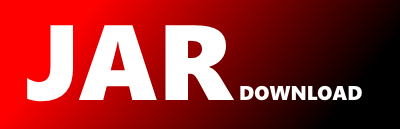
net.gdface.sdk.fse.thrift.FeatureSeThriftClient Maven / Gradle / Ivy
The newest version!
// ______________________________________________________
// Generated by codegen - https://gitee.com/l0km/codegen
// template: thrift/client/perservice/client.service.decorator.class.vm
// ______________________________________________________
package net.gdface.sdk.fse.thrift;
import net.gdface.sdk.fse.CodeBean;
import net.gdface.sdk.fse.FeatureSe;
import com.gitee.l0km.xthrift.thrift.ClientFactory;
import com.gitee.l0km.xthrift.thrift.TypeTransformer;
import com.facebook.swift.service.RuntimeTApplicationException;
import com.google.common.net.HostAndPort;
import static com.google.common.base.Preconditions.*;
/**
* 基于thrift/swift框架生成的client端代码提供{@link FeatureSe}接口的RPC实现(线程安全)
* 转发所有{@link FeatureSe}接口方法到{@link #delegate()}指定的实例
* 所有服务端抛出的{@link RuntimeException}异常被封装到{@link ServiceRuntimeException}中抛出
* Example:
*
* FeatureSeThriftClient thriftInstance = ClientFactory
* .builder()
* .setHostAndPort("127.0.0.1",26413)
* .build(FeatureSe.class, FeatureSeThriftClient.class);
*
* 计算机生成代码(generated by automated tools ThriftServiceDecoratorGenerator @author guyadong)
* @author guyadong
*
*/
public class FeatureSeThriftClient implements FeatureSe {
private final ClientFactory factory;
public ClientFactory getFactory() {
return factory;
}
public FeatureSeThriftClient(ClientFactory factory) {
super();
this.factory = checkNotNull(factory,"factory is null");
}
/**
* @param host RPC service host
* @param port RPC service port
*/
public FeatureSeThriftClient(String host,int port) {
this(ClientFactory.builder().setHostAndPort(host,port));
}
/**
* @param hostAndPort RPC service host and port
*/
public FeatureSeThriftClient(HostAndPort hostAndPort) {
this(ClientFactory.builder().setHostAndPort(hostAndPort));
}
/**
* test if connectable for RPC service
* @return return {@code true} if connectable ,otherwise {@code false}
*/
public boolean testConnect(){
return factory.testConnect();
}
/**
* @return 返回{@link net.gdface.sdk.fse.thrift.client.FeatureSe}实例
*/
protected net.gdface.sdk.fse.thrift.client.FeatureSe delegate() {
return factory.applyInstance(net.gdface.sdk.fse.thrift.client.FeatureSe.class);
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append("FeatureSeThriftClient [factory=");
builder.append(factory);
builder.append(",interface=");
builder.append(FeatureSe.class.getName());
builder.append("]");
return builder.toString();
}
@Override
public boolean addFeature(byte[] featureId,
byte[] feature,
String imgMD5,
int group)
{
net.gdface.sdk.fse.thrift.client.FeatureSe instance = delegate();
try{
return instance.addFeatureToFse(featureId,
feature,
imgMD5,
group);
}
catch(net.gdface.sdk.fse.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean addFeature(byte[] featureId,
byte[] feature,
long appid,
int group)
{
net.gdface.sdk.fse.thrift.client.FeatureSe instance = delegate();
try{
return instance.addFeatureToFseWithAppId(featureId,
feature,
appid,
group);
}
catch(net.gdface.sdk.fse.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void clearAll()
{
net.gdface.sdk.fse.thrift.client.FeatureSe instance = delegate();
try{
instance.clearAllOfFse();
}
catch(net.gdface.sdk.fse.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public CodeBean getFeature(byte[] featureId)
{
net.gdface.sdk.fse.thrift.client.FeatureSe instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getFeatureFromFse(featureId),
net.gdface.sdk.fse.thrift.client.CodeBean.class,
CodeBean.class);
}
catch(net.gdface.sdk.fse.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public CodeBean getFeatureByHex(String featureId)
{
net.gdface.sdk.fse.thrift.client.FeatureSe instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getFeatureByHexFromFse(featureId),
net.gdface.sdk.fse.thrift.client.CodeBean.class,
CodeBean.class);
}
catch(net.gdface.sdk.fse.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean removeFeature(byte[] featureId)
{
net.gdface.sdk.fse.thrift.client.FeatureSe instance = delegate();
try{
return instance.removeFeatureFromFse(featureId);
}
catch(net.gdface.sdk.fse.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean removeFeatureByHex(String featureId)
{
net.gdface.sdk.fse.thrift.client.FeatureSe instance = delegate();
try{
return instance.removeFeatureByHexFromFse(featureId);
}
catch(net.gdface.sdk.fse.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public CodeBean[] searchCode(byte[] code,
double sim,
int rows,
String[] imgMD5Set,
int group)
{
net.gdface.sdk.fse.thrift.client.FeatureSe instance = delegate();
try{
return TypeTransformer.getInstance().toArray(
instance.searchCodeFromFse(code,
sim,
rows,
TypeTransformer.getInstance().to(
imgMD5Set,
String.class,
String.class),
group),
net.gdface.sdk.fse.thrift.client.CodeBean.class,
CodeBean.class);
}
catch(net.gdface.sdk.fse.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int size()
{
net.gdface.sdk.fse.thrift.client.FeatureSe instance = delegate();
try{
return instance.sizeOfFse();
}
catch(net.gdface.sdk.fse.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean updateGroup(byte[] featureId,
int group)
{
net.gdface.sdk.fse.thrift.client.FeatureSe instance = delegate();
try{
return instance.updateGroup(featureId,
group);
}
catch(net.gdface.sdk.fse.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy