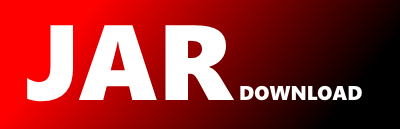
net.gdface.sdk.thrift.FaceApiThriftClientAsync Maven / Gradle / Ivy
// ______________________________________________________
// Generated by codegen - https://gitee.com/l0km/codegen
// template: thrift/client/perservice/client.async.decorator.class.vm
// ______________________________________________________
package net.gdface.sdk.thrift;
import java.nio.ByteBuffer;
import java.util.List;
import java.util.Map;
import net.gdface.image.MatType;
import net.gdface.sdk.CodeInfo;
import net.gdface.sdk.CompareResult;
import net.gdface.sdk.FRect;
import net.gdface.sdk.FaceApi;
import net.gdface.sdk.FseResult;
import net.gdface.thrift.TypeTransformer;
import com.google.common.net.HostAndPort;
import com.google.common.util.concurrent.FutureCallback;
import com.google.common.util.concurrent.ListenableFuture;
import net.gdface.thrift.ClientFactory;
import static com.google.common.base.Preconditions.*;
/**
* 基于thrift/swift框架生成的client端代码提供{@link FaceApi}接口的异步RPC实现(线程安全)
* 转发所有{@link FaceApi}接口方法到{@link #delegate()}指定的实例
* 所有服务端抛出的{@link RuntimeException}异常被封装到{@link ServiceRuntimeException}中抛出
* Example:
*
* FaceApiThriftClientAsync thriftInstance = ClientFactory
* .builder()
* .setHostAndPort("127.0.0.1",26413)
* .build(FaceApi.class, FaceApiThriftClientAsync.class);
*
* 计算机生成代码(generated by automated tools ThriftServiceDecoratorGenerator @author guyadong)
* @author guyadong
*
*/
public class FaceApiThriftClientAsync {
private final ClientFactory factory;
public ClientFactory getFactory() {
return factory;
}
public FaceApiThriftClientAsync(ClientFactory factory) {
super();
this.factory = checkNotNull(factory,"factory is null");
}
/**
* @param host RPC service host
* @param port RPC service port
*/
public FaceApiThriftClientAsync(String host,int port) {
this(ClientFactory.builder().setHostAndPort(host,port));
}
/**
* @param hostAndPort RPC service host and port
*/
public FaceApiThriftClientAsync(HostAndPort hostAndPort) {
this(ClientFactory.builder().setHostAndPort(hostAndPort));
}
/**
* test if connectable for RPC service
* @return return {@code true} if connectable ,otherwise {@code false}
*/
public boolean testConnect(){
return factory.testConnect();
}
/**
* @return 返回{@link net.gdface.sdk.thrift.client.FaceApi.Async}实例
*/
protected net.gdface.sdk.thrift.client.FaceApi.Async delegate() {
return factory.applyInstance(net.gdface.sdk.thrift.client.FaceApi.Async.class);
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append("FaceApiThriftClientAsync [factory=");
builder.append(factory);
builder.append(",interface=");
builder.append(FaceApi.class.getName());
builder.append("]");
return builder.toString();
}
/**
* 默认的{@link FutureCallback}实现
* @author guyadong
*
* @param
*/
public static class DefaultCallback implements FutureCallback{
@Override
public void onSuccess(V result) {
// DO NOTHING
}
@Override
public void onFailure(Throwable t) {
try{
throw t;
}
catch(net.gdface.sdk.thrift.client.ImageErrorException e){
onImageErrorException(e);
}
catch(net.gdface.sdk.thrift.client.NotFaceDetectedException e){
onNotFaceDetectedException(e);
}
catch(net.gdface.sdk.thrift.client.ServiceRuntimeException e){
onServiceRuntimeException(e);
}
catch(Throwable e){
onThrowable(e);
}
}
protected void onImageErrorException(net.gdface.sdk.thrift.client.ImageErrorException e){
System.out.println(e.getServiceStackTraceMessage());
}
protected void onNotFaceDetectedException(net.gdface.sdk.thrift.client.NotFaceDetectedException e){
System.out.println(e.getServiceStackTraceMessage());
}
protected void onServiceRuntimeException(net.gdface.sdk.thrift.client.ServiceRuntimeException e){
System.out.println(e.getServiceStackTraceMessage());
}
protected void onThrowable(Throwable e){
e.printStackTrace();
}
}
/**
* see also {@link net.gdface.sdk.FaceApi#compare2Face(byte[],net.gdface.sdk.CodeInfo,byte[],net.gdface.sdk.CodeInfo)}
*/
public ListenableFuture compare2Face(byte[] imgData1,
CodeInfo facePos1,
byte[] imgData2,
CodeInfo facePos2){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = async.compare2Face(imgData1,
TypeTransformer.getInstance().to(
facePos1,
CodeInfo.class,
net.gdface.sdk.thrift.client.CodeInfo.class),
imgData2,
TypeTransformer.getInstance().to(
facePos2,
CodeInfo.class,
net.gdface.sdk.thrift.client.CodeInfo.class));
return factory.wrap(async,future);
}
public void compare2Face(byte[] imgData1,
CodeInfo facePos1,
byte[] imgData2,
CodeInfo facePos2,
FutureCallbackcallback){
factory.addCallback(compare2Face(imgData1,facePos1,imgData2,facePos2), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#compareCode(byte[],byte[])}
*/
public ListenableFuture compareCode(byte[] code1,
byte[] code2){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = async.compareCode(code1,
code2);
return factory.wrap(async,future);
}
public void compareCode(byte[] code1,
byte[] code2,
FutureCallbackcallback){
factory.addCallback(compareCode(code1,code2), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#compareCodes(byte[],net.gdface.sdk.CodeInfo[])}
*/
public ListenableFuture compareCodes(byte[] code1,
CodeInfo[] codes){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.compareCodes(code1,
TypeTransformer.getInstance().to(
codes,
CodeInfo.class,
net.gdface.sdk.thrift.client.CodeInfo.class)),
new com.google.common.base.Function,double[]>(){
@Override
public double[] apply(java.util.List input) {
return TypeTransformer.getInstance().todoubleArray(
input,
double.class,
double.class);
}
});
return factory.wrap(async,future);
}
public void compareCodes(byte[] code1,
CodeInfo[] codes,
FutureCallbackcallback){
factory.addCallback(compareCodes(code1,codes), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#compareFaces(byte[],byte[],int)}
*/
public ListenableFuture compareFaces(byte[] code,
byte[] imgData,
int faceNum){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.compareFaces(code,
imgData,
faceNum),
new com.google.common.base.Function(){
@Override
public CompareResult apply(net.gdface.sdk.thrift.client.CompareResult input) {
return TypeTransformer.getInstance().to(
input,
net.gdface.sdk.thrift.client.CompareResult.class,
CompareResult.class);
}
});
return factory.wrap(async,future);
}
public void compareFaces(byte[] code,
byte[] imgData,
int faceNum,
FutureCallbackcallback){
factory.addCallback(compareFaces(code,imgData,faceNum), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#compareFeatures(byte[],java.util.List)}
*/
public ListenableFuture> compareFeatures(byte[] code1,
List codes){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture> future = async.compareFeatures(code1,
codes);
return factory.wrap(async,future);
}
public void compareFeatures(byte[] code1,
List codes,
FutureCallback>callback){
factory.addCallback(compareFeatures(code1,codes), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#detectAndCompare2Face(byte[],net.gdface.sdk.FRect,byte[],net.gdface.sdk.FRect)}
*/
public ListenableFuture detectAndCompare2Face(byte[] imgData1,
FRect detectRect1,
byte[] imgData2,
FRect detectRect2){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = async.detectAndCompare2Face(imgData1,
TypeTransformer.getInstance().to(
detectRect1,
FRect.class,
net.gdface.sdk.thrift.client.FRect.class),
imgData2,
TypeTransformer.getInstance().to(
detectRect2,
FRect.class,
net.gdface.sdk.thrift.client.FRect.class));
return factory.wrap(async,future);
}
public void detectAndCompare2Face(byte[] imgData1,
FRect detectRect1,
byte[] imgData2,
FRect detectRect2,
FutureCallbackcallback){
factory.addCallback(detectAndCompare2Face(imgData1,detectRect1,imgData2,detectRect2), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#detectAndGetCodeInfo(byte[],int)}
*/
public ListenableFuture detectAndGetCodeInfo(byte[] imgData,
int faceNum){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.detectAndGetCodeInfo(imgData,
faceNum),
new com.google.common.base.Function,CodeInfo[]>(){
@Override
public CodeInfo[] apply(java.util.List input) {
return TypeTransformer.getInstance().toArray(
input,
net.gdface.sdk.thrift.client.CodeInfo.class,
CodeInfo.class);
}
});
return factory.wrap(async,future);
}
public void detectAndGetCodeInfo(byte[] imgData,
int faceNum,
FutureCallbackcallback){
factory.addCallback(detectAndGetCodeInfo(imgData,faceNum), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#detectCenterFace(byte[])}
*/
public ListenableFuture detectCenterFace(byte[] imgData){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.detectCenterFace(imgData),
new com.google.common.base.Function(){
@Override
public CodeInfo apply(net.gdface.sdk.thrift.client.CodeInfo input) {
return TypeTransformer.getInstance().to(
input,
net.gdface.sdk.thrift.client.CodeInfo.class,
CodeInfo.class);
}
});
return factory.wrap(async,future);
}
public void detectCenterFace(byte[] imgData,
FutureCallbackcallback){
factory.addCallback(detectCenterFace(imgData), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#detectFace(byte[])}
*/
public ListenableFuture detectFace(byte[] imgData){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.detectFace(imgData),
new com.google.common.base.Function,CodeInfo[]>(){
@Override
public CodeInfo[] apply(java.util.List input) {
return TypeTransformer.getInstance().toArray(
input,
net.gdface.sdk.thrift.client.CodeInfo.class,
CodeInfo.class);
}
});
return factory.wrap(async,future);
}
public void detectFace(byte[] imgData,
FutureCallbackcallback){
factory.addCallback(detectFace(imgData), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#detectMaxFace(byte[])}
*/
public ListenableFuture detectMaxFace(byte[] imgData){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.detectMaxFace(imgData),
new com.google.common.base.Function(){
@Override
public CodeInfo apply(net.gdface.sdk.thrift.client.CodeInfo input) {
return TypeTransformer.getInstance().to(
input,
net.gdface.sdk.thrift.client.CodeInfo.class,
CodeInfo.class);
}
});
return factory.wrap(async,future);
}
public void detectMaxFace(byte[] imgData,
FutureCallbackcallback){
factory.addCallback(detectMaxFace(imgData), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#detectMaxFaceAndGetCodeInfo(byte[])}
*/
public ListenableFuture detectMaxFaceAndGetCodeInfo(byte[] imgData){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.detectMaxFaceAndGetCodeInfo(imgData),
new com.google.common.base.Function(){
@Override
public CodeInfo apply(net.gdface.sdk.thrift.client.CodeInfo input) {
return TypeTransformer.getInstance().to(
input,
net.gdface.sdk.thrift.client.CodeInfo.class,
CodeInfo.class);
}
});
return factory.wrap(async,future);
}
public void detectMaxFaceAndGetCodeInfo(byte[] imgData,
FutureCallbackcallback){
factory.addCallback(detectMaxFaceAndGetCodeInfo(imgData), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#getCodeInfo(byte[],int,net.gdface.sdk.CodeInfo[])}
*/
public ListenableFuture getCodeInfo(byte[] imgData,
int faceNum,
CodeInfo[] facePos){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.getCodeInfo(imgData,
faceNum,
TypeTransformer.getInstance().to(
facePos,
CodeInfo.class,
net.gdface.sdk.thrift.client.CodeInfo.class)),
new com.google.common.base.Function,CodeInfo[]>(){
@Override
public CodeInfo[] apply(java.util.List input) {
return TypeTransformer.getInstance().toArray(
input,
net.gdface.sdk.thrift.client.CodeInfo.class,
CodeInfo.class);
}
});
return factory.wrap(async,future);
}
public void getCodeInfo(byte[] imgData,
int faceNum,
CodeInfo[] facePos,
FutureCallbackcallback){
factory.addCallback(getCodeInfo(imgData,faceNum,facePos), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#getCodeInfo(byte[],net.gdface.sdk.CodeInfo)}
*/
public ListenableFuture getCodeInfo(byte[] imgData,
CodeInfo facePos){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.getCodeInfoSingle(imgData,
TypeTransformer.getInstance().to(
facePos,
CodeInfo.class,
net.gdface.sdk.thrift.client.CodeInfo.class)),
new com.google.common.base.Function(){
@Override
public CodeInfo apply(net.gdface.sdk.thrift.client.CodeInfo input) {
return TypeTransformer.getInstance().to(
input,
net.gdface.sdk.thrift.client.CodeInfo.class,
CodeInfo.class);
}
});
return factory.wrap(async,future);
}
public void getCodeInfo(byte[] imgData,
CodeInfo facePos,
FutureCallbackcallback){
factory.addCallback(getCodeInfo(imgData,facePos), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#getFeature(java.util.Map)}
*/
public ListenableFuture getFeature(Map faces){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = async.getFeature(TypeTransformer.getInstance().to(
faces,
ByteBuffer.class,
CodeInfo.class,
byte[].class,
net.gdface.sdk.thrift.client.CodeInfo.class));
return factory.wrap(async,future);
}
public void getFeature(Map faces,
FutureCallbackcallback){
factory.addCallback(getFeature(faces), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#hasFace(byte[])}
*/
public ListenableFuture hasFace(byte[] imgData){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = async.hasFace(imgData);
return factory.wrap(async,future);
}
public void hasFace(byte[] imgData,
FutureCallbackcallback){
factory.addCallback(hasFace(imgData), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#isLocal()}
*/
public boolean isLocal(){
return false;
}
/**
* see also {@link net.gdface.sdk.FaceApi#matDetectAndGetCodeInfo(net.gdface.image.MatType,byte[],int,int)}
*/
public ListenableFuture matDetectAndGetCodeInfo(MatType matType,
byte[] matData,
int width,
int height){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.matDetectAndGetCodeInfoUnchecked(TypeTransformer.getInstance().to(
matType,
MatType.class,
net.gdface.sdk.thrift.client.MatType.class),
matData,
width,
height),
new com.google.common.base.Function,CodeInfo[]>(){
@Override
public CodeInfo[] apply(java.util.List input) {
return TypeTransformer.getInstance().toArray(
input,
net.gdface.sdk.thrift.client.CodeInfo.class,
CodeInfo.class);
}
});
return factory.wrap(async,future);
}
public void matDetectAndGetCodeInfo(MatType matType,
byte[] matData,
int width,
int height,
FutureCallbackcallback){
factory.addCallback(matDetectAndGetCodeInfo(matType,matData,width,height), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#matDetectAndGetCodeInfo(net.gdface.image.MatType,byte[],int,int,int)}
*/
public ListenableFuture matDetectAndGetCodeInfo(MatType matType,
byte[] matData,
int width,
int height,
int faceNum){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.matDetectAndGetCodeInfo(TypeTransformer.getInstance().to(
matType,
MatType.class,
net.gdface.sdk.thrift.client.MatType.class),
matData,
width,
height,
faceNum),
new com.google.common.base.Function,CodeInfo[]>(){
@Override
public CodeInfo[] apply(java.util.List input) {
return TypeTransformer.getInstance().toArray(
input,
net.gdface.sdk.thrift.client.CodeInfo.class,
CodeInfo.class);
}
});
return factory.wrap(async,future);
}
public void matDetectAndGetCodeInfo(MatType matType,
byte[] matData,
int width,
int height,
int faceNum,
FutureCallbackcallback){
factory.addCallback(matDetectAndGetCodeInfo(matType,matData,width,height,faceNum), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#matDetectFace(net.gdface.image.MatType,byte[],int,int)}
*/
public ListenableFuture matDetectFace(MatType matType,
byte[] matData,
int width,
int height){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.matDetectFace(TypeTransformer.getInstance().to(
matType,
MatType.class,
net.gdface.sdk.thrift.client.MatType.class),
matData,
width,
height),
new com.google.common.base.Function,CodeInfo[]>(){
@Override
public CodeInfo[] apply(java.util.List input) {
return TypeTransformer.getInstance().toArray(
input,
net.gdface.sdk.thrift.client.CodeInfo.class,
CodeInfo.class);
}
});
return factory.wrap(async,future);
}
public void matDetectFace(MatType matType,
byte[] matData,
int width,
int height,
FutureCallbackcallback){
factory.addCallback(matDetectFace(matType,matData,width,height), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#matDetectFace(net.gdface.image.MatType,byte[],int,int,int)}
*/
public ListenableFuture matDetectFace(MatType matType,
byte[] matData,
int width,
int height,
int facenum){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.matDetectFaceFacenum(TypeTransformer.getInstance().to(
matType,
MatType.class,
net.gdface.sdk.thrift.client.MatType.class),
matData,
width,
height,
facenum),
new com.google.common.base.Function,CodeInfo[]>(){
@Override
public CodeInfo[] apply(java.util.List input) {
return TypeTransformer.getInstance().toArray(
input,
net.gdface.sdk.thrift.client.CodeInfo.class,
CodeInfo.class);
}
});
return factory.wrap(async,future);
}
public void matDetectFace(MatType matType,
byte[] matData,
int width,
int height,
int facenum,
FutureCallbackcallback){
factory.addCallback(matDetectFace(matType,matData,width,height,facenum), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#matDetectMaxFace(net.gdface.image.MatType,byte[],int,int)}
*/
public ListenableFuture matDetectMaxFace(MatType matType,
byte[] matData,
int width,
int height){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.matDetectMaxFace(TypeTransformer.getInstance().to(
matType,
MatType.class,
net.gdface.sdk.thrift.client.MatType.class),
matData,
width,
height),
new com.google.common.base.Function(){
@Override
public CodeInfo apply(net.gdface.sdk.thrift.client.CodeInfo input) {
return TypeTransformer.getInstance().to(
input,
net.gdface.sdk.thrift.client.CodeInfo.class,
CodeInfo.class);
}
});
return factory.wrap(async,future);
}
public void matDetectMaxFace(MatType matType,
byte[] matData,
int width,
int height,
FutureCallbackcallback){
factory.addCallback(matDetectMaxFace(matType,matData,width,height), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#matDetectMaxFaceAndGetCodeInfo(net.gdface.image.MatType,byte[],int,int)}
*/
public ListenableFuture matDetectMaxFaceAndGetCodeInfo(MatType matType,
byte[] matData,
int width,
int height){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.matDetectMaxFaceAndGetCodeInfo(TypeTransformer.getInstance().to(
matType,
MatType.class,
net.gdface.sdk.thrift.client.MatType.class),
matData,
width,
height),
new com.google.common.base.Function(){
@Override
public CodeInfo apply(net.gdface.sdk.thrift.client.CodeInfo input) {
return TypeTransformer.getInstance().to(
input,
net.gdface.sdk.thrift.client.CodeInfo.class,
CodeInfo.class);
}
});
return factory.wrap(async,future);
}
public void matDetectMaxFaceAndGetCodeInfo(MatType matType,
byte[] matData,
int width,
int height,
FutureCallbackcallback){
factory.addCallback(matDetectMaxFaceAndGetCodeInfo(matType,matData,width,height), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#matGetCodeInfo(net.gdface.image.MatType,byte[],int,int,int,net.gdface.sdk.CodeInfo[])}
*/
public ListenableFuture matGetCodeInfo(MatType matType,
byte[] matData,
int width,
int height,
int facenum,
CodeInfo[] facePos){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.matGetCodeInfo(TypeTransformer.getInstance().to(
matType,
MatType.class,
net.gdface.sdk.thrift.client.MatType.class),
matData,
width,
height,
facenum,
TypeTransformer.getInstance().to(
facePos,
CodeInfo.class,
net.gdface.sdk.thrift.client.CodeInfo.class)),
new com.google.common.base.Function,CodeInfo[]>(){
@Override
public CodeInfo[] apply(java.util.List input) {
return TypeTransformer.getInstance().toArray(
input,
net.gdface.sdk.thrift.client.CodeInfo.class,
CodeInfo.class);
}
});
return factory.wrap(async,future);
}
public void matGetCodeInfo(MatType matType,
byte[] matData,
int width,
int height,
int facenum,
CodeInfo[] facePos,
FutureCallbackcallback){
factory.addCallback(matGetCodeInfo(matType,matData,width,height,facenum,facePos), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#matGetCodeInfo(net.gdface.image.MatType,byte[],int,int,net.gdface.sdk.CodeInfo)}
*/
public ListenableFuture matGetCodeInfo(MatType matType,
byte[] matData,
int width,
int height,
CodeInfo facePos){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.matGetCodeInfoSingle(TypeTransformer.getInstance().to(
matType,
MatType.class,
net.gdface.sdk.thrift.client.MatType.class),
matData,
width,
height,
TypeTransformer.getInstance().to(
facePos,
CodeInfo.class,
net.gdface.sdk.thrift.client.CodeInfo.class)),
new com.google.common.base.Function(){
@Override
public CodeInfo apply(net.gdface.sdk.thrift.client.CodeInfo input) {
return TypeTransformer.getInstance().to(
input,
net.gdface.sdk.thrift.client.CodeInfo.class,
CodeInfo.class);
}
});
return factory.wrap(async,future);
}
public void matGetCodeInfo(MatType matType,
byte[] matData,
int width,
int height,
CodeInfo facePos,
FutureCallbackcallback){
factory.addCallback(matGetCodeInfo(matType,matData,width,height,facePos), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#matHasFace(net.gdface.image.MatType,byte[],int,int)}
*/
public ListenableFuture matHasFace(MatType matType,
byte[] matData,
int width,
int height){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = async.matHasFace(TypeTransformer.getInstance().to(
matType,
MatType.class,
net.gdface.sdk.thrift.client.MatType.class),
matData,
width,
height);
return factory.wrap(async,future);
}
public void matHasFace(MatType matType,
byte[] matData,
int width,
int height,
FutureCallbackcallback){
factory.addCallback(matHasFace(matType,matData,width,height), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#matSearchFaces(net.gdface.image.MatType,byte[],int,int,net.gdface.sdk.CodeInfo,double,int,java.lang.String[],int)}
*/
public ListenableFuture matSearchFaces(MatType matType,
byte[] matData,
int width,
int height,
CodeInfo facePos,
double similarty,
int rows,
String[] imgMD5Set,
int group){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = com.google.common.util.concurrent.Futures.transform(
async.matSearchFaces(TypeTransformer.getInstance().to(
matType,
MatType.class,
net.gdface.sdk.thrift.client.MatType.class),
matData,
width,
height,
TypeTransformer.getInstance().to(
facePos,
CodeInfo.class,
net.gdface.sdk.thrift.client.CodeInfo.class),
similarty,
rows,
TypeTransformer.getInstance().to(
imgMD5Set,
String.class,
String.class),
group),
new com.google.common.base.Function,FseResult[]>(){
@Override
public FseResult[] apply(java.util.List input) {
return TypeTransformer.getInstance().toArray(
input,
net.gdface.sdk.thrift.client.FseResult.class,
FseResult.class);
}
});
return factory.wrap(async,future);
}
public void matSearchFaces(MatType matType,
byte[] matData,
int width,
int height,
CodeInfo facePos,
double similarty,
int rows,
String[] imgMD5Set,
int group,
FutureCallbackcallback){
factory.addCallback(matSearchFaces(matType,matData,width,height,facePos,similarty,rows,imgMD5Set,group), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#matWearMask(net.gdface.image.MatType,byte[],int,int,net.gdface.sdk.CodeInfo)}
*/
public ListenableFuture matWearMask(MatType matType,
byte[] matData,
int width,
int height,
CodeInfo faceInfo){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = async.matWearMask(TypeTransformer.getInstance().to(
matType,
MatType.class,
net.gdface.sdk.thrift.client.MatType.class),
matData,
width,
height,
TypeTransformer.getInstance().to(
faceInfo,
CodeInfo.class,
net.gdface.sdk.thrift.client.CodeInfo.class));
return factory.wrap(async,future);
}
public void matWearMask(MatType matType,
byte[] matData,
int width,
int height,
CodeInfo faceInfo,
FutureCallbackcallback){
factory.addCallback(matWearMask(matType,matData,width,height,faceInfo), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#sdkCapacity()}
*/
public ListenableFuture
© 2015 - 2025 Weber Informatics LLC | Privacy Policy