDetectFace(MatDetectFaceArgs)}
*/
public static class MatDetectFaceArgs{
@ApiModelProperty(value ="图像矩阵类型" ,required=true ,dataType="MatType")
public MatType matType;
@ApiModelProperty(value ="图像矩阵" ,required=true ,dataType="byte[]")
public byte[] matData;
@ApiModelProperty(value ="图像宽度" ,required=true ,dataType="int")
public int width;
@ApiModelProperty(value ="图像高度" ,required=true ,dataType="int")
public int height;
}
/**
* argClass-18
* wrap arguments for method {@link #matDetectMaxFace(MatDetectMaxFaceArgs)}
*/
public static class MatDetectMaxFaceArgs{
@ApiModelProperty(value ="图像高度" ,required=true ,dataType="MatType")
public MatType matType;
@ApiModelProperty(value ="图像高度" ,required=true ,dataType="byte[]")
public byte[] matData;
@ApiModelProperty(value ="图像高度" ,required=true ,dataType="int")
public int width;
@ApiModelProperty(value ="图像高度" ,required=true ,dataType="int")
public int height;
}
/**
* argClass-19
* wrap arguments for method {@link #matGetCodeInfo(MatGetCodeInfoArgs)}
*/
public static class MatGetCodeInfoArgs{
@ApiModelProperty(value ="图像矩阵类型" ,required=true ,dataType="MatType")
public MatType matType;
@ApiModelProperty(value ="图像矩阵" ,required=true ,dataType="byte[]")
public byte[] matData;
@ApiModelProperty(value ="图像宽度" ,required=true ,dataType="int")
public int width;
@ApiModelProperty(value ="图像高度" ,required=true ,dataType="int")
public int height;
@ApiModelProperty(value ="要求返回的人脸特征码数目
\n"
+" 大于0时,如果实际提取到的人脸特征码数目不等于{@code faceNum},则抛出{@link NotFaceDetectedException}
\n"
+" 小于等于0时,返回所有提取到到人脸特征码" ,required=true ,dataType="int")
public int facenum;
@ApiModelProperty(value ="人脸位置信息对象,不可为{@code null}" ,required=true ,dataType="CodeInfo[]")
public CodeInfo[] facePos;
}
/**
* argClass-20
* wrap arguments for method {@link #matGetCodeInfo(MatGetCodeInfoSingleArgs)}
*/
public static class MatGetCodeInfoSingleArgs{
@ApiModelProperty(value ="图像矩阵类型" ,required=true ,dataType="MatType")
public MatType matType;
@ApiModelProperty(value ="图像矩阵" ,required=true ,dataType="byte[]")
public byte[] matData;
@ApiModelProperty(value ="图像宽度" ,required=true ,dataType="int")
public int width;
@ApiModelProperty(value ="图像高度" ,required=true ,dataType="int")
public int height;
@ApiModelProperty(value ="人脸位置信息对象,为{@code null}则抛出异常" ,required=true ,dataType="CodeInfo")
public CodeInfo facePos;
}
/**
* argClass-21
* wrap arguments for method {@link #matHasFace(MatHasFaceArgs)}
*/
public static class MatHasFaceArgs{
@ApiModelProperty(value ="图像矩阵类型" ,required=true ,dataType="MatType")
public MatType matType;
@ApiModelProperty(value ="图像矩阵,为{@code null}则抛出{@link IllegalArgumentException}" ,required=true ,dataType="byte[]")
public byte[] matData;
@ApiModelProperty(value ="图像宽度" ,required=true ,dataType="int")
public int width;
@ApiModelProperty(value ="图像高度" ,required=true ,dataType="int")
public int height;
}
/**
* argClass-22
* wrap arguments for method {@link #matSearchFaces(MatSearchFacesArgs)}
*/
public static class MatSearchFacesArgs{
@ApiModelProperty(value ="图像矩阵类型" ,required=true ,dataType="MatType")
public MatType matType;
@ApiModelProperty(value ="图像矩阵" ,required=true ,dataType="byte[]")
public byte[] matData;
@ApiModelProperty(value ="图像宽度" ,required=true ,dataType="int")
public int width;
@ApiModelProperty(value ="图像宽度" ,required=true ,dataType="int")
public int height;
@ApiModelProperty(value ="人脸位置对象
\n"
+" 为{@code null}时,先做人脸检测再提取特征码
\n"
+" 不为{@code null}时,直接在指定的位置提取特征码
" ,required=true ,dataType="CodeInfo")
public CodeInfo facePos;
@ApiModelProperty(value ="相似度阀值" ,required=true ,dataType="double")
public double similarty;
@ApiModelProperty(value ="最多返回的记录数目" ,required=true ,dataType="int")
public int rows;
}
/**
* argClass-23
* wrap arguments for method {@link #matWearMask(MatWearMaskArgs)}
*/
public static class MatWearMaskArgs{
@ApiModelProperty(value ="图像矩阵类型" ,required=true ,dataType="MatType")
public MatType matType;
@ApiModelProperty(value ="图像矩阵" ,required=true ,dataType="byte[]")
public byte[] matData;
@ApiModelProperty(value ="图像宽度" ,required=true ,dataType="int")
public int width;
@ApiModelProperty(value ="图像高度" ,required=true ,dataType="int")
public int height;
@ApiModelProperty(value ="人脸位置信息" ,required=true ,dataType="CodeInfo")
public CodeInfo faceInfo;
}
/**
* argClass-25
* wrap arguments for method {@link #searchFaces(SearchFacesArgs)}
*/
public static class SearchFacesArgs{
@ApiModelProperty(value ="图片字节数组" ,required=true ,dataType="byte[]")
public byte[] imgData;
@ApiModelProperty(value ="人脸位置对象
\n"
+" 为{@code null}时,先做人脸检测再提取特征码
\n"
+" 不为{@code null}时,直接在指定的位置提取特征码
" ,required=true ,dataType="CodeInfo")
public CodeInfo facePos;
@ApiModelProperty(value ="相似度阀值" ,required=true ,dataType="double")
public double similarty;
@ApiModelProperty(value ="最多返回的记录数目" ,required=true ,dataType="int")
public int rows;
}
/**
* argClass-26
* wrap arguments for method {@link #searchFeatures(SearchFeaturesArgs)}
*/
public static class SearchFeaturesArgs{
@ApiModelProperty(value ="要搜索的特征码" ,required=true ,dataType="byte[]")
public byte[] feature;
@ApiModelProperty(value ="相似度阀值" ,required=true ,dataType="double")
public double similarty;
@ApiModelProperty(value ="最多返回的记录数目" ,required=true ,dataType="int")
public int rows;
}
/**
* argClass-27
* wrap arguments for method {@link #wearMask(WearMaskArgs)}
*/
public static class WearMaskArgs{
@ApiModelProperty(value ="图像数据(jpg,png...)字节数组,为{@code null}则抛出 {@link IllegalArgumentException}" ,required=true ,dataType="byte[]")
public byte[] imgData;
@ApiModelProperty(value ="可见光图像人脸信息" ,required=true ,dataType="CodeInfo")
public CodeInfo faceInfo;
}
/**
* 获取{@link FaceApi}实例的接口,
* 用于应用层SPI方式提供{@link FaceApi}实例
* @author guyadong
*
*/
public static interface InstanceSupplier{
FaceApi instanceOfFaceApi();
}
/**
* web响应数据接口
* @author guyadong
*
*/
public static interface Response{
/**
* 接口方法调用成功
* @param result 调用返回值
*/
void onComplete(Object result);
/**
* 接口方法调用成功,调用方法返回类型为void
*/
void onComplete();
/**
* 接口方法调用抛出异常
* @param e 异常
*/
void onError(Exception e);
}
/**
* 获取{@link Response}接口实例的工厂类接口
* @author guyadong
*
*/
public static interface ResponseFactory{
/**
* @return 返回新的{@link Response}接口实例
*/
Response newFaceApiResponse();
}
/**
* {@link Response}默认实现
* @author guyadong
*
*/
public static class DefaultResponse implements Response{
private static boolean outStrackTrace = false;
private boolean success;
/** RPC调用的返回值 */
private Object result;
/** 异常信息 */
private String errorMessage;
/** 异常堆栈信息 */
private String stackTrace;
@Override
public void onComplete(Object result) {
this.success = true;
this.result = result;
}
@Override
public void onComplete() {
onComplete(null);
}
@Override
public void onError(Exception e) {
success = false;
errorMessage = e.getMessage();
if(errorMessage == null){
errorMessage = e.getClass().getSimpleName();
}
if(outStrackTrace){
StringWriter writer = new StringWriter();
e.printStackTrace(new PrintWriter(writer));
stackTrace = writer.toString();
}
}
public boolean isSuccess() {
return success;
}
public void setSuccess(boolean success) {
this.success = success;
}
public Object getResult() {
return result;
}
public void setResult(Object result) {
this.result = result;
}
public String getErrorMessage() {
return errorMessage;
}
public void setErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
}
public String getStackTrace() {
return stackTrace;
}
public void setStackTrace(String stackTrace) {
this.stackTrace = stackTrace;
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append("DefaultResponse [success=");
builder.append(success);
builder.append(", ");
if (result != null) {
builder.append("result=");
builder.append(result);
builder.append(", ");
}
if (errorMessage != null) {
builder.append("errorMessage=");
builder.append(errorMessage);
builder.append(", ");
}
if (stackTrace != null) {
builder.append("stackTrace=");
builder.append(stackTrace);
}
builder.append("]");
return builder.toString();
}
/**
* 开启输出堆栈信息(默认为不开启)
* 开发时为了调试需要获取详细的异常堆栈信息可以开启
* @param outStrackTrace 要设置的 outStrackTrace
*/
public static void enableStrackTrace() {
outStrackTrace = true;
}
}
/**
* {@link ResponseFactory}接口默认实现
* @author guyadong
*
*/
public static class DefaultResponseFactory implements ResponseFactory{
@Override
public Response newFaceApiResponse() {
return new DefaultResponse();
}
}
public static String DESCRIPTION = "人脸识别SDK核心接口
\n"
+" 此接口中mat前缀的方法图像数据参数为图像矩阵(要求图像宽高必须为偶数),\n"
+" 其他方法中所有byte[]类型的图像参数都指未解码的图像格式(如jpg,bmp,png...),
\n"
+" 目前支持的图像格式类型依赖于JDK的支持能力";
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy