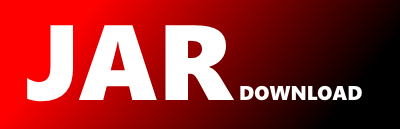
net.gdface.sdk.fse.thrift.client.FeatureSe Maven / Gradle / Ivy
package net.gdface.sdk.fse.thrift.client;
import com.facebook.swift.codec.*;
import com.facebook.swift.codec.ThriftField.Requiredness;
import com.facebook.swift.service.*;
import com.google.common.util.concurrent.ListenableFuture;
import java.io.*;
import java.util.*;
@ThriftService("FeatureSe")
public interface FeatureSe
{
@ThriftService("FeatureSe")
public interface Async
{
@ThriftMethod(value = "addFeatureToFse",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture addFeatureToFse(
@ThriftField(value=1, name="featureId", requiredness=Requiredness.OPTIONAL) final byte [] featureId,
@ThriftField(value=2, name="feature", requiredness=Requiredness.OPTIONAL) final byte [] feature,
@ThriftField(value=3, name="imgMD5", requiredness=Requiredness.OPTIONAL) final String imgMD5,
@ThriftField(value=4, name="group", requiredness=Requiredness.REQUIRED) final int group
);
@ThriftMethod(value = "addFeatureToFseWithAppId",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture addFeatureToFseWithAppId(
@ThriftField(value=1, name="featureId", requiredness=Requiredness.OPTIONAL) final byte [] featureId,
@ThriftField(value=2, name="feature", requiredness=Requiredness.OPTIONAL) final byte [] feature,
@ThriftField(value=3, name="appid", requiredness=Requiredness.REQUIRED) final long appid,
@ThriftField(value=4, name="group", requiredness=Requiredness.REQUIRED) final int group
);
@ThriftMethod(value = "clearAllOfFse",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture clearAllOfFse();
@ThriftMethod(value = "getFeatureByHexFromFse",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture getFeatureByHexFromFse(
@ThriftField(value=1, name="featureId", requiredness=Requiredness.OPTIONAL) final String featureId
);
@ThriftMethod(value = "getFeatureFromFse",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture getFeatureFromFse(
@ThriftField(value=1, name="featureId", requiredness=Requiredness.OPTIONAL) final byte [] featureId
);
@ThriftMethod(value = "removeFeatureByHexFromFse",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture removeFeatureByHexFromFse(
@ThriftField(value=1, name="featureId", requiredness=Requiredness.OPTIONAL) final String featureId
);
@ThriftMethod(value = "removeFeatureFromFse",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture removeFeatureFromFse(
@ThriftField(value=1, name="featureId", requiredness=Requiredness.OPTIONAL) final byte [] featureId
);
@ThriftMethod(value = "searchCodeFromFse",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture> searchCodeFromFse(
@ThriftField(value=1, name="code", requiredness=Requiredness.OPTIONAL) final byte [] code,
@ThriftField(value=2, name="sim", requiredness=Requiredness.REQUIRED) final double sim,
@ThriftField(value=3, name="rows", requiredness=Requiredness.REQUIRED) final int rows,
@ThriftField(value=4, name="imgMD5Set", requiredness=Requiredness.OPTIONAL) final List imgMD5Set,
@ThriftField(value=5, name="group", requiredness=Requiredness.REQUIRED) final int group
);
@ThriftMethod(value = "sizeOfFse",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture sizeOfFse();
@ThriftMethod(value = "updateGroup",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture updateGroup(
@ThriftField(value=1, name="featureId", requiredness=Requiredness.OPTIONAL) final byte [] featureId,
@ThriftField(value=2, name="group", requiredness=Requiredness.REQUIRED) final int group
);
}
@ThriftMethod(value = "addFeatureToFse",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
boolean addFeatureToFse(
@ThriftField(value=1, name="featureId", requiredness=Requiredness.OPTIONAL) final byte [] featureId,
@ThriftField(value=2, name="feature", requiredness=Requiredness.OPTIONAL) final byte [] feature,
@ThriftField(value=3, name="imgMD5", requiredness=Requiredness.OPTIONAL) final String imgMD5,
@ThriftField(value=4, name="group", requiredness=Requiredness.REQUIRED) final int group
) throws ServiceRuntimeException;
@ThriftMethod(value = "addFeatureToFseWithAppId",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
boolean addFeatureToFseWithAppId(
@ThriftField(value=1, name="featureId", requiredness=Requiredness.OPTIONAL) final byte [] featureId,
@ThriftField(value=2, name="feature", requiredness=Requiredness.OPTIONAL) final byte [] feature,
@ThriftField(value=3, name="appid", requiredness=Requiredness.REQUIRED) final long appid,
@ThriftField(value=4, name="group", requiredness=Requiredness.REQUIRED) final int group
) throws ServiceRuntimeException;
@ThriftMethod(value = "clearAllOfFse",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
void clearAllOfFse() throws ServiceRuntimeException;
@ThriftMethod(value = "getFeatureByHexFromFse",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
CodeBean getFeatureByHexFromFse(
@ThriftField(value=1, name="featureId", requiredness=Requiredness.OPTIONAL) final String featureId
) throws ServiceRuntimeException;
@ThriftMethod(value = "getFeatureFromFse",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
CodeBean getFeatureFromFse(
@ThriftField(value=1, name="featureId", requiredness=Requiredness.OPTIONAL) final byte [] featureId
) throws ServiceRuntimeException;
@ThriftMethod(value = "removeFeatureByHexFromFse",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
boolean removeFeatureByHexFromFse(
@ThriftField(value=1, name="featureId", requiredness=Requiredness.OPTIONAL) final String featureId
) throws ServiceRuntimeException;
@ThriftMethod(value = "removeFeatureFromFse",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
boolean removeFeatureFromFse(
@ThriftField(value=1, name="featureId", requiredness=Requiredness.OPTIONAL) final byte [] featureId
) throws ServiceRuntimeException;
@ThriftMethod(value = "searchCodeFromFse",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
List searchCodeFromFse(
@ThriftField(value=1, name="code", requiredness=Requiredness.OPTIONAL) final byte [] code,
@ThriftField(value=2, name="sim", requiredness=Requiredness.REQUIRED) final double sim,
@ThriftField(value=3, name="rows", requiredness=Requiredness.REQUIRED) final int rows,
@ThriftField(value=4, name="imgMD5Set", requiredness=Requiredness.OPTIONAL) final List imgMD5Set,
@ThriftField(value=5, name="group", requiredness=Requiredness.REQUIRED) final int group
) throws ServiceRuntimeException;
@ThriftMethod(value = "sizeOfFse",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
int sizeOfFse() throws ServiceRuntimeException;
@ThriftMethod(value = "updateGroup",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
boolean updateGroup(
@ThriftField(value=1, name="featureId", requiredness=Requiredness.OPTIONAL) final byte [] featureId,
@ThriftField(value=2, name="group", requiredness=Requiredness.REQUIRED) final int group
) throws ServiceRuntimeException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy