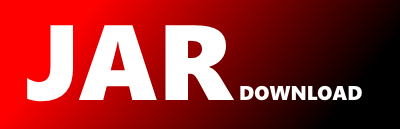
net.gdface.sdk.fse.thrift.FeatureSeThriftClientAsync Maven / Gradle / Ivy
// ______________________________________________________
// Generated by codegen - https://gitee.com/l0km/codegen
// template: thrift/client/perservice/client.async.decorator.class.vm
// ______________________________________________________
package net.gdface.sdk.fse.thrift;
import net.gdface.sdk.fse.CodeBean;
import net.gdface.sdk.fse.FeatureSe;
import net.gdface.thrift.TypeTransformer;
import com.google.common.net.HostAndPort;
import com.google.common.util.concurrent.FutureCallback;
import com.google.common.util.concurrent.ListenableFuture;
import net.gdface.thrift.ClientFactory;
import static com.google.common.base.Preconditions.*;
/**
* 基于thrift/swift框架生成的client端代码提供{@link FeatureSe}接口的异步RPC实现(线程安全)
* 转发所有{@link FeatureSe}接口方法到{@link #delegate()}指定的实例
* 所有服务端抛出的{@link RuntimeException}异常被封装到{@link ServiceRuntimeException}中抛出
* Example:
*
* FeatureSeThriftClientAsync thriftInstance = ClientFactory
* .builder()
* .setHostAndPort("127.0.0.1",26413)
* .build(FeatureSe.class, FeatureSeThriftClientAsync.class);
*
* 计算机生成代码(generated by automated tools ThriftServiceDecoratorGenerator @author guyadong)
* @author guyadong
*
*/
public class FeatureSeThriftClientAsync {
private final ClientFactory factory;
public ClientFactory getFactory() {
return factory;
}
public FeatureSeThriftClientAsync(ClientFactory factory) {
super();
this.factory = checkNotNull(factory,"factory is null");
}
/**
* @param host RPC service host
* @param port RPC service port
*/
public FeatureSeThriftClientAsync(String host,int port) {
this(ClientFactory.builder().setHostAndPort(host,port));
}
/**
* @param hostAndPort RPC service host and port
*/
public FeatureSeThriftClientAsync(HostAndPort hostAndPort) {
this(ClientFactory.builder().setHostAndPort(hostAndPort));
}
/**
* test if connectable for RPC service
* @return return {@code true} if connectable ,otherwise {@code false}
*/
public boolean testConnect(){
return factory.testConnect();
}
/**
* @return 返回{@link net.gdface.sdk.fse.thrift.client.FeatureSe.Async}实例
*/
protected net.gdface.sdk.fse.thrift.client.FeatureSe.Async delegate() {
return factory.applyInstance(net.gdface.sdk.fse.thrift.client.FeatureSe.Async.class);
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append("FeatureSeThriftClientAsync [factory=");
builder.append(factory);
builder.append(",interface=");
builder.append(FeatureSe.class.getName());
builder.append("]");
return builder.toString();
}
/**
* 默认的{@link FutureCallback}实现
* @author guyadong
*
* @param
*/
public static class DefaultCallback implements FutureCallback{
@Override
public void onSuccess(V result) {
// DO NOTHING
}
@Override
public void onFailure(Throwable t) {
try{
throw t;
}
catch(net.gdface.sdk.fse.thrift.client.ServiceRuntimeException e){
onServiceRuntimeException(e);
}
catch(Throwable e){
onThrowable(e);
}
}
protected void onServiceRuntimeException(net.gdface.sdk.fse.thrift.client.ServiceRuntimeException e){
System.out.println(e.getServiceStackTraceMessage());
}
protected void onThrowable(Throwable e){
e.printStackTrace();
}
}
/**
* see also {@link net.gdface.sdk.fse.FeatureSe#addFeature(byte[],byte[],java.lang.String,int)}
*/
public ListenableFuture addFeature(byte[] featureId,
byte[] feature,
String imgMD5,
int group){
net.gdface.sdk.fse.thrift.client.FeatureSe.Async async = delegate();
ListenableFuture future = async.addFeatureToFse(featureId,
feature,
imgMD5,
group);
return factory.wrap(async,future);
}
public void addFeature(byte[] featureId,
byte[] feature,
String imgMD5,
int group,
FutureCallbackcallback){
factory.addCallback(addFeature(featureId,feature,imgMD5,group), callback);
}
/**
* see also {@link net.gdface.sdk.fse.FeatureSe#addFeature(byte[],byte[],long,int)}
*/
public ListenableFuture addFeature(byte[] featureId,
byte[] feature,
long appid,
int group){
net.gdface.sdk.fse.thrift.client.FeatureSe.Async async = delegate();
ListenableFuture future = async.addFeatureToFseWithAppId(featureId,
feature,
appid,
group);
return factory.wrap(async,future);
}
public void addFeature(byte[] featureId,
byte[] feature,
long appid,
int group,
FutureCallbackcallback){
factory.addCallback(addFeature(featureId,feature,appid,group), callback);
}
/**
* see also {@link net.gdface.sdk.fse.FeatureSe#clearAll()}
*/
public ListenableFuture clearAll(){
net.gdface.sdk.fse.thrift.client.FeatureSe.Async async = delegate();
ListenableFuture future = async.clearAllOfFse();
return factory.wrap(async,future);
}
public void clearAll(FutureCallbackcallback){
factory.addCallback(clearAll(), callback);
}
/**
* see also {@link net.gdface.sdk.fse.FeatureSe#getFeature(byte[])}
*/
public ListenableFuture getFeature(byte[] featureId){
net.gdface.sdk.fse.thrift.client.FeatureSe.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.getFeatureFromFse(featureId),
new com.google.common.base.Function(){
@Override
public CodeBean apply(net.gdface.sdk.fse.thrift.client.CodeBean input) {
return TypeTransformer.getInstance().to(
input,
net.gdface.sdk.fse.thrift.client.CodeBean.class,
CodeBean.class);
}
});
return factory.wrap(async,future);
}
public void getFeature(byte[] featureId,
FutureCallbackcallback){
factory.addCallback(getFeature(featureId), callback);
}
/**
* see also {@link net.gdface.sdk.fse.FeatureSe#getFeatureByHex(java.lang.String)}
*/
public ListenableFuture getFeatureByHex(String featureId){
net.gdface.sdk.fse.thrift.client.FeatureSe.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.getFeatureByHexFromFse(featureId),
new com.google.common.base.Function(){
@Override
public CodeBean apply(net.gdface.sdk.fse.thrift.client.CodeBean input) {
return TypeTransformer.getInstance().to(
input,
net.gdface.sdk.fse.thrift.client.CodeBean.class,
CodeBean.class);
}
});
return factory.wrap(async,future);
}
public void getFeatureByHex(String featureId,
FutureCallbackcallback){
factory.addCallback(getFeatureByHex(featureId), callback);
}
/**
* see also {@link net.gdface.sdk.fse.FeatureSe#removeFeature(byte[])}
*/
public ListenableFuture removeFeature(byte[] featureId){
net.gdface.sdk.fse.thrift.client.FeatureSe.Async async = delegate();
ListenableFuture future = async.removeFeatureFromFse(featureId);
return factory.wrap(async,future);
}
public void removeFeature(byte[] featureId,
FutureCallbackcallback){
factory.addCallback(removeFeature(featureId), callback);
}
/**
* see also {@link net.gdface.sdk.fse.FeatureSe#removeFeatureByHex(java.lang.String)}
*/
public ListenableFuture removeFeatureByHex(String featureId){
net.gdface.sdk.fse.thrift.client.FeatureSe.Async async = delegate();
ListenableFuture future = async.removeFeatureByHexFromFse(featureId);
return factory.wrap(async,future);
}
public void removeFeatureByHex(String featureId,
FutureCallbackcallback){
factory.addCallback(removeFeatureByHex(featureId), callback);
}
/**
* see also {@link net.gdface.sdk.fse.FeatureSe#searchCode(byte[],double,int,java.lang.String[],int)}
*/
public ListenableFuture searchCode(byte[] code,
double sim,
int rows,
String[] imgMD5Set,
int group){
net.gdface.sdk.fse.thrift.client.FeatureSe.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.searchCodeFromFse(code,
sim,
rows,
TypeTransformer.getInstance().to(
imgMD5Set,
String.class,
String.class),
group),
new com.google.common.base.Function,CodeBean[]>(){
@Override
public CodeBean[] apply(java.util.List input) {
return TypeTransformer.getInstance().toArray(
input,
net.gdface.sdk.fse.thrift.client.CodeBean.class,
CodeBean.class);
}
});
return factory.wrap(async,future);
}
public void searchCode(byte[] code,
double sim,
int rows,
String[] imgMD5Set,
int group,
FutureCallbackcallback){
factory.addCallback(searchCode(code,sim,rows,imgMD5Set,group), callback);
}
/**
* see also {@link net.gdface.sdk.fse.FeatureSe#size()}
*/
public ListenableFuture size(){
net.gdface.sdk.fse.thrift.client.FeatureSe.Async async = delegate();
ListenableFuture future = async.sizeOfFse();
return factory.wrap(async,future);
}
public void size(FutureCallbackcallback){
factory.addCallback(size(), callback);
}
/**
* see also {@link net.gdface.sdk.fse.FeatureSe#updateGroup(byte[],int)}
*/
public ListenableFuture updateGroup(byte[] featureId,
int group){
net.gdface.sdk.fse.thrift.client.FeatureSe.Async async = delegate();
ListenableFuture future = async.updateGroup(featureId,
group);
return factory.wrap(async,future);
}
public void updateGroup(byte[] featureId,
int group,
FutureCallbackcallback){
factory.addCallback(updateGroup(featureId,group), callback);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy